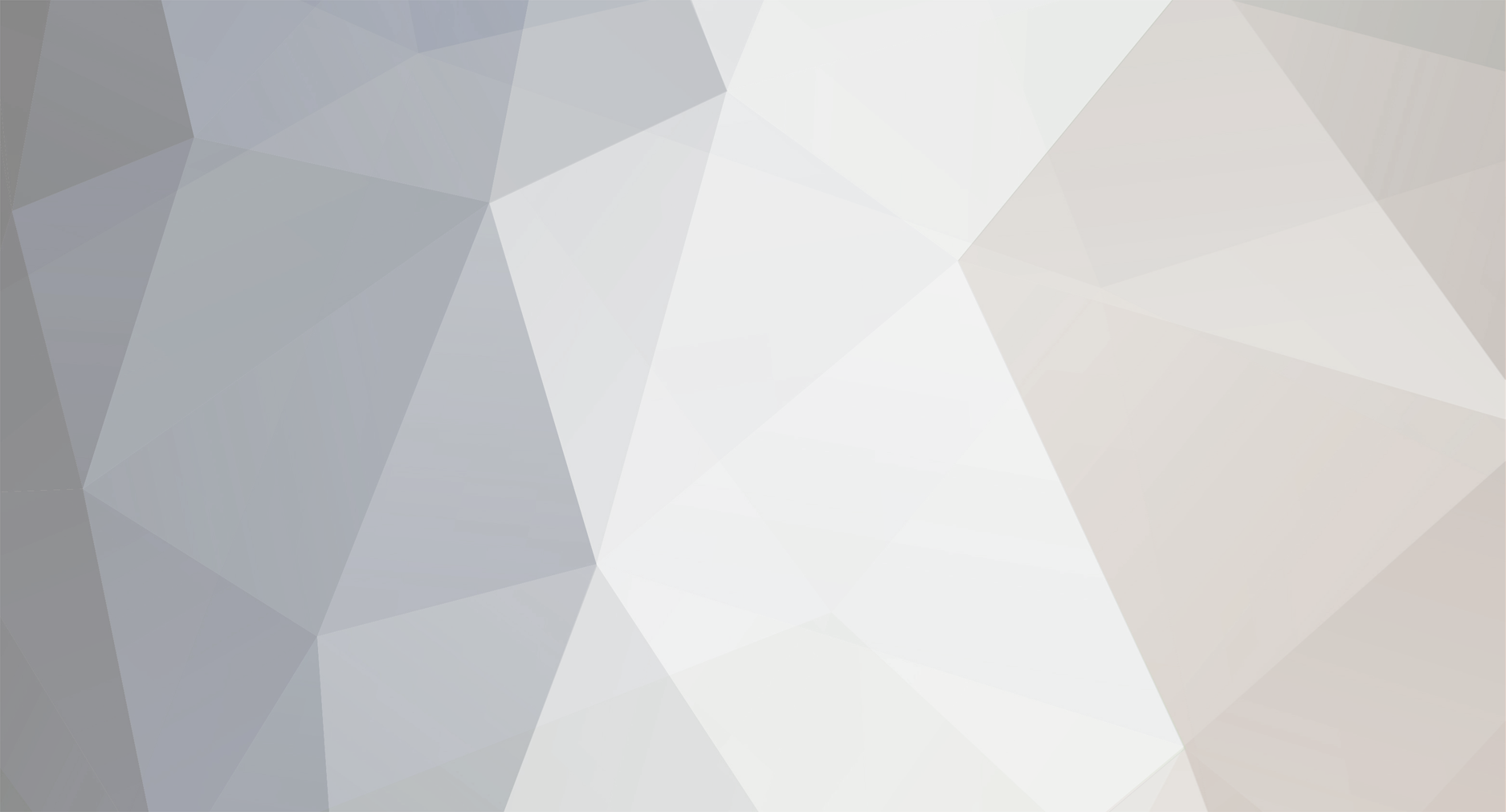
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Sure. Modified something i previously had: <?php function find_file($dir,$file_to_find,$text_to_find,$replacement){ $no_of_files=0; $handler = opendir($dir); while(false !== ($file = readdir($handler))){ if($file != '.' && $file != '..'){ if(is_dir($dir.'\\'.$file)){//if this is a directory, recall the function with the subdirectory defined $sub_dir = $dir.'\\'.$file; find_file($sub_dir,$file_to_find,$text_to_find,$replacement); }else{ if($file==$file_to_find){//this is the file we are looking for $new_contents = str_replace($text_to_find,$replacement,file_get_contents($dir.'\\'.$file)); $h = fopen($dir.'\\'.$file,'w'); fwrite($h,$new_contents); fclose($h); } } } } } echo find_file('C:\wamp\www','test.txt','test','blah'); ?> Would find all files called test.txt in the folder C:\wamp\www and any sub directories, and replace all instances of test with blah.
-
Need query help to place priority on display of search results
GingerRobot replied to simcoweb's topic in PHP Coding Help
Problem is 1s should be on the top? You need to put them in ascending order: <?php $sql = "SELECT * FROM amember_members WHERE is_lender='0' AND category='$category' AND loan_type='$loantype' AND loan_amount='$loanamount' ORDER BY top_3 ASC"; ?> -
Yeah, that would happen. $db_info[name] will contain the same value during each iteration of the while loop. Since you echo it twice in the same iteration, you get the information twice. See here for the FAQ on how to do what you're after.
-
It can, in theory, be done with cURL. In practice, you might run into issues. I can't remember the specifics, but people do have some problems with the file upload via cURL. If you google 'php cURL file upload' or whatever, you should find some examples and a better explanation of the problems you might have.
-
[SOLVED] Basic Question about linking dropdown lists to a new page
GingerRobot replied to Newcomer's topic in PHP Coding Help
When you say echo the results, what do you mean? Are you querying a database with a particular value, or are we talking just how to show which option the user selected? If it's the latter, then it'd be something like: <?php echo $_SERVER['PHP_SELF']; if(isset($_POST['submit'])){ echo 'You selected option: '.$_POST['select']; } ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="POST"> <select name="select"> <option value="one">one</select> <option value="two">two</select> <option value="three">three</select> </select> <input type="submit" name="submit" value="Submit" /> </form> If you're quering a database, its pretty much the same - exept you need to add in the step of quering the database with the value from the drop down, and get the results from the database. -
[SOLVED] clever directory creation on image upload?
GingerRobot replied to delphi123's topic in PHP Coding Help
Yeah, PHP can handle that with the mkdir and the is_dir functions: <?php $y = date('Y'); $m = date('M'); $path_to_images = 'images/'; if(!is_dir($path_to_images.$y)){ mkdir($path_to_images.$y); } if(!is_dir($path_to_images.$y.'/'.$m)){ mkdir($path_to_images.$y.'/'.$m); } $upload_to = $path_to_images.$y.'/'.$m.'/';//this is the folder that you need to upload to ?> -
Whoops, sorry - i'm tired
-
Well, i assume that your message contains a string with no spaces. If all the words had spaces between, it would work. To make it work, remove the spaces in the string that is the 2nd parameter of the explode function: $bad_words = explode(',', 'badword1,badword2,badword3'); A more efficient implementation would be: <?php <?php $black_list = array('badword1','badword2','badword3'); str_replace($blacklist,'#!@%*#',$message); ?> Since it avoids the overhead of the regular expression.
-
Merry Christmas definitely. I'm tired of all this non-christianity rubbish. Apparently some schools are calling it 'Winterval' so as to not offend any other religions. Absolute utter rubbish. Hmm, i had my answer but not a question... Turkey Or Some other meat for your christmas dinner?
-
You can do it all with the GD library. If you google, you'll find plently of tutorials on this.
-
He calls on boxing day? Mine wont be out well into the new year.
-
As for question 2, i assume you refer to something similar to the tags like on this forum? If so, your best bet is to use the htmlentities() function. This will stop any html/javascript code being interpreted. You can then place it inside <pre > </pre > tags to preserve the look of it.
-
You're passing the wrong variable to the mysql_num_rows() function. It should be be $results, not $numresults. Same goes for call to mysql_fetch_array(): $query = "SELECT * FROM Ysers WHERE email LIKE \"%$array%\" ORDER BY email DESC" ; $results=mysql_query ($query) or die (mysql_error()); $row_num =mysql_num_rows ($results); $row= mysql_fetch_array ($results); Dane: FYI, mysql_numrows() is an alias for mysql_num_rows(), though it is depreciated.
-
Again, whats the point in storing images if they're not used anywhere. The point im trying to make is that you'll probably show someone the files at some point, who might link to them etc.
-
As you've pretty much pointed out, it depends entirely on the script. Bad things could occur if you let a script continue to run when there should be some form of error. Consider the pseudocode: if(person doesn't have any enough money){ //show error } //complete purchase Without exiting the script, the purchase is still complete, even though the error is shown. As i said though, wether or not you would run into any problems depends entirely on the script.
-
People often work with error reporting set to ignore notices. You get notices for undefined variables/constants amongst other things. Because they occur with this sort of problem, often the script can continue to run - though it may not always run as expected. For example, this: <?php $array = array('key' =>'some value'); echo $array[key]; ?> Would produce an undefined constant notice. However, PHP can continue to execute the script, because it assumes you meant to enclose the word key in quotes. Setting error reporting to include notices is a good way of finding bugs though. If you made a typo with a variable name somewhere, it'll help you find it.
-
What would be the point in files that weren't linked to anywhere?
-
[SOLVED] Loop results of query into an array
GingerRobot replied to oskom's topic in PHP Coding Help
No problem. I thought it'd be pretty much the right sort of thing. -
To achieve that sort of thing you'll need to look into AJAX. Try: http://www.ajaxfreaks.com/tutorials.php for a starting point. The basic idea is that you'll make a request to another php page, which will return some text which you'll populate your form input with.
-
[SOLVED] Function Names stored in Array
GingerRobot replied to electricshoe's topic in PHP Coding Help
Something like: <?php function somefunction(){ echo 'foo<br />'; } function anotherfunction(){ echo 'bar<br />'; } $array = array('somefunction','notafunction','anotherfunction'); foreach($array as $v){ if(function_exists($v)){ eval($v.'();'); } } ?> Should do it for you. -
[SOLVED] Loop results of query into an array
GingerRobot replied to oskom's topic in PHP Coding Help
For example, here's a modification of something i made before: <?php $sql = "SELECT * FROM `menu` ORDER BY `id`"; $result = mysql_query($sql) or die(mysql_error()); $menu = array(); while($row = mysql_fetch_assoc($result)){ $id = $row['id']; $parentid = $row['parentid']; $link = $row['link'];//location to link to $text = $row['text'];//text of the link $menu[$parentid][$id]['link'] = $link; $menu[$parentid][$id]['text'] = $text; } function menu($menu,$index=0){ echo '<ul>'; foreach($menu[$index] as $k => $v){ echo "<li><a href='".$v['link']."'>".$v['text']."</a></li>"; if(is_array($menu[$k])){ menu($menu,$k); } } echo '</ul>'; } menu($menu); ?> Which works with data like this: -- phpMyAdmin SQL Dump -- version 2.8.2.4 -- http://www.phpmyadmin.net -- -- Host: localhost -- Generation Time: Dec 21, 2007 at 07:57 PM -- Server version: 5.0.24 -- PHP Version: 5.1.6 -- -- Database: `test` -- -- -------------------------------------------------------- -- -- Table structure for table `menu` -- CREATE TABLE `menu` ( `id` int(10) NOT NULL auto_increment, `parentid` int(10) NOT NULL, `link` varchar(255) NOT NULL, `text` varchar(255) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=8 ; -- -- Dumping data for table `menu` -- INSERT INTO `menu` (`id`, `parentid`, `link`, `text`) VALUES (1, 0, '/index.php', 'Home'), (2, 0, '/contactus.php', 'Contact Us'), (3, 0, '/members/index.php', 'Members'), (4, 1, '/about.php', 'About'), (5, 2, '/chat/index.php', 'Live Chat'), (6, 5, '/chat/connected.php', 'Live Help'), (7, 2, '/emailform.php', 'Email'); -
[SOLVED] Loop results of query into an array
GingerRobot replied to oskom's topic in PHP Coding Help
I'm a little confused as to what the displayOrder is all about? Perhaps some sample data from your database might be helpful. Unless i've misunderstood, i think you might have overcomplicated the problem. Ordering by parent ID then by the ID should achieve the correct order, and then it'd just be case of manipulating that into the correct arrays. But as I say, im unsure if this is exactly what you're after. -
[SOLVED] Help from those that are in school
GingerRobot replied to jordanwb's topic in Miscellaneous
I just use the boot of my car to keep my stuff in. So, if you wanted to add wheels, an engine and 4 seats to your locker design... -
How's about: <?php $str="line1 line2 line3 line4"; $insert = "new line"; $lines = explode("\n",$str); $num = count($lines); $insert_after = mt_rand(0,count($lines)-1); $new_str = ''; foreach($lines as $k => $v){ $new_str = $new_str."\n".$v; if($k==$insert_after){ $new_str = $new_str."\n".$insert; } } echo nl2br($new_str); ?>