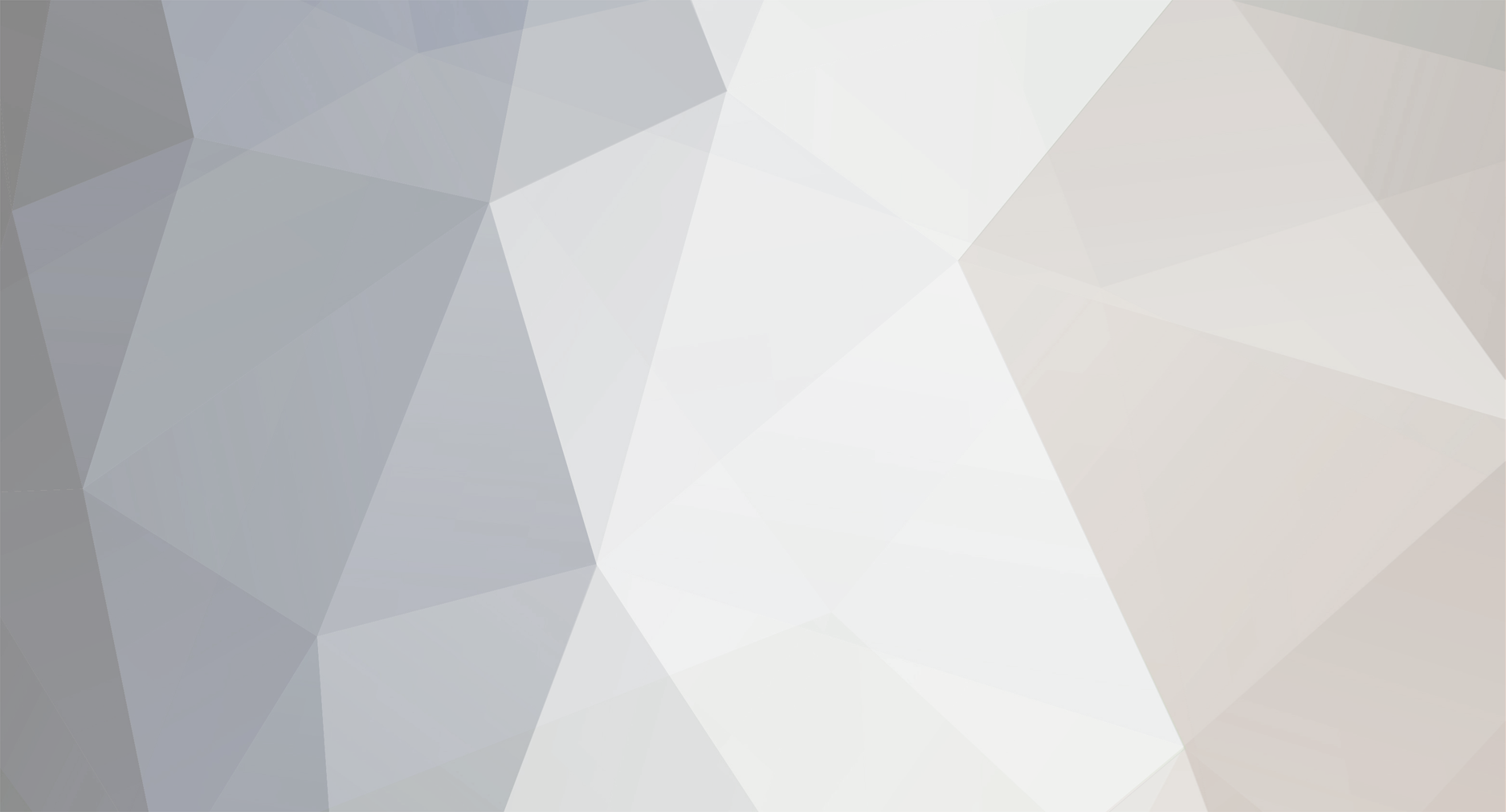
GingerRobot
-
Posts
4,082 -
Joined
-
Last visited
Posts posted by GingerRobot
-
-
If you're looking for this kind of behaviour, you'd be better off using sessions.
-
When you include a file, it is, execution-wise, (almost) identical to swapping the line that does the including for all of the source code in the included file.
In other words this:
file1.php
<?php $text = 'foo'; include('file2.php'); echo 'bar'; ?>
file2.php
<?php echo $text; ?>
Is the same as:
file1.php
<?php $text = 'foo'; echo $text; echo 'bar'; ?>
Edit: Updated to add variables for clarity.
-
why does its value needed to be assign to false here and then use it in that conditioned line?
I've absolutely no idea. I assume you didn't write this code, so maybe try asking the person who did?
I've no idea what this code does so i can't possibly tell you how it works.
-
So you have chained forms? I'd recommend using sessions to work out where each user is and what information they should be filling in.
If you're new to sessions, you might like to check out this tutorial: http://www.phpfreaks.com/tutorial/sessions-and-cookies-adding-state-to-a-stateless-protocol
-
This appears to be more in relation to how to use the debugger feature of your editor rather than an issue with your code, so i've moved it here.
-
-
Yeah, of course, if the values of the arrays themselves are empty strings, you'd get blank lines printed out. But then, if the arrays contained blank lines, i'd expect that the OP would want them to be printed.
the result is, Array ( [1] => [2] => [3] => wqd ) , so wont the foreach just do the same thing? i.e. put in the blank spaces.
However, the point is that, even with the above example, the required information wouldn't be printed if you used a for loop. If we have the following code (which is actually just the original) :
for($i=0;$i<count($result);i++){ echo $array[$i]; }
Then what's printed? first array[0]. That doesn't exist. If you have notices turned on, you'd get a warning. If you dont, we'll have an extra new line. Then array[1] and array[2] are printed. But array[3] isn't printed - our loop finishes when i = 2, since there are 3 elements in the array.
So all in all, you really should be using a foreach loop.
-
Had a quick look arround php.net and empty() looks like the function you need to check to see if the array element it empty.
Yeah that's correct. However, in this case, those entries aren't actually empty -- they don't exist.
The array_diff function maintains association between keys and values of the arrays - that is, the elements in $result have the same keys as they had in $array1. Therefore, you might have an array with, say, keys 0, 2, 5 and 8. The length of the array is 4, but only the elements with keys 0 and 2 will be printed, plus blank likes for the non-existing elements with keys 1 and 3. If that's not very clear, use print_r on the generated array and you'll see what i mean.
The solution? Use a foreach loop instead:
foreach($result as $value){ echo $value . "\n"; }
-
Ok, so you're storing this text in the database: "<img src="http://www.yoursite.com/image.jpg">" ? You don't want to be doing that...just store "http://www.yoursite.com/image.jpg"
Obviously without knowing what the outimage function does, it's a bit difficult. However, the alt parameter is for alternate text - the text you might see if you hover over images. It's for accessibility.
The easiest way to work out what's going wrong is to take a look at the generated HTML. View the source of the page and see what images are supposed to be being displayed. I couldn't actually see any broken images on the page you posted.
-
If they're entering the same link with just the ID different, why not just ask for that? Be easier to validate and would mean if bebo alter anything it shouldn't affect you.
-
evaluate your emails chekc how many url's they have in them how many of wotever you notic ein the most spams, now when teh count gets to a certain amount lets say security level 5 email it to yourself butdont email it to teh recipient otherwise emeail to teh recipient and yourself, that is how spam assasin does it.
when you find a new trennd add it to your lookups,
make sure you check the CC and BCC etc etc for scriupt tags and code
Did you read any of the thread?
some of it yes
Presumably nothing beyond the thread title?
-
evaluate your emails chekc how many url's they have in them how many of wotever you notic ein the most spams, now when teh count gets to a certain amount lets say security level 5 email it to yourself butdont email it to teh recipient otherwise emeail to teh recipient and yourself, that is how spam assasin does it.
when you find a new trennd add it to your lookups,
make sure you check the CC and BCC etc etc for scriupt tags and code
Did you read any of the thread?
-
I want to modify the code i put not making a new code
Err..but your code does the wrong thing. In some cases, it's easier to start from scratch.
-
Personally i wouldn't do it that way, since you can't distinguish between a non-exist/incorrect user and password and an invalidated account - i'd select the activation column and perform the check in your PHP code. That way you can inform the user if they've not validated. They might have forgotten/not got the email and it'd be confusing if you just tell them their password's incorrect.
-
I think i'd go with something like this:
<?php $currPage = 6;//the page you're on $pagesToShow = 5;//the number of pages to show $pages = array($currPage);//an array of the pages we'll show $previousPages = 0;//the number of pages we've added that are before the current one $maxPages = 10;//the maximum number of pages available //Add up to 2 pages before the current one while($previousPages <2 ){ if($currPage-1 > 0){ array_unshift($pages,--$currPage); $previousPages++; $pagesToShow++; }else{ break; } } //add the rest of the pages after, if they exist while($pagesToShow > ){ if($currPage+1 <= $maxPages){ array_push($pages,++$currPage); $pagesToShow++; }else{ break; } } //output the pages foreach($pages as $page){ echo $page.' '; } ?>
Untested, but you should get the general idea.
-
Maybe I'm missing something here? :-\
Naa. It's me that's missing something here - half my brain cells apparently. I'm not really quite sure what i was thinking when i posted that :s Sorry 'bout that.
-
Doesnt the above still assume that the ID=142 is fixed though?
No. The parse_str() function breaks up a string by the & character, with each piece going into $pieces. The code then checks to see if any of the keys of that array are the thing you're looking to remove. If it is, it deletes it. Finally, we glue all the pieces back together with the & character.
The only problem with nrg's solution is that, should the argument be in the middle of the string, we'll lose an ampersand. If you decide to not replace the ampersand, then we'll also have to check to see if we have a rogue one on the end of our string.
-
26 queries? Sounds nasty. Think i'd go with something like:
<?php $alphabet = range('a','z'); $lettersFound = array[]; $sql = "SELECT SUBSTRING(`field`,0,1) AS firstLetter GROUP BY firstLetter"; $result = mysql_query($sql) or trigger_error(mysql_error()); while($row = mysql_fetch_assoc($result){ $lettersFound[] = $row['firstLetter']; } foreach($alphabet as $letter){ if(in_array($letter,$lettersFound){ //there are fields with this starting letter, so display a link }else{ //no fields starting with this letter, just display the letter with no link } } ?>
-
Try this then:
<?php $str = "action=listingview&listingID=142&cmsrealty=admin"; parse_str($str,$pieces); if(array_key_exists('cmsrealty',$pieces){ unset($pieces['cmsrealty']); } $str = implode('&',$pieces); ?>
-
Where does the string come from? It might be easier to just not put it in in the first place?
In any case, is &cmsrealty=admin always the last part of the string? If so, you could do something like this:
<?php $str = "action=listingview&listingID=142&cmsrealty=admin"; $pieces = explode('&',$str); unset($pieces[count($pieces)-1]); $str = implode('&',$pieces); ?>
-
The xampp documentation tells you which php.ini is used for the web based php and the cli php.
As will the output of php_info()
-
How can I use an if/then statement or the empty() to make it not display a type if there is no type listed?
Pretty much as you describe there. The logic is: if the variable is not empty, search using the variable. If it's not, dont add a where clause.
-
This probably means the cURL library is not installed. If you run a page with just this in:
<?php php_info(); ?>
You'll be able to verify this. If you cannot find a section called curl/libcurl, then it is not installed.
-
The error in the proof being that you've ignored the last term of S2.
[SOLVED] checkbox
in PHP Coding Help
Posted
In order for a checkbox to be ticked, you don't set it's value, you set checked="checked". You might like to check out my tutorial on checkboxes and databases:
http://www.phpfreaks.com/tutorial/working-with-checkboxes-and-a-database