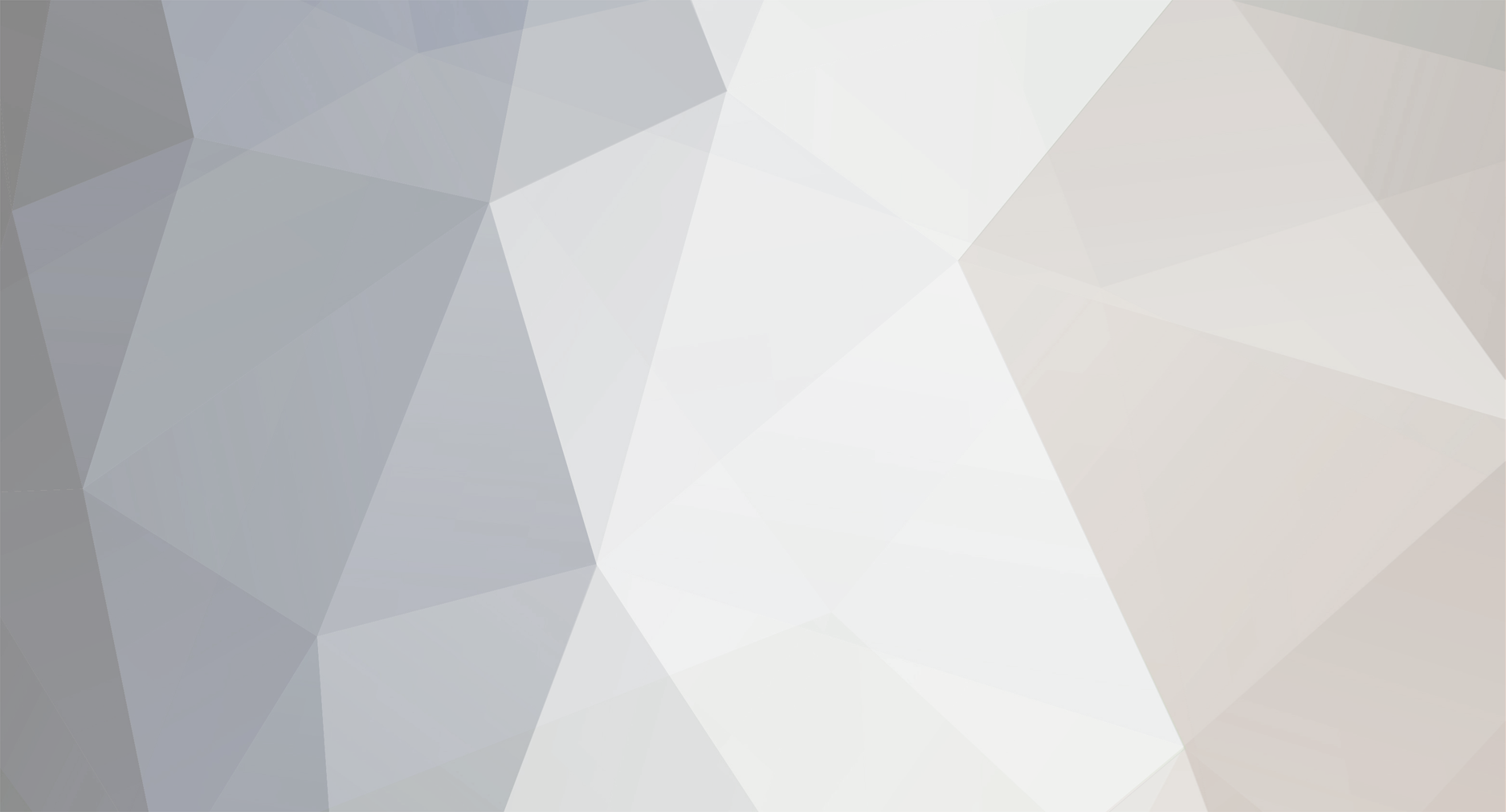
ChenXiu
Members-
Posts
177 -
Joined
-
Last visited
Everything posted by ChenXiu
-
my preg_match_all works perfectly. I just can't figure out how to loop through the "$matches" array it creates. preg_match_all( $pattern , $string , $matches); The code print_r($matches) yields the following super simple array. ( [0] => Array ( [0] => 123456wordsandwordsandmorestuff$40.97 [1] => 987654wordsandmorewords$26.79 ) [1] => Array ( [0] => 123456 <----------this goes with the [0] below [1] => 987654 <----------this goes with the [1] below ) [2] => Array ( [0] => $40.97 <-----------this goes with the [0] above [1] => $26.79 <-----------this goes with the [1] above ) ) The problem is I can't figure out how to do the foreach() loop to get this: SKU number 123456 costs $40.97 SKU number 978654 costs $26.79 I know the answer will be embarrasingly simple..... but I've tried at least 200 different foreach variations and I am close to giving up. Thanks.
-
May I please ask one final question on this topic? Question: Does this line of code "global $ch;" cause cURL to be inited each time I call this function later in my script? And, if so, can my code be modified so that cURL is only inited once at the beginning of the script? (unneccessarily initing cURL over and over when it only needs to be inited once wastes script execution time): $ch = curl_init(); // I want to only init cURL just once function my_curl_function($url_with_SKUs) { global $ch; curl_setopt($ch, CURLOPT_URL,$url_with_SKUs); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); $result = curl_exec($ch); $decoded = json_decode($result,true); foreach($decoded as $my_results) { // do something } } // some php code here my_curl_function($url); // some php code here my_curl_function($url); // some php code here my_curl_function($url);
-
Uh oh.... fetchALL didn't work. "error stack trace blah blah blah" @Barand, yes PDO. I don't use PDO. I'm still stuck in PHP circa 1999. (But at least I learned *2* things today 😀)
-
@mac_gyver & @Barand: THANK YOU! 😀 Just in this one thread I've learned 3 new things! • array_chunk (very cool!) • using "implode" instead of nested foreach loop! • mySQL "fetchALL" (I didn't know that existed -- I only knew about fetch_obj, fetch_assoc, and fetch_array) Thank you again!!
-
In the example in my previous post, "$sku" was a normal array of SKU numbers. What would I do if the SKU numbers came from a mySQL database? Given the following mySQL query: $result = $db->query("select sku_numbers from table"); Should I put the mySQL result into an array, and then use array_chunk, like this? $result = $db->query("select sku_numbers from table"); while ($each = $result->fetch_assoc()) { $sku[] = $each["sku_numbers"]; } foreach(array_chunk( $sku, 5) as $chunk { // do stuff Or is there a better ("more efficient") mySQL style code to use? Thank you!
-
Ohhhh okay I see what you meant. Instead of nested foreach loop, the "implode" function does the same thing, but much more efficient! THANK YOU!
-
Thank you. When using array_chunk, is this nested "foreach" loop the BEST way to return values, for the API/cURL script I had described: $myArray = array( '1' , '2' , '3' , '4' , '5' , '6' , '7' , '8' , '9' ); $chunked = array_chunk( $myArray , 5 ); foreach($chunked as $key => $value) { foreach ($value as $key1 => $value1) { // $value1 is the result I would want } }
-
An API allows me to access information on an unlimited number of SKUs that I submit via cURL. However, their "multiple-lookup" URL limits the SKU numbers to maximum of 5 at a time. Question: Given a list of 100 SKU numbers with a maximum of 5 at a time, what is the best way to loop through them? Their API format is: https://example.com?key=secret&SKU=1234&SKU=4444&SKU=555&SKU=0101&SKU=3333 My PHP script makes the cURL calls, and place the results into a $_SESSION. Here is an example of my code using 7 SKU numbers: $ch = curl_init(); my_curl_function($url_with_SKUs) { curl_setopt($ch, CURLOPT_URL,$url_with_SKUs); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); $result = curl_exec($ch) $_SESSION["curlResults"][] = $result // I put the results into Session. } $sku = array( '1234', '5678', '4444', '2222', '9393', '1111', '8689' ); $url = 'https://example.com?key=secret'; $append = ''; for($i=0;$i<count($sku);$i++){ $append .= '&SKU='.$sku[$i]; if(($i+1) % 5 == 0) { $url .= $append; $append = ''; my_curl_function($url); } } ...but my code has flaws: • when I use modulus, there are still the final left-over SKU numbers that don't get put in the URL • I'm not even sure I should be using a function at all • Should I even be doing a "for" loop? Or would a "while" loop or a "do" loop be better? Even though this seems to be SUPER basic novice PHP stuff, I can't seem to put this all together in my brain. I've spent weeks on this... Your suggestions will be most welcome.
-
Arrays use brackets. What is the syntax if I wanted a bracketed variable inside brackets? For example "https://www.example.com/index.php?animal=horse" has this $_GET variable: $_GET["animal"] Security vulnerabilities notwithstanding, the following syntax does not work: echo $_SESSION[$_GET["animal"]]; I assume it does not work because it's a bracketed variable inside brackets. What syntax makes this work? Thank you!
-
Barand, thank you, but in your answer you used the phrase, "...unless you tell it what could potentially be there." I was hoping that the query itself tells PHP what could potentially be there. My query here demonstrates that "rose" and "carnation" could potentially be there: SELECT * FROM prettyflowers WHERE flower = 'rose' UNION SELECT * from prettyflowers WHERE flower = 'carnation' ...etc., etc. That's why I thought PHP could somehow keep track of what's not in the table ( "carnation" in this example). Currently my only solution is to do TWO separate queries: • "check and see if it is there" query, and • the main query Like this: $not_in_table = array(); // CAPTURES WHAT DOESN'T EXIST IN TABLE foreach($flowers as $foo) { //NOTE: "$flowers" would be list of flowers input by visitor if ($result = $db->query("select exists(select * from prettyflowers where flower = '$foo') as c")->fetch_assoc()) { if(!$result['c']) { $not_in_table[] = $foo; } } } // NOW I RUN MY MAIN QUERY // (using "UNION SELECT" because customer will input 100 flowers, and we want to keep the same order as input by customer) $main_query = "select * from prettyflowers where flower = 'rose' UNION SELECT * FROM prettyflowers WHERE flower = 'carnation' "; $flowerList = $db->query("$main_query"); while ($flower = $flowerList->fetch_assoc()) { echo $flower["color"]; echo '<BR>'; } // AND THEN I CAN LET VISITOR KNOW WHICH ONES WEREN'T IN TABLE echo 'Sorry, these were not in database<BR>'; foreach ($not_in_table as $absent) { echo "$absent <BR>"; }
-
The code I provided was just a silly example to articulate my question: How to capture list of what keys are not in a given table. If a given table has the keys 1, 2, 3, 4, 5 in it, and my query is select blah from table where key = 2, 3, 8, and 9 Obviously "8" and "9" are not in the table, and that's what I want captured by PHP. PHP would give me this result: $not_in_table = array( '8', '9' ); So while I'm doing my queries and retrieving valid results, etc., I am also wanting PHP to capture/keep-track-of keys not in the table.
-
Table name: "prettyflowers" +--------+--------+ | flower | color | +--------+--------+ | daisy | yellow | | rose | red | | orchid | purple | | lily | pink | +--------+--------+ Query: SELECT color FROM prettyflowers WHERE flower = 'rose' UNION SELECT color from prettyflowers WHERE flower = 'carnation' UNION SELECT color from prettyflowers WHERE flower = 'orchid' UNION SELECT color from prettyflowers WHERE flower = 'tulip'; Question: My query requests data on 4 flowers, but the table only has data on 2 of the flowers. (Notice that "carnation" and "tulip" are absent from the table.) When running a Union Select query like the one above, is there a way for PHP/mySQL to return a list of what's NOT present in the table? Here's a non-working "pseudocode" to illustrate what I'm looking for instead of: while ($results = $query->fetch_assoc()) { ...... maybe there's something simple like while ($results != $query->fetch_assoc()) { $array_of_things_not_in_table[ ]= Thanks!
-
I almost took offense, thinking you were singling me out... But I see you're this way with everyone https://forums.phpfreaks.com/profile/109381-ginerjm/content/
-
Correct, one session at a time. By "One big session," I mean place the session variables under one named variable. Analogy: imagine a manila folder containing photos of fruits. Option 1.) Manila folder is unlabeled: $_SESSION["apple"] = 'red'; $_SESSION["grape"] = 'green'; $_SESSION["lemon"] = 'yellow'; Option 2.) Manila folder is labeled "fruit": $_SESSION["fruit"]["apple"] = 'red'; $_SESSION["fruit"]["grape"] = 'green'; $_SESSION["fruit"]["lemon"] = 'yellow'; I'm trying to learn PHP best practices. Which is "best practice?" I know, because my PHP code generates the $ordernumber value, and immediately places it in session before serving to browser. Thank you.
-
Silly? Really? So if I have <input type="hidden" name="price value="50.00">, you think it's silly to set $_SESSION["price"] = '50.00'; and on destination page check that $_SESSION["price"] == $_POST["price"] I'm wondering why "the whole thing is silly." Thank you.
-
To prevent misuse on some $_GET and $_POST pages, I use sessions to make sure Get, Post, and other variables aren't monkeyed with. Example: if($_SESSION["ordernumber"] !== $_POST["ordernumber"]) { exit(header("location:goAwayHacker.com")); } When I reviewed my final code, I've used lots of individual sessions to do the checks-and-balances: e.g. $_SESSION["ordernumber"], $_SESSION["userid"], $_SESSION["sku_numbers"], $_SESSION["date"], $_SESSION["this"], $_SESSION["that"], $_SESSION["etc"] Question: Would having just ONE big session as an array containing all the aforementioned sessions be equally secure? Does having one big session as an array make PHP work harder and slow things down? $_SESSION["security"] = array( 'ordernumber' => '1234', 'userid' => 'MyDogRover', etc. etc. etc..... So, for example, instead of if($_SESSION["ordernumber"] !== $_POST["ordernumber"]) { exit(header("location:goAwayHacker.com")); } ... I would do: if($_SESSION["security"]["ordernumber"] !== $_POST["ordernumber"]) { exit(header("location:goAwayHacker.com")); } Thank you.
-
Dear Moderator, please delete this thread. I nothing less than bungled my question. I have never bungled a question before. This one is...... bungled. I meant "padding" when typing the word "margin." I did not mean "margin." Thank you.
-
Given the following css rule: body { margin: 0 100px; } I then want the margin to start shrinking at a viewport width of 600px: @media screen and (max-width: 600px) { body { margin: 0 8.333vw; } } The Challenge: The aforementioned css rule causes the margin to become zero only when the viewport width reaches zero... Is there a way to cause the margin to smoothly become zero when the viewport reaches, say, 400px? It appears the only way to do this is by using chain of several diminishing @media queries. (And, using "transition: ease all .2s" to liquify the transition points.) Is there a fancy css rule using calc (along with exponents perhaps) that can accomplish this? (I've tried doing things "viewport squared," but it appears you cannot multiply viewports times itself.) Any ideas would be appreciated.
-
Thank you! I also enjoyed reading the mathematics behind specificity. Cool!
-
Given the following html table, are both css rules 100% identical in effect? Why? Or why not? <table class="classname"><tr><td>Hello World</td></tr></table> table.classname td { ......... } - versus - .classname td { ......... } Thank you.
-
I just now tried your code, and it works! For my own learning purposes, may I please complicate things a bit? 😀 Let's say I have 2 tables: Table1 Company | Description | sku_number | Price ACME | Recliner | sku_111111 | $10.00 ACME | Table | sku_22222 | $7.00 Table2 Company | Description | sku_number | Price ZUNFXZ | Lounger | sku_111111 | $50.00 ZUNFXZ | Bench | sku_22222 | $3.00 I like how the following code *always* uses the descriptions from Table1, even when price is higher in Table2 create table highestTable as SELECT * , MAX(Price) FROM ( SELECT * FROM table1 UNION ALL SELECT * FROM table2) both_tables GROUP BY sku_number; BUT... I want the resulting MAX(Price) table to reflect the value of the "Company" column where the MAX(Price) came from. Like this: MaxPriceTable Company | Description | SKU_number | Price ZUNFXZ | Recliner | sku_111111 | $50.00 <--- uses description from Table1, and shows Company that MAX(Price) came from ACME | Table | sku_22222 | $3.00 <--- uses description from Table1, and shows Company that MAX(Price) came from Since the above code already does everything I want, is there a modification to the code that yields the Company that the MAX(Price) came from, while leaving all other columns untouched?
-
Both tables are supplied to me every morning, exact same format, and primary key is sku_number. I create my own table based on the best price between the 2 tables. However, even though sku numbers match, there are always slight color variations. Example: table1 | sku12345 | beige | $6.00 | | sku99999 | pink | $44.00 | table2 | sku12345 | tan | $15.00 | | sku99999 | red | $30.00 | | sku50505 | orange | $7.00 | Currently my max price code gives me this: | sku12345 | beige | $15.00 | <--- but should be "tan" because max price is from table2 | sku99999 | pink | $44.00 | | sku50505 | orange | $7.00 | ( OOPS.... I see you posted code while I was typing my answer 😀 ) Should I try your code now? Or might my answer changed your response?
-
2 mySQL tables have the same 3 columns: "Color" "Price" and "sku_number." To create a "maximum price" table from those tables, this works great: create table highestTable as SELECT * , MAX(Price) FROM ( SELECT * FROM table1 UNION ALL SELECT * FROM table2) both_tables GROUP BY sku_number; However, the newly created MAX table only has colors from table1. How can I make the newly created MAX table have the color from the table with the highest Price?
-
convert GET to POST (and AVOID $_SESSIONS !!! )
ChenXiu replied to ChenXiu's topic in PHP Coding Help
I am not the owner of the "links" you want me to onClick. [Admins: if you want to delete this entire thread, that might be a good idea -- it is obvious I did a terrible job of describing the scenario.] There are several big-company 3rd party "Top 10 places to Sell Widgets" out there that I have no control over. Like this big company fictitious website: "Top10widgets.com: GET BEST PRICES FOR YOUR WIDGETS" Visitor has widget they want to sell. They go to Top10widgets.com and input SKU number "ABCXYZ" onto their page, and sees a list like this: ChenXiuThisIsMe.com: $6.00 <a href="https://myCompany.com/referal.php?referer=top10widgets&SKU=ABCXYZ">SELL HERE</a> Somecompany1.com $5.00 <a href="https://myCompany.com/referal.php?referer=top10widgets&SKU=ABCXYZ">SELL HERE</a> Somecompany2.com $4.00 <a href="https://myCompany.com/referal.php?referer=top10widgets&SKU=ABCXYZ">SELL HERE</a> Somecompany3.com $3.00 <a href="https://myCompany.com/referal.php?referer=top10widgets&SKU=ABCXYZ">SELL HERE</a> Somecompany4.com $3.00 <a href="https://myCompany.com/referal.php?referer=top10widgets&SKU=ABCXYZ">SELL HERE</a> Visitor sees my company at the top of that list, offering the best price, and chooses my company by clicking that "SELL HERE" link. I have no control over the Top10widgets.com whether they use onclick, target_blank, etc. What they have is what they have. It's a referral link that sends visitor to my website. If visitor is already on my website with $_POST values of widgets they've already priced, then midstream visitor decides to go to Top10widgets.Com and clicks their "SELL HERE" link to bring them back to my website, all existing $_POST data on my website gets lost because the "SELL HERE" link on Top10widgets.com is a $_GET request. -
convert GET to POST (and AVOID $_SESSIONS !!! )
ChenXiu replied to ChenXiu's topic in PHP Coding Help
I love the idea of Ajax (thank you Barand!). But I should have explained better. The visitor manually prices their widgets on my website to see my offering prices, like this: 1.) Visitor has a box of 5 widgets sitting on their desk. 2.) Visitor manually types in the SKU numbers from those 5 widgets and clicks "Submit" to see my Offering Prices. 3.) Visitor may enter all 5 SKU numbers at once, click "Submit" and see all 5 prices all at once, or, they can enter and submit them one-at-a-time, until all 5 widgets appear with prices. 4.) NORMALLY, the Visitor would then enter their name and address and click "Complete Order" and then a Packing Slip is generated. However, before the visitor "completes their order," they might choose to price one of those widgets on one of those 3rd-Party "Best Price" comparison-style websites that are popularly used for pricing widgets. Those 3rd Party websites have all of us companies listed, with our respective offering prices, and a "Sell Here" button next to each of our company's prices: <a href="https://myWebsite/link.php?Referer=RefererCompany&SKU=XYZ>Sell Here</a> When the visitor clicks that "Sell Here" button on that 3rd party website, that causes the Pricing Page described above to reload with the $_GET request, thus causing all the $_POST data from the previously priced 5 widgets to vanish. (Unless I use Sessions) So although I hope I somehow could use Ajax, I don't see how it would fit in. I hope there is a way to use Ajax -- I'm all ears on any suggestions on this. (And, of course, any alternatives if Ajax is not possible.) p.s. @Gizmola.... you said, "This sounds like a "you" problem." haha -- yes, you caught me there -- okay, so maybe not 1000 lines of code, maybe just 15 lines of code.... but I've always aspired to always use really short "one-liner" code.... so to me, 15 lines of $_SESSION code really does feel like 1000 lines 😀 Also, my website is intentionally designed so visitors never have "register" or "log in." Although having required registration/login would programatically solve lots of issues, my customers demand to not have to "log in" (they consider "not having to register" to be a feature). p.s.3 Oh my goodness... I think this is my longest ever post :-) But I'm thisssss closssseeee [making pinching finger gesture] to being all done 😀😀😀