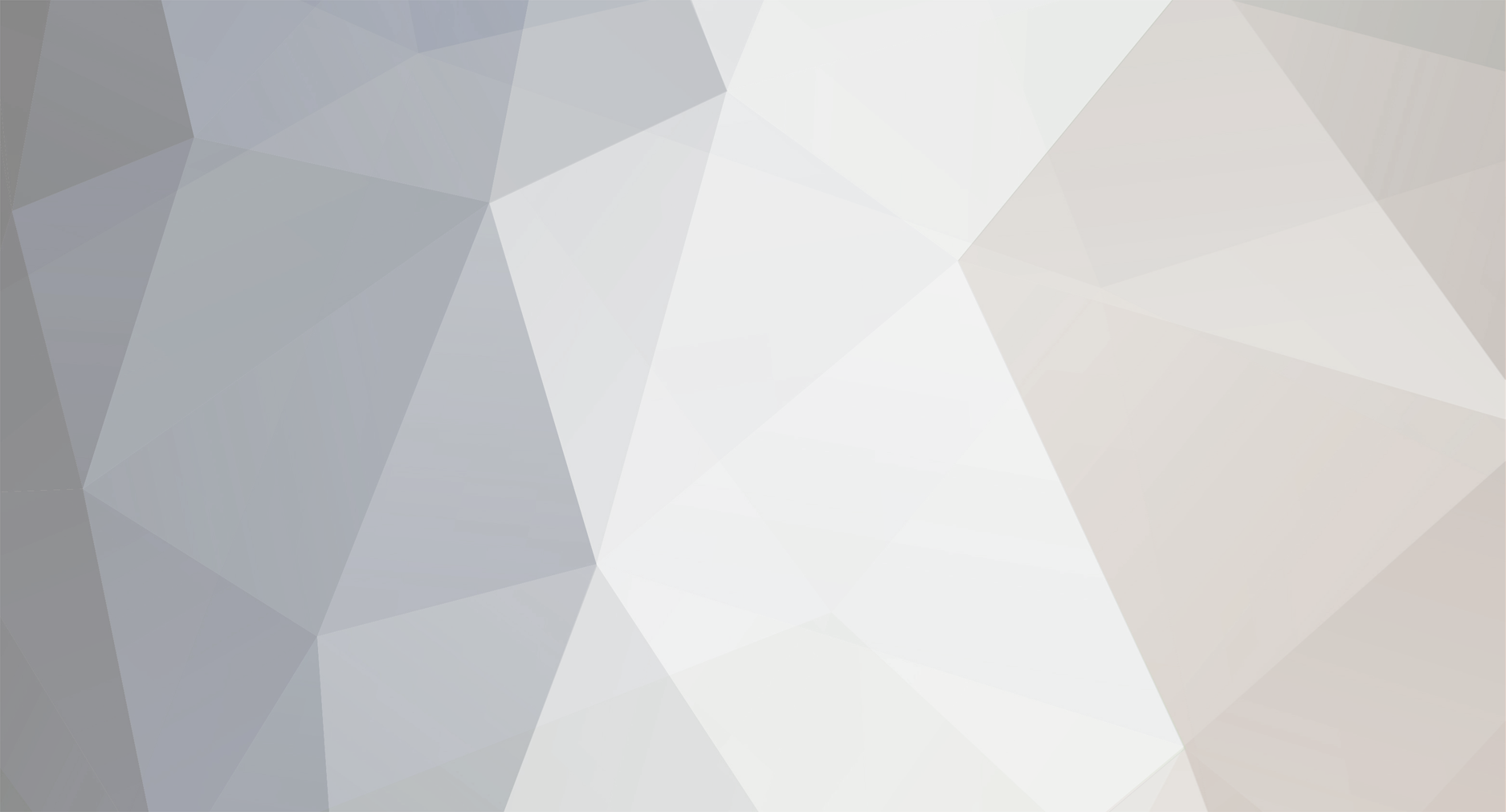
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
One thing that stands out immediately to me (perhaps only because i made the same mistake, and spent ages looking for it!) is that in your postfields, you do not set the value of the submit button. You should have something like "login=12345&password=56789&submitbuttonname=submitbuttonvalue" Imagine that the site you are accessing is written in PHP: <?php if(!isset($_POST['submit']){ //redirect to form page }else{ //process form } ?> This could explain why you are being sent back to the login page. Otherwise, i suggest you get firefox, and the live HTTP headers extension which is very useful for finding out exactly what is being passed to the host.
-
[SOLVED] rand() Returning Priviously selected values.
GingerRobot replied to centerwork's topic in PHP Coding Help
The way i'd do it: Two tables in your database, one containing those numbers you don't want selected, and your table that you are trying to retrieve a random result from. Im suggeting using a table for the excluded numbers since i assume this will change. So, we have the table `excluded` containing a single column `number`. We then use the query: SELECT * FROM yourtable LEFT JOIN excluded ON excluded.number = yourtable.id WHERE excluded.number IS NULL ORDER BY RAND() LIMIT 1 Which selects one row, randomly ordered, where the id of our table is not in the excluded table. You would then update the excluded table with the id of the row retrieved. -
See this topic from yesterday, or this FAQ for AJAX solutions.
-
Well you're not actually submitting a form are you? You're simply using a button which calls a javascript function which creates what, a pop up window? Im sure the best way would be to pass a parameter to your javascript function.
-
Yeah, its A*-G or unclassified.
-
display page, wait , then forward to another page
GingerRobot replied to boom's topic in PHP Coding Help
You can't do it with PHP. PHP is a server side language. By the time output is shown in the browser, your php code has been executed. To do a time-delayed re-direct, use a meta refresh: <meta http-equiv="refresh" content="5;url=http://example.com" /> Above code would redirect to example.com after 5 seconds. -
There's really no need to bumb after half an hour. Anyway, it looks like this is more to do with the javscript you're using. I've no idea how that works, so i can't say if it's possible to pass the song url to the player. Hopefully, you could simply add a parameter to your open_win() function, which contains the url.
-
[SOLVED] using arrays with mysql query results
GingerRobot replied to Optimo's topic in PHP Coding Help
Well do it like this then, and see how you would use it: <?php $sql = mysql_query("SELECT * FROM `yourtable`") or die(mysql_error()); $unit_data = array(); while($row = mysql_fetch_assoc($sql)){ $id = $row['id']; $unit_data[$id]['name'] = $row['name']; $unit_data[$id]['crew'] = $row['crew']; $unit_data[$id]['type'] = $row['type']; $unit_data[$id]['money'] = $row['money']; $unit_data[$id]['deterium'] = $row['deterium']; } print_r($unit_data[1]);//print out Lance's data foreach($unit_data[2] as $v){ //cycle through the second set of data } $total = 0; foreach($unit_data as $v){ $total += $v['money']; } echo 'Total cost: '.$total;//show the total of all the money fields ?> -
Yeah, thats fine - you can only insert one row at a time anyway. Though it should be: foreach ($array as $value) Note the $ sign. Also, your signiture should really read $AXiSS = $Graphic_Designer;, with a single equals sign. Unless it was deliberate of course
-
Well, GetSQLValueString() is a user defined function. I've no idea what it does. However, the problem would seem to be that it is expecting a string, but you are passing an array to it. Since you are selecting multiple things (from a drop down box i assume?) it is an array. Its a bit difficult to give help. I've no idea what your code does/is supposed to do.
-
When you include a file, what effectively happens is that all of the code inside the included file is dumped inside the file doing the including. Therefore, you need to place this line: $this_page = $_SERVER['PHP_SELF']; Before the include is done, and take it out of header.php We're supposed to guess that question from the subject 'pretty simple question'? You might be clairvoyant - im not though.
-
Slight change of subject there. I suggest you google - im sure you'll find what you need.
-
[SOLVED] using arrays with mysql query results
GingerRobot replied to Optimo's topic in PHP Coding Help
Well, i *think* from your question what you're after is: <?php $sql = mysql_query("SELECT * FROM `yourtable`") or die(mysql_error()); $x = 0; $unit_names = array(); $unit_crew = array(); $unit_type = array(); $unit_money = array(); $unit_deterium = array(); while($row = mysql_fetch_assoc($sql)){ $unit_names[] = $row['name']; $unit_crew[] = $row['crew']; $unit_type[] = $row['type']; $unit_money[] = $row['money']; $unit_deterium[] = $row['deterium']; echo '<br/>x='.$x'<br />Unit Name: '.$row['name'].'<br />Unit Deterium: '.$row['deterium'].'<br />Unit type:'.$row['type'].'<br />Unit Money: '.$row['money'].'<br />Unit Crew:'.$row['crew'].'<br /><br />'; $x++; } ?> You'd then use the relevant array and key to get the information you need further on in the script. I would suggest, however, that you use the id field (that i hope you have) as the key for the arrays. It would make things easier to keep track of. -
You can either set in your php.ini file or you can use the error_reporting() function if you only want to make the changes for one particular script.
-
[SOLVED] Schizophrenic PHP under Win2003 server
GingerRobot replied to rekabis's topic in PHP Coding Help
If you want an easy ride, try wamp -
Array keys which are not integers should be enclosed in single quotes, e.g.: $_SESSION['online'] You'll see them not done like this a lot of the time, since you only recieve a notice for this kind of minor error, and a lot of people have PHP set up not to report notices.
-
Edit: Ignore that As far as i know, you can't do anything using if, elseif, else statements in ternary form. Even if you could, it'd get pretty messy.
-
[SOLVED] Problems with dynamic drop down menu
GingerRobot replied to scarlson's topic in PHP Coding Help
Nearly - you need to put it outside the loop: <?php //connect to database $sql = "SELECT `id`,`state` FROM `states`";//select all our states $result = mysql_query($sql) or die(mysql_error()); echo '<select name="state" onChange="sendRequest(this.value)">';//the select box with the javascript function to change the cities echo '<option value="none">Select a State</option>'; while(list($id,$state) = mysql_fetch_row($result)){ echo '<option value="'.$id.'"'.'>'.$state.'</option>'; [color=red]//How do i add a "select state" option first????[/color] } echo '</select>'; ?> <br /><br /> <div id="container"> <select name="cities"> <option value="unselected">Please select a state first</option> </select> </div> -
[SOLVED] Problems with dynamic drop down menu
GingerRobot replied to scarlson's topic in PHP Coding Help
So Alabama is selected by default? Either add an option as the first option saying 'please select a state' or select the cities which are from alabama and display them in the cities text box to start with, rather than the current 'please select a state' message. Since the javascript action occurs when a change is made, having alabama first means it wont load the cities. -
[SOLVED] i need to make a member feature
GingerRobot replied to mybluehair's topic in PHP Coding Help
There are literally hundreds of membership system tutorials out there - i suggest you google. There used to be one on this site, though i can't seem to find it at the moment. -
You will need to escape the first two double quotes though - <?xml version=\"1.0\"?>
-
[SOLVED] Problems with dynamic drop down menu
GingerRobot replied to scarlson's topic in PHP Coding Help
Good, glad to help. Can you mark the topic as solved please? -
Indeed. Though sometimes experience can be worth more than a degree in my opinion. Of course - we rely on computer programs daily to prevent death.
-
[SOLVED] Problems with dynamic drop down menu
GingerRobot replied to scarlson's topic in PHP Coding Help
Try the following example. I've taken the javascript straight from http://www.ajaxfreaks.com/tutorials/1/0.php - i suggest you take a look there to understand a little more about ajax. This would be the page you want to show the drop downs on: <script type="text/javascript"> function createRequestObject() { var req; if(window.XMLHttpRequest){ // Firefox, Safari, Opera... req = new XMLHttpRequest(); } else if(window.ActiveXObject) { // Internet Explorer 5+ req = new ActiveXObject("Microsoft.XMLHTTP"); } else { // There is an error creating the object, // just as an old browser is being used. alert('Problem creating the XMLHttpRequest object'); } return req; } // Make the XMLHttpRequest object var http = createRequestObject(); function sendRequest(id) { // Open PHP script for requests http.open('get', 'getcities.php?id='+id); http.onreadystatechange = handleResponse; http.send(null); } function handleResponse() { if(http.readyState == 4 && http.status == 200){ // Text returned FROM the PHP script var response = http.responseText; if(response) { // UPDATE ajaxTest content document.getElementById("container").innerHTML = response; } } } </script> <?php //connect to database $sql = "SELECT `id`,`state` FROM `states`";//select all our states $result = mysql_query($sql) or die(mysql_error()); echo '<select name="state" onChange="sendRequest(this.value)">';//the select box with the javascript function to change the cities while(list($id,$state) = mysql_fetch_row($result)){ echo '<option value="'.$id.'"'.'>'.$state.'</option>'; } echo '</select>'; ?> <br /><br /> <div id="container"> <select name="cities"> <option value="unselected">Please select a state first</option> </select> </div> And this is getcities.php: <?php //connect to database $id = $_GET['id']; $sql = "SELECT `id`,`city` FROM `cities` WHERE `state_id`=$id";//select the cities from the given state $result = mysql_query($sql) or die(mysql_error()); //display the contents of our div tag echo '<select name="cities">'; while(list($id,$city) = mysql_fetch_row($result)){ echo '<option value="'.$id.'">'.$city.'</option>'; } echo '</select>'; ?> This is just a very quick example - but hopefully you'll get the general idea. -
You can 'submit' a multipart form with a file using cURL. Never done it myself though.