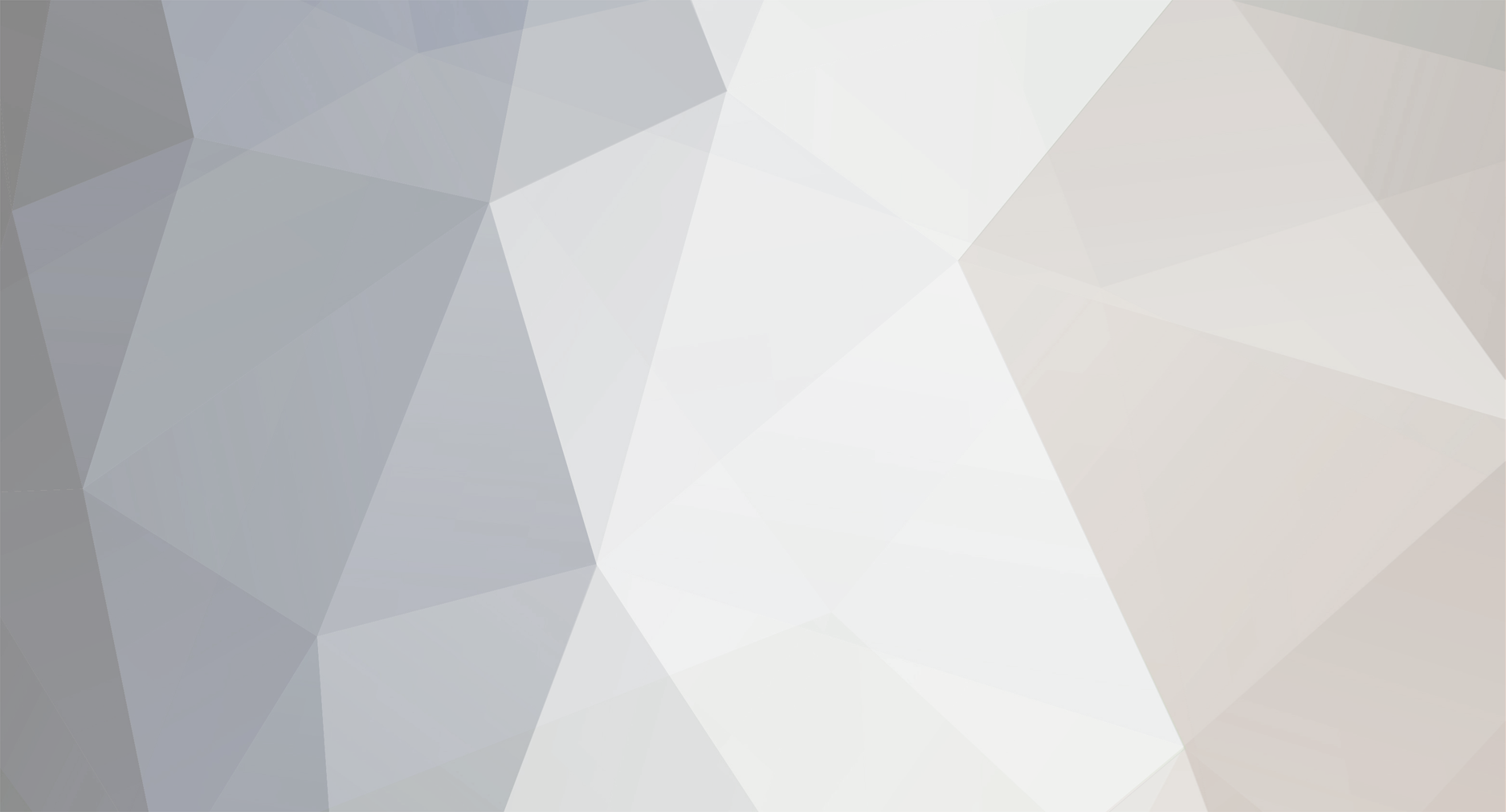
ahmedarain24
New Members-
Posts
6 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
ahmedarain24's Achievements
-
To resolve this issue, you can try the following steps: Increase the maximum number of user connections: You can do this by modifying the MySQL configuration file (typically my.cnf or my.ini). Look for the max_user_connections setting and increase its value. Keep in mind that increasing this value too much may have an impact on the performance of your server, so it's important to find a balance. Optimize your WordPress site: If your website is receiving a high number of simultaneous requests, it may be worth optimizing your site to reduce the number of database connections required. This can include caching techniques, database query optimizations, and minimizing unnecessary plugin usage. Check for problematic plugins or themes: Sometimes, certain plugins or themes can cause excessive database connections. Try disabling all plugins and switching to a default WordPress theme to see if the issue persists. If the problem goes away, you can then enable each plugin/theme one by one to identify the culprit. Use a database connection pooling plugin: There are plugins available for WordPress that implement database connection pooling, which can help manage and reuse database connections more efficiently. This can be useful in scenarios where your site consistently reaches the maximum number of connections. Contact your hosting provider: If you're unable to resolve the issue on your own, reach out to your hosting provider for assistance. They may be able to provide insights specific to your server environment and offer guidance on optimizing the database configuration.
-
I reviewed your code, and it seems that the issue lies in the conditional logic for displaying validation errors. In your code, the validation errors are displayed within the else block of the main if ($_SERVER['REQUEST_METHOD'] === 'POST') condition. However, this else block only executes when the form is not submitted or when there are validation errors. To fix the issue and ensure that the validation errors are displayed correctly, you can move the error display code outside of the else block and place it after the HTML form. Here's the modified code: <?php // ... Your existing code ... if ($_SERVER['REQUEST_METHOD'] === 'POST') { // ... Your existing validation code ... // If there are no validation errors, store the form data if (empty($errors)) { // ... Your existing code ... } } // Display validation errors if any if (!empty($errors)) { echo '<div id="confirmedError">'; echo '<p>Please fix the following errors:</p>'; echo '<ul>'; foreach ($errors as $error) { echo '<li>' . $error . '</li>'; } echo '</ul>'; echo '</div>'; } ?> By moving the error display code outside the else block, it will be executed regardless of whether the form was submitted or not. This way, when there are validation errors, they will be displayed correctly below the form. Make sure to place the modified code in the appropriate location within your file, following the HTML form
-
It seems like you're experiencing an issue where the array containing product information is not accessible or empty when including it in different files. This could be due to the scope of the variables or the order in which the files are included. To address this, you can consider the following approaches: 1. Use sessions: Store the product information in a session variable instead of an included file. You can set the session variable in one file and access it in other files where you need the product information. Make sure you start the session at the beginning of each file using `session_start()`. For example: In the file where you create the array: ```php session_start(); $_SESSION['product_info'] = $productInfoArray; ``` In the file where you generate the PDF or need the product information: ```php session_start(); $productInfoArray = $_SESSION['product_info']; ``` 2. Use a configuration file: Instead of including a file that creates the array, you can define a configuration file where you store the product information as constants or variables. Include this configuration file in the files where you need the product information. For example: In the configuration file (`config.php`): ```php $productInfoArray = [ // Product information here ]; ``` In the file where you generate the PDF or need the product information: ```php include 'config.php'; // Use $productInfoArray here ``` 3. Pass the array as a function parameter: Instead of including a separate file, you can create a function that returns the product information array and call that function wherever you need the information. For example: In a common file (`functions.php`): ```php function getProductInfo() { return [ // Product information here ]; } ``` In the file where you generate the PDF or need the product information: ```php include 'functions.php'; $productInfoArray = getProductInfo(); ``` By using one of these approaches, you can ensure that the product information array is accessible across different files in your project. Choose the approach that best fits your project structure and requirements.
-
Based on the code snippet you provided, it appears that you're using the variable `$search` in your SQL query. However, the issue might lie in the way you are constructing and using this variable in your PHP code. Here are a few things to consider: 1. SQL injection vulnerability: Directly interpolating user input into SQL queries can expose your application to SQL injection attacks. It is highly recommended to use prepared statements or parameterized queries to prevent this vulnerability. Here's an example of how you can modify your code to use prepared statements: ```php // Assuming you have a database connection established earlier $search = '%'.$search.'%'; // Add wildcards to match any occurrences of the search term $sql = "SELECT * FROM user_data WHERE CONCAT_WS(' ', firstname, lastname) LIKE ?"; $stmt = $pdo->prepare($sql); $stmt->execute([$search]); $results = $stmt->fetchAll(); ``` 2. Wildcards in the LIKE comparison: To match partial names, you need to include wildcard characters (`%`) in the `LIKE` comparison. I've modified the code snippet above to add the `%` wildcards before and after the `$search` variable. Make sure that the value of `$search` contains the actual search term you want to use. 3. Debugging and error handling: If you're still not getting any results, it's a good practice to add error handling to your code to catch any potential errors. You can use `try-catch` blocks or check for errors using `PDO::errorInfo()`. ```php try { // Assuming you have a database connection established earlier $search = '%'.$search.'%'; // Add wildcards to match any occurrences of the search term $sql = "SELECT * FROM user_data WHERE CONCAT_WS(' ', firstname, lastname) LIKE ?"; $stmt = $pdo->prepare($sql); $stmt->execute([$search]); $results = $stmt->fetchAll(); } catch (PDOException $e) { echo "Error: " . $e->getMessage(); } ``` By implementing these modifications, you should be able to execute the search query correctly in PHP and retrieve the desired results from the MySQL database.
-
Based on the error message you provided, it seems that the hostname xxxxxxx.hosting-data.io cannot be resolved. This could be due to a few potential reasons: Incorrect hostname: Please double-check that the hostname you are using is correct. Ensure there are no typos and that the hostname corresponds to the correct database server provided by ionos. Network or DNS issues: The error ENOTFOUND suggests that the hostname cannot be found. This could be caused by network connectivity issues or DNS resolution problems. Ensure that your network connection is stable and try running the code again. You can also try using a different network or DNS server to see if that resolves the issue. Firewall or security restrictions: It's possible that there are firewall rules or security restrictions in place that prevent your Node.js application from accessing the specified hostname or port. Check if there are any network-level restrictions in your environment, such as firewall settings, that might be blocking the connection. Additionally, make sure you have installed the mysql package by running npm install mysql in your project directory. This command installs the MySQL driver for Node.js, which is necessary for establishing a connection with the MySQL database. If you continue to experience issues, it might be helpful to consult with your hosting provider (ionos) for further assistance and ensure that you have the correct hostname, port, username, and password for connecting to the MySQL database.
-
Based on the code you provided, it seems that you are trying to convert the value of `newent` to uppercase using the `strtoupper()` function. However, it appears that the uppercase conversion is not working as expected and accepting any character. To properly convert the value to uppercase in JavaScript, you should use the `toUpperCase()` method instead of `strtoupper()`. Here's the corrected code: ```javascript newent.value = newent.value.toUpperCase(); if (newent.value === 'B' || newent.value === 'P' || newent.value === 'T' || newent.value === 'D') { EnterSw.value = 0; return true; } else { alert('INVALID HAND / MAIN INVALID'); newent.select(); return false; } ``` With this modification, the `toUpperCase()` method will convert the value of `newent` to uppercase, and the subsequent comparisons will check against uppercase characters ('B', 'P', 'T', 'D').