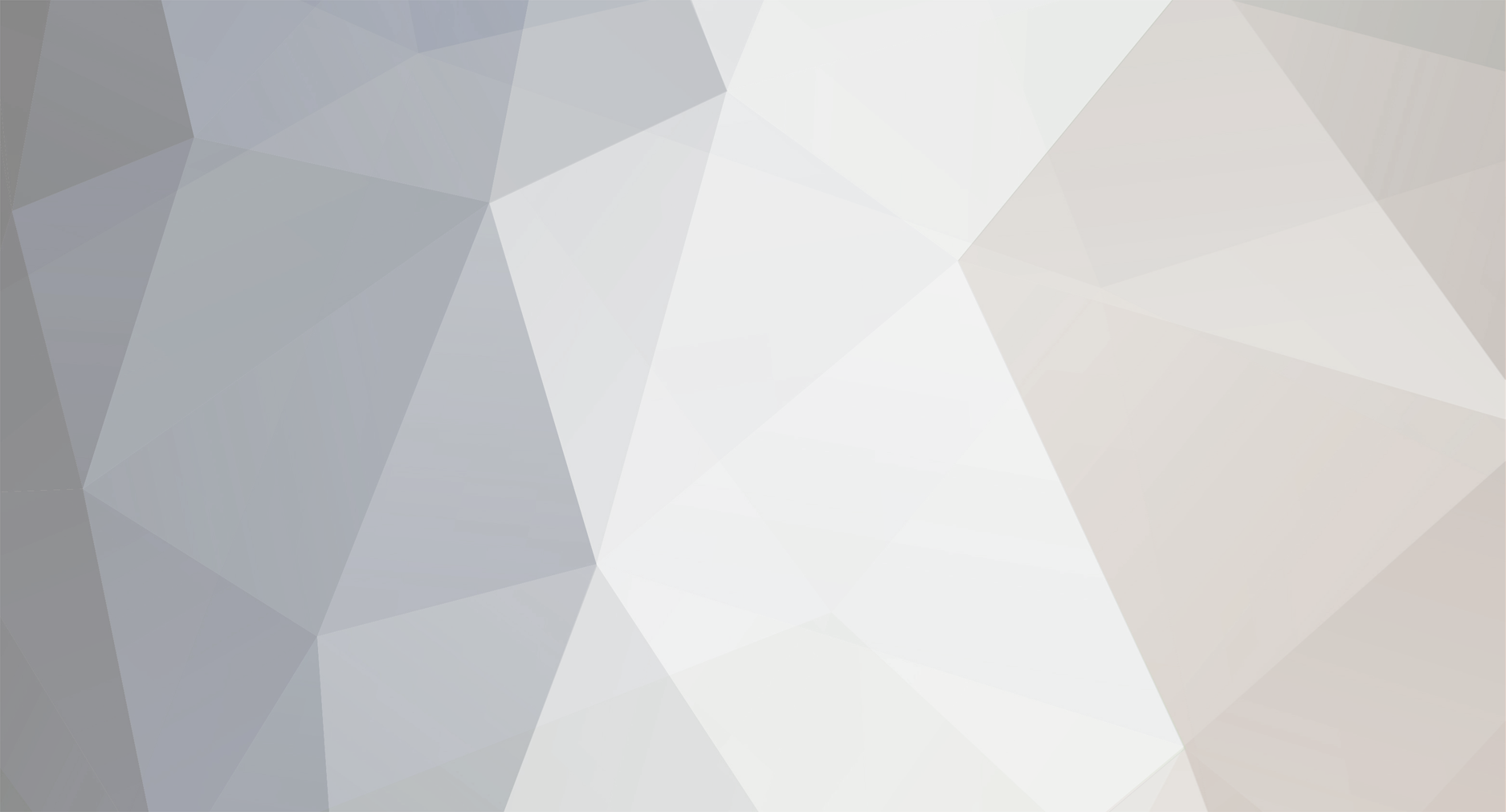
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
if ($_SERVER['REQUEST_METHOD'] === 'POST' && !empty($_POST)) { ..variables.. $query = 'INSERT INTO tabel (column(n), column(n+1), ..) VALUES ($_POST[n], $_POST[n+1], ..)'; mysql_query($query); }
-
Your query is wrong you'll get: SELECT * FROM objects WHERE zupanija = "obiteljske"{$val} ORDER BY id DESC{$val} ORDER BY id DESC{$val} ORDER BY id DESC{$val} ORDER BY id DESC while this should be: SELECT * FROM objects WHERE zupanija = "obiteljske" AND column(n)={$val(n)} AND column(n+1)={$val(n+1)} .. ORDER BY id DESC
-
This 5 lines of code do the same thing your 15 lines of code do <select name="gender"> <option>-</option> <option value="1"<?php $gender === 1 ? ' selected="selected"' : '' ?>>Male</option> <option value="2"<?php $gender === 2 ? ' selected="selected"' : '' ?>>Female</option> </select>
-
If you only have a category and a subcategory and nothing deeper then use: [categories](1)---(*)[subcategories] This has the advantage that no products can be coupled to a parent category
-
What is the format you are gettin' them in? And what is your local time?
-
do your subcategories have sub-subcategories? if not then you need to alter your db design to: [categories](1)---(*)[subcategories] if these subcategories can have subcategories then you need to add a column (parent_id) to your categories table to allow recursion +---------------+ | categories | +---------------+ | id INT | | parent_id INT | +---------------+ Now if you add a subcategory you set the id of the parent category in the parent_id INSERT INTO categories (id, parent_id) VALUES (1, 0); // parent category INSERT INTO categories (id, parent_id) VALUES (2, 1); // child category INSERT INTO categories (id, parent_id) VALUES (3, 2); // child-child category INSERT INTO categories (id, parent_id) VALUES (4, 3); // child-child-child category ..
-
Dutch: Waarom zijn alle belgen toch zo lui? $months = array(1 => 'January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'November', 'December'); $years = array(); $currentYear = (int) date('Y'); for ($i = $currentYear; $i > $currentYear - 100; --$i) { // sorry 100+ shouldn't surf the web $years[] = $i; }
-
You are referring to Ajax if you are using extjs.com script.js var jsonStore = new Ext.data.JsonStore({ autoLoad: true, autoSave: false, url: "ajax.db-info.php" }); // do something with jsonStore ajax.db-info.php $query = 'SELECT * FROM tabel'; $result = mysql_query($query); $rows = array(); if (mysql_num_rows($result)) { while ($row = mysql_fetch_assoc($result)) { $rows[] = $row; } } print json_encode($rows);
-
Read this: http://us2.php.net/manual/en/features.file-upload.post-method.php "i hate ajax": Do you even know what ajax is? And no, Ajax wouldn't be able to help you in determing the filesize as it would just like your form upload it to the server and then the server would evaluate it and return that size in JSON or some other format.
-
<?php class UserManager { protected $_simpleXml; public function __construct($dataSource { $this->_simpleXml = simplexml_load_file($dataSource); if (!isset($this->_simpleXml->user)) { throw new Exception('Incorrect data source given.'); } } public function addUser($details) { $this->_simpleXml->addChild('key', $details['key']); $this->_simpleXml->addChild('string', $details['string']); } public function deleteUser($username) { for ($i = 0; $i < $this->_simpleXml->user; $i++) { if ($this->_simpleXml->user[$i]->key === $username) { unset($this->_simpleXml->user[$i]); break; } } } public function __toString() { return $this->_simpleXml->asXml(); } } $userManager = new UserManager('/path/to/file.xml'); $userManager->deleteUser('User1'); print $userManager; ?>
-
<table> <tr> <th>Column Heading 1</th> <th>Column Heading 2</th> <th>Column Heading 3</th> <th>Column Heading 4</th> </tr> <?php if (mysql_num_rows($searchResult)) { while ($row = mysql_fetch_assoc($searchResult)) { print '<tr>'; print "\t<td>" . $row['column1'] . '</td>'; print "\t<td>" . $row['column2'] . '</td>'; print "\t<td>" . $row['column3'] . '</td>'; print "\t<td>" . $row['column4'] . '</td>'; print '</tr>'; } } else { print '<tr><td colspan="4">Search returned no results</td></tr>'; } ?> </table>
-
are you sure allow_url_fopen is on?
-
all sdk_*() functions are located under a directory you specify and is present in the include_path this works even in safe_mode or open_basedir
-
How To Check PC's Regional Settings With PHP?
ignace replied to Dan_Mason's topic in PHP Coding Help
<?php print $_SERVER['HTTP_ACCEPT_LANGUAGE']; ?> or consider my content negotiation class: http://pastebin.com/fee1ffde For more information google: http accept headers (http://en.wikipedia.org/wiki/List_of_HTTP_headers) -
Google
-
You have actually 2 options: 1) disable the function you do not want the user to use and create a counterpart which provides this functionality under controlled circumstances, for example: sdk_fopen(..) which would add additional control structures to verify the user is opening a file they are authorized to open 2) enable all functions and set open_basedir (http://be.php.net/manual/en/ini.sect.safe-mode.php#ini.open-basedir) or set safe_mode (http://be.php.net/manual/en/ini.sect.safe-mode.php#ini.safe-mode) to on if you only want to disable including remote files consider disabling allow_url_fopen
-
somebody told me to put in the formula "session_start();" session_start() is not a formula it's a function. "but here my knowledge ends." strange way of putting it.. Anyway upload your code so that we can investigate and provide a solution
-
Hi, Does anyone know of a javascript script which can parse php? I tried finding it via google but all turned up were javascript parser written in php. So that i can do: parser.isValid('<?php ..php code.. ?>'); Thanks
-
K found it! modified my variables_order to egpcs added APPLICATION_ENV to my system variables instead of user variables logged off, logged on worked
-
[SOLVED] Exclude a directory from being able to view
ignace replied to ignace's topic in Apache HTTP Server
w00t got it! <LocationMatch "(\$RECYCLE.BIN|System Volume Information)"> Order allow,deny Deny from all </LocationMatch> -
[SOLVED] Exclude a directory from being able to view
ignace replied to ignace's topic in Apache HTTP Server
didn't work, i also used this which didn't work either <DirectoryMatch "^\$"> Order allow,deny Deny from all Satisfy All </DirectoryMatch> -
Hi How can i exclude a directory from being viewed? When i go to my localhost i get an additional $RECYLE.BIN folder
-
Well i know it is possible because i've seen people do it, they added an environment variable to their os and then launched their script the environment variable was needed to modify the mode (production, development, staging or testing) in which the script was operating and/or it could/couldn't throw errors/exceptions. I was only dumb enough not to ask how they did it as i assumed that just adding it to your environment variable would suffice
-
And how am i supposed to call putenv() in htaccess or my os? I need a solution whereby i can add VARIABLE to my environment variables and that i can use getenv() to retrieve that value so far i haven't been able
-
Hi, I have created a .htaccess file with the following line: SetEnv VARIABLE value in my php script i do: define('VARIABLE', getenv('VARIABLE') ? getenv('VARIABLE') : 'default-value'); But this doesn't work it always gives me default-value. I also tried export VARIABLE=value which also doesn't work anyone know how i can get this working on both linux and windows?