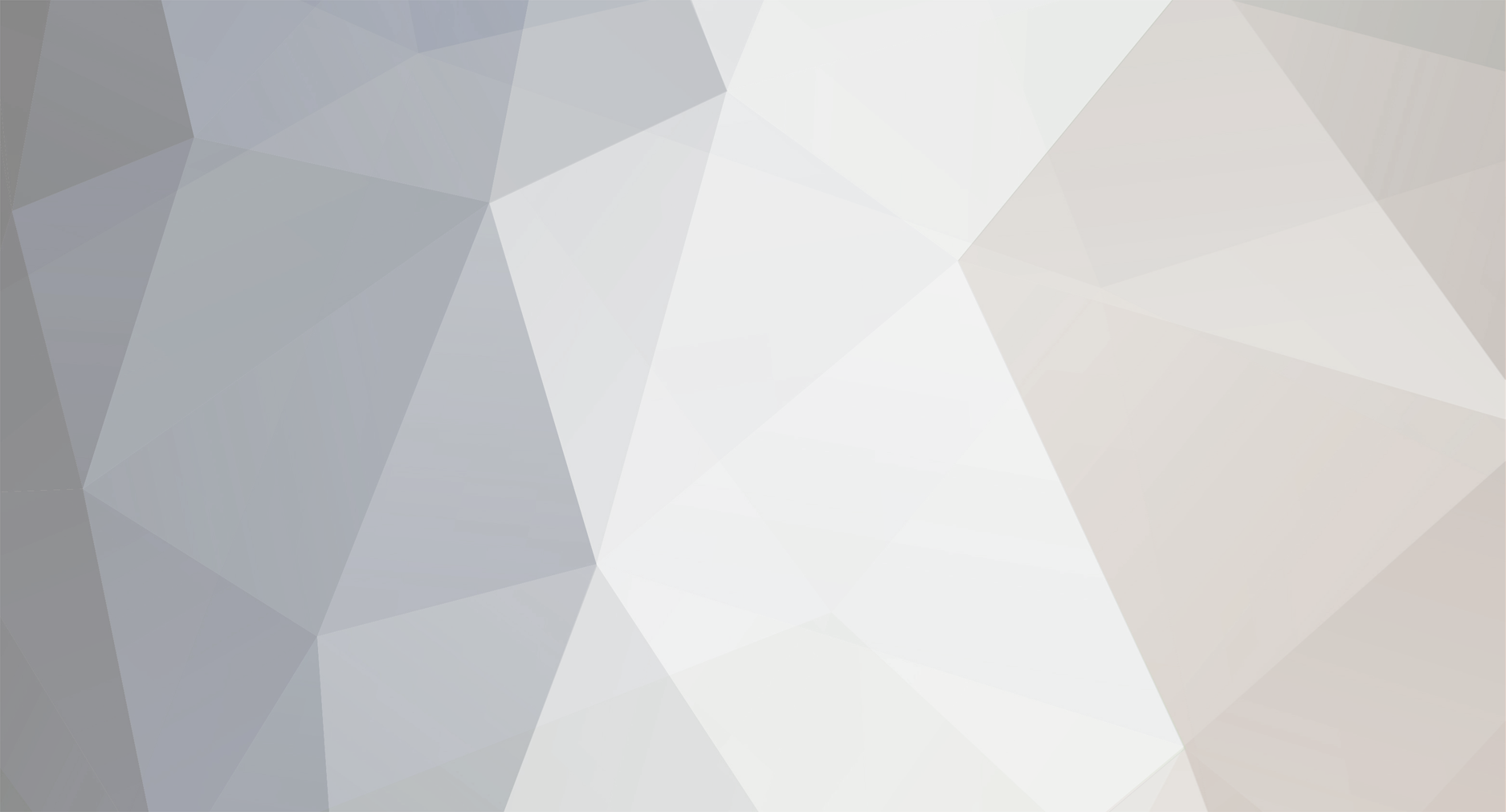
hadoob024
Members-
Posts
192 -
Joined
-
Last visited
Everything posted by hadoob024
-
I'm having a similar problem. Are you able to create MySQL users using phpMyAdmin? I've read through the docs and searched the forums but can't seem to find an answer to the question.
-
could there be a problem with the if/else logic? could it just not be getting to that part of the code, which is why no cookie is being set? does the header redirection work? so it seems that if you just run the test script, where the only command is the setcookie() command, it works, right? i wonder if that header right after the setcookie() command is causing the cookie not to be set. just to test it, i'd try commenting out the header() and putting an exit() in there. if the cookie gets set, then you know what the problem is.
-
Here's the function declaration for setcookie(): [code] bool setcookie ( string name [, string value [, int expire [, string path [, string domain [, bool secure]]]]] ) [/code] Setcookie() expects an integer for the third parameter, but I think you called it using a string value as the third parameter.
-
Have you tried something like: [a href=\"http://www.dtheatre.com/scripts/formmail\" target=\"_blank\"]http://www.dtheatre.com/scripts/formmail[/a]
-
I use the following, and this doesn't clear the form, and allows the user to go back to the form page with all their answers intact: [code] echo '<FORM><INPUT TYPE="button" VALUE="Back" onClick="history.go(-1);return true;"></FORM>'; [/code]
-
Cleaning information retrieved from database
hadoob024 replied to hadoob024's topic in PHP Coding Help
Yup. I do that too. I check lengths and type of info entered into the form, then I set a variable equal to the $_POST variable passed thru. I then verify it using eregi(). I also use trim(), strip_tags(), etc. And only after it passes all these checks do I actually store the info in the db. But the book suggested that just to be on the safe side, to also then verify the info when it's pulled out of the db but before displaying it. -
Cleaning information retrieved from database
hadoob024 replied to hadoob024's topic in PHP Coding Help
Well, this is for a real estate website, so let's see, I have 8 small text fields (like around 30 chars), 2 integer fields, and 1 field for a listing description that's 240 chars max. Like I know to use htmlentities() to clean up these fields for proper display, but do I need to run everything through some eregi() checks or something to validate the information again before displaying it? Or does this all depend on how secure the db server is? -
I was reading through this PHP security book and it recommends cleaning/screening/sanitizing information retrieved from the db prior to displaying it. Is this something that everyone recommends, or is it considered overkill?
-
hmm... maybe your problem is that $_SESSION variables are accessed using the following syntax: $_SESSION['seclevel'] and in your query string, you're accessing the value using: $_SESSION[seclevel] maybe you're just missing the single quotes? i've also read about using the tickmarks (`) around table and field names. could this be what's missing?
-
Is there a way to see if a connection is open? Like after I open a connection, if I encounter an error, before exiting out of the script, I send myself an email with the error message and append any error message to my error_log. But I also wanted to check and see if there're any open db connections, and if so, close them out before exiting. Is this possible? I'm looking at the mysql functions right now, and can't tell if any of them can help me with this.
-
Cool! Hey man, THANKS for all the help!!! Now that I know what I'm doing, I think I'm ready to get started.
-
that makes sense. yeah. i didn't even consider something going wrong with just deleting old records. i just assumed that as long as the code was ok, and i thoroughly tested it, that i would be ok. i'm glad i decided on posting this topic. removing the item from the main table, and storing it in a archive/storage table definitely sounds like the way to go. probably saved me from a ton of headaches down the road.
-
oh no, i wasn't just writing it off altogether. just for my situation in particular. i don't need to know or keep track of users who don't enter in information for the two fields that i'm talking about. so if they don't enter anything, i'll just store an empty string. that sounds right, right?
-
cool man... thanks for the tips. yeah, i think i'll end up going with the NOT NULL value, and if for one of my fields nothing is entered, i'll just store "" in the db for that field value. thanks again..
-
Cool. Thanks! A couple of follow-ups to your replies. So how do I define the empty string value, just leave it blank and do anything, and MySQL interprets this as an empty string? Also, instead of allowing NULL values, where a value isn't entered in my form, should I just store a simple text string in the db for that field like "nothing"? And then when searching on the db, I check the value of this field, and if it's equal to "nothing", I just ouput blank space? Thanks a bunch!
-
Hmm. That does make sense. How difficult is the process of dumping the info to another table? Also, on a side note, what are your thoughts for storing images in a db? Because I will also need to think of how to handle the images associated with the listing information that I'm storing.
-
OK. I've been messing around with PHP now for a bit and I think I'm getting comfortable with it, so now I'm ready to implement the database that I've designed. It's for a real estate site, so here're the fields that I have and the data types that they're gonna be: uniqueid (auto-incrementing & primary key) - INT firstname - CHAR lastname - CHAR phonenumareacode - TINYINT phonenumprefix - TINYINT phonenumsuffix - TINYINT emailaddress - CHAR companyname - CHAR askingprice - INT listingtype (values are numbers 1-16) - TINYINT location (2 or 3 letter values) - CHAR city - CHAR listingdescription - CHAR picture (store path) - CHAR dateadded - BIGINT Now, all the fields are required except for "picture" and "companyname", so based on a previous post that I read, I guess that: "If you want a column to store NULL when there is no data then you set it to allow null and the default to null." So a couple of questions that I have are: 1) Do I need to define default values for all my table fields, or just the ones that don't require a value to be inserted? 2) I'm using phpMyAdmin to do my MySQL work, how do I define the default value to be null? Just type in NULL? I checked the documentation but it doesn't say. 3) I want "uniqueid" to be auto-incrementing and the primary key, so I know that when performing an INSERT, I don't insert a value for this field. But what I want to know is that if this is the value that is returned by the function mysql_insert_id()? Well, that's about it. I wish there was some more useful information in the phpMyAdmin documentation so that I could figure these out for myself. Oh well. Thanks.
-
cool. thanks. yeah, i wasn't sure about it. i guess i'll have to go with plan b. i was thinking of adding a new field to my db that stores the timestamp. and then check this value in my script that performs searches before the section that performs the search. something like: $postdate = time() - 2592000; $timestampquery = mysql_query("DELETE FROM tablename WHERE dateadded < '$postdate'") or trigger_error('Error deleting records older than 30 days\r\n'.mysql_error(), E_USER_ERROR); is that what you usually do?
-
My web hosting company offers phpMyAdmin to administer MySQL dbs. My question is, is there anyway to have records that are older than X amount of time be deleted automatically? Or do I need to add some PHP code to my site to handle this? thanks.
-
How about something like: [code] <?php // check for posted form if (isset($_POST['login'])) { // see if the code the user typed matched the generated code if (strtoupper($_POST['code']) == $_SESSION['code']) { $_SESSION['uid'] = $uid; $_SESSION['pwd'] = $pwd; $sql = "SELECT * FROM user WHERE userid = '$uid' AND password = PASSWORD('$pwd')"; $result = mysql_query($sql); if (!$result) { error('A database error occurred while checking your '.'login details.\\nIf this error persists, please '. 'contact [email protected].'); } if (mysql_num_rows($result) == 0) { unset($_SESSION['uid']); unset($_SESSION['pwd']); } echo 'Congratulations, you entered the correct code.'; } else { echo 'You have entered the wrong code. Please <a href="index.php">try again</a>.'; } } else { ?> [/code]
-
Hello. I got a quick question. My site's split up into 2 files. The form page and the form processing page. Anyway, I was implementing sessions to help for security purposes, but the problem was that it was creating was that if a user made a mistake and had to go back to the form, using sessions would cause all the form fields to clear. I came across this suggestion to preserve the entered values for the form fields and have a question regarding the session_cache_limiter() command: [code]header("Expires: Sat, 01 Jan 2000 00:00:00 GMT"); header("Last-Modified: ".gmdate("D, d M Y H:i:s")." GMT"); header("Cache-Control: post-check=0, pre-check=0",false); session_cache_limiter("must-revalidate"); [/code] Anyway, I just wanted to know what the repercussions are for doing this? Is this going to cause other issues? Thanks.
-
OK. I seemed to have gotten past the permissions error. I changed the permissions of that folder and now I'm not getting those errors anymore. I'm still getting jumbled up garbage when I try to display the image though. Here's the code: [a href=\"http://pastebin.com/633809\" target=\"_blank\"]http://pastebin.com/633809[/a] Any thoughts on why the image isn't being displayed?
-
i've actually been working on putting together some secure code for handling file uploads. i'm also trying to validate an image file that gets uploaded. here's the code that i have so far: [code] //This section of code handles the image file upload. //First we verify that something was uploaded. if (($_FILES['picture']['size'] != 0) && ($_FILES['picture']['tmp_name'] != '')) { //checks and sees if there were any errors during the upload process if ($_FILES['picture']['error'] > 0) { switch ($_FILES['picture']['error']) { //file size is greater than that allowed by PHP as set in php.ini case 1: errorcheck(1, '<B>File size exceeded maximum allowable file size of 100k.</B><P>'); break; //file size is greater than that allowed by MAX_FILE_SIZE as set in form case 2: errorcheck(1, '<B>File size exceeded maximum allowable file size of 100k.</B><P>'); break; case 3: errorcheck(1, '<B>File only partially uploaded.</B><P>'); break; case 4: errorcheck(1, '<B>No file uploaded.</B><P>'); break; default: errorcheck(1, '<B>An unknown file upload error occurred.</B><P>'); break; } exit; } //need to check and make sure file MIME type is acceptable if (($_FILES['picture']['type'] != 'image/jpeg') && ($_FILES['picture']['type'] != 'image/jpg') && ($_FILES['picture']['type'] != 'image/gif') && ($_FILES['picture']['type'] != 'image/pjpeg')) { errorcheck(1, '<B>File is not of correct type. <BR />Valid file types are ".JPG", ".JPEG", or ".GIF".</B><P>'); } //this is a simple quick test. if accessing the picture is impossible, //or if it isn't a valid picture, getimagesize() will return FALSE. if (!getimagesize($_FILES['picture']['tmp_name'])) { errorcheck(1, '<B>File is not of correct type. <BR />Valid file types are ".JPG", ".JPEG", or ".GIF".</B><P>'); } //checks if picture is an uploaded file if (is_uploaded_file($_FILES['picture']['tmp_name'])) { //pathname for where we're moving file $picpath = '/home/virtual/site109/fst/var/www/html/uploadtmp/'.$_FILES['picture']['name']; //checks if file size is less than 100k if (filesize($_FILES['picture']['tmp_name']) <= 102400) { //double-checking filetype as the 'type' field check from above //isn't always reliable or secure $name = $_FILES['picture']['name']; $temparray = explode(".", $name); $ext = array_pop($temparray); if (($ext != "jpg") && ($ext != "jpeg") && ($ext != "JPG") && ($ext != "JPEG") && ($ext != "gif") && ($ext != "GIF")) { errorcheck(1, '<B>File is not of correct type. <BR />Valid file types are ".JPG", ".JPEG", or ".GIF".</B><P>'); } //moving file to location specified by $picpath if (!move_uploaded_file($_FILES['picture']['tmp_name'], $picpath)) { //encrypting email address to avoid spam-bots $support = email_encrypt('[email protected]'); //composing error message for file not being moved properly $errormsg = '<B>There was a problem processing the image file that was uploaded.<BR /> Please try again. If problem persists, continue without uploading a picture,<BR /> and email the image separately as an attachment to '.$support.'.'; errorcheck(1, $errormsg); } } //if it's not less than 100k else { errorcheck(1, "<B>File size has exceeded maximum allowable file size of 100k.</B><P>"); } } //if file is not a uploaded file, but something is there else { $errormessage = 'Possible file upload attack with file: '.$_FILES['picture']['name']; trigger_error($errormessage, E_USER_ERROR); } //if file is uploaded successfully, then it falls through all the if/else //statments and ends up here which means that we have a successful upload. $uploadsuccess = 1; } //need to check if no file was selected for upload from our form or if nothing was //uploaded because it exceeded the constant MAX_FILE_SIZE else { if (isset($_FILES['picture']['name'])) { if ($_FILES['picture']['name'] != '') { errorcheck(1, "<B>File size has exceeded maximum allowable file size of 100k,<BR />and might or might not be the correct file type.</B><P>"); } } } [/code] Let me know what you think...
-
OK. So it's looking like I can't just take the uploaded image and display it. It seems like I have to move it to a temp directory and then I can display it. Anyway, I just modified my file upload script. Everything works fine until the following. I first specify a pathname to a directory that I created called "uploadtmp" which resides in my public HTML folder: [code]$picpath = '/home/virtual/site109/fst/var/www/html/uploadtmp/'.$_FILES['picture']['name'];[/code] I then move the file to this location using the following: [code]if (!move_uploaded_file($_FILES['picture']['tmp_name'], $picpath))[/code] However, this command gives me the following error message: "Error Message: move_uploaded_file(/home/virtual/site109/fst/var/www/html/uploadtmp/akira01.jpg): failed to open stream: Permission denied Error Code: 2" What is causing this permissions error? Thanks.
-
hmmm... that might work, but i know that i've already sent some html as output, so i'm guessing that i will end up getting one of those "cannot modify header" error messages. think there's anything else i can try?