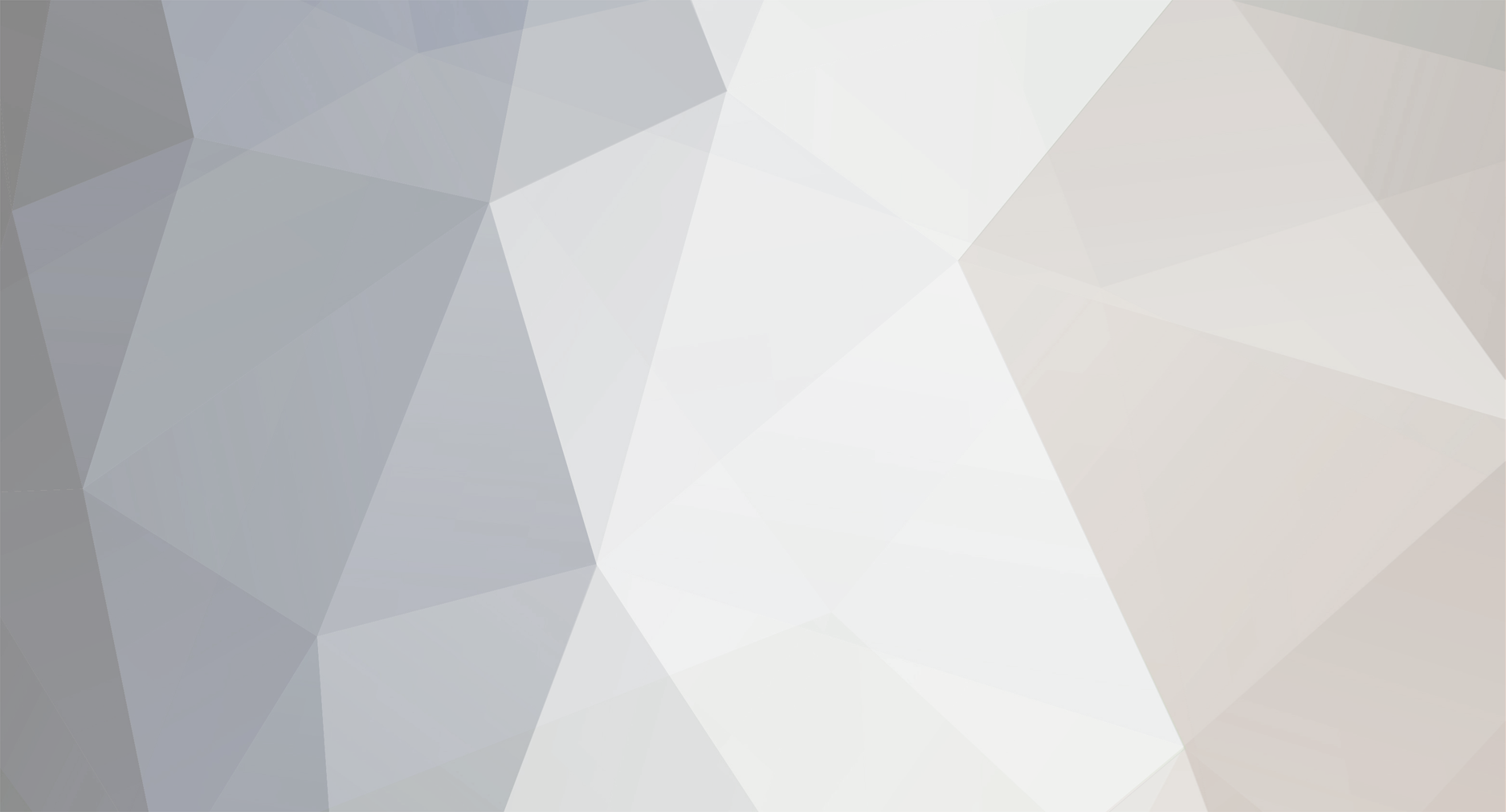
Brian_15
-
Posts
5 -
Joined
-
Last visited
Posts posted by Brian_15
-
-
Now its woking
<?php class Time_zone { protected $regions = array( 'Africa' => DateTimeZone::AFRICA, 'America' => DateTimeZone::AMERICA, 'Antarctica' => DateTimeZone::ANTARCTICA, 'Artic' => DateTimeZone::ARCTIC, 'Asia' => DateTimeZone::ASIA, 'Atlantic' => DateTimeZone::ATLANTIC, 'Australia' => DateTimeZone::AUSTRALIA, 'Europe' => DateTimeZone::EUROPE, 'Indian' => DateTimeZone::INDIAN, 'Pacific' => DateTimeZone::PACIFIC ); public function get_list() { $time_zones = array(); foreach ($this->regions as $name => $mask) { foreach(DateTimeZone::listIdentifiers($mask) as $time_zone) { $time_zones[$name][$time_zone] = '[UTC/GMT ' . $this->get_offset($time_zone) . '] ' . $time_zone; } } return $time_zones; } protected function get_offset($time_zone) { $_tz = new DateTimeZone($time_zone); $_dt = new DateTime('now', $_tz); return $_dt->format('P'); } }
<?php $time_zone = new Time_zone(); ?> <select> <?php foreach($time_zone->get_list() as $region => $list) { ?> <optgroup label="<?=$region?>"> <?php foreach($list as $time_zone => $name) { ?> <option value="<?=$time_zone?>"><?=$name?></option> <?php } ?> </optgroup> <?php } ?> </select>
Output
<select> <optgroup label="Africa"> <option value="Africa/Abidjan">[UTC/GMT +00:00] Africa/Abidjan</option> <option value="Africa/Accra">[UTC/GMT +00:00] Africa/Accra</option> ................................... </optgroup> <optgroup label="America"> <option value="America/Adak">[UTC/GMT -09:00] America/Adak</option> <option value="America/Anchorage">[UTC/GMT -08:00] America/Anchorage</option> ...................................
-
Testing code
$time_zone = new Time_zone(); ?> <select> <?php foreach($time_zone->generate_list() as $region => $list) { ?> <optgroup label="<?=$region?>"> <?php foreach($list as $name) { ?> <option value="<?=$name?>"><?=$name?></option> <?php } ?> </optgroup> <?php } ?> </select>
Current output
<option value="[UTC/GMT +00:00] Africa/Abidjan">[UTC/GMT +00:00] Africa/Abidjan</option>
Expected output
<option value="Africa/Abidjan">[UTC/GMT +00:00] Africa/Abidjan</option>
-
How do improve this class to output offset like + 02:00, - 02:00
What i want to achieve is this output: [ UTC/GMT - 11:00 ] - Timezone name
class Time_zone { private $regions = array( 'Africa' => DateTimeZone::AFRICA, 'America' => DateTimeZone::AMERICA, 'Antarctica' => DateTimeZone::ANTARCTICA, 'Artic' => DateTimeZone::ARCTIC, 'Asia' => DateTimeZone::ASIA, 'Atlantic' => DateTimeZone::ATLANTIC, 'Australia' => DateTimeZone::AUSTRALIA, 'Europe' => DateTimeZone::EUROPE, 'Indian' => DateTimeZone::INDIAN, 'Pacific' => DateTimeZone::PACIFIC ); public function generate_list() { $time_zones = array(); foreach ($this->regions as $name => $mask) { $time_zones[$name] = DateTimeZone::listIdentifiers($mask); } return $time_zones; } } $tz = new Time_zone(); ?> <pre><?=print_r($tz->generate_list());?></pre>
HTTP_ACCEPT_LANGUAGE en-US to en_US
in PHP Coding Help
Posted · Edited by Brian_15
Need help with modifying current code to work with en_US instead of en-US and so on.