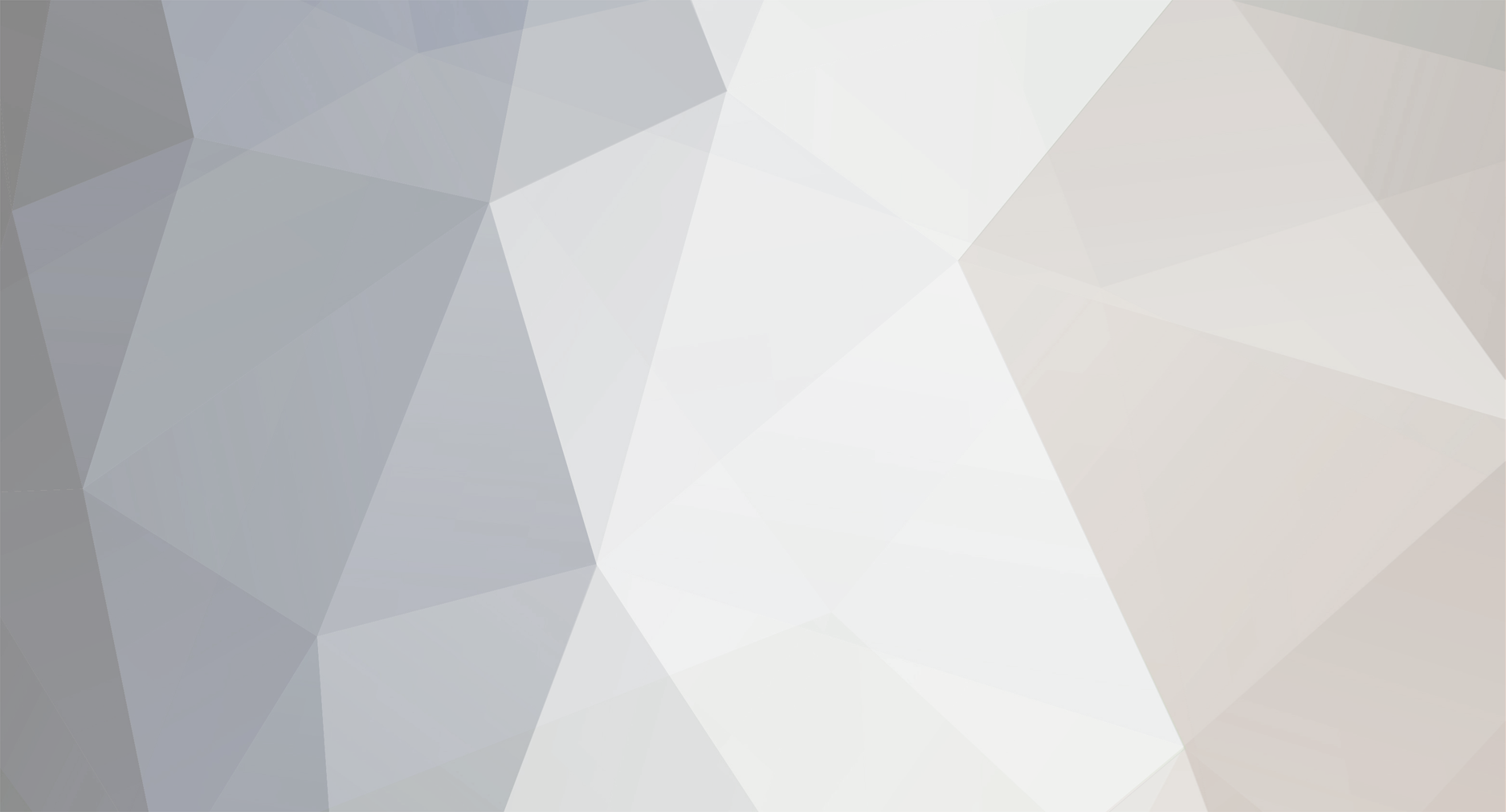
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
<?php $error = $_GET['error']; $errors = explode('+', $error); if (in_array('performance', $errors)) { ..performance is in_array.. } else { ..performance not in array.. } ?>
-
Random number generator on click which then emails number
ignace replied to danibee2k's topic in PHP Coding Help
yep that is no problem, not even in a database context -
Is it to use in php or just desktop? If in php i would take a look at the COM library: http://www.php.net/manual/en/book.com.php to retrieve what you want and: http://www.php.net/manual/en/book.pdf.php to create the pdf file
-
Copying a single file to multiple sub-directories
ignace replied to johnc71's topic in PHP Coding Help
You had a beautiful pseudo-code example, why not implement it? <?php $indexHtml = 'path/to/index/html/file'; $directoryListing = scandir('path/to/directory'); foreach ($directoryListing as $directory) { if (is_dir($directory) && is_writeable($directory)) { $directoryName = basename($directory); if (substr($directoryName, 0, 4) === 'demo') { copy($indexHtml, $directory .DIRECTORY_SEPARATOR. 'index.html'); } } } ?> -
Very nice of you but this is a php help forum which means you come here for help not to help those who are not seeking it
-
please make this topic solved
-
Random number generator on click which then emails number
ignace replied to danibee2k's topic in PHP Coding Help
More resources: http://be.php.net/manual/en/function.uniqid.php http://be.php.net/manual/en/function.mt-rand.php -
Random number generator on click which then emails number
ignace replied to danibee2k's topic in PHP Coding Help
how long must that unique number be? <?php $rand = sha1(time()); // 40-chars ?> -
I don't believe it's possible with php to only get a piece of an image however javascript can http://www.google.be/search?q=javascript+image+crop&ie=utf-8&oe=utf-8&aq=t&rls=org.mozilla:nl:official&client=firefox-a
-
Help Required! How To Open Each Record Using Php
ignace replied to niccki's topic in PHP Coding Help
You can do this in 2 ways the first would be using javascript and is probably the most recommended and the second way is by using php. The first way would be like this: <script type="text/javascript"> function showMore(id) { var el = document.getElementById(id); if (el.style.display == 'none') { el.style.display = 'block'; } else { el.style.display = 'none'; } } </script> <h1 onclick="showMore('a-unique-name')">Some Title</h1> <p style="display: none" id="a-unique-name">some text</p> The second would be like this: <?php $titleId = 0; if (isset($_GET['title_id'])) { $titleId = $_GET['title_id']; } ..query.. while ($title = mysql_fetch_assoc($queryResult)) { ..title.. if ($title['id'] === $titleId) { ..show description.. } } ?> although for accessibility reasons we should re-commend a mix of both -
Iterate over all the records and only modify the first column
-
check this topic: http://www.phpfreaks.com/forums/index.php/topic,249972.msg1171810.html#msg1171810
-
create an image in one php file, load it in another php file.
ignace replied to SirXaph's topic in PHP Coding Help
I think i know what the problem is your host doesn't allow you to change the ini settings nor does it display any errors apparently. Try this: Create a new php file with the following lines: echo 'first: ' . ini_get('memory_limit'); ini_set('memory_limit', '16MB'); echo 'after: ' . ini_get('memory_limit'); if your host doesn't allow you to change ini settings then these values won't change. -
That is because you need to change the password in the users table first so when they sign up their password is stored using md5 instead of password and when you check if the user exsits you perform the same algorithm. Btw you could have kept using password. insert the user into the database: INSERT INTO users SET username = '$username', password = PASSWORD('$password') then to check if the user exists: SELECT * FROM users WHERE username = '$username' AND password = PASSWORD('$password')
-
Would the connection remain open in this case?
ignace replied to adrianTNT's topic in PHP Coding Help
Sorry i didn't see you said pconnect Yes when you are using pconnect your connection remains open for future use. Which means that the connection will only be made once. So every new visitor will use the already existing open connection; You do not have to change your code or anything. pconnect will automatically checks if a connection already exists. If it exists, then it will use the already existing instead of creating a new connection. Persistent connections are used for performance but they may be a security problem. -
The last part should be: <?php else if (sizeof($_POST) && isset($_POST['answer'])) { ..validate.. ..verify answer.. // if a valid answer was supplied then remove it from the survey array_shift($_SESSION['survey']); if (sizeof($_SESSION['survey'])) { echo displaySurveyEntry($_SESSION['survey'][0]); } else { // all questions have been answered } } ?> Their is also a little bug in the code: <?php while ($answer = mysql_fetch_assoc($queryResult)) { $surveyEntry['answers'][] = $answer; } ?> should be: <?php while ($answer = mysql_fetch_assoc($_queryResult)) { $surveyEntry['answers'][] = $answer; } ?>
-
I rewrote a great deal of your code, this should do the trick in a more proper fashion. Read it and verify you understand the code. <?php session_start(); function htmlSelect($name, $options) { $htmlSelect = "<select name=\"$name\" id=\"$name\">\r\n"; foreach ($options as $option => $optionText) { $htmlSelect .= "\t<option value=\"$option\">$optionText</option>\r\n"; } return $htmlSelect . "</select>\r\n"; } function htmlForm($formBody, $action, $method = 'post', $enctype = 'application/x-www-form-urlencoded') { $htmlForm = "<form action=\"$action\" method=\"$method\" enctype=\"$enctype\">"; $htmlForm = $htmlForm . $formBody . '<input type="submit" name="submit" id="submit" value="Submit" />'; return $htmlForm . '</form>'; } function getCategory($category) { $categoryQuery = "SELECT * FROM categories WHERE cat_id = '$category'"; $queryResult = mysql_query($categoryQuery); return mysql_fetch_assoc($queryResult); } function displaySurveyEntry($surveyEntry) { echo $surveyEntry['question']['question']; print_r($surveyEntry['answers']); } /** * If no category is set display the form and wait for it to be submitted */ if (!(isset($_GET['cat']) && is_numeric($_GET['cat']))) { // ?cat=<number> should always be set or you will get the select form again $categoryQuery = "SELECT * FROM categories"; $queryResult = mysql_query($categoryQuery); $categories = array(); while ($category = mysql_fetch_assoc($queryResult)) { $categories[$category['id']] = $category['category']; } echo htmlForm(htmlSelect('cat', $categories), $PHP_SELF, 'get'); } /** * If the survey hasn't yet been created or a refresh has been ordered, then start creating/refreshing the survey. */ else if (!(isset($_SESSION['survey']) && sizeof($_SESSION['survey'])) || isset($_GET['refresh'])) { // ?refresh=1 to refresh the survey best to use as last $category = getCategory($_GET['cat']); $category = $category['id']; $questionQuery = "SELECT * FROM questions WHERE cat_id = '$category'"; $queryResult = mysql_query($questionQuery); $survey = array(); while ($question = mysql_fetch_assoc($queryResult)) { $surveyEntry =& $survey[]; $surveyEntry['question'] = $question; $questionId = $question['id']; $answerQuery = "SELECT * FROM answers WHERE quest_id = '$questionId'"; $_queryResult = mysql_query($answerQuery); while ($answer = mysql_fetch_assoc($queryResult)) { $surveyEntry['answers'][] = $answer; } } $_SESSION['survey'] = $survey; echo displaySurveyEntry($_SESSION['survey'][0]); } if (sizeof($_POST) && isset($_POST['answer'])) { ..validate.. ..verify answer.. // if a valid answer was supplied then remove it from the survey array_shift($_SESSION['survey']); echo displaySurveyEntry($_SESSION['survey'][0]); } ?>
-
You realize you have a serious whole in your application - about the size of 16 football fields? Try the following url: uploaded_files.php?username=../.. and see what it lists..
-
PHP thumbnail generator question (Use URL instead of filename?)
ignace replied to Skipjackrick's topic in PHP Coding Help
This should do the trick http://mediumexposure.com/techblog/smart-image-resizing-while-preserving-transparency-php-and-gd-library The used functions in this function are remote file aware. Another one which could be used is http://shiftingpixel.com/2008/03/03/smart-image-resizer/ -
You never declared $newpass. You use it for the first time in your insert query $newpass doesn't exist prior to that event thus evaluating to null or an empty string.
-
Yep your right you can change this behavior by changing the lifetime of the cookie which holds the session identifier http://be2.php.net/manual/en/function.session-set-cookie-params.php This script should be placed in your index.php or some script that is always included so that you get logged in on every page. Create an additional function called is_logged() to verify a user has been logged in. <?php if (is_logged()) { ..logged in logic.. } else { ..not logged logic.. } ?> if you are include'ing a page in every php file then know that you only want to log a user when he is not logged in: <?php if (!is_logged()) { if (isset($_SESSION['username']) && isset($_SESSION['password'])) { logon($_SESSION['username'], $_SESSION['password']); } } ?>
-
1) Never EVER store credential information inside a cookie!!!! These are stored on the client side and it is very easy to read, modify and even create them. So if you only rely on authentication and no authorization then i could pretend to be you, full power over the system and able to destroy everything to which you have power to. 2) To create remember me script you need to store account information inside the session so when a user visits your website you check if the session information is available, retrieve it and perform the authentication process just like the user would have by using the authentication form. Something like: <?php session_start(); if (isset($_SESSION['username']) && isset($_SESSION['password'])) { logon($_SESSION['username'], $_SESSION['password']); } else { header('Location: /logon.php'); } ?>
-
Would the connection remain open in this case?
ignace replied to adrianTNT's topic in PHP Coding Help
No php automatically does what is called garbage collection when the end of the script has been reached -
You can not do this directly, you need to go by a script on your remote server who retrieves that specific piece of information. On your remote server you put this file: <?php print disk_total_space('/'); ?> On your server you then do: <?php $diskspace = file_get_contents('http://domain/path/to/file/php', 'r'); // assuming your server allows to read remote files echo $diskspace; ?>