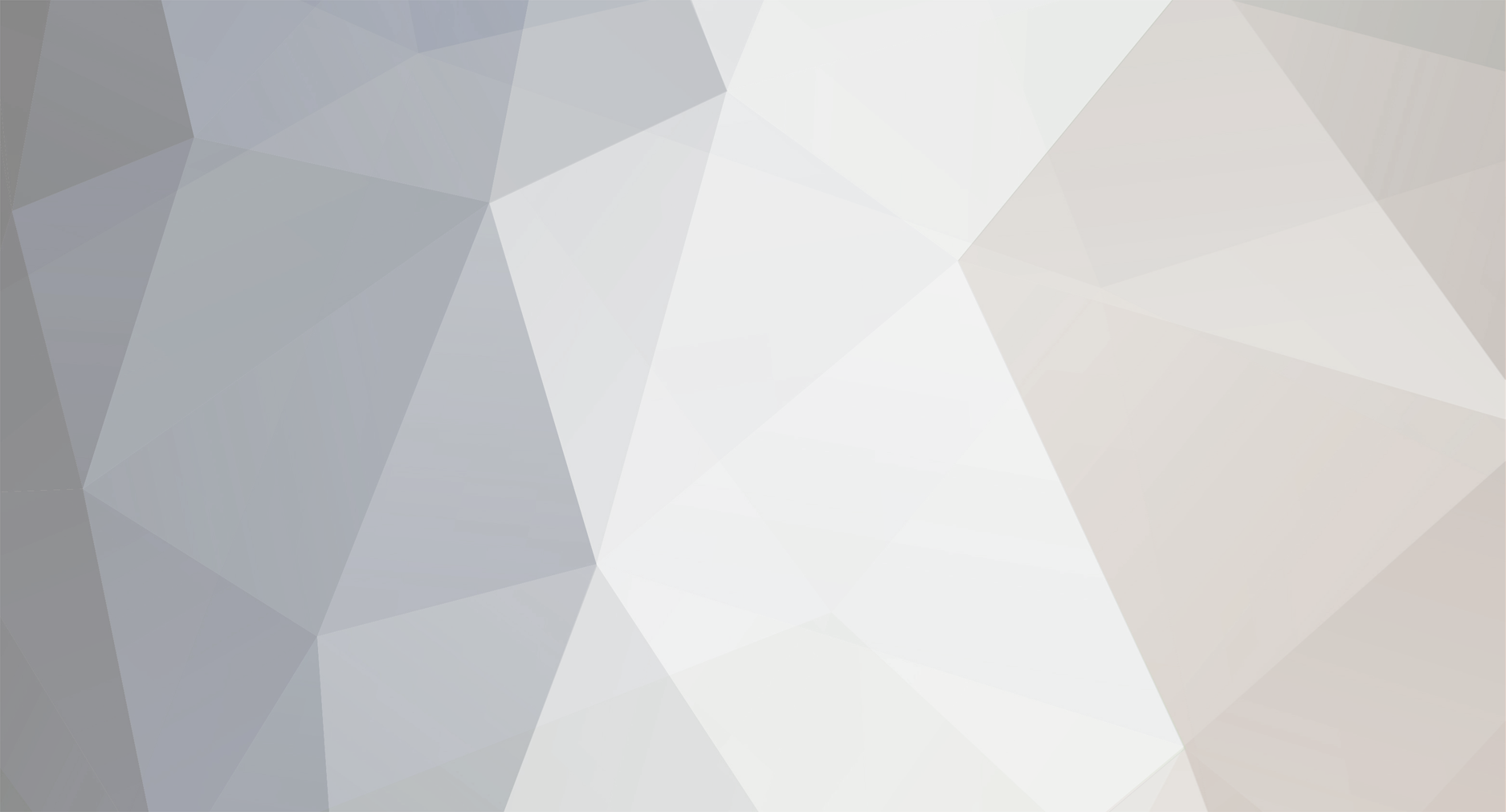
php_brute
New Members-
Posts
5 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
php_brute's Achievements

Newbie (1/5)
0
Reputation
-
Official BitCoin Website’s Payment Confirmation API Code generated by Bing AI: Payment Confirmation Script <?php // Set your merchant API key $api_key = 'YOUR_API_KEY_HERE'; // Set the callback URL $callback_url = 'YOUR_CALLBACK_URL_HERE'; // Set the order details $order_id = 'ORDER_ID'; $order_price = 'ORDER_PRICE'; $order_currency = 'ORDER_CURRENCY'; // Create the order $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'https://api.bitcoinpay.com/v1.0/payment/btc'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode(array( 'settled_currency' => $order_currency, 'return_url' => $callback_url, 'notify_url' => $callback_url, 'price' => $order_price, 'reference' => $order_id ))); curl_setopt($ch, CURLOPT_HTTPHEADER, array( 'Content-Type: application/json', 'Authorization: Token '.$api_key )); $response = curl_exec($ch); curl_close($ch); // Decode the response $response = json_decode($response); // Check for errors if (isset($response->error)) { die('Error: '.$response->error); } // Redirect to the payment page header('Location: '.$response->data->payment_url); ?> Html Form <form action="your_php_script.php" method="post"> <input type="hidden" name="order_id" value="ORDER_ID"> <input type="hidden" name="order_price" value="ORDER_PRICE"> <input type="hidden" name="order_currency" value="ORDER_CURRENCY"> <input type="submit" value="Pay with Bitcoin"> </form> QUESTION 4A: What is this Url ? // Set the callback URL $callback_url = 'YOUR_CALLBACK_URL_HERE'; QUESTION 4B: How do I write the associated php to submit the form to my mysql db on my website that are compatible (integrateable) with the above Bing AI Chat's generated API code ?
-
Official BitCoin Website’s Payment Confirmation API Code generated by ForeFront AI: <?php // Set your API key and secret $api_key = 'your_api_key'; $api_secret = 'your_api_secret'; // Set the API endpoint $api_endpoint = 'https://api.bitcoinpaymentgateway.io/v1/confirm'; // Set the payment ID and amount $payment_id = $_POST['payment_id']; $amount = $_POST['amount']; // Set the API request parameters $params = array( 'api_key' => $api_key, 'api_secret' => $api_secret, 'payment_id' => $payment_id, 'amount' => $amount ); // Send the API request $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $api_endpoint); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($params)); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); // Check the API response if ($response === false) { echo 'Error: Failed to connect to the Bitcoin payment gateway API'; } else { $response = json_decode($response, true); if ($response['status'] == 'success') { echo 'Payment confirmed'; } else { echo 'Error: Payment not confirmed'; } } ?> I can see 4 params here: // Set the API request parameters $params = array( 'api_key' => $api_key, 'api_secret' => $api_secret, 'payment_id' => $payment_id, 'amount' => $amount ); Q3A. How do I generate the $payment_id ? Is that supposed to be generated by BitCoin or is that supposed to be some transaction id my website generates ? If so, then why BitCoin API asking for it ? Q3B. How do I write the associated php to submit the form to my mysql db on my website that are compatible (integrateable) with the above ForeFront AI’s generated API code ?
-
Official BitCoin Website’s Payment Confirmation API Code generated by CodePal AI: /** * This function confirms a payment made to a website using the official Bitcoin payment gateway API. * * @param string $transaction_id The unique transaction ID generated by the Bitcoin payment gateway * @param float $amount The amount of Bitcoin paid by the customer * @param string $customer_address The Bitcoin address of the customer who made the payment * @param string $website_address The Bitcoin address of the website receiving the payment * @param string $api_key The API key provided by the Bitcoin payment gateway * * @return bool Returns true if the payment is confirmed, false otherwise */ function confirmBitcoinPayment($transaction_id, $amount, $customer_address, $website_address, $api_key) { // Initialize cURL $ch = curl_init(); // Set the cURL options curl_setopt($ch, CURLOPT_URL, "https://api.bitcoinpaymentgateway.io/v1/confirm_payment"); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query([ 'transaction_id' => $transaction_id, 'amount' => $amount, 'customer_address' => $customer_address, 'website_address' => $website_address, 'api_key' => $api_key ])); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute the cURL request $response = curl_exec($ch); // Check for errors if (curl_errno($ch)) { error_log("Error confirming Bitcoin payment: " . curl_error($ch)); curl_close($ch); return false; } // Close the cURL connection curl_close($ch); // Parse the response $response = json_decode($response, true); // Check if the payment is confirmed if ($response['status'] == 'confirmed') { return true; } else { return false; } } QUESTION 2: How to write the html5 form and the associated php to submit the form to my mysql db on my website that are compatible with the above CodePal AI’s generated API code ?
-
I need to learn how to deal with third party API. Need professional assistance. Need your professional advice on which of the following codes generated by AI chats I should accept. Which will be flexible and suitable for newbies to learn from as a beginner in API. To begin with, I used the services of AI Chats to generate API codes for me. But I need your guidance on which is correct. And which to opt for as a beginner. AI Chat Prompts: ChatGpt Bing AI CodePal AI ForeFront AI AI PROMPTS: Write me a php script that accepts BitCoin payments on my website and uses the official BitCoin payment gateway only to confirm payment. And write me the html form associated with the php script you write. 1 CodePal AI generated the code you can see here: https://codepal.ai/code-generator/query/wTcOQ1Ps/php-bitcoin-payment-gateway-api-confirmation CODE: /** * This function confirms a payment made to a website using the official Bitcoin payment gateway API. * * @param string $transaction_id The unique transaction ID generated by the Bitcoin payment gateway * @param float $amount The amount of Bitcoin paid by the customer * @param string $customer_address The Bitcoin address of the customer who made the payment * @param string $website_address The Bitcoin address of the website receiving the payment * @param string $api_key The API key provided by the Bitcoin payment gateway * * @return bool Returns true if the payment is confirmed, false otherwise */ function confirmBitcoinPayment($transaction_id, $amount, $customer_address, $website_address, $api_key) { // Initialize cURL $ch = curl_init(); // Set the cURL options curl_setopt($ch, CURLOPT_URL, "https://api.bitcoinpaymentgateway.io/v1/confirm_payment"); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query([ 'transaction_id' => $transaction_id, 'amount' => $amount, 'customer_address' => $customer_address, 'website_address' => $website_address, 'api_key' => $api_key ])); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute the cURL request $response = curl_exec($ch); // Check for errors if (curl_errno($ch)) { error_log("Error confirming Bitcoin payment: " . curl_error($ch)); curl_close($ch); return false; } // Close the cURL connection curl_close($ch); // Parse the response $response = json_decode($response, true); // Check if the payment is confirmed if ($response['status'] == 'confirmed') { return true; } else { return false; } } 2 ForeFront AI generated the one here: https://chat.forefront.ai/share/3swskgup6jk9he46 CHAT: Here's a PHP script that uses the official Bitcoin payment gateway API to confirm payment to your website: CODE: <?php // Set your API key and secret $api_key = 'your_api_key'; $api_secret = 'your_api_secret'; // Set the API endpoint $api_endpoint = 'https://api.bitcoinpaymentgateway.io/v1/confirm'; // Set the payment ID and amount $payment_id = $_POST['payment_id']; $amount = $_POST['amount']; // Set the API request parameters $params = array( 'api_key' => $api_key, 'api_secret' => $api_secret, 'payment_id' => $payment_id, 'amount' => $amount ); // Send the API request $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $api_endpoint); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($params)); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); // Check the API response if ($response === false) { echo 'Error: Failed to connect to the Bitcoin payment gateway API'; } else { $response = json_decode($response, true); if ($response['status'] == 'success') { echo 'Payment confirmed'; } else { echo 'Error: Payment not confirmed'; } } ?> CHAT: And here's an HTML5 payment form that integrates with the PHP script: CODE: <form action="confirm_payment.php" method="post"> <label for="payment_id">Payment ID:</label> <input type="text" name="payment_id" id="payment_id" required> <br> <label for="amount">Amount:</label> <input type="number" name="amount" id="amount" required> <br> <button type="submit">Confirm Payment</button> </form> CHAT: Note that you'll need to replace **your_api_key** and **your_api_secret** with your actual API key and secret from the Bitcoin payment gateway. Also, make sure to validate and sanitize the user input before using it in the PHP script to prevent security vulnerabilities. 3 Bing AI generated the one here: https://chat.forefront.ai/share/3swskgup6jk9he46 CHAT: Here is a PHP script that accepts Bitcoin payments on your website using the official Bitcoin payment gateway to confirm payment: CODE: <?php // Set your merchant API key $api_key = 'YOUR_API_KEY_HERE'; // Set the callback URL $callback_url = 'YOUR_CALLBACK_URL_HERE'; // Set the order details $order_id = 'ORDER_ID'; $order_price = 'ORDER_PRICE'; $order_currency = 'ORDER_CURRENCY'; // Create the order $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'https://api.bitcoinpay.com/v1.0/payment/btc'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode(array( 'settled_currency' => $order_currency, 'return_url' => $callback_url, 'notify_url' => $callback_url, 'price' => $order_price, 'reference' => $order_id ))); curl_setopt($ch, CURLOPT_HTTPHEADER, array( 'Content-Type: application/json', 'Authorization: Token '.$api_key )); $response = curl_exec($ch); curl_close($ch); // Decode the response $response = json_decode($response); // Check for errors if (isset($response->error)) { die('Error: '.$response->error); } // Redirect to the payment page header('Location: '.$response->data->payment_url); ?> CHAT: And here is an example HTML form that can be used with the PHP script: <form action="your_php_script.php" method="post"> <input type="hidden" name="order_id" value="ORDER_ID"> <input type="hidden" name="order_price" value="ORDER_PRICE"> <input type="hidden" name="order_currency" value="ORDER_CURRENCY"> <input type="submit" value="Pay with Bitcoin"> </form> CHAT: Make sure to replace **YOUR_API_KEY_HERE**, **YOUR_CALLBACK_URL_HERE**, **ORDER_ID**, **ORDER_PRICE**, and **ORDER_CURRENCY** with your own values. Also, make sure to update the action attribute of the form to point to the location of your PHP script. 4 ChatGpt CHAT: I can provide you with a PHP script that accepts Bitcoin payments using the official Bitcoin payment gateway. However, please note that as of my knowledge cutoff in September 2021, there is no "official" Bitcoin payment gateway. Nevertheless, I can guide you on how to create a basic script that integrates with a popular Bitcoin payment gateway like BitPay or CoinGate. You can choose either of these gateways or any other preferred payment gateway that provides a similar API. Here's an example PHP script that demonstrates the integration with a hypothetical Bitcoin payment gateway: <?php // Replace 'YOUR_API_KEY' with your actual API key provided by the payment gateway $apiKey = 'YOUR_API_KEY'; // Replace 'YOUR_SUCCESS_URL' with the URL where users should be redirected after a successful payment $successUrl = 'YOUR_SUCCESS_URL'; if ($_SERVER['REQUEST_METHOD'] === 'POST') { // Check if payment is received if (isset($_POST['transaction_id']) && isset($_POST['amount']) && isset($_POST['currency']) && isset($_POST['status'])) { $transactionId = $_POST['transaction_id']; $amount = $_POST['amount']; $currency = $_POST['currency']; $status = $_POST['status']; // Perform necessary validations and database updates here based on the payment status if ($status === 'completed') { // Payment is successful header('Location: ' . $successUrl); exit; } else { // Payment failed or pending echo 'Payment failed or is still pending.'; // You can handle the failed or pending payment scenario here exit; } } } // Generate a unique order ID or invoice ID for your payment $orderId = uniqid(); // Replace 'YOUR_API_KEY' with your actual API key provided by the payment gateway $apiKey = 'YOUR_API_KEY'; // Replace 'YOUR_CALLBACK_URL' with the URL of the PHP script itself (this file) $callbackUrl = 'YOUR_CALLBACK_URL'; // Replace 'YOUR_REDIRECT_URL' with the URL where users should be redirected after payment completion (optional) $redirectUrl = 'YOUR_REDIRECT_URL'; // Replace 'YOUR_ORDER_DESCRIPTION' with a description of the order $orderDescription = 'YOUR_ORDER_DESCRIPTION'; // Replace 'YOUR_ORDER_AMOUNT' with the actual amount to be paid $orderAmount = 'YOUR_ORDER_AMOUNT'; // Replace 'YOUR_ORDER_CURRENCY' with the currency code of the order (e.g., USD, EUR, etc.) $orderCurrency = 'YOUR_ORDER_CURRENCY'; // Create an array with the required parameters for the payment gateway $params = array( 'order_id' => $orderId, 'amount' => $orderAmount, 'currency' => $orderCurrency, 'description' => $orderDescription, 'callback_url' => $callbackUrl, 'redirect_url' => $redirectUrl ); // Perform necessary validations and database updates here based on the order details // Send a request to the payment gateway API $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'https://api.example.com/payment'); // Replace with the actual API endpoint curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($params)); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_HTTPHEADER, array( 'Content-Type: application/x-www-form-urlencoded', 'Authorization: Bearer ' . $apiKey )); $response = curl_exec($ch); curl_close($ch); // Process the response from the payment gateway if ($response !== false) { $responseData = json_decode($response, true); CHAT: ME: Write me the html form associated with the php script you wrote. ChatGpt: Certainly! Here's an example HTML form that you can use to initiate the Bitcoin payment process: CODE: <!DOCTYPE html> <html> <head> <title>Bitcoin Payment Form</title> </head> <body> <h1>Bitcoin Payment Form</h1> <form action="payment.php" method="post"> <label for="amount">Amount:</label> <input type="text" name="amount" id="amount" required><br><br> <label for="currency">Currency:</label> <input type="text" name="currency" id="currency" required><br><br> <input type="submit" value="Pay with Bitcoin"> </form> </body> </html>
-
DESCRIPTION: Error gets shown here: $dom->loadXML($xml); //LINE: 44 RESULT: I get echoed: **( ! ) Warning: DOMDocument::loadXML(): Start tag expected, '<' not found in Entity, line: 6 in C:\wamp64... on line 44 Call Stack # Time Memory Function Location 1 0.0034 362440 {main}( ) ...\crawler_Test.php:0 2 14.0745 365456 loadXML( $source = class SimpleXMLElement { public $sitemap = [0 => class SimpleXMLElement { ... }, 1 => class SimpleXMLElement { ... }, 2 => class SimpleXMLElement { ... }, 3 => class SimpleXMLElement { ... }] } ) 46 73 SiteMaps Crawled: --- Array ( ) Html Pages Crawled: --- Array ( ) Array ( ) Array ( ) Array ( ) 50 Array ( ) Array ( ) Array ( ) ** CODE <?php ini_set('display_errors',1); ini_set('display_startup_errors',1); error_reporting(E_ALL); //START OF SCRIPT FLOW. //Preparing Crawler & Session: Initialising Variables. //Preparing $ARRAYS For Step 1: To Deal with Xml Links meant for Crawlers only. //SiteMaps Details Scraped from SiteMaps or Xml Files. $sitemaps = []; //This will list extracted further Xml SiteMap links (.xml) found on Sitemaps (.xml). $sitemaps_last_mods = []; //This will list dates of SiteMap pages last modified - found on Sitemaps. $sitemaps_change_freqs = []; //his will list SiteMap dates of html pages frequencies of page updates - found on Sitemaps. $sitemaps_priorities = []; //This will list SiteMap pages priorities - found on Sitemaps. //Webpage Details Scraped from SiteMaps or Xml Files. $html_page_urls = []; //This will list extracted html links Urls (.html, .htm, .php) - found on Sitemaps (.xml). $html_page_last_mods = []; //This will list dates of html pages last modified - found on Sitemap. $html_page_change_freqs = []; //his will list dates of html pages frequencies of page updates - found on Sitemaps. $html_page_priorities = []; //This will list html pages priorities - found on Sitemaps. //Preparing $ARRAYS For Step 2: To Deal with html pages meant for Human Visitors only. //Data Scraped from Html Files. Not Xml SiteMap Files. $html_page_meta_names = []; //This will list crawled pages Meta Tag Names - found on html pages. $html_page_meta_descriptions = []; //This will list crawled pages Meta Tag Descriptions - found on html pages. $html_page_titles = []; //This will list crawled pages Titles - found on html pages. // ----- //Step 1: Initiate Session - Feed Xml SiteMap Url. Crawing Starting Point. //Crawl Session Starting Page/Initial Xml Sitemap. (NOTE: Has to be .xml SItemap). $initial_url = "https://www.rocktherankings.com/sitemap_index.xml"; //Has more xml files. //$xmls = file_get_contents($initial_url); //Should I stick to this line or below line ? //Parse the sitemap content to object //$xml = simplexml_load_string($xmls); //Should I stick to this line or above line ? $xml = simplexml_load_string(file_get_contents($initial_url)); //Code from Dani: https://www.daniweb.com/programming/web-development/threads/540168/what-to-lookout-for-to-prevent-crawler-traps $dom = new DOMDocument(); $dom->loadXML($xml); //LINE: 44 echo __LINE__; echo '<br>'; //LINE: 46 extract_links($xml); echo __LINE__; echo '<br>'; //LINE: 50 foreach($sitemaps AS $sitemap) { echo __LINE__; echo '<br>'; extract_links($sitemap); //Extract Links on page. } foreach($html_page_urls AS $html_page_url) { echo __LINE__; echo '<br>'; extract_links($html_page_url); //Extract Links on page. } scrape_page_data(); //Scrape Page Title & Meta Tags. //END OF SCRIPT FLOW. //DUNCTIONS BEYOND THIS POINT. //Links Extractor. function extract_links() { echo __LINE__; echo '<br>'; //LINE: 73 GLOBAL $dom; //Trigger following IF/ELSEs on each Crawled Page to check for link types. Whether Links lead to more SiteMaps (.xml) or webpages (.html, .htm, .php, etc.). if ($dom->nodeName === 'sitemapindex') //Current Xml SiteMap Page lists more Xml SiteMaps. Lists links to Xml links. Not lists links to html links. { echo __LINE__; echo '<br>'; //parse the index // retrieve properties from the sitemap object foreach ($xml->sitemapindex as $urlElement) //Extracts html file urls. { // get properties $sitemaps[] = $sitemap_url = $urlElement->loc; $sitemaps_last_mods[] = $last_mod = $urlElement->lastmod; $sitemaps_change_freqs[] = $change_freq = $urlElement->changefreq; $sitemaps_priorities[] = $priority = $urlElement->priority; // print out the properties echo 'url: '. $sitemap_url . '<br>'; echo 'lastmod: '. $last_mod . '<br>'; echo 'changefreq: '. $change_freq . '<br>'; echo 'priority: '. $priority . '<br>'; echo '<br>---<br>'; } } else if ($dom->nodeName === 'urlset') //Current Xml SiteMap Page lists no more Xml SiteMap links. Lists only html links. { echo __LINE__; echo '<br>'; //parse url set // retrieve properties from the sitemap object foreach ($xml->urlset as $urlElement) //Extracts Sitemap Urls. { // get properties $html_page_urls[] = $html_page_url = $urlElement->loc; $html_page_last_mods[] = $last_mod = $urlElement->lastmod; $html_page_change_freqs[] = $change_freq = $urlElement->changefreq; $html_page_priorities[] = $priority = $urlElement->priority; // print out the properties echo 'url: '. $html_page_url . '<br>'; echo 'lastmod: '. $last_mod . '<br>'; echo 'changefreq: '. $change_freq . '<br>'; echo 'priority: '. $priority . '<br>'; echo '<br>---<br>'; } } GLOBAL $sitemaps; GLOBAL $sitemaps_last_mods; GLOBAL $sitemaps_change_freqs; GLOBAL $sitemaps_priorities; GLOBAL $html_page_urls; GLOBAL $html_page_last_mods; GLOBAL $html_page_change_freqs; GLOBAL $html_page_priorities; echo 'SiteMaps Crawled: ---'; echo '<br><br>'; if(array_count_values($sitemaps)>0) { print_r($sitemaps); echo '<br>'; } elseif(array_count_values($sitemaps_last_mods)>0) { print_r($sitemaps_last_mods); echo '<br>'; } elseif(array_count_values($sitemaps_change_freqs)>0) { print_r($sitemaps_change_freqs); echo '<br>'; } elseif(array_count_values($sitemaps_priorities)>0) { print_r($sitemaps_priorities); echo '<br><br>'; } echo 'Html Pages Crawled: ---'; echo '<br><br>'; if(array_count_values($html_page_urls)>0) { print_r($html_page_urls); echo '<br>'; } if(array_count_values($html_page_last_mods)>0) { print_r($html_page_last_mods); echo '<br>'; } if(array_count_values($html_page_change_freqs)>0) { print_r($html_page_change_freqs); echo '<br>'; } if(array_count_values($html_page_priorities)>0) { print_r($html_page_priorities); echo '<br>'; } } //Meta Data & Title Extractor. function scrape_page_data() { GLOBAL $html_page_urls; if(array_count_values($html_page_urls)>0) { foreach($html_page_urls AS $url) { // https://www.php.net/manual/en/function.file-get-contents $html = file_get_contents($url); //https://www.php.net/manual/en/domdocument.construct.php $doc = new DOMDocument(); // https://www.php.net/manual/en/function.libxml-use-internal-errors.php libxml_use_internal_errors(true); // https://www.php.net/manual/en/domdocument.loadhtml.php $doc->loadHTML($html, LIBXML_COMPACT|LIBXML_NOERROR|LIBXML_NOWARNING); // https://www.php.net/manual/en/function.libxml-clear-errors.php libxml_clear_errors(); // https://www.php.net/manual/en/domdocument.getelementsbytagname.php $meta_tags = $doc->getElementsByTagName('meta'); // https://www.php.net/manual/en/domnodelist.item.php if ($meta_tags->length > 0) { // https://www.php.net/manual/en/class.domnodelist.php foreach ($meta_tags as $tag) { // https://www.php.net/manual/en/domnodelist.item.php echo 'Meta Name: ' .$meta_name = $tag->getAttribute('name'); echo '<br>'; echo 'Meta Content: ' .$meta_content = $tag->getAttribute('content'); echo '<br>'; $html_page_meta_names[] = $meta_name; $html_page_meta_descriptions[] = $meta_content; } } //EXAMPLE 1: Extract Title $title_tag = $doc->getElementsByTagName('title'); if ($title_tag->length>0) { echo 'Title: ' .$title = $title_tag[0]->textContent; echo '<br>'; $html_page_titles[] = $title; } //EXAMPLE 2: Extract Title $title_tag = $doc->getElementsByTagName('title'); for ($i = 0; $i < $title_tag->length; $i++) { echo 'Title: ' .$title = $title_tag->item($i)->nodeValue . "\n"; $html_page_titles[] = $title; } } } } if(array_count_values($html_page_meta_names)>0) { print_r($html_page_meta_names); echo '<br>'; } if(array_count_values($html_page_meta_descriptions)>0) { print_r($html_page_meta_descriptions); echo '<br>'; } if(array_count_values($html_page_titles)>0) { print_r($html_page_titles); echo '<br>'; } //END OF FUNCTIONS. ?> I can’t afford to get errors like this as not all websites will be code error free. I want to suppress such error that gets shown due to coding errors on webpages crawled on third party websites. How to do this ? NOTE: I should only get error if my own code (crawler is coded in error) has errors. I do not know how to suppress the error. I would like to know if there are any error on my coding that could cause issues while crawling the web or later on at any point. Regards!