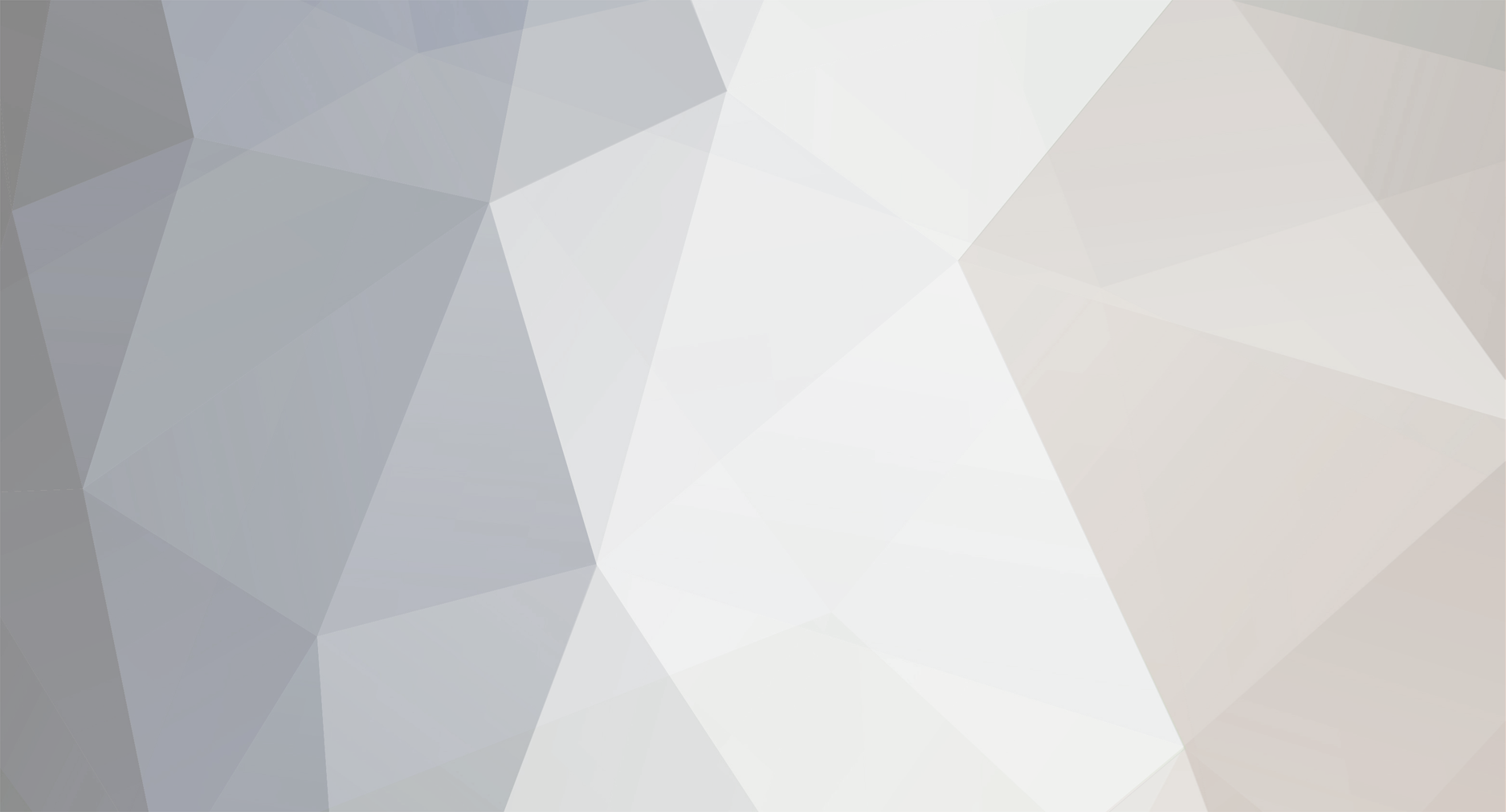
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
I think i'd build an array of pages which you might want to include. Something like this: $pages = array('register','register_done','news'); if(isset($_GET['step']) && in_array($_GET['step'],$pages)){ include($_GET['step'].'.php'); }else{ include('homepage.php'); }
-
Err. Yes. They're pretty important. They're generally the single most helpful thing in finding out why a script's not running the way you expected it to. It's damn difficult finding a typo without them. With regard to the error, you should check to make sure that $_GET['step'] is set before trying to use it: if(isset($_GET['step']) && $_GET['step'] == 'register'){ include_once('register.php'); }else{ include_once('homepage.php'); } You might wonder why you don't still have problems with this if statement. After all, you're still making a test against $_GET['step'] so if it doesn't exist you might think you'd still get a warning. But no. PHP (like most languages) uses short-cut evaluation of logical expressions. That is, whilst evaluating the expression, if it has already worked out the result, it won't check the rest of the comparisons. So, in this case, the isset() test will be evaluated first. If it is false, then the second part of the statement wont be tested since false & expression is false regardless of the result of expression.
-
You should take a look at escaping your data - mysql_real_escape_string
-
1.) Are you using different pages for each link? That really defeats the entire object of using pagination. 2.) Your logic is wrong where you define counter. You have it as if $_GET['counter'] is false(roughly the same as not set) then set counter to it's value. Try: if (isset($_GET["counter"])) { $counter = $_GET["counter"]; } else { $counter = 0; } 3.) You might like to check out this tutorial It's written using a database rather than for files, but the same principles still apply.
-
Well, you're defining which emails you want to send to inside your loop which runs for every record in the table. In order to only send to those emails, you should specify that in your query and use the email address in the record. Something like: require("config.php"); $q = mysql_query("SELECT * FROM users WHERE active = 'yes' AND email IN ('tpatterson@cheezyfries.net','traey@hxcteam.com') ORDER BY user_id"); $i = 1; while ($b = mysql_fetch_array($q)) { $email = $b['email']; $to1 = $email; $headers = 'MIME-Version: 1.0' . "\r\n"; $headers = "From: Solon Sportsmen Club <noreply@solonsportsmen.org>\n"; $headers .= "Reply-to: noreply@solonsportsmen.org\n"; $headers .= "Bcc: $to1" . ', '; $headers .= "Bcc: noreply@solonsportsmen.org\n"; $headers .= "Bcc: noreply@solonsportsmen.org\n"; $headers .= "Bcc: noreply@solonsportsmen.org\n"; $headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n"; mail($to, $subject, $message, $headers); echo "Newsletter has been sent to:<br />$i - $email<br />"; $i++; } That said, you should check the notes from the php manual about the mail() function:
-
I could be wrong but it would seem logical to me that text would be far easier to analyse and extract information from than an image would be. Perhaps it would be more secure insofar as the majority of tools that currently exist that might break captchas are targetted at images but i would imagine creating a tool to break a text-based captcha would be easier than an image based one.
-
Yes. Load it with the appropriate version of imagecreatefrom(gif/jpeg/png/etc) and use the imagecopyresampled function
-
Are you searching a database? You'll want to look into full-text searching.
-
about bookmarks application in php & mysql web development ebook
GingerRobot replied to peacengell's topic in PHP Coding Help
1.) You might want to try reading your code. The function you've tried to call is do_html_heading(); the function you wrote is called do_html_header() 2.) I assume you meant to call the function from outside of it. I can't imagine you want to recursively create a header. 3.) where is $title defined? function do_html_header($title) { // print an HTML header ?> <html> <head> <title><?php echo $title;?></title> <style> body { font-family: Arial, Helvetica, sans-serif; font-size: 13px } li, td { font-family: Arial, Helvetica, sans-serif; font-size: 13px } hr { color: #3333cc; width=300px; text-align:left} a { color: #000000 } </style> </head> <body> <img src="bookmark.gif" alt="PHPbookmark logo" border="0" align="left" valign="bottom" height="55" width="57" /> <h1>PHPbookmark</h1> <hr /> <?php } if($title) { do_html_header($title); } -
You can't have HTML and an image output by the same page. It just doesn't make sense. The browser has no idea what it's supposed to display. Two options: 1.) Write the code that creates the image in one file (lets say image.php ) and make that the source for an img tag: <img src="path/to/image.php" /> 2.) Are you actually doing any manipulation with this image? Or are you just displaying an image as-is? If so, you really shouldn't be loading it into an image resource and displaying it. Just put the location of the image as the source of the tag.
-
[SOLVED] Administrative Images
GingerRobot replied to The Little Guy's topic in PHPFreaks.com Website Feedback
Would it really be that hard to create some images yourself? -
I assume you mean on this forum? User's can't delete their own messages. You can edit your post up to a certain time after you created it. I can't remember the exact amount of time, but it should be a couple of minutes.
-
Meh, i could quite easily believe the teacher had no idea what they were talking about.
-
It's amazing how the concept of a random, representative sample escapes some people isn't it?
-
Should I stay with IE 6 or upgrade to IE 7 as a Web Designer
GingerRobot replied to andrewsCWD's topic in Miscellaneous
I didn't think the firefox auto updater forced major new versions? Like, don't you have to manually download FF 3? -
The apostrophe has no special meaning in regex, so it doesn't need to be escaped and nor does the comma. In fact, inside square brackets, the . has so special meaning either. "/^[a-zA-Z,'.\-\s]*$/" You do realise you're allowing the empty string through with this, don't you?
-
Should I stay with IE 6 or upgrade to IE 7 as a Web Designer
GingerRobot replied to andrewsCWD's topic in Miscellaneous
I'd also question the wisdom of not testing IE7. -
[SOLVED] PHP MySql database search code not returning results
GingerRobot replied to sandbudd's topic in PHP Coding Help
In MySQL, the hash (#) comments the rest of the line out. You should consider changing your table name to avoid problems in the future, though you can get around the problem by placing the table name inside backticks (`). -
I personally liked the example given in my java lecture last week. Consider a set of shape classes - Square, Circle etc. Now, we want certain methods to be common to all shapes. We might have a draw() method. We also want to be able to call this method without knowing what shape we're drawing. One approach might be to have a parent class - Shape, which defines the methods we want the classes to have in common and all other shapes to inherit and overload. For example: class Shape{ void draw(){ //draw method } } class Circle extends Shape{ void draw(){ //special information for drawing a circle } } class Square extends Shape{ void draw(){ //special information for drawing a square } } However, we have a problem. What should happen if you call draw() on a Shape class? What does a general shape look like? In order to prevent confusion and miss-use of the classes, you might define Shape to be an abstract class and draw to be an abstract method, or you might make Shape an interface. In either case, the method draw() can't be called unless you have a particular shape. It helped me understand a bit anyway
-
You need to call mysql_insert_id() after inserting - the description of the function does say "Get the ID generated from the previous INSERT operation". For example: $insert = mysql_query("INSERT INTO log (`userid`) VALUES('{$mem['id']}')") or die(mysql_error()); $newid = mysql_insert_id(); $insert2 = mysql_query("INSERT INTO fishlog (`fish`, `logid`) VALUES('{$fish['code']}', '$newid')") or die(mysql_error());
-
So what does happen? Are you sure that you named your submit button with a capital S and the value with a capital L? I ask since none of your other varaiables/array indexes are using capitals... Do you have error_reporting set to E_ALL and display_errors turned on?
-
That's not a good method. If you have simultaneous requests to this page, there's no guarantee that after selecting the maximum id and adding one, another record wont be inserted by another request before this request does the insertion. You should use mysql_insert_id to prevent this.
-
They're not particularly difficult to get around - which is why i wouldn't rely on them. To be able to work round it, you need to understand how it works. When the form is loaded, some sort of pseudorandom number/string is stored in a hidden field. It is also stored in a session. When the form is submitted, the values in the hidden field and the session are compared. If they're equal, it means the user loaded the form prior to submitting it. If they're not, it means someone posted the form directly, without visiting the form page first. So, in order to have an automated request do this, it must be able to accept a cookie so that the session ID can be created on the first request and be the same on the second. Happily, cURL provides cookie functionality. So that's what you need to look up.
-
In other words you're trying to circumvent some check that's in place to ensure that a user is actually making the request and not an automated script? I don't really care if that's what you're trying to do, but you might as well be honest about it.