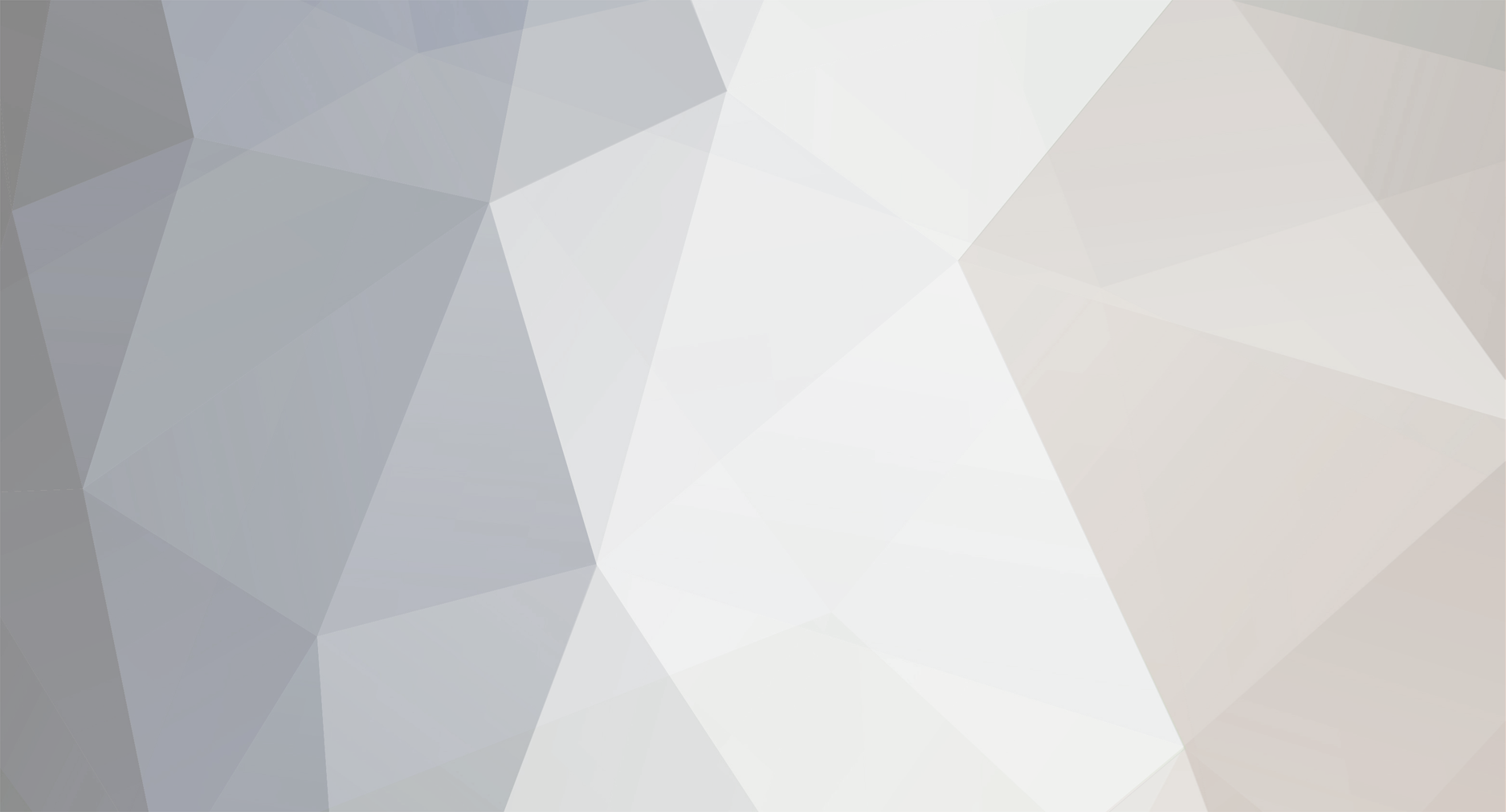
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Yeah, if you set the value of the checkboxes to be the ID of the private message, and make the checkboxes an array, you can use the implode function on the checkbox array, and then use the mysql IN clause to delete them all. More efficient than looping through and doing a separate query for each. Something like: <?php $ids = implode(",",$_POST['checkboxes'];//assuming numerical IDs. If alphanumeric, use "','" as glue. mysql_query("DELETE FROM `yourtable` WHERE `id` IN ($ids)")or die(mysql_error());
-
Doesn't that still boil down to a form being submitted? In which case, you will again need to validate the data. Also, it is normal for any gaming website like that to carry a disclaimer stating that you are not responsible for external websites - and viewing is done at the user's risk. You cannot protect all the idiots - if one of your user's wants to go to another website and put in their username/password, then there's nothing you can do about it.
-
You'll need to set the curl option RETURNTRANSFER to 1 to get the contents in a string. e.g: <?php $ch = curl_init(); curl_setopt($ch,CURLOPT_URL,'your website'); curl_setopt($ch,CURLOPT_RETURNTRANSFER,TRUE); //other curl options $result = curl_exec($ch); You'll then need to use some regular expressions on the returned string, $result, to find the data you need. Theres a subforum on this site for regular expressions, or try looking in the manual: preg_match() On a side note, some dictionary sites do offer an API, which would possibly be an easier way to go. A quick google shows that urbandictionary.com does, but take a look for the one you want.
-
[SOLVED] run a MySQL select quary with insensitive case.
GingerRobot replied to shlomikalfa's topic in MySQL Help
Mysql is ,by default, case insenstive. A standard comparison query (using the = sign) is case insenstive. You can make searches case senstive, however. See: http://dev.mysql.com/doc/refman/5.0/en/case-sensitivity.html -
Change an image based on landing page - Possible?
GingerRobot replied to Dave3765's topic in PHP Coding Help
You could do it all through sessions. When someone comes to either page, you first check to see if the session exists. If it does, we know they've already been on your website, and so don't need to do anything. If, on the other hand, it does not exist, we simply set which image we should display throughout the site. On each page, we load the correct image - the name/link to which will be in the session. -
Very bad idea. Not all browsers will send the http referrer, some filewalls block it, and it can be faked (see cURL for example). You will end up blocking legitimate users, whilst illegitimate users would be able to get round it. You must validate all form data. That is the only solution. Just because your form is set up with a maximum length of 2, do NOT assume this is all you will get. Just because you are retrieving data from a drop down list, do NOT assume the only data you will recieve is contained within the drop down. As i say, you must simply validate ALL of the data from the user.
-
Im confused by your code. Why do you have a text box and a select box that are both using the $_GET value and both have the same name? One (i would assume the later, so the select box) will overwrite the other. Second, did you actually make the changes? I still see $row['3'] rather than $country1[3] Third, in this line: if($row['3'] == $_GET['Country']['0']) You've used Country with a capital C - both of your form elements use a lower case c. Array keys are case sensitive.
-
Not true. mysql_fetch_array, when used without any parameters, returns BOTH an associative and a numerically indexed array. It's perfectly valid to use numerical indexes, even if it does make your code a little unreadable. Your issue appears to be that you are assigning the returned array from the mysql_fetch_array function to a different variable, $country1. Try: if($country1['3'] == $_GET['Country']['0'])
-
[SOLVED] 'php-include' - call this file every 30 seconds?
GingerRobot replied to mellojoe's topic in PHP Coding Help
Erm, no you couldn't. -
Check the source of the generated code. Your browser converts the character codes to their correct symbols to display them.
-
The should always work, if you use them correctly. Perhaps if you show us some code where you are having problems we can help you.
-
You're missing the semi-colon at the end of this line: $from = $email
-
Searching string for integer length - help?!
GingerRobot replied to musclehead's topic in PHP Coding Help
Aside from the point that in your example, the integer does not have 10 digits, you'll need to use regular expressions: <?php $str = 'This is my string 1234567890 and it contains an integer'; preg_match_all('|\d{10,10}|',$str,$numbers); print_r($numbers); ?> Edit: I Misread that. If this number only occurs once, and you just want to check for it's existance, then something like this would work: <?php $str = 'This is my string 1234567890 and it contains an integer'; if(preg_match('|\d{10,10}|',$str,$numbers)){ echo '10 digit number found! The number was: '.$numbers[0]; }else{ 'No 10 digit number within this string!'; } ?> -
How to prevent the default error message from being displayed ?
GingerRobot replied to jd2007's topic in PHP Coding Help
I wonder if Barand has his 'telepathy upgrade board' yet (http://www.phpfreaks.com/forums/index.php/topic,165168.msg725975.html#msg725975) What is the problem? -
Displaying username from foreign key
GingerRobot replied to swollenpickles's topic in PHP Coding Help
You don't need to perform an additional query, you can do it with a join: <?php $query = "SELECT membersreview.*, member.Member AS MemberName FROM membersreview,member WHERE membersreview.ComidId=3 AND membersreview.MemberId=member.MemberId ORDER BY membersreview.ReviewDate ASC"; $resultSet = mysql_query($query) or die(mysql_error()); // returns assoc array of current row until there are no rows left while($row = mysql_fetch_assoc($resultSet)) { print "<table>"; print "<tr>"; print "<td>Member:</td>"; print "<td>" . $row["MemberName"] . "</td>"; print "</tr>"; print "<tr>"; print "<td>Review Date:</td>"; print "<td>" . $row["ReviewDate"] . "</td>"; print "</tr>"; print "<tr>"; print "<td>Rating:</td>"; print "<td>" . $row["UserRating"] . " out of 5</td>"; print "</tr>"; print "<tr>"; print "<td>User Comments:</td>"; print "<td>" . $row["UserComments"] . "</td>"; print "</tr>"; print "</table>"; } ?> -
Still a little unclear. The only thing i can assume is that the code you posted is all of your php code - in which case, im guessing that the problem is you've not retrieved the relevant variables from the $_POST array, and you server is set up with register_globals off (a good thing!) So, try this code: <?php // This is only displayed if they have submitted the form if (isset($_POST['search'])) {//this is a method people would use for checking to see if the form has been submitted //the name we use is the submit button's name - in this case, 'search' $find = $_POST['find'];//set $find to its value in the post array print "<p><h2>Search Results</h2></p>"; //If they did not enter a search term we give them an error if ($find == "") { print "<p>You forgot to enter a search term. Can't search without a search term!</p>"; exit; } // If they enter a search term connect to the database. require_once("inc/connection.php"); // filter out white space, tags etc. $find = strtoupper($find); $find = strip_tags($find); $find = trim ($find); // Search the database for the search term entered by the user. $searchresults = mysql_query("SELECT * FROM comic WHERE upper($field) LIKE '%$find%'"); print $searchresults; // display the results while($result = mysql_fetch_array( $searchresults )) { print $result['ComicTitle']; print "<br />"; print $result['ReleaseDate']; print "<br />"; print $result['ComicPrice']; print "<br />"; print $result['ComicDescription']; print "<br />"; } //This counts the number or results. If no result, they get the no matching entries message. $anymatches=mysql_num_rows($searchresults); if ($anymatches == 0) { print "You searched for " . $find . ". Sorry, no entries matched your search."; } } ?> Notice the change to how you check if the form has been submitted - you don't need your hidden field.
-
That rather depends on what validation you wish to do. If you are simply wanting to check if anything has been put into the fields, then using the empty() function would suffice. As was suggested, you could place all of the post fields into an array, and loop through, checking each in turn. There are plenty of other validation techniques you might want to use. Perhaps you want to check the length of certain fields? Try the strlen() function. Perhaps you want to check the form of certain strings (email addresses for example). If so, you'll need to use regular expressions. There's a forum on this site for those, so take a look there. The point im trying to make is that there's no set rules for this. It all depends on the specific situation - you'll need different validation rules for different things.
-
So what happens? Do you get an error? I say this so many times - we need to know what the problem is before we do anything! Help us to help you.
-
I would let you borrow mine, but, you know, it's kinda necessary.
-
You mean your not telepathic? And there i was, thinking you were omniscient...
-
You need to echo your html: <?php if ( $numPages > 1 ) { echo '<a href="../untitled-2.php" title="targetis" target="_self">hghg</a>'; } ?>
-
[SOLVED] Loop through url variables as a structure
GingerRobot replied to mjahkoh's topic in PHP Coding Help
Haha No problem. -
Something like: <?php $sql = "SELECT COUNT(`steaks`) FROM `players`"; $result = mysql_query($sql) or die(mysql_error()); $num_steaks = mysql_result($result,0); echo 'The total number of steaks is: '.$num_steaks; ?>
-
[SOLVED] Loop through url variables as a structure
GingerRobot replied to mjahkoh's topic in PHP Coding Help
Try: <?php foreach($_GET as $key => $value){ echo "$key = $value"; } ?> -
[SOLVED] white space creating problems in session variables
GingerRobot replied to mubashir's topic in PHP Coding Help
Bizarre. Can we see some more of the code you are using?