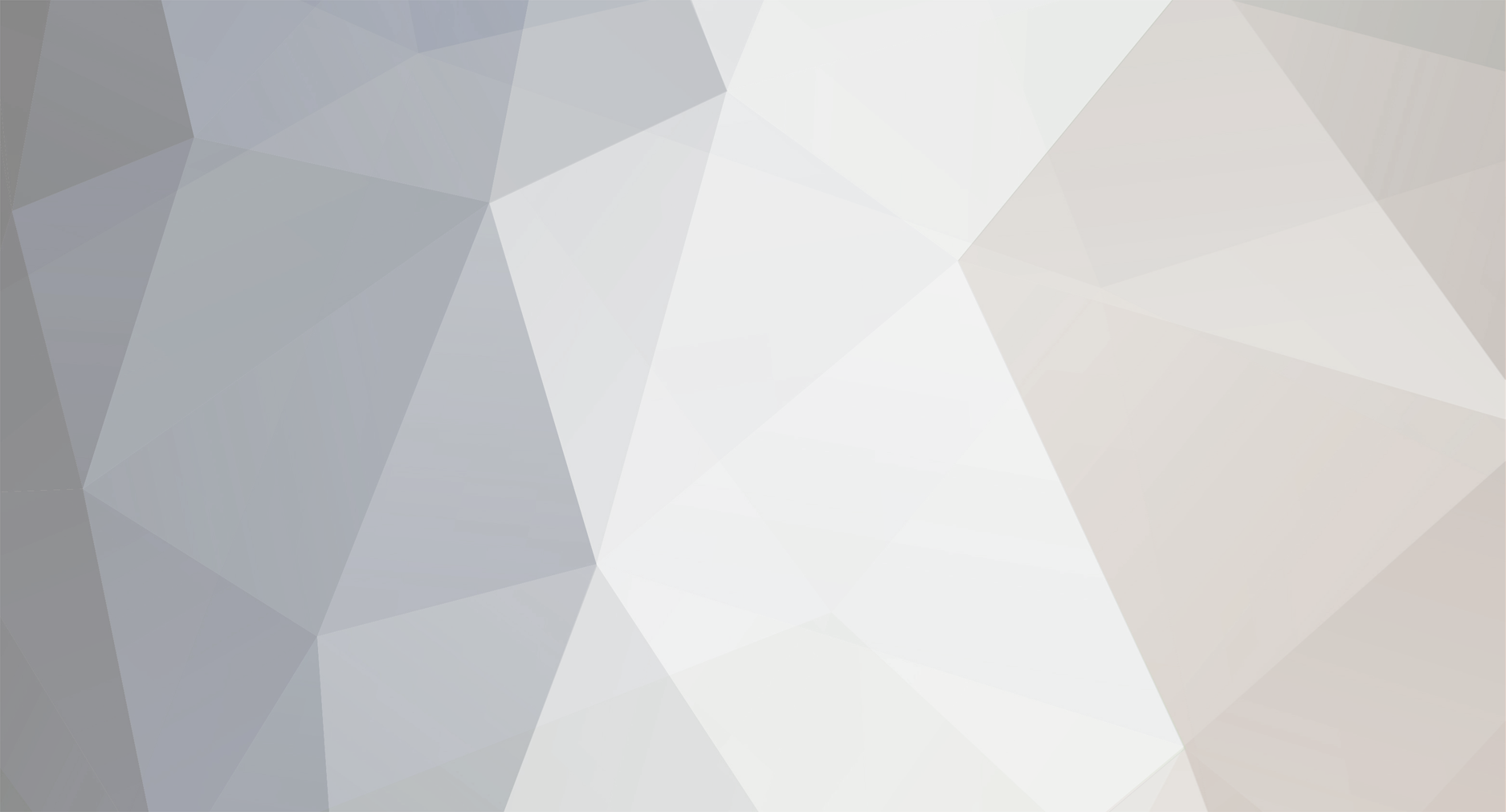
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Here's a cleaned up version: <?php $user_area_location = 'account.php'; // Location of the user area $error = array(); if (isset($_GET['action'])) { switch ($_GET['action']) { case 'logoff': unset($_SESSION['loggedIn']); array_push($error, 'You were logged off.'); break; } } if (!$error) { if (empty($_POST['username'])) { array_push($error, 'You didn\'t supply a username'); } if (empty($_POST['password'])) { array_push($error, 'You didn\'t supply a password'); } function filterUsername($username) { return preg_replace('/[^a-z]/i', '', $username); } function filterPassword($password) { return preg_replace('/[^a-z0-9]/i', '', $password); } $username = filterUsername($_POST['username']); $password = filterPassword($_POST['password']); $result = mysql_query("SELECT username, email, name FROM `users` WHERE username = '$username' AND password = md5('$password')"; if (false !== $result && mysql_num_rows($result)) { $row = mysql_fetch_assoc($result); $_SESSION['loggedIn'] = true; $_SESSION['userName'] = $row['username']; $_SESSION['userMail'] = $row['email']; $_SESSION['name'] = $row['name']; header('Location: ' . $user_area_location); exit(0); } else { array_push($error, 'The username or password you provided were not correct'); } } ?> <table cellspacing="2" cellpadding="0" border="0"> <form method="post" action="login.php"> <tr> <td>Username:</td> <td><input type="text" name="username"/></td> </tr> <tr> <td>Password:</td> <td><input type="password" name="password"/></td> </tr> <tr> <td></td> <td><input type="submit" name="submit" value="Login!"/> <a href="forgot.php">I forgot my username or password</a></td> </tr> </form> </table> <?php if (!empty($error)): ?> <div id="error2"> <ul> <?php foreach ($error as $key => $value): ?> <li><?php echo '<li>' . $value . '</li>'; ?></li> <?php endforeach; ?> </ul> </div> <?php endif; ?> @twilitegxa md5($password) won't help you as it leaves you vulnerable to an attack (Rainbow Table) and you should instead use a salt, like: md5( concat( password_salt, md5( '$password' ) ) )
-
Storing calculation results in database, or doing them on the fly?
ignace replied to galvin's topic in MySQL Help
I am unfamiliar with NCAA but you would store all points a player has won during a round, at the end of the game you would sum all sub totals to a total for ranking. round #1: player_id, points 3, 5 .. round #2: player_id, points 3, 2 ... SELECT sum(points) AS total_points FROM table GROUP BY player_id. You could store the total points in a separate rankings table so the calculation only has to be done once (the points in a rankings table should never change). -
This is a really simple assignment function GenerateTree($size) { $tree = ''; if ($size < 9 || $size > 17) $size = 9; //credit JonnyThunder for ($x = 0; $x < $size; $x++) { $spacer = str_repeat('0', ($size - $x)); $stars = str_repeat('*', ($x*2)+1); $tree .= $spacer . $stars . $spacer . "\n"; } //end credit $spacer = str_repeat('0', ceil($size/2)-1); $tree .= $spacer . '*' . $spacer . "\n"; $tree .= $spacer . '*' . $spacer . "\n"; $tree .= $spacer . '*' . $spacer . "\n"; return $tree; } function DisplayGreeting($type, $size = 9) { $tree = GenerateTree($size); $coloredTree = ''; foreach (str_split($tree) as $char) { $coloredTree .= DisplayChar($type, $char); } return $coloredTree; } function DisplayChar($type, $char) { $colors = array('red', 'gold');//favorites if ($type === 'holiday greeting tree') $colors = array('red', 'green'); if ($type === 'Sac State') $colors = array('gold', 'green'); //if ($type === 'surprise') // default if ($char != 0 && $char != '*') return $char; if ($char == 0) return '<span style="color:'.$colors[0].'">0</span>'; if ($char == '*') return '<span style="color:'.$colors[1].'">*</span>'; } Seems like you will be the only one who gets that extra 30%
-
True, but it also leaves your application in an unusable state and the back-button may not be an option as it may contain the root-cause (eg POST) resulting in the user re-entering the URL (think reboot the application/system). It's good to log exceptions and the usage of an ID/support-ticket is more then ideal, but with some added features you could increase usability: catch (Exception $e) { $ID = Site::TopLevelException($e);//log & return ID // this page is mostly static include('errors/uncaught-exception.php');//display's support-ID and provides extra information + options exit(0); } A click is easier then manually re-entering the entire URL
-
Yes, this is perfectly possible. By default your URL will look like (and all translations will translate to this one): http://www.domain.top/my/page Your database provides translations for my & page for other languages, so in Dutch I would be able to access your page like: http://www.domain.top/mijn/pagina Or in French: http://www.domain.top/mon/page Based on the locale (fr_FR, nl_BE) you would translate everything after domain.top to your default language (hence the word translation).
-
You could create something like: acl (user_id, role_id, permission, rule) Or you could create something like: user (.., group_id, .., permission, ..) group (.., permission, ..) where permission is an integer-value and acts as a bit-map where each bit represents an allowed/disallowed rule, easy and effective if you only have <= 32 permissions (bigint gives you 64) This has the advantage of being able to give individual permissions, select it like: SELECT g.permission | u.permission AS permissions, .. FROM user u JOIN group g ON u.group_id = g.id WHERE .. Use it in your application like: if ($row['permissions'] & SOME_PERMISSION) I just wrote this out of the top of my head and am actually uncertain their is a perfect conversion between MySQL int and PHP int altough it should work (for the int-part). A bigint will result in a float and possibly in a loss of data.
-
Why not just download TinyMCE it's free. http://tinymce.moxiecode.com/download.php
-
I'm secretly hijacking your thread Your .htaccess should something like: Options +FollowSymlinks RewriteEngine On RewriteRule (.*) city.php?name=$1 Your PHP would then be something like: if (empty($_GET['name'])) { header('Location: ..'); exit(0); } function filterCityName($cityName) { return preg_replace('/[^a-z]/i', '', $cityName); } $name = filterCityName($_GET['name']); $query = "SELECT .. FROM cities WHERE name = '$name'"; $result = mysql_query($query); if (false === $result) { trigger_error("Query '$query' failed and returned " . mysql_error()); header('Location: ..'); exit(0); } $row_count = mysql_num_rows($result); if (0 === $row_count) { //unknown city name provided //what should be the procedure? } //what should be displayed?
-
Otherwise your PHP will not be displayed but will show up in the HTML source. PHP code is harmless until you include- or eval it.
-
That technique has some disadvantages like: 1) Before you are able to edit the data you need to strip all HTML, and afterwards again apply the template before you insert it into the database. Which means that you have each template twice (once on file, and once in your DB). 2) If the user wants to export the data to some different format (eg PDF, XML) all data has to be stripped prior to the PDF generation. Which makes your data inflexible. Basically, your technique renders your DB completely useless and you would have been better off if you would have just when the user submits his data, apply the template on the data and store it into a .html file instead of passing through the DB (re-doing the entire process every time the user edits the page data). A setup like eg templates (id, name, path) contents (id, template_id, title, summary, ..) would have been more beneficial.
-
Teddy, that's you
-
Store HTML in a database? Your data should be format-independent. Your entire explanation makes me wonder if you have ever heard of a template (engine)? @siric I have seen websites that deployed a CMS system where they should have clearly used a tailored solution eg http://www.mijn-recepten.be/hoofdgerechten - great if you are looking for a recipe that starts with the letter K
-
You'll need a .htaccess with Rewrite rules to create (search (or ask) the Apache forum) http://www.travel.com/london http://www.travel.com/paris http://www.travel.com/tokyo This will pass london/paris/tokyo to a pre-defined script (eg city.php?name=london) Query the DB and display the result.
-
Blizzard and Facebook uses it, I've toyed with it in the past but it never appealed to me. It's quite useful when you don't have access to a scripting language or if you don't mind that all data (XML) is publicly available (unless you parse XSLT server-side, but that defeats the purpose IMO). I think I've found a CMS application once that was hosted on a central PHP server and allowed you to add FTP-servers, add data, add HTML/CSS templates, and drag-and-drop (+adjust look eg number of items) data on these templates. Once you were done, you could publish (XML+XSLT+CSS+images) it to your FTP-server. I tried to find it through Google but nothing turned up. Using my delicious bookmarks I found pagelime (http://pagelime.com/) but it's not what I was looking for. I remember it was still in beta when I first came across it.
-
@Teddy avoid the use of * as much as you can and write the field names you which to use. This avoids that your memory is polluted with data that your program doesn't use.
-
So, any exceptions will need to be defined, is that what you mean? Since i have to throw an exception for it to be caught it would be only if i misspelled or tried to throw an undefined exception? It's best to execute your program within a try/catch block with "catch (Exception $e)" to make sure no exception is left uncaught which is a likely case as your program is subject to change, new classes/exceptions can be introduced. And a slightest mistake may result in an unusable state for the end-user. A good example are some Java-based applications like Eclipse which has(/had?) a tendency to stop running while you were still writing code (due to some background tasks), something like: Really annoying. Oh I will be using this object for extra error information, My objects will retain error information specific to the functions being used, so having access to the object itself during an error should be quite useful in logging the error in as much detail as possible. I already thought you would say something like this but how do you know which methods the object exposes?
-
Oh, I thought you meant to write his own xmlns:rhodry so he could use something like <rhodry:talk message="hello world"/> all perfectly possible using X-series stuff (I think, I can vaguely remember we did something similar in an XSLT class)
-
What do you mean by a contact management tool? Something like an agenda?
-
Completely misses the point of wire-frames, as they are meant to be quick, rough, and to give you and your client an idea of where should be what? Not, how should it look? During a meeting you would refine these wire-frames to match your client's view. A mock-up (Photoshop wire-frame) just doesn't give you that flexibility, your client can easily take your pen and draw a few boxes, it's much harder for him to draw those same boxes in your Photoshop program, and drawing on a Photoshop print-out is possible but hard to spot. Not to mention that a mock-up easily takes about an half-hour to an hour to create, putting the focus on detail where it should have been on layout. Prototyping indeed has quite some advantages 1) client can see results quickly, leading to an increased trust 2) clients can "feel" their website 3) prototypes can be transformed to HTML templates 4) many WYSIWYG tools available to create the prototype (http://www.axure.com/) And some disadvantages: 1) The more reliable, feature-rich products are quite costly 2) Most tools miss widget's that are considered common (eg YouTube player, Twitter, ..) 3) Focus may shift towards detail instead of layout 4) time-consuming if more complex tasks needs to be wire-framed (eg form with ajax dropdown) 5) difficult for the client to add changes All-in-all I like wire-frames they are quick, rough, but get the point across. One constant in software engineering: CHANGE
-
Hi Rhodry, Do a read-up on XSLT, DTD, XML, XPath, XQuery, .. as that's what you are looking for. Afterwards you can take it a step further and like Daniel suggested write your own. xmlns:rhodry="path/to/rhodry/dtd" (<rhodry:talk/>) http://www.w3schools.com - is a good place to start
-
The biggest draw-back of exception's is that when not caught, they leave your website in an unusable state to the end-user. I can't yet find any draw-backs to this, but for what would you use it?
-
Have you tried Googling?
-
The best way would probably be to use single quotes and realpath, like: $filepath = realpath('files\subfolder1\test.txt'); realpath modifies the directory separator to match the OS directory separator.
-
You want blabla.com to be a service which other websites query to validate/fetch certain data? Client: $hwid = 234325; $username= 'Mastershake'; $response = file_get_contents('http://www.blabla.com/find.php?' . http_build_query(array('hwid' => $hwid, 'username' => $username)); Server: $hwid = $_GET['hwid']; $username = $_GET['username']; //query database if (false !== $result && mysql_num_rows($result)) { echo 'Valid'; exit(0); } echo 'Invalid'; This is very basic and you may want to look into RESTful and SOAP