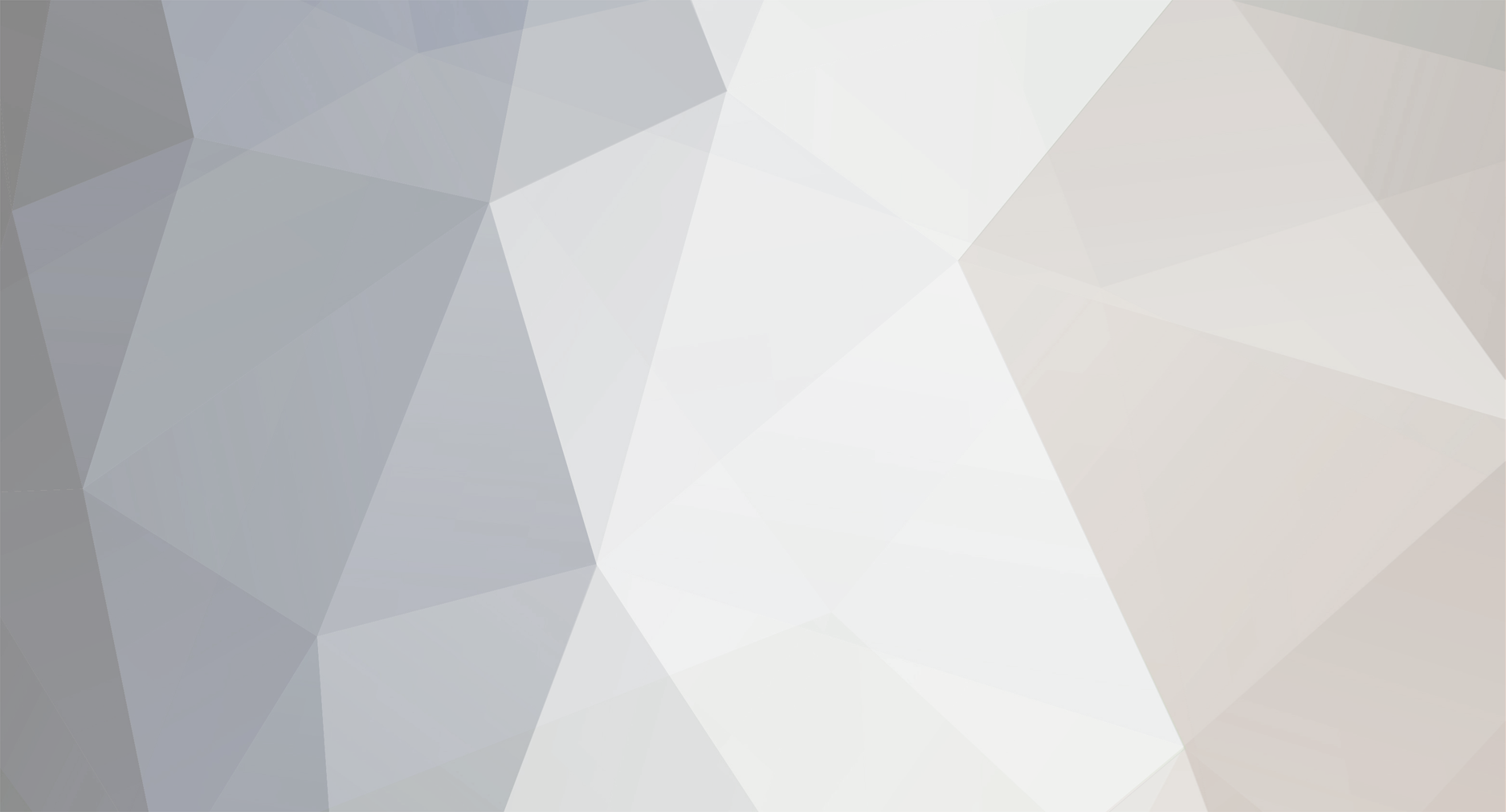
stevochegeoj
New Members-
Posts
7 -
Joined
-
Last visited
Everything posted by stevochegeoj
-
I have a bookings table represented by the code below, i wish to add a mouseover function to display customer details eg. number of persons in a room,meal plan etc.All data is fetched from the database.How can i go about it? <?php session_start(); if (!isset($_SESSION["user"])) { header("location:index.php"); } require_once(__DIR__ . '/db.php'); //Get all the rooms from the database $roomsSQL = "SELECT TRoom FROM room"; $days = 30; $roomsArray = array(); $roomsResult = mysqli_query($con, $roomsSQL); $roomsArray = array(); while ($row = mysqli_fetch_assoc($roomsResult)) { array_push($roomsArray, $row); } //Get all date bound bookings date_default_timezone_set('Africa/Nairobi'); $startDate = $_GET['start_date'] ?? date("Y-m-d"); $endDate = date('Y-m-d', strtotime($startDate . " + $days days")); $sql = "SELECT tbl_events.id, tbl_events.title, tbl_events.start, tbl_events.end, tbl_events.color, room.TRoom\n FROM tbl_events\n INNER JOIN roombook\n ON tbl_events.roombook_id = roombook.id\n INNER JOIN room\n ON roombook.TRoom = room.TRoom\n WHERE tbl_events.start BETWEEN '$startDate' AND '$endDate'\n OR (tbl_events.start < '$startDate' AND tbl_events.end > '$startDate');"; $result = mysqli_query($con, $sql); $bookingsArray = array(); while ($row = mysqli_fetch_assoc($result)) { array_push($bookingsArray, $row); } /** * Gets the color for a cell * * @param string $room the room to check * * @param string $date the date to check * * @param array $bookingsArray the current bookings to iterate through */ function getCellColor(string $room, string $date, array $bookingsArray) { foreach ($bookingsArray as $booking) { if($room == $booking['TRoom'] && $date >= $booking['start'] && $date <= $booking['end']){ return $booking['color']; } } } /** * Gets the content that should be in every single cell * * @param string $room the room to check * * @param string $date the date to check * * @param array $bookingsArray the current bookings to iterate through */ function getCellContent(string $room, string $date, array $bookingsArray) { foreach ($bookingsArray as $booking) { if($room == $booking['TRoom'] && $date >= $booking['start'] && $date <= $booking['end']){ return $booking['title']; } } return null; } function getRowSpan(string $room, string $date, $bookingsArray, $remainingCells) { if(!is_null(getCellContent($room, $date, $bookingsArray))){ $end = null; foreach ($bookingsArray as $booking) { if($room == $booking['TRoom'] && $date >= $booking['start'] && $date <= $booking['end']){ $end = $booking['end']; $endDateDifference = date_diff(date_create($date), date_create($end))->format("%a"); return ($endDateDifference < $remainingCells) ? $endDateDifference : $remainingCells; } } } } function cellAlreadyCovered(string $room, string $date, $bookingsArray, $currentIndex) { if(!is_null(getCellContent($room, $date, $bookingsArray))){ $start = null; foreach ($bookingsArray as $booking) { if($room == $booking['TRoom'] && $date >= $booking['start'] && $date <= $booking['end']){ $start = $booking['start']; $startDateDifference = date_diff(date_create($start), date_create($date))->format("%a"); return $startDateDifference > 0 && $currentIndex != 0; } } } return false; } ?> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <!----> <body> <div id="wrapper"> <!--/. NAV TOP --> <!-- --> <!-- /. NAV SIDE --> <div id="page-wrapper"> <div id="page-inner"> <div class="row"> <div class="col-md-12"> <h1 class="page-header">Booking Table</h1> <div class="table-responsive overflow-auto" style="overflow-x: auto;"> <table class="table table-hover table-bordered" style="font-size: 8px"> <thead> <tr> <th>Days</th> <?php foreach($roomsArray as $room) : ?> <th><?= $room['TRoom'] ?></th> <?php endforeach; ?> </tr> </thead> <tfoot> <tr> <th>Days</th> <?php foreach($roomsArray as $room) : ?> <th><?= $room['TRoom'] ?></th> <?php endforeach; ?> </tr> </tfoot> <tbody> <?php for($i = 0; $i < $days; $i++): ?> <tr> <th><?= date('D, d/m/Y', strtotime($startDate . " + $i days")) ?></th> <?php foreach($roomsArray as $room) : ?> <?php if(!cellAlreadyCovered($room['TRoom'], date('Y-m-d', strtotime($startDate . " + $i days")), $bookingsArray, $i)) : ?> <td rowspan="<?= getRowSpan($room['TRoom'], date('Y-m-d', strtotime($startDate . " + $i days")), $bookingsArray, ($days - $i)) ?>" style="background-color: <?= getCellColor($room['TRoom'], date('Y-m-d', strtotime($startDate . " + $i days")), $bookingsArray) ?>;"> <?= getCellContent($room['TRoom'], date('Y-m-d', strtotime($startDate . " + $i days")), $bookingsArray) ?> </td> <?php endif; ?> <?php endforeach; ?> </tr> <?php endfor; ?> </tbody> </table> </div> <div class="d-flex align-items-center justify-content-between" style="text-align: left"> <a href="table.php?start_date=<?= date('Y-m-d', strtotime($startDate . " - $days days")) ?>" class="btn btn-primary">Previous 7 Days</a> <a href="table.php?start_date=<?= date('Y-m-d', strtotime($startDate . " + $days days")) ?>" class="btn btn-primary ml-2">Next 7 Days</a> </div> <div class="d-flex align-items-center justify-content-between" style="alignment: right"> <a href="table2.php?start_date=<?= date('Y-m-d', strtotime($startDate . " - $days days")) ?>" class="btn btn-primary">Previous 30 Days</a> <a href="table2.php?start_date=<?= date('Y-m-d', strtotime($startDate . " + $days days")) ?>" class="btn btn-primary ml-2">Next 30 Days</a> </div> </div> </div> <!-- /. ROW --> </div> </div> </div> <!-- /. PAGE INNER --> </div> <!-- /. PAGE WRAPPER --> <!-- /. WRAPPER --> <!-- JS Scripts--> <script src="assets/js/jquery-1.10.2.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/morris.js/0.2.7/morris.min.js"></script> <!-- jQuery Js --> <!-- Bootstrap Js --> <script src="assets/js/bootstrap.min.js"></script> <!-- Metis Menu Js --> <script src="assets/js/jquery.metisMenu.js"></script> <!-- DATA TABLE SCRIPTS --> <script src="assets/js/dataTables/jquery.dataTables.js"></script> <script src="assets/js/dataTables/dataTables.bootstrap.js"></script> <script> $(document).ready(function () { //$('#dataTables-example').dataTable(); }); </script> <!-- Custom Js --> <script src="assets/js/custom-scripts.js"></script> </body> </html>
-
<?php $tsql = "select * from roombook ORDER BY FIELD(stat, 'Checked in', 'Booked', 'Deposit Confirmation', 'Email/phone', 'Checked out')"; $tre = mysqli_query($con, $tsql); while ($trow = mysqli_fetch_array($tre)) { $co = $trow['stat']; if ($co != "Cancelled" ) { echo "<tr> <th>" . $trow['FName'] . " " . $trow['LName'] . "</th> <th>" . $trow['Email'] . "</th> <th>" . $trow['TRoom'] . "</th> <th>" . $trow['cin'] . "</th> <th>" . $trow['cout'] . "</th> <th>" . $trow['stat'] . "</th> <th><a href='roombook.php?rid=" . $trow['id'] . " ' class='btn btn-primary'>Action</a></th> </tr>"; } } ?> here it is
-
$tsql = "select * from roombook ORDER BY FIELD(stat, 'Checked in', 'Booked', 'Deposit Confirmation', 'Email/phone', 'Checked out')"; i re wrote my code as above but still it does not give the desired result. however when i run the same code on phpmyadmin it seems to work. what could be the issue?
-
I have this code that i tried running it on my phpmyadmin it works fine ,but when i use it on my php code to query its not working.What could be the issue? SELECT * FROM `roombook` ORDER BY (CASE stat WHEN 'Checked in' THEN 1 WHEN 'Booked' THEN 2 WHEN 'Deposit Confirmation' THEN 3 WHEN 'Email/phone' THEN 4 WHEN 'Checked Out' THEN 5 ELSE 'Cancelled' END) ASC, stat ASC
-
multiple selection in an option list
stevochegeoj replied to stevochegeoj's topic in PHP Coding Help
my problem with this is, the option list is populated from the database. Do you mind implementing the code you posted in the quoted code? <select name="troom" multiple class="form-control" required> <option selected="true" disabled="disabled">--Select a room--</option> $records =mysqli_query($con, "select * from room where TRoom not in (select TRoom from roombook where cin <= '$checkout' and cout >= '$checkin');"); while ($data = mysqli_fetch_array($records)) { echo "<option value='" . $data['TRoom'] . "'>" . $data['TRoom'] . "</option>"; //displaying data in option menu } } ?> </select> -
multiple selection in an option list
stevochegeoj replied to stevochegeoj's topic in PHP Coding Help
i don't why its returning an empty list. or how will it display? i am learning php