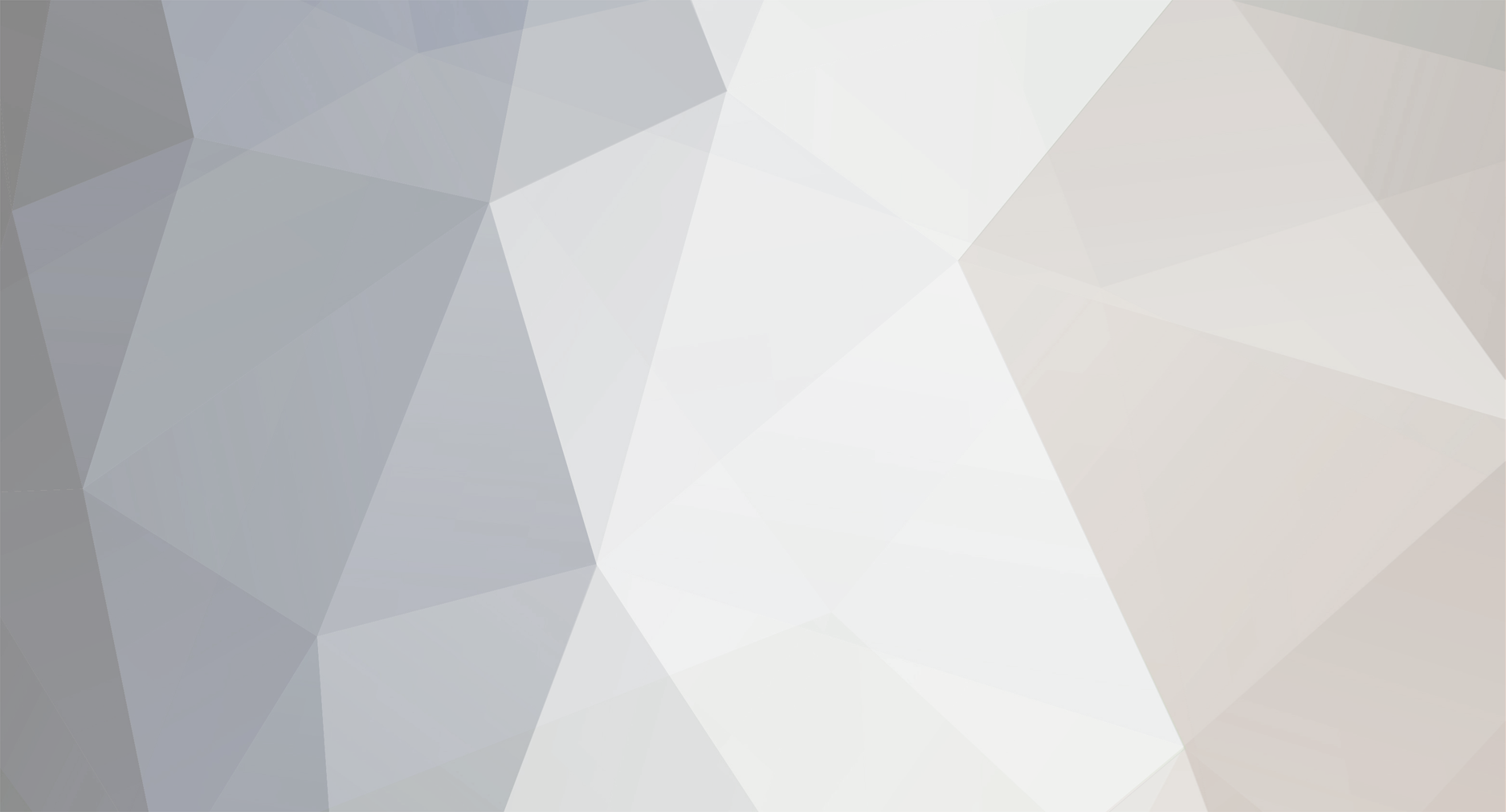
xyph
-
Posts
3,711 -
Joined
-
Last visited
-
Days Won
1
xyph's Achievements

Advanced Member (4/5)
12
12
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.