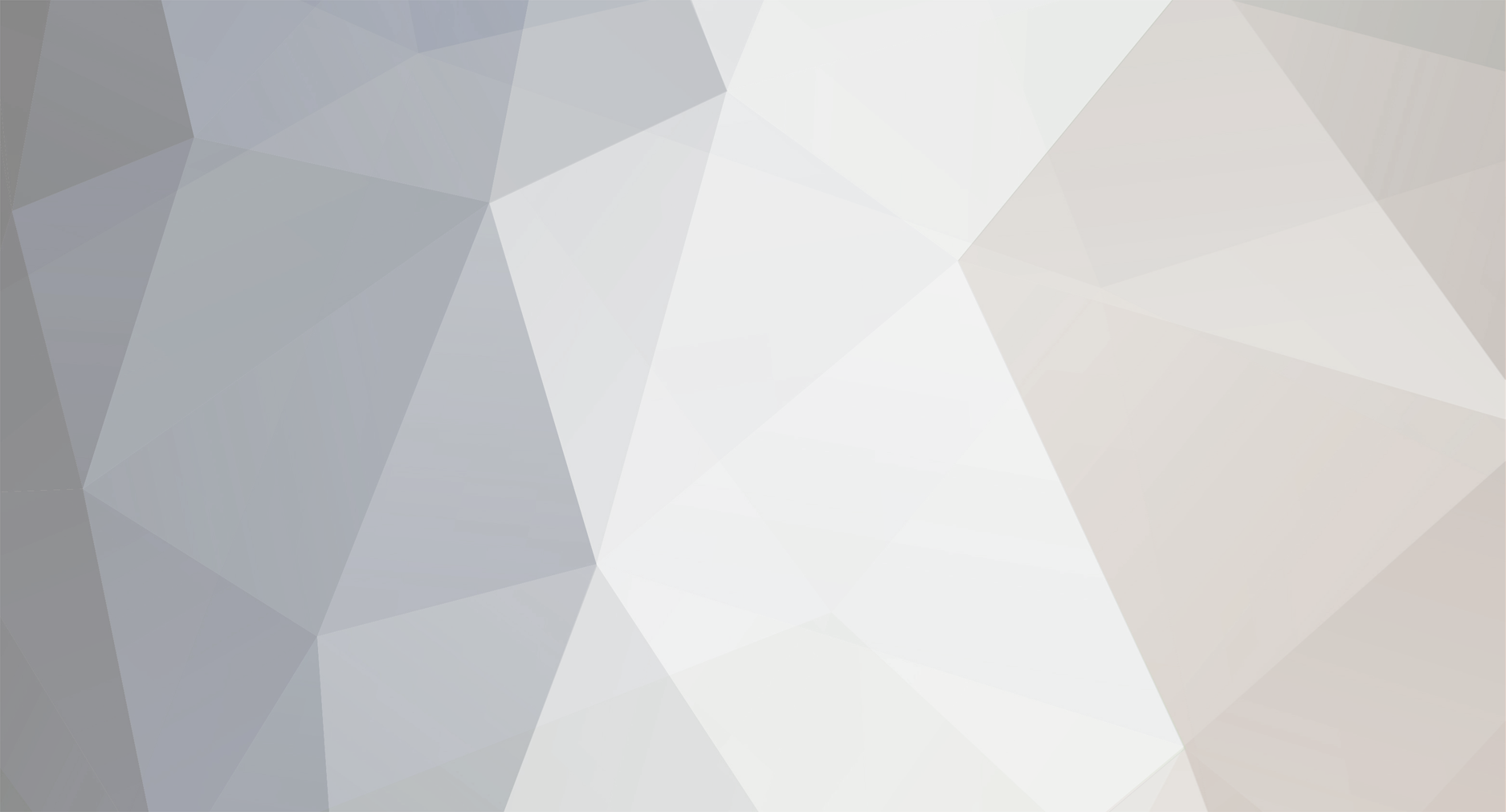
Heretic86
-
Posts
45 -
Joined
-
Last visited
Posts posted by Heretic86
-
-
I am playing around with the Web Audio API now.
I set up a test application to play back an audio file. One of my desired options (at least currently) is to shift the pitch. An easy way to do this is to adjust the playbackRate property. So, it seems to work fine in Chrome and Firefox. Great, lets make it awesomer! Is that a word? I call to preservePitch elsewhere in the code.
I threw in the following code for a Canvas Spectrum Analyzer, which works great:
function graphAnimator(){ requestAnimationFrame(graphAnimator); let fbc_array = new Uint8Array(analyzer.frequencyBinCount); analyzer.getByteFrequencyData(fbc_array); ctx.clearRect(0, 0, graph.width, graph.height); ctx.fillStyle = "#00FFAA"; for (let i = 0; i < 100; i++){ let x = i * 3; let h = -(fbc_array[i * 8] / 12); ctx.fillRect(x, 20, 2, h); } } function setupGraph(){ graph.width = 222; graph.height = 20; ctx = graph.getContext('2d'); aCtx = new window.AudioContext(); analyzer = aCtx.createAnalyser(); audio_source = aCtx.createMediaElementSource(audio); audio_source.connect(analyzer); analyzer.connect(aCtx.destination); graphAnimator(); }
Now, once I do this, and I grab my slider and play with it (ok, that sounded better in my head just now) to adjust the Playback Rate, it works in Chrome but not in Firefox?
Honestly I love the Spectrum Analyzer Canvas more than the rest of what I have so I am open to scrapping that stuff because I would rather be able to adjust the Pitch without having to adjust the Playback Rate. Ive seen a few scripts out there that can do that, but Im pretty well clueless on how to get them set up! Essentially, a Slider (Range) and adjust the Pitch without messing with the Playback Rate. I really want to dump the Playback rate and strictly ONLY adjust a Pitch.
Any suggestions / samples to adjust the Pitch of a MP3 / Ogg / Oga file without needing to revert to adjusting the Playback Rate consistently on every browser? (Well... except Internut Exploder)...
-
Hot Dog! (*bark bark*) It works now!
Thank you very much! I knew it would be one small thing that I had missed! Ive been banging my head against a wall for weeks on this, and you got it fixed!
-
3 hours ago, kicken said:
This may be your issue
MDN: <iframe>: The Inline Frame element
Try adding allow-same-origin to your sandbox attribute.
I will put it back in. I had it in there initially. And this is posted above, but this is the error Im getting now:
Cross-Origin Request Blocked: The Same Origin Policy disallows reading the remote resource at https://www.webucate.me/cors_csp/jsondata.php. (Reason: CORS header ‘Access-Control-Allow-Origin’ does not match ‘https://www.webucate.me’).
This is my Content Security Policy:
<?php header('Cross-Origin-Resource-Policy: same-origin', false); header("Cache-Control: no-store, no-cache, must-revalidate, max-age=0"); header("Cache-Control: post-check=0, pre-check=0", false); header("Pragma: no-cache"); function csp_policy(){ header("Content-Security-Policy: " . "default-src 'none';" . "sandbox allow-scripts;" . "base-uri 'none';" . "img-src 'self';" . "style-src 'self' css/*;" . "navigate-to 'none';" . "form-action 'none';" . "script-src 'report-sample' 'unsafe-inline' 'unsafe-eval' " . "https://" . $_SERVER['SERVER_NAME'] . "/cors_csp/js/video.js " . "https://" . $_SERVER['SERVER_NAME'] . "/cors_csp/jsondata.php " . "data: ;" . "worker-src 'self';" . "media-src https://" . $_SERVER['SERVER_NAME'] . "/cors_csp/playvideo.php;" . "connect-src https://" . $_SERVER['SERVER_NAME'] . ";" . "child-src 'none';" . "report-uri https://" . $_SERVER['SERVER_NAME'] . "/cors_csp/csp_violation/index.php;" . "report-to {\"group\" : \"default\", \"max_age\" : 1800, \"endpoints\" : [{ \"url\" : \"https://" . $_SERVER['SERVER_NAME'] . "/cors_csp/csp_violation/index.php\"}]};" . ""); } ?>
What I would really like is a SIMPLE EXAMPLE that WORKS. So, Sandboxed Iframe, XHR or Fetch, and PHP must to read a cookie. I just cant seem to get a handle on the numerous headers that are all involved, and despite all the googling, I cant find any real working examples intended for demonstration purposes.
Think you could help with that?
-
2 hours ago, kicken said:
I'd suggest you try re-encoding the file, maybe in another format, and see if it helps. Light web searching suggests your issue is just that there is no duration metadata available and also suggests that may be somewhat common for .ogg in particular.
I fingered it out. Wait, that came out wrong. Take Two, Action! I figured it out!
Apparently this works much more way lots gooder-er...
audio.ontimeupdate = function(){ audioController.value = audio.currentTime * (100 / audio.duration); currentTime.innerHTML = Math.floor(audio.currentTime / 60) + ":" + ("0" + Math.floor(audio.currentTime) % 60).slice(-2); totalTime.innerHTML = Math.floor(audio.duration / 60) + ":" + ("0" + Math.ceil(audio.duration) % 60).slice(-2); }
-
I tried loadedmetadata too but not really getting very far. Format doesnt matter to me much, so I could care less if its an MP3, OGG, or a freakin 8 Track Tape, but I dont think the metadata on 8 Track Tapes is easily readable by Javascript.
*idea* If I use a constant bit rate, could I use a Loaded and Total property?
-
5 minutes ago, kicken said:
You don't await anything, you use the durationchange event.
audio.addEventListener('durationchange', function(){ console.log('Duration changed'); setDuration(audio.duration); });
It seems like that would work but it does not work as expected
Console log.
console.log("Duration changed " + audio.duration); Duration changed 11.174603 Duration changed 21.363809 Duration changed 31.107482 Duration changed 41.431655 Duration changed 51.494603 Duration changed 61.37034 Duration changed 65.159546
Perhaps I need to look at another property? What I am looking for is the total duration of an .ogg file, not how much can currently play. Is there a better way to do this?
-
Ok, let me try to explain my goal again.
I want users to upload their own scripts that they can run in an iframe.
Since that is pretty dangerous to other users, I think the best thing to do is sandbox scripts in an iframe. Perhaps I am wrong. And I can not simply say "never let users run their own scripts" because it directly contradicts my goals. Running user scripts safely is the MAIN GOAL.
I think it is a good idea to also use CORS so that those scripts do not try to make calls to anything EXCEPT one specific location on my server where their scripts are loaded from. This includes Inline Scripts.
To load those scripts, I need to be able to send an Ajax / Fetch request to that specific page to load their scripts, which requires a cookie to access which scripts are theirs, and ultimately edit and save those scripts.
Since other users will run those scripts, the Cookie has to be HTTPONLY so one user can not read another users cookies.
But when I send the Ajax / Fetch request to get their data, I cant read their cookie! I think it is something either in CORS or in the way that I making my javascript requests.
Is this a better explanation?
-
How would I write an async function that checks the value of an Audio Object has a duration that is not Infinity?
async () => { let duration = await whatGoesHere?(); setDuration(duration); }
Obviously the above code wont work. Im getting an Audio object back, but when I first get it, the audio.duration is Infinity and comes down later with metadata which also appears unreliable. So how can I use an async function without writing 300 lines of .then setTimeout .catch and do this rather simply?
-
I am close to giving up on this since I feel like no one has even looked at the code... I just dont get why I cant send the cookie even though I am sending what I think is all the proper credentials with the request...
const loadLocalXMLCookie = async function(data = { action : 'getDisplayName' } ) { let url = 'jsondata.php'; const response = await fetch(url, { method: 'POST', mode: 'cors', cache: 'no-cache', credentials: 'same-origin', headers: { 'Content-Type': 'application/json' }, redirect: 'follow', referrerPolicy: 'same-origin', body: JSON.stringify(data) })
-
2 hours ago, requinix said:
I ask because various resources I can find all say that Origin: null means the AJAX request is coming from a file:// location.
I saw the same thing, but it is not. Its hosted on a web server. Granted it is a Localhost server, but it still uses an IP, hell, it even has to route through my VPN IP to get back to itself. I have even gone so far as to set up another Domain Name (hosts file) and make my requests that way so the Iframe part of the page is coming from a different domain and it still doesnt work. I just dont have any idea where I am doing it wrong!
-
11 hours ago, requinix said:
I'll ask once again because I'm pretty sure the correct answer is not what you've been saying:
Is the Javascript code running from a document context of www.webucate.me or something else? Are you perhaps running it as a local file?
Nope. I am sure.
-
1 hour ago, requinix said:
iframe or not, if your code is running on the same domain you're trying to send a request to then you don't "need" CORS, and the default behavior of browsers and servers should be fine...
The same request and response you posted. That's the OPTIONS and, presumably, its reply, right? Because they say that the allowed origin is the exact same thing that the error message is complaining is not present.
What are the headers from a failed AJAX request and response?Should be same as above:
Console (Firefox):
Access to fetch at 'https://www.webucate.me/cors_csp/jsondata.php' from origin 'null' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: The 'Access-Control-Allow-Origin' header has a value 'https://www.webucate.me' that is not equal to the supplied origin. Have the server send the header with a valid value, or, if an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled. iframe.php:59 POST https://www.webucate.me/cors_csp/jsondata.php net::ERR_FAILED
Network Request Headers:
OPTIONS /cors_csp/jsondata.php HTTP/1.1 Host: www.webucate.me User-Agent: Mozilla/5.0 (Windows NT 6.1; Win64; x64; rv:87.0) Gecko/20100101 Firefox/87.0 Accept: */* Accept-Language: en-US,en;q=0.5 Accept-Encoding: gzip, deflate, br Access-Control-Request-Method: POST Access-Control-Request-Headers: content-type Origin: null DNT: 1 Connection: keep-alive Pragma: no-cache Cache-Control: no-cache
Response Headers:
HTTP/1.1 200 OK Date: Thu, 08 Apr 2021 02:03:52 GMT Server: Apache/2.4.41 (Win64) OpenSSL/1.1.1c PHP/7.4.11 X-Powered-By: PHP/7.4.11 Access-Control-Allow-Credentials: true Access-Control-Allow-Headers: Content-Type, Origin Access-Control-Allow-Methods: Content-Type, Access-Control-Allow-Headers, Access-Control-Allow-Origin, Authorization, Allow, POST, OPTIONS Access-Control-Allow-Origin: https://www.webucate.me Access-Control-Max-Age: 1 Set-Cookie: TestCookie=123456; expires=Fri, 09-Apr-2021 02:03:52 GMT; Max-Age=86400; path=/; domain=www.webucate.me; secure; HttpOnly; SameSite=Strict Content-Length: 40 Keep-Alive: timeout=5, max=100 Connection: Keep-Alive Content-Type: application/json; charset=utf-8
And finally, PHP source (simple test):
<?php header("Content-Type: application/json; charset=utf-8"); header('Cross-Origin-Resource-Policy: same-site', false); header('Access-Control-Allow-Credentials: true', false); header('Access-Control-Allow-Headers: Content-Type, Origin', false); header('Access-Control-Allow-Methods: Content-Type, Access-Control-Allow-Headers, Access-Control-Allow-Origin, Authorization, Allow, POST, OPTIONS', false); header('Access-Control-Allow-Origin: https://www.webucate.me', false); header('Access-Control-Max-Age: 1', false); header('X-Frame-Options: SAMEORIGIN' ); $cookie = (isset($_COOKIE['TestCookie'])) ? $_COOKIE['TestCookie'] : 'NoCookies'; $time = time(); $msg = array("time" => $time, "cookie" => $cookie); echo json_encode($msg); setcookie('TestCookie','123456', [ 'expires' => time() + 86400, 'path' => '/', 'domain' => 'www.webucate.me', 'secure' => true, 'httponly' => true, 'samesite' => 'Strict', ]); ?>
I know the first thing you will look at, Allow Origin header. But when I try to disable that, this is what comes up in the console:
Cross-Origin Request Blocked: The Same Origin Policy disallows reading the remote resource at https://www.webucate.me/cors_csp/jsondata.php. (Reason: CORS header ‘Access-Control-Allow-Origin’ missing).
So its like it requires it but I cant implement it. If you want, all the source code is in the zip file in the first post...
Thank you, by the way...
-
1 hour ago, requinix said:
Now I'm lost because I thought this was a CORS question.
The Javascript code that is running and sending AJAX requests. Is it doing so from a document context of www.webucate.me or some other domain?
That is the intent. Its Iframed so the content can be posted on other websites. Iframe also allows Sandboxing the code, which if it comes from another domain, should be restricted access to the rest of the window and document content. And visa versa. Iframe keeps it as just a Container to prevent malicious code from compromising other sites too. It should prevent popups, accessing the rest of the document element, keystrokes when not focused, etc. The cookie is needed for connecting to the Users account to "like" or "share" or whatever.
So yes. I know I set something up very wrong. I am not sure if it needs to be treated as samesite or none (which I have experimented with) but it doesnt seem to matter what I do, I dont see the Origin header being sent, and I am not sure why I cant get it to send. Even on Kubuntu Firefox running in VMware so totally different browser and machine.
-
2 hours ago, requinix said:
You can't set the Origin, that's protected, so if it's sending "null" then that probably means you're not running from a suitable location.
That aside, the cookie is SameSite=Strict, so if you're not running from www.webucate.me then you can't use it.
Even if the Iframe source is the same?
-
Request Headers:
OPTIONS /cors_csp/jsondata.php HTTP/1.1 Host: www.webucate.me User-Agent: Mozilla/5.0 (Windows NT 6.1; Win64; x64; rv:87.0) Gecko/20100101 Firefox/87.0 Accept: */* Accept-Language: en-US,en;q=0.5 Accept-Encoding: gzip, deflate, br Access-Control-Request-Method: POST Access-Control-Request-Headers: content-type Origin: null DNT: 1 Connection: keep-alive Pragma: no-cache Cache-Control: no-cache
Response Headers:
HTTP/1.1 200 OK Date: Wed, 07 Apr 2021 08:44:44 GMT Server: Apache/2.4.41 (Win64) OpenSSL/1.1.1c PHP/7.4.11 X-Powered-By: PHP/7.4.11 Access-Control-Allow-Credentials: true Access-Control-Allow-Headers: Content-Type, Origin Access-Control-Allow-Methods: Content-Type, Access-Control-Allow-Headers, Access-Control-Allow-Origin, Authorization, Allow, POST, OPTIONS Access-Control-Allow-Origin: https://www.webucate.me Access-Control-Max-Age: 1 Set-Cookie: TestCookie=123456; expires=Thu, 08-Apr-2021 08:44:44 GMT; Max-Age=86400; path=/; domain=www.webucate.me; secure; HttpOnly; SameSite=Strict Content-Length: 40 Keep-Alive: timeout=5, max=99 Connection: Keep-Alive Content-Type: application/json; charset=utf-8
Network Tab: Status - Not Allowed
CORS Allow Origin Not Matching Origin
Console.log says this:
Cross-Origin Request Blocked: The Same Origin Policy disallows reading the remote resource at https://www.webucate.me/cors_csp/jsondata.php. (Reason: CORS header ‘Access-Control-Allow-Origin’ does not match ‘https://www.webucate.me’). Cross-Origin Request Blocked: The Same Origin Policy disallows reading the remote resource at https://www.webucate.me/cors_csp/jsondata.php. (Reason: CORS request did not succeed).
---
The origin isnt being sent? How do I set this up to send the Origin?
Since I have to now guess, can I blame it on COVID?
-
It appears that MySql does not play well with numbered indexes. So... moving on...
If one field had an array of objects, such as a list of songs or something, how would we extract all names from one result?
For example, the database result for just "pets" could be something like this string:
[{"id" : "1", "name": "fido", "age":"5"}, {"id" : "2", "name": "rover", "age":"3"},{"id" : "3", "name": "woofie", "age":"1"}]
Then from that list, get just the Name property from each member of the array? Admittedly, this may not be the best approach, its just me learning more about what the potential of using JSON in MySQL offers. Theres just gonna be a LOT of objects and I was hoping to keep things in one table cell that held a lot of data...
-
26 minutes ago, requinix said:
The whole point of an HttpOnly-flagged cookie is that you cannot read or write to it in code. It's right there in the name.
Well, according to the link to the following article, the "withCredentials" flag (XMLHttpRequest, credentials: 'include' in fetch()), you CAN have a cookie set with HTTPONLY and STILL send that cookie without allowing script access. It is an Exception to the HTTPONLY cookie can not be read by scripts rule.
https://medium.com/@_graphx/if-httponly-you-could-still-csrf-of-cors-you-can-5d7ee2c7443
QuoteEnter XMLHttpRequest
XMLHttpRequest is a wonderful function of JavaScript that allows developers to interact with the server and allow for dynamic updates of a page without having to refresh the page. It makes for clean, flashy web apps. Below is the malicious website code with part of the JavaScript used to execute the attack:
However, since it’s JavaScript, we should not be allowed via client-side script to access the cookie, according to the OWASP article on the subject. Normally this would be the case, but the XMLHttpRequest has a property called withCredentials. According to the article, the withCredentials property “Is a boolean that indicates whether or not cross-site Access-Control requests should be made using credentials such as cookies or authorization headers”. So according to everything we have thus far, we can use session cookies programatically unless the HttpOnly flag is set, right?
Wrong. There appears to be an exception to the client-side script access prohibition for XMLHttpRequest that will still allow you to access the session cookie even with the HttpOnly flag set as long as the withCredentials property is set to true. This means that we can still execute CSRF using a victims session cookie that is properly secured with the HttpOnly flag.
So it isnt the HTTPONLY flag that is causing my issue. I am just not familiar enough with CORS setups.
Anyway, as far as I can tell, it looks like there is an "Origin" header that is supposed to be sent. Obviously I havent set up my request to include the Origin header, and I think that *might* be why this isnt working?
-
What I would like to do is to is to have a User Forum that allows Users to upload their own Javascript files that will run on other Users computers.
I am aware of how dangerous that is. It can be done IF done properly. The User scripts are intended to be run ONLY inside a Sandboxed Iframe with very restrictive CORS policies in place. I have already done this. CORS and Sandboxing allows preventing all XMLHttpRequests / Fetch requests to external sites so there is much less chance of a Users computer being compromised or trying to download malicious packages. I take that back. There is ONE place they should be allowed to retrieve data from, which is a simple AJAX request to my server, which will be one page that serves up their scripts.
I have run into an issue however. I need to be able to pass an HTTPONLY cookie through the AJAX request so that the User is able to use the Sandbox for its intended purposes. On my PHP side where the AJAX request is sent, I am not getting any cookie data sent from the Browser. Im not sure if I should turn off HTTPONLY on it for the CORS request tho.
I am not sure where to even begin troubleshooting this. Could one of you very experienced people take a peek at my code and offer any advice as to why I am not able to read cookies on my XML Requests?
---
Page where I am having trouble working within CORS Policies and still being able to read Cookies...
https://www.webucate.me/cors_csp/
Data URL that I am trying to use AJAX to get data from (test json object, time + cookie with a value of 123456):
https://www.webucate.me/cors_csp/jsondata.php
Source Code:
https://www.webucate.me/cors_csp/cors.zip
-
Hello all, this is my introduction on this forum. Hopefully I can get some questions answered and answer a few that I have answers to.
So, first question, what subforum would be best suited for a CORS related question? I know to not post that question here as this is an Introduction forum.
-
Hi!
I want to have an Indexed Array with Indeces I define.
In PHP I can do it like this:
$my_array = [1=> "foo", 3=> "bar", 11 => "baz"];
I've tried this but MySQL JSON field type does not like it...
[ 1 : {"name" : "Foo"}, 3 : {"name" : "bar"}]
The variations I have tried end up making the Index an Object Property not an Array Index. How do I set the Index in a JSON Field Type in a MySQL database?
Web Audio API Pitch - Firefox vs Chrome
in Javascript Help
Posted
How do I implement both Analyzer and set playbackRate in Firefox which works in Chrome?