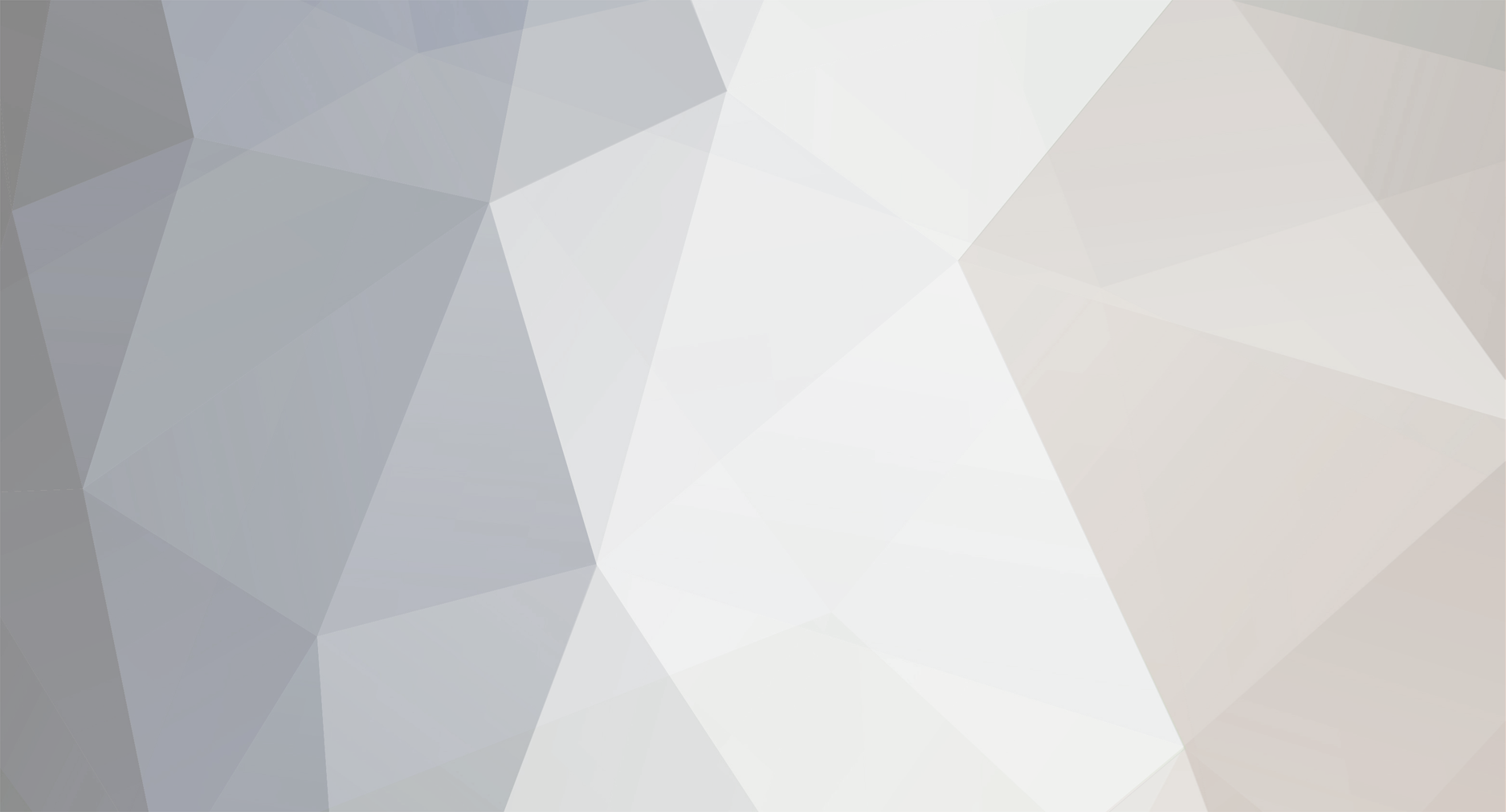
kgrenier
New Members-
Posts
1 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
kgrenier's Achievements

Newbie (1/5)
0
Reputation
-
I've created an html form for employee conference room requests which is validated & emailed via a php script "index_a.php". Some fields are required and some fields are optional. When the employee hits submit - index_a.php validates input, if there's an error - the user receives an error message. If it was successful the user gets a thank you message and 2 emails are sent (1 to the employee and 1 to my attention) containing all required and optional field information. I need to be able to strip out any BLANK OPTIONAL FIELDS in the 2 emails that are sent out. How do I do that? index_a.php code [pre]<?php //include("header.html"); // Receiving variables @$name = addslashes($_POST['name']); @$phone = addslashes($_POST['phone']); @$email = addslashes($_POST['email']); @$alternate_name = addslashes($_POST['alternate_name']); @$alternate_phone = addslashes($_POST['alternate_phone']); @$alternate_email = addslashes($_POST['alternate_email']); @$hostname = addslashes($_POST['hostname']); @$hostphone = addslashes($_POST['hostphone']); @$hostemail = addslashes($_POST['hostemail']); @$dept_name = addslashes($_POST['dept_name']); @$dept_other = addslashes($_POST['dept_other']); @$purpose_meeting = addslashes($_POST['purpose_meeting']); @$purpose_other = addslashes($_POST['purpose_other']); @$conference_room_selection = addslashes($_POST['conference_room_selection']); @$day_date = addslashes($_POST['day_date']); @$mtg_start_time = addslashes($_POST['mtg_start_time']); @$select_am_or_pm = addslashes($_POST['select_am_or_pm']); @$number_of_attendees = addslashes($_POST['number_of_attendees']); @$mtg_sign = addslashes($_POST['mtg_sign']); @$comments = addslashes($_POST['comments']); @$Additional_Whiteboard = addslashes($_POST['Additional_Whiteboard']); @$whiteboards_amt = addslashes($_POST['whiteboards_amt']); @$Easel_nonpostit = addslashes($_POST['Easel_nonpostit']); @$easel_amt = addslashes($_POST['easel_amt']); @$Easel_postit = addslashes($_POST['Easel_postit']); @$easel_postit_amt = addslashes($_POST['easel_postit_amt']); @$overhead_projector = addslashes($_POST['overhead_projector']); @$polycomphone = addslashes($_POST['polycomphone']); @$laptop = addslashes($_POST['laptop']); @$laptop_amt = addslashes($_POST['laptop_amt']); @$lcd_projector = addslashes($_POST['lcd_projector']); @$TV_VCR_DVD = addslashes($_POST['TV_VCR_DVD']); @$need_1_tvcombo = addslashes($_POST['need_1_tvcombo']); @$need_2_tvcombo = addslashes($_POST['need_2_tvcombo']); @$video_camera_tripod = addslashes($_POST['video_camera_tripod']); @$external_network_conn = addslashes($_POST['external_network_conn']); @$external_network_conn_amt = addslashes($_POST['external_network_conn_amt']); @$internal_network_conn = addslashes($_POST['internal_network_conn']); @$intnetworkconnamt = addslashes($_POST['intnetworkconnamt']); @$powerstrips = addslashes($_POST['powerstrips']); @$powerstrips_amt = addslashes($_POST['powerstrips_amt']); @$food = addslashes($_POST['food']); @$table_placement = addslashes($_POST['table_placement']); @$submitted = addslashes($_POST['submitted']); // Validation if (strlen($name) == 0 ) { die (include("answer/error_name.html")); } if (strlen($phone) <4) { die (include("answer/error_phone.html")); } if (strlen($phone) >25) { die (include("answer/error_phone.html")); } if (strlen($phone) == 0 ) { die (include("answer/error_phone.html")); } if (! ereg('[A-Za-z0-9_-]+\@[A-Za-z0-9_-]+\.[A-Za-z0-9_-]+', $email)) { die(include("answer/error_phone.html")); } if (strlen($email) == 0 ) { die(include("answer/error_phone.html")); } if (strlen($alternate_name) >=25) { die(include("answer/error_alternate_name.html")); } if (strlen($alternate_phone) <0) { die(include("answer/error_alternate_phone.html")); } if (strlen($alternate_phone) >25) { die(include("answer/error_alternate_phone.html")); } if (! ereg('[A-Za-z0-9_-]+\@[A-Za-z0-9_-]+\.[A-Za-z0-9_-]+', $alternate_email)) { die(include("answer/error_alternate_email.html")); } if (strlen($hostname) <4) { die(include("answer/error_host_name.html")); } if (strlen($hostname) >25) { die(include("answer/error_host_name.html")); } if (strlen($hostname) == 0 ) { die(include("answer/error_host_name.html")); } if (strlen($hostphone) <4) { die(include("answer/error_host_phone.html")); } if (strlen($hostphone) >25) { die(include("answer/error_host_phone.html")); } if (strlen($hostphone) == 0 ) { die(include("answer/error_host_phone.html")); } if (! ereg('[A-Za-z0-9_-]+\@[A-Za-z0-9_-]+\.[A-Za-z0-9_-]+', $hostemail)) { die(include("answer/error_host_email.html")); } if (strlen($hostemail) == 0 ) { die(include("answer/error_host_email.html")); } if (strlen($dept_name) <2) { die(include("answer/error_dept_name.html")); } if (strlen($dept_name) >45) { die(include("answer/error_dept_name.html")); } if (strlen($dept_name) == 0 ) { die(include("answer/error_dept_name.html")); } if (strlen($dept_other) <0) { die(include("answer/error_dept_other.html")); } if (strlen($dept_other) >25) { die(include("answer/error_dept_other.html")); } if (strlen($purpose_meeting) <4) { die(include("answer/error_purpose_meeting.html")); } if (strlen($purpose_meeting) >450) { die(include("answer/error_purpose_meeting.html")); } if (strlen($purpose_meeting) == 0 ) { die(include("answer/error_purpose_meeting.html")); } if (strlen($purpose_other) <0) { die(include("answer/error_purpose_meeting_other.html")); } if (strlen($purpose_other) >450) { die(include("answer/error_purpose_meeting_other.html")); } if (strlen($conference_room_selection) <2) { die(include("answer/error_conf_room_selection.html")); } if (strlen($conference_room_selection) >5) { die(include("answer/error_conf_room_selection.html")); } if (strlen($conference_room_selection) == 0 ) { die(include("answer/error_conf_room_selection.html")); } if (strlen($day_date) <4) { die(include("answer/error_day_date.html")); } if (strlen($day_date) >45) { die(include("answer/error_day_date.html")); } if (strlen($day_date) == 0 ) { die(include("answer/error_day_date.html")); } if (strlen($mtg_start_time) <2) { die(include("answer/error_meeting_start_time.html")); } if (strlen($mtg_start_time) >45) { die(include("answer/error_meeting_start_time.html")); } if (strlen($mtg_start_time) == 0 ) { die(include("answer/error_meeting_start_time.html")); } if (strlen($select_am_or_pm) !=2) { die(include("answer/error_meeting_am_pm.html")); } if (strlen($select_am_or_pm) == 0 ) { die(include("answer/error_meeting_am_pm.html")); } if (strlen($number_of_attendees) <1) { die(include("answer/error_attendees.html")); } if (strlen($number_of_attendees) >20) { die(include("answer/error_attendees.html")); } if (strlen($number_of_attendees) == 0 ) { die(include("answer/error_attendees.html")); } if (strlen($mtg_sign) <1) { die(include("answer/error_sign.html")); } if (strlen($mtg_sign) >100) { die(include("answer/error_sign.html")); } if (strlen($mtg_sign) == 0 ) { die(include("answer/error_sign.html")); } if (strlen($comments) >=450) { die(include("answer/error_comments.html")); } if (strlen($Additional_Whiteboard) >=25) { die(include("answer/error_addl_whiteboards.html")); } if (strlen($whiteboards_amt) >=25) { die(include("answer/error_addl_whiteboards_amt.html")); } if (strlen($Easel_nonpostit) >=25) { die(include("answer/error_easel_nonpostit.html")); } if (strlen($easel_amt) >=25) { die(include("answer/error_easel_nonpostit_amt.html")); } if (strlen($Easel_postit) >=25) { die(include("answer/error_easel_postit.html")); } if (strlen($easel_postit_amt) >=25) { die(include("answer/error_easel_postit_amt.html")); } if (strlen($overhead_projector) >=25) { die(include("answer/error_overhead_projector.html")); } if (strlen($polycomphone) >=25) { die(include("answer/error_polycom_phone.html")); } if (strlen($laptop) >=25) { die(include("answer/error_laptop.html")); } if (strlen($laptop_amt) >=25) { die(include("answer/error_laptop_amt.html")); } if (strlen($lcd_projector) >=25) { die(include("answer/error_lcd_projector.html")); } if (strlen($TV_VCR_DVD) >=25) { die(include("answer/error_tv_vcr_dvd_combo.html")); } if (strlen($need_1_tvcombo) >=25) { die(include("answer/error_tv_vcr_dvd_combo_need1.html")); } if (strlen($need_2_tvcombo) >=25) { die(include("answer/error_tv_vcr_dvd_combo_need2.html")); } if (strlen($video_camera_tripod) >=25) { die(include("answer/error_video_cam_tripod.html")); } if (strlen($external_network_conn) >=25) { die(include("answer/error_ext_network.html")); } if (strlen($external_network_conn_amt) >=25) { die(include("answer/error_ext_network_amt.html")); } if (strlen($intnetworkconnamt) >=25) { die(include("answer/error_int_network_amt.html")); } if (strlen($powerstrips) >=25) { die(include("answer/error_powerstrip.html")); } if (strlen($powerstrips_amt) >=25) { die(include("answer/error_powerstrip_amt.html")); } if (strlen($food) <0) { die(include("answer/error_food.html")); } if (strlen($food) >25) { die(include("answer/error_food.html")); } if (strlen($food) == 0 ) { die(include("answer/error_food.html")); } //Sending Email to form owner # Email to Owner $pfw_header = "From: $email"; $pfw_subject = "Iweb: Conference Room Request"; $pfw_email_to = "name@name.com"; $pfw_message = "An employee has requested a conference room.\n" . "Listed below are the contact information and items needed for this meeting.\n" . "\n" . "name: $name\n" . "phone: $phone\n" . "email: $email\n" . "\n" . "alternate_name: $alternate_name\n" . "alternate_phone: $alternate_phone\n" . "alternate_email: $alternate_email\n" . "\n" . "hostname: $hostname\n" . "hostphone: $hostphone\n" . "hostemail: $hostemail\n" . "\n" . "dept_name: $dept_name\n" . "dept_other: $dept_other\n" . "purpose_meeting: $purpose_meeting\n" . "purpose_other: $purpose_other\n" . "\n" . "conference_room_selection: $conference_room_selection\n" . "\n" . "day_date: $day_date\n" . "\n" . "mtg_start_time: $mtg_start_time\n" . "\n" . "select_am_or_pm: $select_am_or_pm\n" . "\n" . "number_of_attendees: $number_of_attendees\n" . "\n" . "mtg_sign: $mtg_sign\n" . "\n" . "comments: $comments\n" . "\n" . "\n" . "Additional_Whiteboard: $Additional_Whiteboard\n" . "\n" . "whiteboards_amt: $whiteboards_amt\n" . "\n" . "Easel_nonpostit: $Easel_nonpostit\n" . "\n" . "easel_amt: $easel_amt\n" . "\n" . "Easel_postit: $Easel_postit\n" . "\n" . "easel_postit_amt: $easel_postit_amt\n" . "\n" . "overhead_projector: $overhead_projector\n" . "\n" . "polycomphone: $polycomphone\n" . "\n" . "laptop: $laptop\n" . "\n" . "laptop_amt: $laptop_amt\n" . "\n" . "lcd_projector: $lcd_projector\n" . "\n" . "TV_VCR_DVD: $TV_VCR_DVD\n" . "\n" . "need_1_tvcombo: $need_1_tvcombo\n" . "\n" . "need_2_tvcombo: $need_2_tvcombo\n" . "\n" . "video_camera_tripod: $video_camera_tripod\n" . "\n" . "external_network_conn: $external_network_conn\n" . "\n" . "external_network_conn_amt: $external_network_conn_amt\n" . "\n" . "internal_network_conn: $internal_network_conn\n" . "\n" . "intnetworkconnamt: $intnetworkconnamt\n" . "\n" . "powerstrips: $powerstrips\n" . "\n" . "powerstrips_amt: $powerstrips_amt\n" . "\n" . "food: $food\n" . "\n" . "table_placement: $table_placement\n" . "\n" . "submitted: $submitted\n"; @mail($pfw_email_to, $pfw_subject ,$pfw_message ,$pfw_header ) ; //Sending auto respond Email to user # Email to Owner $pfw_header = "From: name@name.com"; $pfw_subject = "Your Conference Room Request "; $pfw_email_to = "$email"; $pfw_message = "Thank you for your conference room request.\n" . "\n" . "Your request has been sent to xyz@xyz.com\n" . "\n" . "You will be contacted to confirm that your room and items are available and have been reserved.\n" . "\n" . "If you need to contact someone right away - please email xyz@xyz.com\n" . "\n"; @mail($pfw_email_to, $pfw_subject ,$pfw_message ,$pfw_header ) ; //saving record in a text file $pfw_file_name = "conference_room_request.txt"; $pfw_first_raw = "name,phone,email,alternate_name,alternate_phone,alternate_email,hostname,hostphone,hostemail,dept_name,dept_other,purpose_meeting,purpose_other,conference_room_selection,day_date,mtg_start_time,select_am_or_pm,number_of_attendees,mtg_sign,comments,Additional_Whiteboard,whiteboards_amt,Easel_nonpostit,easel_amt,Easel_postit,easel_postit_amt,overhead_projector,polycomphone,laptop,laptop_amt,lcd_projector,TV_VCR_DVD,need_1_tvcombo,need_2_tvcombo,video_camera_tripod,external_network_conn,external_network_conn_amt,internal_network_conn,intnetworkconnamt,powerstrips,powerstrips_amt,food,table_placement,submitted\r\n"; $pfw_values = "$name,$phone,$email,$alternate_name,$alternate_phone,$alternate_email,$hostname,$hostphone,$hostemail,$dept_name,$dept_other,$purpose_meeting,$purpose_other,$conference_room_selection,$day_date,$mtg_start_time,$select_am_or_pm,$number_of_attendees,$mtg_sign,".str_replace ("\r\n","<BR>",$comments ).",$Additional_Whiteboard,$whiteboards_amt,$Easel_nonpostit,$easel_amt,$Easel_postit,$easel_postit_amt,$overhead_projector,$polycomphone,$laptop,$laptop_amt,$lcd_projector,$TV_VCR_DVD,$need_1_tvcombo,$need_2_tvcombo,$video_camera_tripod,$external_network_conn,$external_network_conn_amt,$internal_network_conn,$intnetworkconnamt,$powerstrips,$powerstrips_amt,$food,$table_placement,$submitted\r\n"; $pfw_is_first_row = false; if(!file_exists($pfw_file_name)) { $pfw_is_first_row = true ; } if (!$pfw_handle = fopen($pfw_file_name, 'a+')) { die("Cannot open file ($pfw_file_name)"); exit; } if ($pfw_is_first_row) { if (fwrite($pfw_handle, $pfw_first_raw ) === FALSE) { die("Cannot write to file ($pfw_filename)"); exit; } } if (fwrite($pfw_handle, $pfw_values) === FALSE) { die("Cannot write to file ($pfw_filename)"); exit; } fclose($pfw_handle); include("answer/success.html"); ?> [/pre] HTML Form "form_conf_room.html" [pre]<!doctype html public "-//w3c//dtd html 3.2 final//en"> <html> <head> <title> XYZ | Meeting Room Administration | Room Request Form</title> <meta name="author" content="name@name.com"> <meta name="keywords" content=""> <meta name="description" content="Room Request Form"> <script type="text/javascript"><!-- if(window.attachEvent) window.attachEvent("onload",setListeners); function setListeners(){ inputList = document.getElementsByTagName("INPUT"); for(i=0;i<inputList.length;i++){ inputList.attachEvent("onpropertychange",restoreStyles); inputList.style.backgroundColor = ""; } selectList = document.getElementsByTagName("SELECT"); for(i=0;i<selectList.length;i++){ selectList.attachEvent("onpropertychange",restoreStyles); selectList.style.backgroundColor = ""; } } function restoreStyles(){ if(event.srcElement.style.backgroundColor != "" && event.srcElement.style.backgroundColor != "#a0d0ff"){ event.srcElement.style.backgroundColor = "#a0d0ff"; /* color of choice for AutoFill */ document.all['googleblurb'].style.display = "block"; } }//--> </script> <!-- START HEADER --> <!--#include virtual="/header/index.html" --> <STYLE type="text/css"> td { font-family: Arial, Helvetica, sans-serif; font-size: 14px; color: #666666; } .style1 {color: #FFFFFF} .style3 {color: #FFFFFF; font-size: 16; } .style4 { font-size: 18px; font-weight: bold; } .style5 {font-size: 16px} .style6 { font-size: 12px; font-style: italic; } .style8 {font-size: 12; font-style: italic; } .style9 { font-size: 24px; font-weight: bold; } </STYLE> <body> <!-- END HEADER --> <!-- START EXTRA NAV BAR --> <table width="790" cellpadding="3" cellspacing="0" border="0" bgcolor="#ffffff"> <tr bgcolor="#ffffff"> <td width="680" align="left" valign="top"><font face="arial, helvetica, verdana, sans serif" size="1" color="#999999"> > <a href="index.html"><font color="#999999">Central Support Services/Facilities</a> > <a href="http://www.xyz.com/facilities/meeting.html">Meeting Room Administration</a> > Conference Room Setup & Request Form</td></tr> <tr bgcolor="#ffffff"> <td width="790" align="left" valign="top"><img src="../images/spacer/spacer.gif" width="1" height="1" border="0" alt="" /> </td> </tr> </table> <!-- END EXTRA NAV BAR --> <!-- START SECTION TITLE A --> <table width="790" cellpadding="0" cellspacing="0" border="0" bgcolor="#5b7ebd"> <tr bgcolor="#5b7ebd"> <td width="790" align="left" valign="top"><img src="../images/spacer/5b7ebd.gif" width="1" height="1" border="0" alt="" /> </td> </tr> </table> <table width="790" cellpadding="0" cellspacing="0" border="0" bgcolor="#5b7ebd"> <tr> <td width="30" align="left" valign="top" bgcolor="#5b7ebd"><img src="../images/spacer/5b7ebd.gif" width="30" height="1" border="0" alt="" /> </td> <td width="760" align="left" valign="top" bgcolor="#5b7ebd"> <table width="760" cellpadding="5" cellspacing="0" border="0" bgcolor="#5b7ebd"> <tr> <td width="760" align="left" valign="top" bgcolor="#5b7ebd"><font face="arial, helvetica, verdana, sans serif" size="3" color="#ffffff">Central Support Services/Facilities</td></tr> </table> </td> </tr> </table> <br> <!-- END SECTION TITLE A --> <!-- START MAIN BODY --> <!-- start side navigation include goes here --> <!-- end side navigation --> <!-- start page-topic title --> <table width="790" cellpadding="0" cellspacing="0" border="0" bgcolor="#5b7ebd"> <tr bgcolor="#5b7ebd"> <td width="790" align="left" valign="top"><img src="../images/spacer/5b7ebd.gif" width="1" height="1" border="0" alt="" /> </td> </tr> </table> <table width="790" cellpadding="0" cellspacing="0" border="0" bgcolor="#5b7ebd"> <tr> <td width="1" align="left" valign="top" bgcolor="#5b7ebd"><img src="../images/spacer/5b7ebd.gif" width="1" height="1" border="0" alt="" /> </td> <td width="20" align="left" valign="top" bgcolor="#5b7ebd"><img src="../images/spacer/5b7ebd.gif" width="1" height="1" border="0" alt="" /> </td> <td width="507" align="left" valign="top" bgcolor="#cccccc"><font face="arial, helvetica, verdana, sans serif" size="3" color="#000000"> Conference Room Setup & Request Form</td><td width="1" align="left" valign="top" bgcolor="#cccccc"><img src="../images/spacer/cccccc.gif" width="1" height="1" border="0" alt="" /> </td> <td width="1" align="left" valign="top" bgcolor="#cccccc"><img src="../images/spacer/cccccc.gif" width="1" height="1" border="0" alt="" /> </td> </tr> </table> <table width="790" cellpadding="0" cellspacing="0" border="0" bgcolor="#5b7ebd"> <tr bgcolor="#5b7ebd"> <td width="790" align="left" valign="top"><img src="../images/spacer/5b7ebd.gif" width="1" height="1" border="0" alt="" /> </td> </tr> </table> <br /> <!-- end page-title topic --> <table width="790" cellpadding="3" cellspacing="0" border="0"> <tr> <td width="790" align="left" valign="top"> <!-- start main content --> <div id="googleblurb" style="display:none;"> You can use the AutoFill function on the Google toolbar to fill out the highlighted fields on this form. <a href="http://toolbar.google.com/autofill_help.html" title="AutoFill Help Page">Learn more</a>.</div> <form method="post" action="http://www.xyz.com/projects/facilities_c/php/index_a.php"> <table border="1" cols="6" cellspacing="0 width="100%"> <!--first row--> <tr valign="top" bgcolor="#f0ecec"> <td width="15%">Requestor's Name: <input type="text" name="name" /></td> <td width="15%">Requestor's Phone: <input type="text" name="phone" /></td> <td width="5%">Requestor's Email:<br> <input type="text" name="email" /></td> </tr> <tr valign="top"> <td width="15%">Alternate Contact Name: <input type="text" name="alternate_name" /></td> <td width="15%">Alternate Phone:<br> <input type="text" name="alternate_phone" /></td> <td width="5%">Alternate Email:<br> <input type="text" name="alternate_email" /></td> </tr> <tr valign="top" bgcolor="#f0ecec"> <td width="15%">XYZ Host's Name: <input type="text" name="hostname" /></td> <td width="15%">Host Phone:<br> <input type="text" name="hostphone" /></td> <td width="5%">Host Email:<br> <input type="text" name="hostemail" /></td> </tr> <tr valign="top"> <td width="15%">Department Name: <input type="text" name="dept_name" /></td> <td width="15%">Other:<br> <input type="text" name="dept_other" /></td> <td align="left" rowspan="6" width="5%" bgcolor="#5b7ebd"><div align="left"><span class="style3"> <span class="style4">Questions?</span><br> <a href="mailto:xyz@xyz.com"><img src="centralsupport_email.gif" width="250" height="59" border="0"></a> <br> <strong>How To Submit Your Request:</strong> <br> #1-fill out this online form & hit "submit" at bottom of page<br> #2-or fill out MS Word Version form <br> <a href="http://www.xyz.com/docs/facilities/conference_room_request_form.doc">Click here to download form.</a><br> <br> <strong>Lead Times:</strong><br> Standard Setups: 2 business days<br> Setup w/Video Camera/Tripod: 4 business days<br> <br> <strong>Standard Set-ups/Room Configurations:</strong><br> information on standard setups - <a href="http://www.xyz.com/projects/facilities/form_conf_room_setup.html">click here</a><br> <br> <strong>Cancellations:</strong><br> #1-delete from Meeting Maker<br> #2-email <a href="mailto:xyz@xyz.com">xyz@xyz..com</a><br> <br> </span></div></td> </tr> <tr valign="top" bgcolor="#f0ecec"> <td width="15%">Purpose of Meeting: <input type="text" name="purpose_meeting" /></td> <td width="15%">Other:<br> <input type="text" name="purpose_other" /></td> </tr> <tr valign="top"> <td width="15%">Conference Room:<br> <select name="conference_room_selection"> <option value="6/702">6/702</option> <option value="6/670">6/670</option> <option value="6/666">6/666</option> <option value="6/638">6/638</option> <option value="3/123">3/123</option> <option value="2/267">2/267</option> <option value="1/174">1/174</option> <option value="Cafeteria">Cafeteria</option> </select></td> <td width="15%">Day and Date:<br> <input type="text" name="day_date" /></td> </tr> <tr valign="top" bgcolor="#f0ecec"> <td width="15%">Actual start time of Meeting<br> <span class="style6">(set-up will be completed no less than 1/2 hour prior to start time)</span>: <input type="text" name="mtg_start_time" /></td> <td width="15%">AM or PM? <br> <select name="select_am_or_pm"> <option value="AM">AM</option> <option value="PM">PM</option> </select></td> </tr> <tr valign="top"> <td width="15%">Total Number of Attendees: <input type="text" name="number_of_attendees" /></td> <td width="15%">Title of meeting for signage<br> (where applicable):<br> <input type="text" name="mtg_sign" /></td> </tr> <tr valign="top" bgcolor="#5b7ebd"> <td colspan="2" width="15%"><div align="left"> <span class="style1"><strong>Comments:</strong></span> <br> <br> <textarea name="comments" cols="35" rows="5"></textarea> </div></td> </tr> <tr valign="top" bgcolor="#5b7ebd"> <td colspan="3" width="15%"><div align="left"><br> <div align="center" class="style1 style5"><strong>Any Additional Equipment Needs: </strong></div><br> </div></td> </tr> <tr valign="top"> <td bgcolor="#f0ecec" width="15%"><div align="left"> <input name="Additional_Whiteboard" type="radio" value="Additional_Whiteboard"> <strong>Additional Whiteboards:</strong><br> Total number required: <input type="text" name="whiteboards_amt" /> </div></td> <td width="15%"><div align="left"> <input name="Easel_nonpostit" type="radio" value="Easel_nonpostit"> <strong>Easel w/flip chart (non Post-It type)/markers: </strong>(1 marker per easel supplied) <br> Total number Easels required: <input type="text" name="easel_amt" /> </div></td> <td bgcolor="#f0ecec" width="15%"><div align="left"> <input name="Easel_postit" type="radio" value="Easel_postit"> <strong>Easel w/flip chart (Post-It type)/markers</strong>: (1 marker per easel supplied) <br> Total number Easels required:<br> <input type="text" name="easel_postit_amt" /> </div></td> </tr> <tr valign="top"> <td width="15%" bgcolor="#f0ecec"><div align="left"> <input name="overhead_projector" type="radio" value="overhead_projector"> <strong>Overhead Projector </strong><br> <em>(for view graphs) </em></div></td> <td width="15%"><div align="left"> <input name="polycomphone" type="radio" value="polycomphone"> <strong>Polycom Phone</strong><br> <em>(Please note: you would need to provide your own Roll Call pin numbers) </em><em> </em> </div></td> <td bgcolor="#f0ecec" width="15%"><div align="left"> <input name="laptop" type="radio" value="laptop"> <strong>Laptop: </strong><em><br> (Please note: we have a limited number of laptops, they may not always be available). </em> <br> Total number laptops required:<br> <input type="text" name="laptop_amt" /> </div></td> </tr> <tr valign="top" bgcolor="#f0ecec"> </tr> <tr valign="top"> </tr> <tr valign="top" > <td bgcolor="#f0ecec" width="15%"><div align="left"> <input name="lcd_projector" type="radio" value="lcd_projector"> <strong>LCD Projector</strong> <br> </div></td> <td width="15%"><div align="left"> <input name="TV_VCR_DVD" type="radio" value="TV_VCR_DVD"> <strong> TV/VCR/DVD combo on cart: </strong><em>(we have two carts)</em>: </div> <label> <input name="need_1_tvcombo" type="radio" value="need_1_tvcombo"> Need 1 </label> <br> <label> <input name="need_2_tvcombo" type="radio" value="need_2_tvcombo"> Need 2 </label> </td> <td bgcolor="#f0ecec" width="15%"><div align="left"> <input name="video_camera_tripod" type="radio" value="video_camera_tripod"> <strong>Video Camera/Tripod</strong><em><br> (Please note: must be rented-requires minimum 4-business days advance notice) </em></div></td> </tr> <tr valign="top" bgcolor="#f0ecec"> <td></td> </tr> <tr valign="top"> <td bgcolor="#f0ecec" width="15%"><div align="left"> <input name="external_network_conn" type="radio" value="external_network_conn"> <strong>External Network Connection: </strong><span class="style8"><br> (Please note: must have 2-business days advance notice if more than one(1) connection is required). </span> <br> Total number of connections required:<br> <input type="text" name="external_network_conn_amt" /> </div></td> <td width="15%"><div align="left"> <input name="internal_network_conn" type="radio" value="internal_network_conn"> <strong>Internal Network Connection: </strong><span class="style8"><br> (Please note: must have 2-business days advance notice). </span><br> Total number of connections required:<br> <input type="text" name="intnetworkconnamt" /> </div></td> <td bgcolor="#f0ecec" width="15%"><div align="left"> <input name="powerstrips" type="radio" value="powerstrips"> <strong>Power Strips</strong>: <em><br> (*2-3 people per strip & need 2 business days advance notice) </em> <br> Total number of power strips required:<br> <input type="text" name="powerstrips_amt" /> </div></td> </tr> <tr valign="top" bgcolor="#5b7ebd" font="#ffffff"> <td colspan="3" width="15%"><div align="left"><br> <div align="center" class="style1 style9">Food Service </div> <br> </div></td> </tr> <tr valign="top" bgcolor="#5b7ebd""> <td colspan="2" width="15%"><div align="left"> <div align="center" class="style1"> <div align="left">Will you be ordering food for this event?: <select name="food"> <option value="yes_ordering_food">Yes</option> <option value="not_ordering_food">No</option> </select> </div> </div> </div></td> <td> <div align="left"><span class="style1">Placement of food tables:</span> <select name="table_placement"> <option value="food_tables_inside">Inside Room</option> <option value="food_tables_outside">Outside Room</option> </select> </div></td> </tr> <tr valign="top" bgcolor="#5b7ebd"> <td colspan="3" width="15%"><div align="left"> <div align="center" class="style1"> <br> Place your catering order at <a href="http://www.xyz.com/facilities/food.html">http://www.xyz.com/facilities/food.html</a><br> Email your food order directly to <a href="mailto:food@xyz.com">food@xyz.com</a></div><br> </div> </div></td> </tr> <tr valign="top"> <td colspan="2" width="15%"><div align="left"><strong>Items not supplied include, but are not limited to:</strong><br> Pointers - laser or regular<br> Pens/pencils/extra markers<br> Writing paper<br> Nametags, tent cards<br> Blank VHS tapes, CD's and DVD's (these can be ordered through NEOS)<br> Placing orders for food<br> </div></td> </tr> </table> <br /> <p><input name="submit" type="image" src="submit.gif"> <!--<input name="submit" type="submit" value="Submit" />old--> <input type="hidden" name="submitted" value="TRUE"> <br /> </p> </form> <!-- end main content --> </td> </tr> </table> <!-- END MAIN BODY --> <!-- START LAST_MODIFIED --> <!--#include virtual="/footer/last_mod_pre.txt" --> <!--#echo var="LAST_MODIFIED"--> <!--#include virtual="/footer/last_mod_post.txt" --> <!-- END LAST_MODIFIED --> <!-- START FOOTER --> <!--#include virtual="/footer/index.html" --> <!-- END FOOTER --> </body> </html> [/pre] Here's what the email message looks like - you can see that there are optional fields that contain no data-how do I get rid of it? [pre]Envelope-to: name@mail.xyz.com Date: Mon, 4 Feb 2008 11:51:51 -0500 To: name@xyz.com Subject: Iweb: Conference Room Request From: customer@xyz.com An employee has requested a conference room. Listed below are the contact information and items needed for this meeting. name: Jane Doe phone: ext. 33592333 email: customer@xyz.com alternate_name: Kerri Hohnlery alternate_phone: ext. 33344332 alternate_email: kHohnlery@xyz.com hostname: Ava Sklanod hostphone: ext. 3333333525 hostemail: asklanod@xyz.com dept_name: XYZ dept_other: n/a purpose_meeting: customer meeting purpose_other: n/a conference_room_selection: 6/666 day_date: Monday 3/10/08 mtg_start_time: 9:30 select_am_or_pm: AM number_of_attendees: 12 mtg_sign: Fitness Center Meeting comments: Additional_Whiteboard: Additional_Whiteboard whiteboards_amt: 1 Easel_nonpostit: Easel_nonpostit easel_amt: Easel_postit: Easel_postit easel_postit_amt: 3 overhead_projector: polycomphone: polycomphone laptop: laptop_amt: lcd_projector: lcd_projector TV_VCR_DVD: need_1_tvcombo: need_2_tvcombo: video_camera_tripod: external_network_conn: external_network_conn_amt: 5 internal_network_conn: intnetworkconnamt: powerstrips: powerstrips_amt: food: yes_ordering_food table_placement: food_tables_inside submitted: TRUE[/pre]