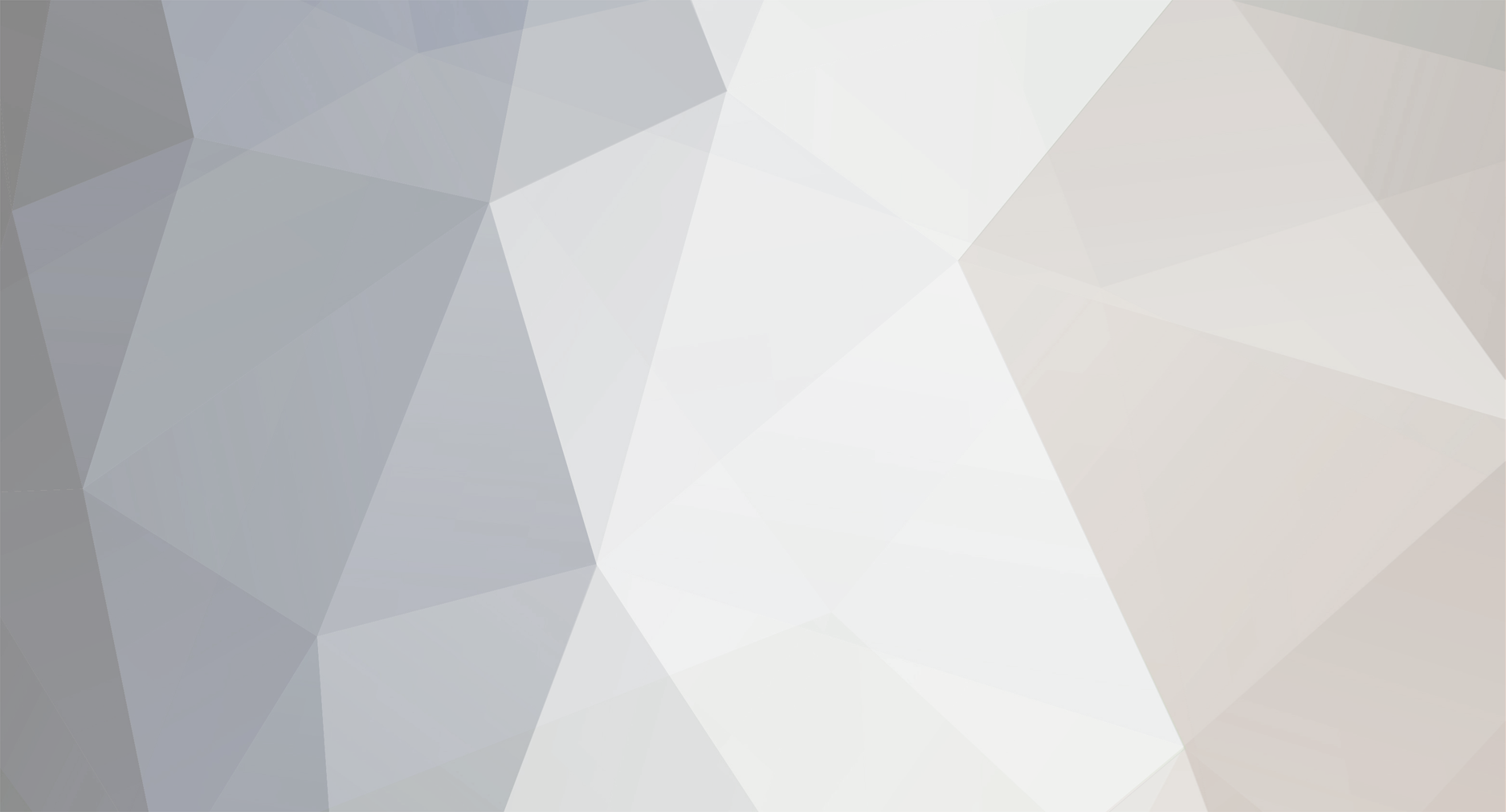
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
That wouldn't work. You'd need to do: <?php echo '<form action="post-premiumreview.php?id='.$subid.'" method="POST">';?>
-
You should use the later really. Otherwise you are using an undefined index. Whilst php can deal with this, you will get a notice if you've have error reporting set to include notices.
-
So you're selecting a list of items from a database which you want to sort for output as a drop down list, and then add in the other option on the end? Without your current code i cant offer more help than that it will look something like: <?php $sql = "SELECT `entry` FROM `yourtable` WHERE `entry` != 'other' ORDER BY `entry`"; $result = mysql_query($sql) or die(mysql_error()); echo '<select name="select field">'; while($row = mysql_fetch_assoc($result)){ echo '<option value="'.$row['entry'].'">'.$row['entry'].'</option>'; } echo '<option value="other">other</option>'; ?>
-
Well, im no expert in these things whatsoever - however im sure you'll also need to configure your router. You'll need to allow access on port 80. If you don't know what you're doing, you'll probably end up having a bit of a security threat though.
-
Strings enclosed in single quotes are taken to be literal - that is, any variables inside them are not evaluated. For example: <?php $foo = 'bar'; echo 'The string is: $foo'; ?> Produces the exact text: The string is: $foo If you enclose the string in double quotes, however, variables are evaluated. So: <?php $foo ='bar'; echo "The string is: $foo"; ?> Produces: The string is bar So, if you want to echo variables within your string, you must either use double quotes or concatenation: <?php $var = 'some variable'; //either echo "Some other text $var a bit more text"; // or echo 'Some other text '.$var.' a bit more text'; ?>
-
Indeed. You want asort() which preserves the keys duing the sort.
-
[SOLVED] Displaying Text from MySQL
GingerRobot replied to Helminthophobe's topic in PHP Coding Help
Most people would usually use the function prior to the display of the text, rather than before you put it in the database. Afterall, the reason you need to use the function is to format the display in HTML. The original text didn't contain break line tags, so you shouldn't store those in the database. -
Interesting Problem..... Can anybody solve it????
GingerRobot replied to php_novice123's topic in PHP Coding Help
All possible with the hungarian algorithm. For an N*M matrix, you add in 'dummy' rows/columns of sufficient value to not affect the result, then ignore any of the results which use a dummy row/column. To maximize the result, find the highest number within the maxtix, x, and repalace all numbers within the matrix with x-n. -
Interesting Problem..... Can anybody solve it????
GingerRobot replied to php_novice123's topic in PHP Coding Help
As i said, it can be solved using the hungarian algorithm: http://en.wikipedia.org/wiki/Hungarian_algorithm Since the hungarian algorithm can solve the problem in polynomial time, we have no real issue with large matrixes. -
Have you been paying people again roopurt?
-
it's not just you. my host is one of the largest in the UK, yet have only just upgraded all of their main packages and default server packages to PHP5 and MySQL 5 (from php 4.3.1/mysql 3.2.23 - so even mysql is lagging also) considering the benefits of php5 (including performance and development), and also considering that if any changes are required to take a site from 4 to 5 they're generally very minor and simple, it's more surprising that php5 has been so slow to be adopted. Isn't the issue with most hosts that they were still running php 4 with register_globals turned on? Thus upgrading to php 5 and having them turned off ends up breaking a lot of peoples websites? Personally i think it's their own fault, though i can understand the problem a web hosting company might face!
-
[SOLVED] Assign each value from mysql query automatically?
GingerRobot replied to scooter41's topic in PHP Coding Help
No problem. Just fyi, the use of the double dollar sign means you are using a variable variable: the name of the variable you assign $v to is the value of the variable $k. Perhaps another example illustrates that better: <?php $foo = 'bar'; $bar = 'test'; echo $$foo; //is equivilant to echo $bar, since 'bar' is the value of $foo //produces test ?> -
In this case, i personaly favour an approach where you have two levels for each item in your array: a parent and a child. I think this makes it easier to deal with than the case where you have nested parent child relationships within your array. This is probably easier to explain by example. I wrote this a while back for another post: <?php function menu($submenu,$index='Parent'){ foreach($submenu[$index] as $k => $v){ echo "<li><a href='$v'>$k</a></li>"; if(is_array($submenu[$k])){ echo '<ul>'; menu($submenu,$k); echo '</ul>'; } } } $submenu = array(); $submenu["Parent"]["Home"] = "/index.php"; $submenu["Parent"]["Contact Us"] = "/contactus.php"; $submenu["Parent"]["Members"] = "/members/index.php"; $submenu["Home"]["About"] = "/about.php"; $submenu["Contact Us"]["Live Chat"] = "/chat/index.php"; $submenu["Live Chat"]["Live Help"] = "/chat/connected.php"; $submenu["Contact Us"]["Email"] = "/emailform.php"; echo '<ul>'; menu($submenu); echo '</ul>'; ?> Given your current array structure, it would be relatively easy to transform that array to work with the above function. Something like: <?php $array = array(array('Outdoors','Regions','Southern Rivers'),array('Outdoors','Activity','Scenic Viewing')); function createarray($array){ foreach($array as $k => $v){ foreach($v as $k2 => $v2){ if($k2 == 0){ $parent = 'Parent'; }else{ $parent = $array[$k][$k2-1]; } $new_array[$parent][$v2] = $v2; } } return $new_array; } ?>
-
[SOLVED] Assign each value from mysql query automatically?
GingerRobot replied to scooter41's topic in PHP Coding Help
Sure. Try: <?php foreach($row as $k => $v){ $$k = $v; } ?> -
Well, ive never worked with this type of database, however the obvious question to ask is if you have the php_dba extension loaded? Check your php.ini file for this line: ;extension=php_dba.dll You'll need to remove the semi colon.
-
You'll first need to get the contents of the webpage which can, in most cases, be achieved with the use of the file_get_contents() function. In some cases you'll need to use cURL, however. You'll then need to use regular expressions to find your text/hyperlink: preg_match()
-
Typo on this line: foeach($_POST["delete"] as $key => $id) Next time, try posting the actual error message and the line in question. Saves people the time it takes to look through it.
-
Sorry? You want to get the most recently added products? If so just modify your query to only select 2 items, and have it orded by the time added in descending order: $sql = "SELECT * FROM `yourtable` ORDER BY `time` DESC LIMIT 2"
-
See this FAQ on multi-column results: http://www.phpfreaks.com/forums/index.php/topic,95426.0.html
-
What exactly does that mean? We're not actually psychic here you know. What actually happens? What specifically is the problem?
-
Any number of classes can inherit from a single parent class. If class A is your parent, then class B,C,D etc can all be children of that class, without inheriting from each other. However, php does not support multiple inheritance, which is where a class extends multiple classes - that is, it has more than 1 parent. For example, class C cannot inherit from class A and B. You question wasn't entirely clear, however i think you wanted something like: <?php class three{ function three(){ } } class one extends three{ function one(){ } } class two extends three{ function two(){ } } ?>
-
What exactly do you mean? Do you mean there is a website which has lots of <a href=...> tags that you would like to find and get the addresses of the websites they link to? If so, then yes, its completely possible. You'll firstly need to get the contents of the website, which can, in most cases, be achieved with the use of the file_get_contents() function. With some websites, you will need to use cURL however. Once you have the contents of the website in a string, you'll need to use regular expressions to find the links. You'll be wanting the preg_match_all() or the ereg() functions for that. Edit: I just noticed you said 'using html'. If thats what you meant, then no, its not possible. I misread that as 'using php'.
-
Having looked at your code a bit more, i have to say im completely and utterly lost. Why do you echo the html declaration and <head> tags inside a loop? Are you actually sure that the code you posted is the code that is being run? The reason i ask is that the loop in the code you posted terminates in this line: <title><? echo $row['title']; } ?> - Register</title> Yet, from a look at the source code, the loop appears to go on further, since the </head> and <body> tags form part of this repeated loop. Also, why do you include config.php 3 times?
-
What do you mean by a continuous loop? The only loops i can see are the two while loops which deal with the mysql data. Unless there was unlimited data in your database, that couldn't be an infinite loop. What actually happens when you run the script? What is it supposed to do? More information is really needed.
-
You're rather re-inventing the wheel. Try using the array_walk_recursive() function which is specifically for applying a function to every member of a multi dimensional array. Try: <?php $data = array(1, 2, "cool"=>7, 7=>3, "e"=>array(3,5,7), "blah"=>3, array(2,4,6) ); function increment(&$v){ echo $v++; } function pr($arr) { echo '<pre>'; print_r($arr); echo '</pre>'; } pr($data); array_walk_recursive($data,'increment'); pr($data); ?>