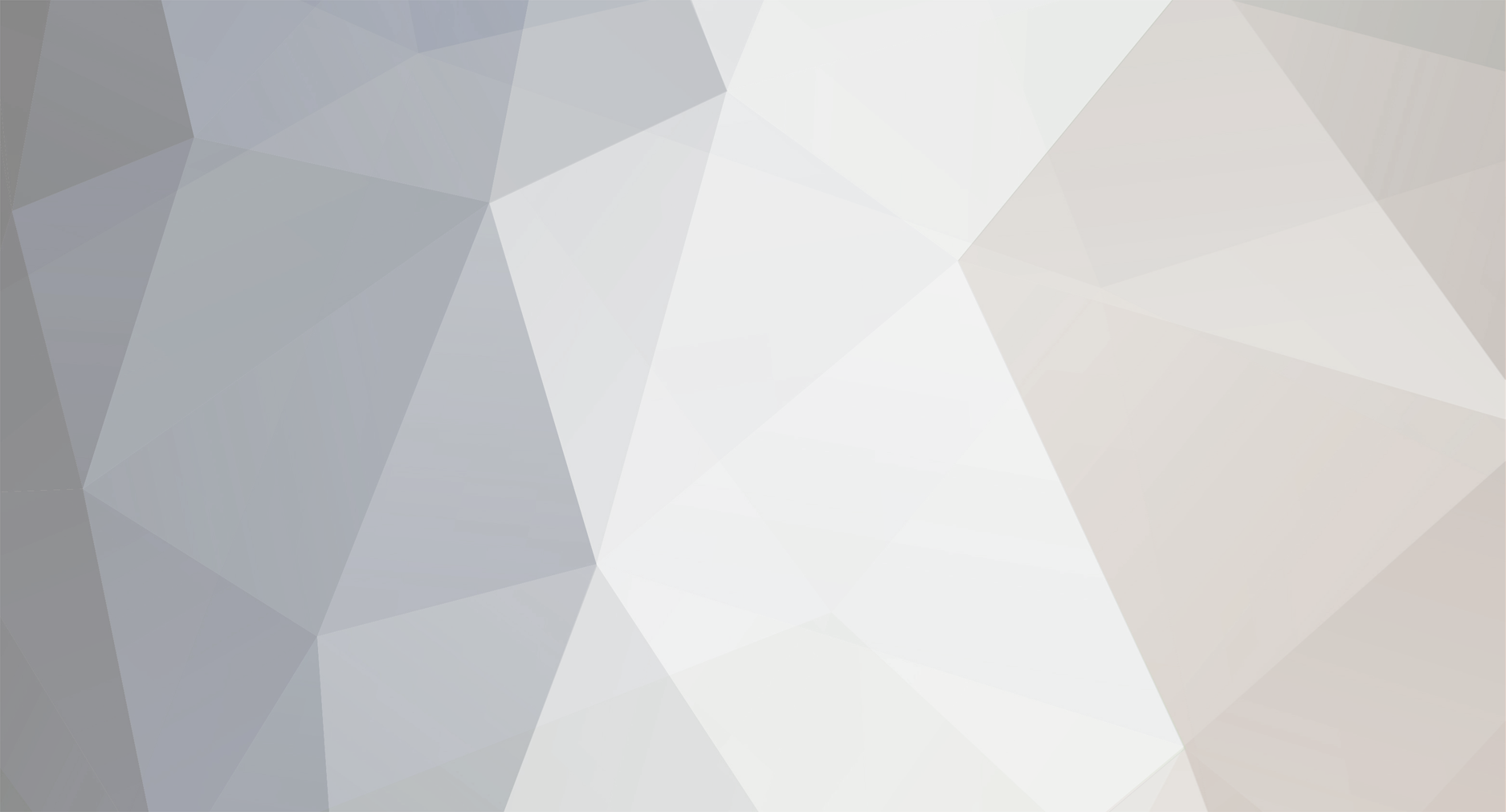
kproctor
Members-
Posts
17 -
Joined
-
Last visited
kproctor's Achievements
-
Alright, I'll try to explain better. Below is code that I put together with the help of google and fine folks on this form. This code goes to a website and gets information and stores the values in an array. I store the search values in a $_session variable. Problem: The form submits with the correct information the first time around, but when the user clicks the browser back button and selects a new value from a drop down and clicks submit the select options does not pass the correct select option values on the second submit. If the user clicks back again and changes nothing and submits the form again the values update. ** If I hit the refresh button after the second submit the field taking information from the drop down menu will update. The user form is saved in a separate file from the below code // Generates the select option lenderOptions = ""; //Iterate through the array of lenders foreach($lender_list as $value => $label) { //Trim the value $label = trim($label['name']); //If label is empty skip this record if(empty($label)) { continue; } //Create new option for the current lender $lenderOptions .= "<option value='{$value}'>{$label}</option>\n"; //////// HTML form info <select name="existing_FI" class="field-style field-full align-none" required> <option>Current FI</option> <?php echo $lenderOptions; ?> </select> $html = file_get_contents('https://www.website.com'); //get the html returned from the following url $lender_doc = new DOMDocument(); libxml_use_internal_errors(TRUE); //disable libxml errors if(!empty($html)){ //if any html is actually returned $lender_doc->loadHTML($html); libxml_clear_errors(); //remove errors for yucky html $lender_xpath = new DOMXPath($lender_doc); //get each tr from table $lender_row = $lender_xpath->query('//tr'); if($lender_row->length > 0){ foreach($lender_row as $row){ //echo $row->nodeValue . "<br/>"; } } } ?> <?php $lender_list = array(); $lender_and_type = $lender_xpath->query('//tr'); if($lender_and_type->length > 0){ //loop through all the lenders foreach($lender_and_type as $pat){ //get the name of the lender $name = $lender_xpath->query('td[@align="left"]', $pat)->item(0)->nodeValue; $fi_types = array(); //reset $fi_types for each lender $types = $lender_xpath->query('td', $pat); //loop through all the types and store them in the $fi_types array foreach($types as $type){ $fi_types[] = $type->nodeValue; //the lender type } //store the data in the $lender_list array $lender_list[] = array('name' => $name, 'types' => $fi_types); } } if(empty($_SESSION['existing_FI'])){ $fi_key_new = 0; }else{ // get current bank details $fi_key_exisiting = $_SESSION['existing_FI']; if (empty($fi_key_existing)) { $fi_key_existing = 0; } else { $fi_key_existing = $fi_key_existing; } $selected_lender = ($lender_list[$fi_key_exisiting]); foreach($selected_lender as $item) { $fi_name = $item[0]; $variable = $item[1]; $six_months = $item[2]; $one_year = $item[3]; $two_year = $item[4]; $three_year = $item[5]; $four_years = $item[6]; $five_years = $item[7]; } $comparisonRate = array('variable' =>$variable, '6' =>$six_months, '12' =>$one_year, '24'=>$two_year, '36'=>$three_year, '48'=>$four_years, '60'=>$five_years); } ?> <?php if(empty($_SESSION['new_FI'])){ $fi_key_new = 0; }else{ // get new bank details $fi_key_new = $_SESSION['new_FI']; if (empty($fi_key_new)) { $fi_key_new = 0; } else { $fi_key_new = $fi_key_new; } $selected_lender = ($lender_list[$fi_key_new]); foreach($selected_lender as $item) { $new_fi_name = $item[0]; $new_variable = $item[1]; $new_six_months = $item[2]; $new_one_year = $item[3]; $new_two_year = $item[4]; $new_three_year = $item[5]; $new_four_years = $item[6]; $new_five_years = $item[7]; } $newComparisonRate = array('variable' =>$new_variable, '6' =>$new_six_months, '12' =>$new_one_year, '24'=>$new_two_year, '36'=>$new_three_year, '48'=>$new_four_years, '60'=>$new_five_years); } ?>
-
It is coming from a function that scrapes another website. If my logic is off, why is it working?
-
Hi, Below is two blocks of code that I have been trying to convert to a function. Both sections are very similar. The below code does work. // get current bank details $fi_key_exisiting = $_SESSION['existing_FI']; if (empty($fi_key_existing)) { $fi_key_existing = 0; } else { $fi_key_existing = $fi_key_existing; } $selected_lender = ($lender_list[$fi_key_exisiting]); foreach($selected_lender as $item) { $fi_name = $item[0]; $variable = $item[1]; $six_months = $item[2]; $one_year = $item[3]; $two_year = $item[4]; $three_year = $item[5]; $four_years = $item[6]; $five_years = $item[7]; } $comparisonRate = array('variable' =>$variable, '6' =>$six_months, '12' =>$one_year, '24'=>$two_year, '36'=>$three_year, '48'=>$four_years, '60'=>$five_years); ?> <?php // get new bank details $fi_key_new = $_SESSION['new_FI']; if (empty($fi_key_new)) { $fi_key_new = 0; } else { $fi_key_new = $fi_key_new; } $selected_lender = ($lender_list[$fi_key_new]); foreach($selected_lender as $item) { $new_fi_name = $item[0]; $new_variable = $item[1]; $new_six_months = $item[2]; $new_one_year = $item[3]; $new_two_year = $item[4]; $new_three_year = $item[5]; $new_four_years = $item[6]; $new_five_years = $item[7]; } $newComparisonRate = array('variable' =>$new_variable, '6' =>$new_six_months, '12' =>$new_one_year, '24'=>$new_two_year, '36'=>$new_three_year, '48'=>$new_four_years, '60'=>$new_five_years);
-
I got it to work.. I had a typo....
-
I have the below code that is returning the following error: Warning: Invalid argument supplied for foreach() in /Library/WebServer/Documents/lessons101/scrapping.php on line 67 7 The number 7 is the result of the echo statement. I did this to confirm that a value is being passed. When I replace the $fi_key_exisiting variable in the for each statement the correct result is returned. I have no idea what's causing the error. Thoughts please. $fi_key_exisiting = $_SESSION['existing_fi']; if (empty($fi_key_existing)) { $fi_key_existing = 0; } else { $fi_key_existing = $fi_key_existing; } echo $fi_key_exisiting; $selected_lender = ($lender_list[$fi_key_exisiting]); foreach($selected_lender as $item) { $fi_name = $item[0]; $variable = $item[1]; $six_months = $item[2]; $one_year = $item[3]; $two_year = $item[4]; $three_year = $item[5]; $four_years = $item[6]; $five_years = $item[7]; }
-
Thank you for the reply. I am getting this error message. Warning: trim() expects parameter 1 to be string, array given in /Library/WebServer/Documents/lessons101/SwitchCalculator.php on line 33 Question relating to best practice. The form I am creating is big with lots of functions. What is the recommended lengh of code before it should be set in a separate file and then use the include function.
-
from a website. I have made some progress. Here is what I have now. I like the dropdown list idea. The only problem I have to solve now is to remove the blank keys. <?php echo "<select>"; $lender_list = ($lender_list); foreach($lender_list as $key => $value) { echo '<option value="'. $key. '">' .$value['name'] . '</option>'; } echo "</select>"; ?>
-
HI, The below code returns these results all the way to 44: 0 1 2 3ATB Financial 4Alterna Bank 5Alterna Savings 6Bank of Montreal 7Bank of Nova Scotia 8CIBC Mortgages 9Caisses Desjardins ... 44 There are two things I need to do 1. Remove the blank keys - 0,1,2,44 2. I want to assign the remaining 40 items to a form select option. Thank you for any help. <?php $lender_list = array_filter($lender_list); foreach($lender_list as $key => $value) { //$mykey = $key; echo $key; echo $value['name']; echo"<br>"; } ?>
-
Weird, when I assign the result to a variable and echo it outside the foreach statement the extra character is removed. Does anyone know why this happens?
-
Hi, The below foreach loop is adding characters to the results that should not be there. Any idea why this is happening and how I can fix it. It's returning these results B 4.3900 The B should not be there. ------------------------------------- Here is where the results are being pulled from [37] => Array ( [name] => FI Name [types] => Array ( [0] => FI Name [1] => 2.7000 [2] => 3.1400 [3] => 3.1400 [4] => 3.0400 [5] => 3.6500 [6] => 4.3900 [7] => 4.6400 ) foreach($selected_lender as $item) { echo"<br>"; echo $item[6]; echo"<br>"; //echo $item }
-
And I get closer maybe.... This: echo $rate; //echo substr_count($rate, ' '); $rate_details = explode(" ",$rate); echo print_r($rate_details); returns this: 4 Year 4.390% 4.420% Array ( [0] => 4 [1] => Year [2] => [3] => [4] => [5] => [6] => [7] => [8] => [9] => [10] => [11] => [12] => [13] => [14] => [15] => [16] => [17] => [18] => [19] => [20] => [21] => [22] => [23] => [24] => [25] => [26] => [27] => 4.390% [28] => [29] => [30] => [31] => [32] => [33] => [34] => [35] => [36] => [37] => [38] => [39] => [40] => [41] => [42] => [43] => [44] => [45] => [46] => [47] => [48] => [49] => [50] => [51] => [52] => [53] => 4.420% [54] => [55] => [56] => [57] => [58] => [59] => [60] => [61] => [62] => [63] => [64] => [65] => [66] => [67] => [68] => [69] => [70] => [71] => [72] => [73] => [74] => [75] => [76] => [77] => ) 1
-
okay.. this is getting me closer. echo $royal_list[5] ['types'][8]; It returns 5 Year 4.640% 4.660% Now I need to extract "5 Years" and 4.640% for the results and assign them to a variable for use. thinking...
-
and I only want the first number for the second part of the array
-
Hi I I have the following the following results that are returned from an array. I want to be able to just call one part of the array. Example: $array[7][2]. I have tried this but no result is returned. [7] => Array ( [name] => Convertible [types] => Array ( [0] => Term Posted Rates APR(2) [1] => Convertible [2] => 6 Month 3.140% 3.350% [3] => Closed [4] => 1 Year 3.140% 3.250%
-
To help clariy I am trying to add X days to some days in the past. (this date will be greater than today). I want to take this date and subtract it from today to determine how many days until that date.