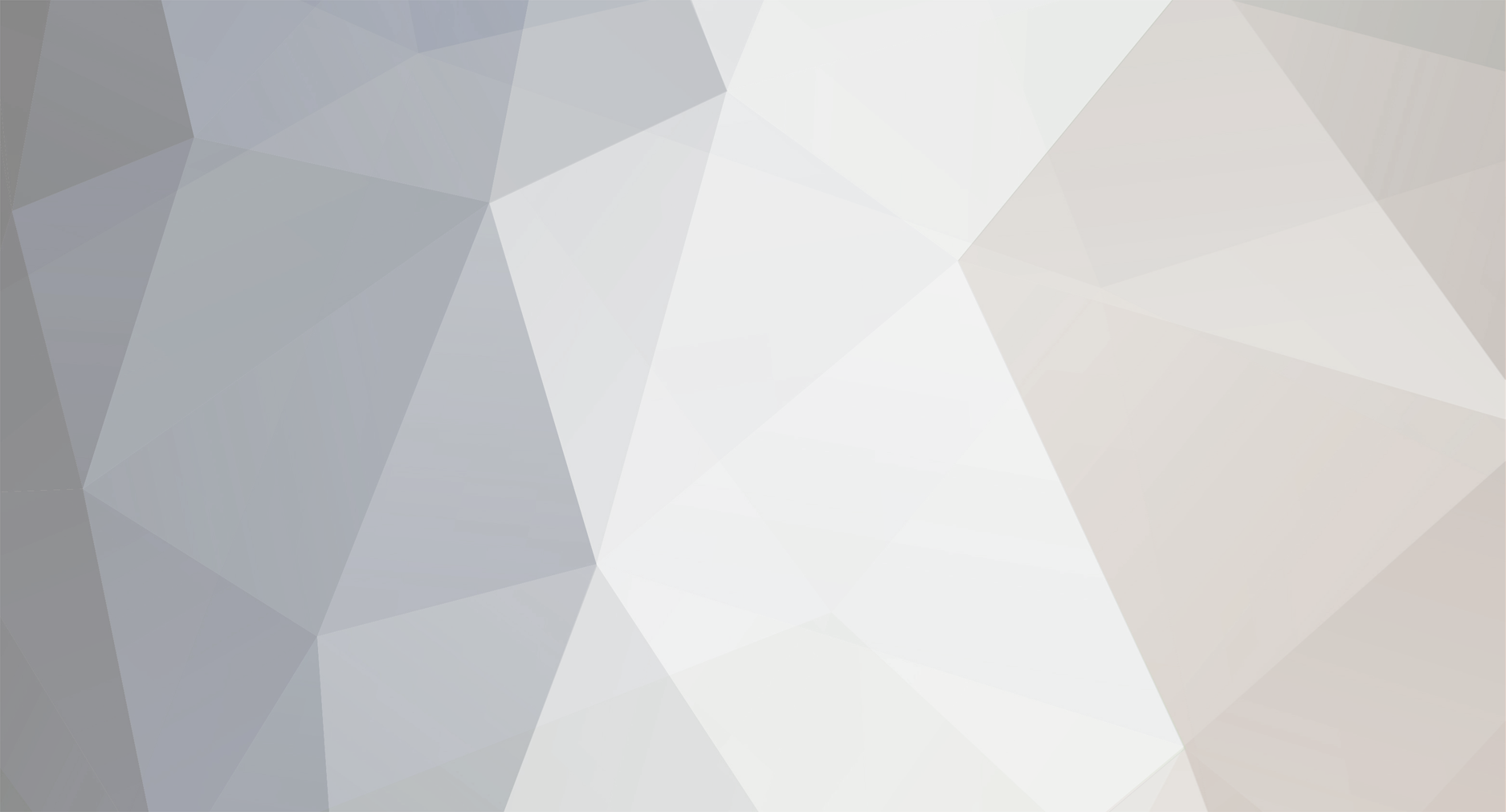
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Ok, its returning the same thing that a normal mysql_query would do - a result resource. Try: $sql = "SELECT MAX(element_key) AS max_element_key, MAX(matrix_num) AS max_matrix_num FROM matrix"; $matrixResult = mysql_db_query($db,$sql,$cid); $result = mysql_fetch_assoc($maxtrixResult); echo $result['max_element_key'];
-
You'll need to use AJAX if you want to avoid an entire page reload. If you google, you'll find plently of examples. Wether or not you'll find something showing exactly what you want to do, i dont know, but the process will be the same - the javascript will make a request to a php page which will run the system command for you.
-
Help me and my multiple buttons in one form problem
GingerRobot replied to ternto333's topic in PHP Coding Help
The action of your form shouldn't be the javascript function - it should be in the onClick attribute: e.g. onClick="return javascriptFunction();" -
Well, you're using a user defined function, mysql_db_query - i dont know what it returns - does it return an array?. If you're not sure either, then try: $sql = "SELECT MAX(element_key) AS max_element_key, MAX(matrix_num) AS max_matrix_num FROM matrix"; $result = mysql_db_query($db,$sql,$cid); var_dump($result); And see what you get
-
Help me and my multiple buttons in one form problem
GingerRobot replied to ternto333's topic in PHP Coding Help
I assume you are setting the value of document.pressed with an onClick attribute of the buttons? I'm just wondering if the form's default action is peptide_view_csv.php and there's an issue with the change of form action. -
So basically you need to work out which items the user has entered a value into the Qty field? Without knowing what you're doing with these results, its a little difficult to give something specific. But this would be the general idea: <?php foreach($_POST['Qty'] as $k => $v){ if(strlen($v) > 0 && $v!=0 && ctype_digit($v)){//if the field contains some value, which is not 0 but is an integer echo 'User ordered '.$v.' of item with ID no:'.$_POST['Id'][$k]; } } ?>
-
Well, there's a missing while condition and a missing closing brace to end your function definition. To fix the parse errors, try: <?php require_once("functions.php"); function docleanup() { global $torrent_dir, $signup_timeout, $max_dead_torrent_time, $autoclean_interval; set_time_limit(0); ignore_user_abort(1); do { $res = mysql_query("SELECT id FROM torrents WHERE external = 'no'"); $ar = array(); while ($row = mysql_fetch_array($res)) { $id = $row[0]; $ar[$id] = 1; } if (!count($ar)) break; $dp = @opendir($torrent_dir); if (!$dp) break; $ar2 = array(); while (($file = readdir($dp)) !== false) { if (!preg_match('/^(\d+)\.torrent$/', $file, $m)) continue; $id = $m[1]; $ar2[$id] = 1; if (isset($ar[$id]) && $ar[$id]) continue; $ff = $torrent_dir . "/$file"; } closedir($dp); if (!count($ar2)) break; $deadtime = deadtime(); mysql_query("UPDATE snatched SET seeder='no' WHERE seeder='yes' AND last_action < FROM_UNIXTIME($deadtime)"); $deadtime = time() - $signup_timeout; mysql_query("DELETE FROM users WHERE status = 'pending' AND added < FROM_UNIXTIME($deadtime) AND last_login < FROM_UNIXTIME($deadtime) AND last_access < FROM_UNIXTIME($deadtime) AND last_access != '0000-00-00 00:00:00'"); $deadtime = time() - $signup_timeout; $user = mysql_query("SELECT invited_by FROM users WHERE status = 'pending' AND added < FROM_UNIXTIME($deadtime) AND last_access = '0000-00-00 00:00:00'"); $arr = mysql_fetch_assoc($user); if (mysql_num_rows($user) > 0) { $invites = mysql_query("SELECT invites FROM users WHERE id = $arr[invited_by]"); $arr2 = mysql_fetch_assoc($invites); if ($arr2[invites] < 10) { $invites = $arr2[invites] +1; mysql_query("UPDATE users SET invites='$invites' WHERE id = $arr[invited_by]"); } mysql_query("DELETE FROM users WHERE status = 'pending' AND added < FROM_UNIXTIME($deadtime) AND last_access = '0000-00-00 00:00:00'"); } $secs = 28*86400; $dt = sqlesc(get_date_time(gmtime() - $secs)); mysql_query("DELETE FROM messages WHERE added < $dt"); function autoinvites($length, $minlimit, $maxlimit, $minratio, $invites) { $time = sqlesc(get_date_time(gmtime() - (($length)*86400))); $minlimit = $minlimit*1024*1024*1024; $maxlimit = $maxlimit*1024*1024*1024; $res = mysql_query("SELECT id, invites FROM users WHERE class > 0 AND enabled = 'yes' AND downloaded >= $minlimit AND downloaded < $maxlimit AND uploaded / downloaded >= $minratio AND warned = 'no' AND invites < 10 AND invitedate < $time") or sqlerr(__FILE__, __LINE__); if (mysql_num_rows($res) > 0) { while ($arr = mysql_fetch_assoc($res)) { if ($arr[invites] == 9) $invites = 1; elseif ($arr[invites] == 8 && $invites == 3) $invites = 2; mysql_query("UPDATE users SET invites = invites+$invites, invitedate = NOW() WHERE id=$arr[id]") or sqlerr(__FILE__, __LINE__); } } } autoinvites(10,1,4,.90,1); autoinvites(10,4,7,.95,2); autoinvites(10,7,10,1.00,3); autoinvites(10,10,100000,1.05,4); $torrents = array(); $res = mysql_query("SELECT torrent, seeder, COUNT(*) AS c FROM peers GROUP BY torrent, seeder"); while ($row = mysql_fetch_assoc($res)) { if ($row["seeder"] == "yes") $key = "seeders"; else $key = "leechers"; $torrents[$row["torrent"]][$key] = $row["c"]; } $res = mysql_query("SELECT torrent, COUNT(*) AS c FROM comments GROUP BY torrent"); while ($row = mysql_fetch_assoc($res)) { $torrents[$row["torrent"]]["comments"] = $row["c"]; } $fields = explode(":", "comments:leechers:seeders"); $res = mysql_query("SELECT id, seeders, leechers, comments FROM torrents WHERE external = 'no'"); while ($row = mysql_fetch_assoc($res)) { $id = $row["id"]; $torr = $torrents[$id]; foreach ($fields as $field) { if (!isset($torr[$field])) $torr[$field] = 0; } $update = array(); foreach ($fields as $field) { if ($torr[$field] != $row[$field]) $update[] = "$field = " . $torr[$field]; } if (count($update)) mysql_query("UPDATE torrents SET " . implode(",", $update) . " WHERE id = $id"); } }while($var == 'somevalue');//replace with your condition } ?> However, i wouldn't be at all surprised if this doesn't run the way you want it to - i would imagine there is some logic errors in there somewhere. Without proper indentation, you're going to cause yourself all sorts of problems. And don't forget to change the while condition from the one i added in. Edit: p.s. Its a dollar sign.
-
[SOLVED] Is it possible to strip string formats?
GingerRobot replied to Solarpitch's topic in PHP Coding Help
strip_tags()? -
Pointless debates are the best ones!
-
From: http://dev.mysql.com/doc/refman/5.0/en/what-is-mysql.html I dont know where your mentor gets his information, but, im sorry, its wrong. AFAIK, pretty much the only two pronunciations ever used are either My Ess Que Ell (e.g. My S-Q-L) or My Sequel.
-
I think you're probably making this more complicated than necessary. Is there actually another date on that page? If not, this would suffice: <?php $str = '<table width="100%" border="0" cellpadding="5" cellspacing="0" class="table th" style="border:10px #016801 solid;"> <tr> <th width="41%" bgcolor="#319a00" style="border:none;"> <div align="left">Current Status </div></th> <th width="59%" bgcolor="#319a00" style="border:none;"> <div align="right">Pro Number <span>I894451293</span> </div></th> </tr> <td bgcolor="#fcf920" colspan="2"><span class="current-status">This shipment was delivered on time on 11/02/07 AT 12:57PM- EST</span></td> </table>'; preg_match('|[0-9]{2}/[0-9]{2}/[0-9]{2}|',$str,$matches); print_r($matches); ?> Edit: If there is another date, then use: <?php $str = '<table width="100%" border="0" cellpadding="5" cellspacing="0" class="table th" style="border:10px #016801 solid;"> <tr> <th width="41%" bgcolor="#319a00" style="border:none;"> <div align="left">Current Status </div></th> <th width="59%" bgcolor="#319a00" style="border:none;"> <div align="right">Pro Number <span>I894451293</span> </div></th> </tr> <td bgcolor="#fcf920" colspan="2"><span class="current-status">This shipment was delivered on time on 11/02/07 AT 12:57PM- EST</span></td> </table>'; preg_match('|<span class="current-status">.*([0-9]{2}/[0-9]{2}/[0-9]{2})|',$str,$matches); $date = $matches[1]; echo $date; ?>
-
I agree - I just made the easiest change
-
How about the query: SELECT * FROM `yourtable` WHERE UNIX_TIMESTAMP(`yourfield`) BETWEEN UNIX_TIMESTAMP() AND UNIX_TIMESTAMP()+60*60*24*14 It does beg the question of why you are storing a date in the datetime format, but in a text field - use a datetime field!
-
We're still missing two semi-colons. When you get that sort of error, always take a look at the line before too - it's a VERY common mistake. <?php include "mice.php"; mysql_connect($server, $db_user, $db_pass) or die (mysql_error()); mysql_select_db("mysql_dbname"); $abc = $_GET['id']; $sql = "select * from items WHERE id = '$abc'"; $result = mysql_query($sql) or die("Problem with the query: $sql<br>" . mysql_error()); $row = mysql_fetch_array($result); $Title = $row['Title']; $code1 = $row['code1']; $pass = $row['pass']; $email = $row['Email']; $mymail = $email; $SubJect = 'Edit your myroho posting'; $FrOm = "admin@myroho.com"; $BoDy = "You made a posting called $Title on myroho\n\n"; $BoDy .= "You can now edit or delete them on the site, just follow the instructions below.\n\n"; $BoDy .= "To edit your posting, visit:\n"; $BoDy .= "http://www.myroho.com/edit_step_one.php\n\n"; $BoDy .= "To delete your posting, visit:\n"; $BoDy .= "http://www.myroho.com/delete_step_one.php\n\n"; $BoDy .= "To make the amendments you need the following posting reference:$code1\n"; $BoDy .= "and the following password:$pass\n"; $BoDy .= "Please save these details in a folder so you can edit or delete your posting.\n\n"; $BoDy .= "When you make future postings you will be able to choose your own password.\n\n"; $BoDy .= "Please note: We reserve the right to refuse or delete postings that we believe are inappropriate or which breach our terms and conditions.\n\n"; $BoDy .= "Best Wishes\n"; $BoDy .= "the myroho team\n"; $send = mail($mymail, $SubJect, $BoDy, "From: $FrOm"); } } ?> Edit: Beaten to it, but there's the other line without a semi colon - mysql_select_db("mysql_dbname") - so posted anyway
-
Well, very simply, something like this might suffice: <?php $files = array(); $path_to_folder = 'path/to/your/folder/of/blogs/';//where is this folder? foreach(glob($path_to_folder.'*.php') as $file){//cycle through each .php file in that folder - i assume it ONLY contains blogs? $files[] = $file; } rsort($files);//sort our files foreach($files as $file){ include($file); echo '<br />'; } ?> Given the consistant naming format, sorting by the file name will work fine to sort them by date/ I'm assuming you do want to include the files, since you said they are .php files - rather than plain text files which you'll want to grab with file_get_contents() and echo. Personally, i think you're far better off using a database for storing your blogs though.
-
You're missing a semi colon at the end of this line: $row = mysql_fetch_array($result)
-
Try: <?php $list_ignore = array ('.','..','exemples','phpmyadmin','sqlitemanager'); $msg = $langues[$langue]['txt_no_projet']; $folders = array(); //Open the file system object for reading $handle=opendir("."); //Iterate through the file objects and put the folders into an array while ($file = readdir($handle)) { if (is_dir($file) && !in_array($file,$list_ignore)) { $folders[] = $file; } } //Close the file system object closedir($handle); //Sort the folder array sort($folders); //Iterate through the array and display the folders foreach ($folders as $file) {//note $file not $folder $msg = ''; echo "<a class=\"ditem\" href=\"$file\">"; echo "<img src=\"dossier.gif\" alt=\"Folder Icon\" title=\"Folder Icon\" class=\"noBorderImg\" /> "; echo "$file</a><br />"; } echo $msg; ?>
-
Just took a look at a quick test i put together for a post on the forum the other day - the AJAX request seems to work perfectly fine for me in firefox. Running 2.0.0.9 without the firebux extension. The javascript it uses is the same as can be found here: http://www.ajaxfreaks.com/tutorials/1/0.php
-
You've got a duplicate double quote. Change this line: echo "<img src=\"header.jpg"" alt=\"\" />"; to: echo "<img src=\"header.jpg\" alt=\"\" />"; Edit: Or better yet, change the whole lot to: <?php if($show_header == "no"){ echo '<img src="header.jpg" alt="" />'; } else{ echo '<img src="flashy_header.jpg" alt="" />'; } ?> We don't need the double quotes to surround the string, since we're not echoing any variables. So lets use single quotes and make things a bit easier to read.
-
Well, what is it you are actually trying to do? You may be better off not re-inventing the wheel, and using an existing site's API. I see mapquest offers one, and im pretty sure google maps do too.
-
No problem. Can you mark the topic as solved please?
-
Im a bit confused as to what you want your array to contain? Is it supposed to be like: $example_data = array( array(year,metric tons), array(year2,metric tons2), ); If so, try: <?php mysql_select_db($database_conn_imni, $conn_imni); $query_rs = "SELECT `year`, metric_tons FROM tbl_data_annual"; $rs = mysql_query($query_rs, $conn_imni) or die(mysql_error()); $array = array(); while(list($year,$tons) = mysql_fetch_row($rs)){ $array[] = array($year,$tons); } echo '<pre>'.print_r($array,1).'</pre>';//lets check the output to make sure its what we ned ?>
-
[SOLVED] Help with parsing data in text file
GingerRobot replied to speaker219's topic in PHP Coding Help
Well, if you know exactly what data you want, then there's no need for all these foreach loops. Try: <?php $str = file_get_contents('http://207.210.102.183/tracker.txt'); $bits = explode('---',$str); $station14 = $bits[13];//array key is one less than station number, since keys start from 0 $station15 = $bits[14]; $lines = explode("\n",$station14);//explode by the new line $artist14 = $lines[3];//the lines we want are the third and fourth from each station $track14 = $lines[4]; $lines = explode("\n",$station15);//repeat for station 15 $artist15 = $lines[3]; $track15 = $lines[4]; echo "Station 14<br />Artist: $artist14<br />Track: $track14<br /><br />Station 15<br />Artist: $artist15<br />Track: $track15"; ?> -
Indeed. As i say, using firefox with the live HTTP headers extension makes that sort of thing much easier to spot.