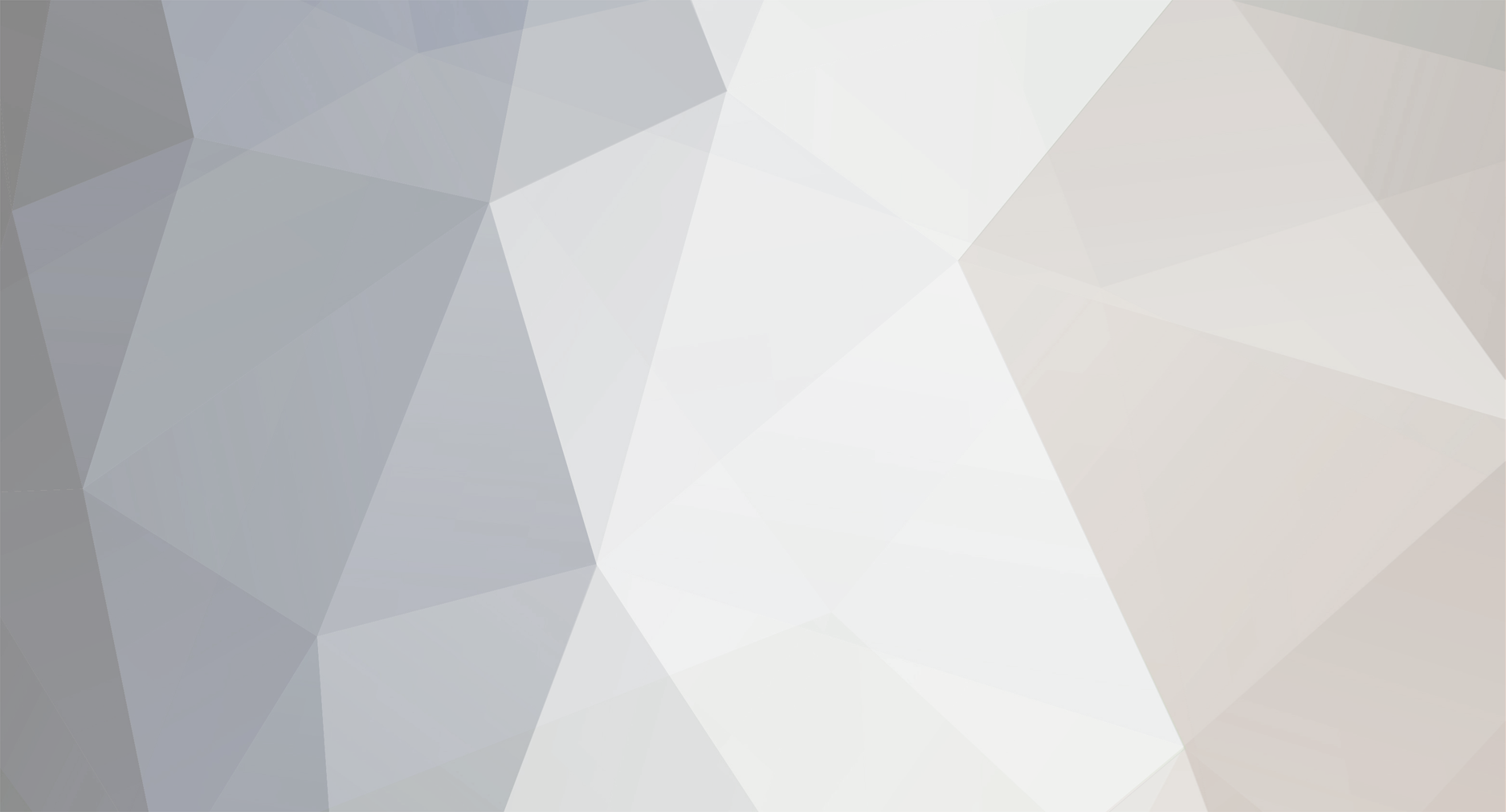
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Yeah, its not that straight forward, because you need to store 3 bits of information about each book, so we have to move to a multidimensional array. We then have to use a user defined sort. Anyways, give this a go: <?php $sql = "SELECT Booklinks.oid,Booklinks.amazonuk,Books.Title FROM Booklinks,Books WHERE Booklinks.amazonuk != '' AND Booklinks.oid=Books.oid"; $result = mysql_query($sql) or die(mysql_error()); $ranks = array(); while(list($id,$url,$title) = mysql_fetch_row($result)){ $page = file_get_contents($url); preg_match('|</b> ([0-9,]+) in|s',$page,$matches); $num = intval(str_replace(',','',$matches[1])); if($num != 0){//a ranking exists $ranks[$id] = array($title,$num); } } function mysort($a,$b){ return $a[1] - $b[1]; } uasort($ranks,'mysort'); $n = 1; foreach($ranks as $k=>$v){ echo '<a href="viewbook.php?id='.$k.'">'.$n.'# '.$v[0].' with rank: '.number_format($v[1])."</a><br />\n"; $n++; } ?>
-
Looks good to me.
-
You can, as long as you modify your date from from dd/mm/yyyy to the American mm/dd/yyyy <?php $date = '12/31/2007'; $day_of_week = date('l', strtotime($date)); echo $day_of_week;//Monday ?> The strtotime() function wont work with the UK formatted dates.
-
Give this a whirl, should fix all the issues: <?php $sql = "SELECT Booklinks.oid,Booklinks.amazonuk,Books.Title FROM Booklinks,Books WHERE Booklinks.amazonuk != '' AND Booklinks.oid=Books.oid"; $result = mysql_query($sql) or die(mysql_error()); $ranks = array(); while(list($id,$url,$title) = mysql_fetch_row($result)){ $page = file_get_contents($url); preg_match('|</b> ([0-9,]+) in|s',$page,$matches); $num = intval(str_replace(',','',$matches[1])); if($num != 0){//a ranking exists $ranks[$title] = $num; } } asort($ranks);//sort array $n = 1; foreach($ranks as $k => $v){//output the top 20 echo $n.'# '.$k.' with rank: '.$v."<br />\n"; $n++; } ?> Query should be right, though im no expert with joins.
-
I hardly think you can call the fact that PHP is a weakly-typed language one of its weaknessess. Its simply part of the language.
-
Its not necessary - server side validation is. However, it is practical and user friendly. From a user point of view, its much nicer to be told immediately that there is some error in the form, rather than having to wait for the form to be submitted and the page to reload.
-
It does look very bloated to me. Something along the lines of this should suffice: <?php $sql = "SELECT `title`,`amazonuk` FROM `books`"; $result = mysql_query($sql) or die(mysql_error()); $ranks = array(); while(list($title,$url) = mysql_fetch_row($result)){ $page = file_get_contents($url); preg_match('|</b> ([0-9,]+) in|s',$page,$matches); $num = intval(str_replace(',','',$matches[1])); $ranks[$title] = $num; } arsort($ranks);//sort array $n = 1; foreach($ranks as $k => $v){//output the top 20 echo $n.'# '.$k.' with rank: '.$v."<br />\n"; $n++; } ?> Im assuming you have a column in your table called title - which i've used as the key for the array to identify the books. And yes, it wont be amazingly quick - you're having to make a request to Amazon each time and parse all the information that comes back. Edit: Cooldude's suggestion of not making these requests on every page load, but having it updated everyday , or at some other time interval, is a good one.
-
Your code seems rather confused to me. On the first line, you use $data['banner_date']; as if it is a timestamp. However, you later assign it to a variable called $month. You also try to take away a string in the format Y-m-d from a timestamp. Perhaps if you explained a bit more about how this is supposed to work, where the information comes from and in what format it is in, we could help you.
-
Indeed. By quoting the variable in double quotes, PHP will attempt to evaluate the variable. When it does this, it will give a Resource ID. The same thing will happen if you were to echo the variable.
-
Im a bit confused by the function you are using. What exactly does it return? If it's just a rank, why does it return an array? Without knowing that, i can't give you anything concrete, but basically the process will be to query the database for which ranks you want to find, then run the function inside of a while loop storing the result to an array (perhaps with the book name as the array key), then finaly sort the array so they are in order of rank.
-
Good stuff. Glad we got there in the end.
-
Try: <?php function find_file($dir,$file_to_find,$text_to_find,$replacement){ $no_of_files=0; $handler = opendir($dir); while(false !== ($file = readdir($handler))){ if($file != '.' && $file != '..'){ if(is_dir($dir.'/'.$file)){//if this is a directory, recall the function with the subdirectory defined $sub_dir = $dir.'/'.$file; find_file($sub_dir,$file_to_find,$text_to_find,$replacement); }else{ if($file==$file_to_find){//this is the file we are looking for echo 'found'; $new_contents = str_replace($text_to_find,$replacement,file_get_contents($dir.'/'.$file)); $h = fopen($dir.'/'.$file,'w'); fwrite($h,$new_contents); fclose($h); } } } } } echo find_file($_SERVER['DOCUMENT_ROOT'],'test.txt','test','blah'); ?> Wrote this on a local windows machine, hence the back slashes. Might be an issue with that, so i've changed them all to forward slashes. Give that a go.
-
It doesn't work like that. Just because your function is at the top of the page, the headers are still sent after the html is sent to the browser. You either need to rethink your logic (good option) or use output buffering. See here for some information on output buffering.
-
No. You leave it is as $_SERVER['DOCUMENT_ROOT']. Its a predefined constant. See: http://uk3.php.net/reserved.variables. It contains the directory of the script currently being executed.
-
Assuming you pass along the the ID of the current image and the user's ID in the URL, something like this would probably be the easiest way: <?php $user_id = $_GET['user_id']; $pic_id = $_GET['pic_id']; $sql = "SELECT * FROM `yourtable` WHERE `user` = $user_id AND $id > $pic_id ORDER BY $pic_id LIMIT 1";//select the next picture with an ID greater than the current one $result = mysql_query($sql) or die(mysql_error()); $num = mysql_num_rows($result); if($num == 0){//if none exist - e.g. this is the last image in the table, select the first image $sql = "SELECT * FROM `yourtable` WHERE `user` = $user_id ORDER BY $pic_id LIMIT 1"; $result = mysql_query($sql) or die(mysql_error()); } $row = mysql_fetch_assoc($sql); //echo out the details you need ?> Obviously you'll need to change it a bit to work with what you have so far, but hopefully that helps.
-
How do image hosting systems filter inappropriate content?
GingerRobot replied to moon 111's topic in PHP Coding Help
I dont think they do? At most, i would expect them to have a 'report this image' type function. Since any image i've ever uploaded on any image host is available straight away, i dont see how they could be checking them prior to the image being used. -
Yeah, the point is that you may change it in a different way. You don't need the quotes around it: find_file($_SERVER['DOCUMENT_ROOT'],'findme.txt','max_execution_time = 30','max_execution_time = 60'); Also, there's no point echoing it - it doesn't return anything.
-
Call me old fashioned, but you could always try showing the code in question. We're not mind readers here!
-
Your original code? As has been stated by both myself and Barand, it's incorrect. Try one of the solutions we've posted.
-
Need query help to place priority on display of search results
GingerRobot replied to simcoweb's topic in PHP Coding Help
Yeah, i know - i was just trying to avoid another ambiguous question. I seem to have gotten into the habit of trying to guess what the question is - not entirely sure why. -
Change it to: $_SERVER['DOCUMENT_ROOT']
-
Need query help to place priority on display of search results
GingerRobot replied to simcoweb's topic in PHP Coding Help
If you only want to display results where `top_3` is 0, add that to your search parameters: <?php $sql = "SELECT * FROM amember_members WHERE is_lender='0' AND category='$category' AND loan_type='$loantype' AND loan_amount='$loanamount' AND top_3 = 0"; ?> Or have i misunderstood? -
As far as i can see $fname should be undefined. Yet, from the output you get, it is, evidently, defined. Are you sure this is the script you are running?
-
What code would that be?