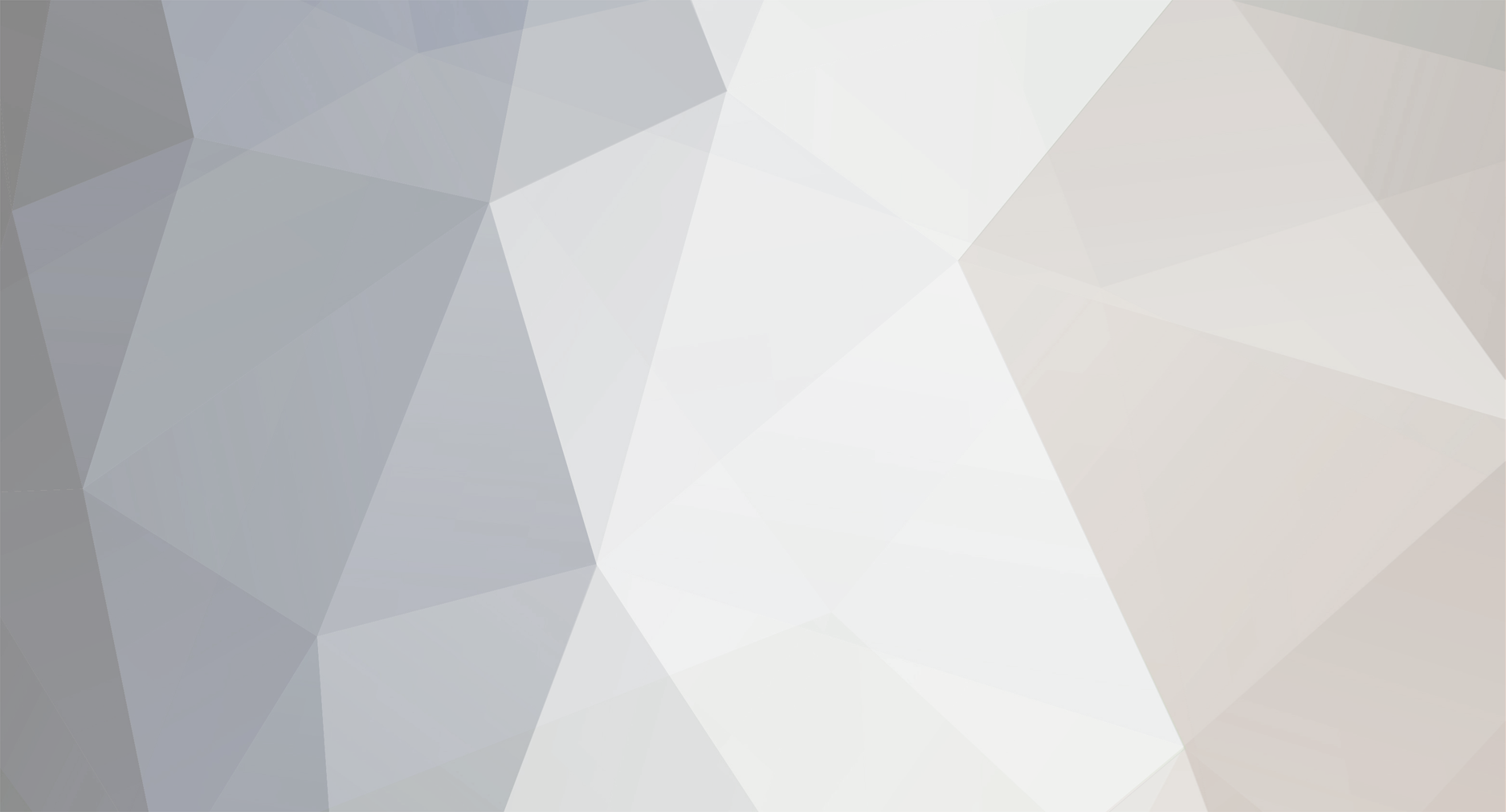
GingerRobot
-
Posts
4,082 -
Joined
-
Last visited
Posts posted by GingerRobot
-
-
There was one mistake in there...the for loop should be initialized with x as 0, not 1. The following generates 4 google logos in one image, using the code i provided:
<?php $im = imagecreatefromgif("http://www.google.co.uk/intl/en_uk/images/logo.gif"); $barcodewidth = imagesx($im); $barcodeheight = imagesy($im); //after creating the barcode $barcodesRequired = 4; $im2 = imageCreate($barcodewidth,$barcodeheight*$barcodesRequired); for($x = 0; $x< $barcodesRequired; $x ++){ imagecopy($im2,$im,0,$barcodeheight*$x,0,0,$barcodewidth,$barcodeheight); } Header( "Content-type: image/gif"); ImageGif($im2); ImageDestroy($im); ImageDestroy($im2);
So it seems to be working for me. Perhaps you could post the actual code you're using now?
-
So you mean that if a there is, say, "john" in the name2 column of a given row and you search for "john", then it won't find it, but it will if "john" is in the name1 column of a given row?
Can you post your updated code?
-
Using the first option, the imagegif function takes an optional second parameter: a filename to save the image to. So, you could do this for example:
ImageGif($im,"barcode.gif"); $barcodesRequired = 3; $output = str_repeat("<img src='barcode.gif' /><br/>",$barcodesRequired); echo $output;
If you take this approach, then you'll have to remove this line:
Header( "Content-type: image/gif");
As the content you're serving is no longer a gif image, it's HTML.
It might be that you specifically wanted to output a single image with the barcode appearing multiple times though. In which case, you'd want the second option:
//after creating the barcode $barcodesRequired = 4; $im2 = imageCreate($barcodewidth,$barcodeheight*$barcodesRequired); for($x = 1; $x< $barcodesRequired; $x ++){ imagecopy($im2,$im,0,$barcodeheight*$x,0,0,$barcodewidth,$barcodeheight); } Header( "Content-type: image/gif"); ImageGif($im2); ImageDestroy($im); ImageDestroy($im2); return;
If you're easy either way, the first option is probably better...it'll use less resources.
-
Ah thanks mate your a genuis SOLVED.
Superb. I get the credit and wildteen did all the work
Don't forget to mark your topic as solved (You'd already done it).
-
If I understand correctly it's necessary to use LIKE instead of the equal sign ( = ) in SQL Query's when using wildcards then ?!
That's correct, yes.
-
By "it wont echo anything" do you mean the page is entirely blank? If so, do you have error reporting and display errors turned on? Are you sure you're also not being redirected and it is in fact gang_listing.php that is blank?
-
You could either save the image and output multiple HTML image tags, or you'll have to create a larger canvas and copy the image onto it multiple times using imagecopyresampled
-
Hi mate. Yea I read that after I posted, moved it after connection string however still no luck
Did you also do this?:
Remove the . after '$g_name'.
You should have:
$sql1="INSERT INTO form_data (your_team, opp_team, date1, time1, g_name, result, writeup) VALUES ('$your_team','$opp_team','$date1','$time1', '$g_name', '$result', '$writeup') ";
-
You do love regular expressions don't you CV? Though I thought they wanted to remove the heading tags.
<?php $mytext = "<h1>Title One</h1><br>This is text one<br><br><h1>Title Two</h1><br>This is text two<br><br><h1>Title Three</h1><br>This is text three"; $titles = explode("<br>",$mytext); for($x=0;$x<count($titles);$x+=3){ echo "<a href=somepage.php?id=" . (($x/3)+1) . ">" . strip_tags($titles[$x]) . "</a><br>\n"; } ?>
-
Well that's roughly what i posted here:
SELECT * FROM yourtable WHERE colour=(SELECT colour FROM `incoming` WHERE ...)
Fitting it in with what you have:
$id = (int) $_GET['id']; $sql = "SELECT * FROM yourtable WHERE colors=(SELECT colors FROM `incoming` WHERE id=$id)"; $result = mysql_query($sql) or trigger_error(mysql_error(),E_USER_ERROR);
-
I'd start by adding some error checking:
mysql_query($sql,$con) or trigger_error("Error in query: $sql <br>Error was:" . mysql_error(),E_USER_ERROR); mysql_query($sql1,$con) or trigger_error("Error in query: $sql1 <br>Error was:" . mysql_error(),E_USER_ERROR);
-
Meh, it's the simplest things that are usually the hardest to spot.
-
I'm still not sure i follow exactly, but did you want something like this then?
$colors = array($_GET['color1'],$_GET['color2'],$_GET['color3']); $colors = implode("','",$colors); $sql = "SELECT * FROM yourtable WHERE colors IN '$colors'"; $result = mysql_query($sql) or trigger_error(mysql_error(),E_USER_ERROR); //display the results
-
Do you have a snippet of code like that? been trying to solve this for last few hours now and no go
thanks
Why don't you post up what you've tried then and we can see where you're going wrong.
-
A quick google search showed up that Joola uses something similar to prevent access to files which are included by others. So yes, if you are using this in the same way and defining that constant on the pages which include these files, then it will work.
However, i'd prefer to place files which i don't want to grant access to outside of the web root.
-
The error isn't generated by the call to mysql_unbuffered_query (which should have been obvious from the error message), but from your "or die" action. I assume you wanted to call mysql_error()....
-
I don't think i understand. I assume you didn't mean something like the following:
$color = 'green'; $sql = "SELECT * FROM yourtable WHERE color='$color'"; $result = mysql_query($sql) or trigger_error(mysql_error(),E_USER_ERROR); //display the results
Or did you mean that the colour in question is selected from the colour of another row given by some criteria? Something like a "show similar articles (or colours)"?
In which case, you can either perform one query to extract the colour or do it in one:
SELECT * FROM yourtable WHERE colour=(SELECT colour FROM `incoming` WHERE ...)
-
Is it possible to get it to format like this
Of course. I'd explode by the breakline and loop through every third element of the resulting array. Thiw will give us the elements with the titles in. We can use strip_tags to remove the <h1> tags.
-
First time i've seen the output buffering used like this in a while...
Anyway, the problem is a tiny one. You've a rogue quote mark on this line:
<a href=<?php echo($baseuri."?tabindex=".$curindex);?>">
If you'd echoed out $_GET['tabindex'] or taken a look at the page your browser was going to, you'd probably have spotted that '...?tabindex=1" ' isn't quite right. Try:
<a href='<?php echo($baseuri."?tabindex=".$curindex);?>'>
-
There's no easy way to do that...you need to search each field. For example:
SELECT * FROM yourtable WHERE field1='somevalue' OR field2 = 'somevalue' ...
But, why do you have 6 fields storing names in them? Chances are, there's a better way to store you data.
-
Not seen that one before, but according to the manual:
Errors/Exceptions
Every call to a date/time function will generate a E_NOTICE if the time zone is not valid, and/or a E_STRICT or E_WARNING message if using the system settings or the TZ environment variable. See also date_default_timezone_set()
So i'm assuming there's no timezone set in your PHP setup.
By the by, you don't need both of those functions to do what you want. You either want mk_time() with no parameters, date("U") or time().
-
If i understand you correctly, an easy work-around would be to use the LIKE operator rather than the = operator in your query. For example:
if(showingAllGenres){ $genres = '%'; }else{ $genres = $_POST['genre']; } //Similarly for the group $sql .= "WHERE groep.id LIKE '$groups' AND genre.id = '$genres'";
I hope you understand what I mean because my english isn't that great
Unfortunately (or, perhaps from your perspective, fortunately) your English seems better than most. Also, it's nice to see a new member trying to help other members as well as asking their own question
-
Or, if you're timestamp is coming from a database, you can do it directly in the query: http://dev.mysql.com/doc/refman/5.1/en/date-and-time-functions.html#function_date-format
-
Why are you adding the javascript after? Why not have them disabled by using the disabled attribute?
Newbie: can't access a method return value.
in PHP Coding Help
Posted
Well are you actually assigning the return value of the method to anything? Or you should be able to pass the return value directly into the var_dump() function:
var_dump($associacao_dao->listar($limit, $offset));