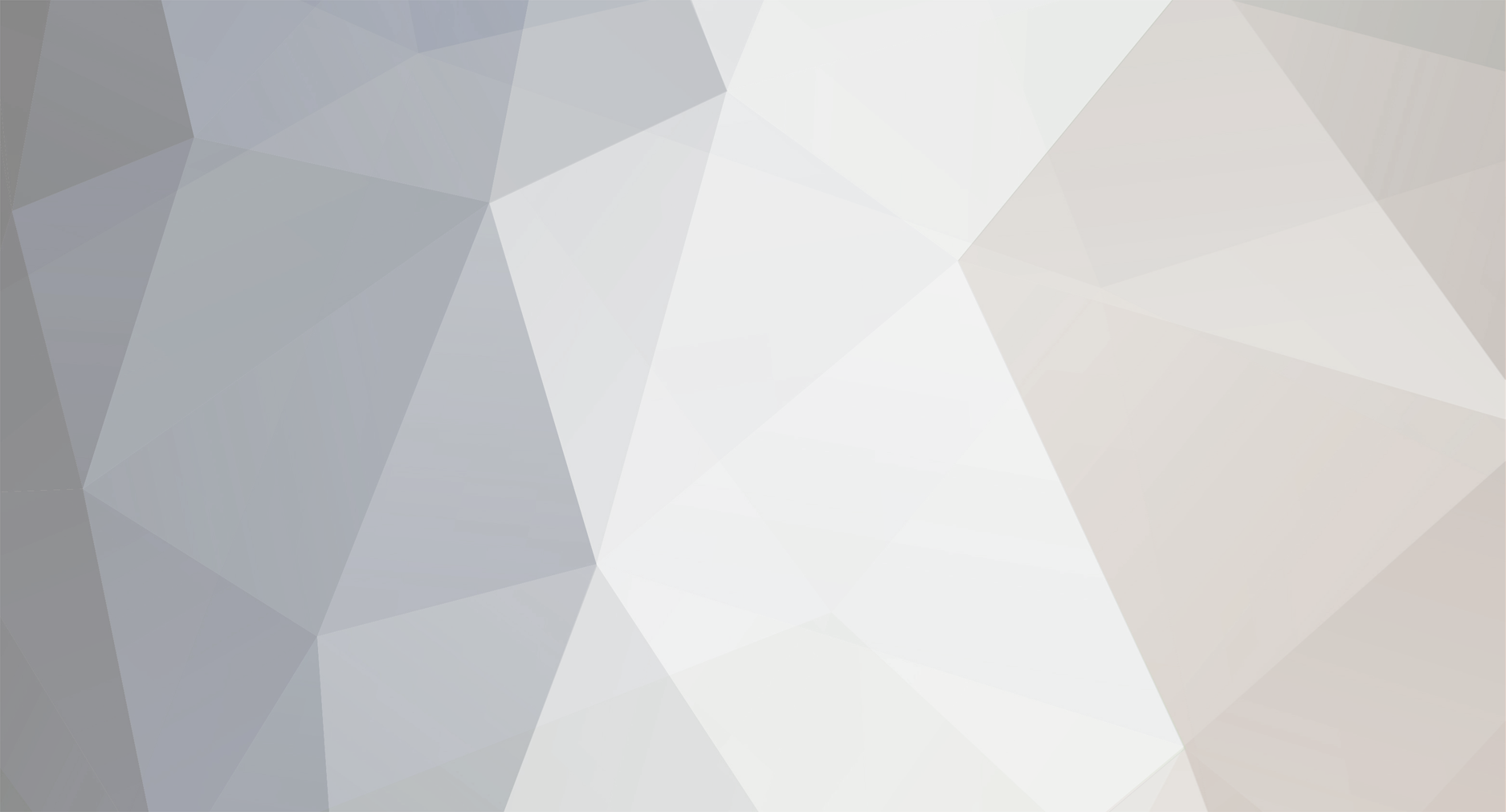
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Yes these are mere examples. I'm not the one they hired to write the code for them.
-
defined(!DB_USERNAME) is the same as defined(0) I think you mean !defined('DB_USERNAME') (mind the ') $result = $this->database->db_query("SELECT * FROM users WHERE id={$id}"); $final = mysqli_fetch_array($result); You either fully abstract your database or you don't, not trying to use both or you'll still end up screwed. The same goes for: __construct(MySqlDatabase $database) In OO we have a rule: program to an interface, not an implementation. Thus something like: interface DatabaseInterface { function connect($user, $pass, $host = 'localhost'); function disconnect(); function isConnected(); function prepare($sql); function execute($sql = null, $params = array()); function escape($value); } class MySQLDatabase implements DatabaseInterface { // implement the above methods } class UserGateway { private $db = null; function __construct(DatabaseInterface $db) { $this->db = $db; } function findById($id) { return new User( $this->db->select()->from('users')->where('id', $id); ); } function fetchAll($count = null, $offset = null) { if($count != null && $offset != null) { return new UserList( $this->db->select()->from('users')->limit($count, $offset); ); } else if($count != null) { return new UserList( $this->db->select()->from('users')->limit($count); ); } else { return new UserList( $this->db->select()->from('users'); ); } } }
-
On the profile page you would call: $user = $gateway->findUserById(1);
-
class CertifiedAds { function getAd($id = null) { return $id === null ? new AdList($this->db->fetchAll('SELECT * FROM ads')) : new AdList($this->db->fetchRow('SELECT * FROM ads WHERE id = ..')); } }
-
I hope Database.php contains a Database class? If so, you write: class UserGateway { private $db = null; function __construct(Database $db) { $this->db = $db; } function getAllUsers() { return new UserList($this->db->fetchAll('SELECT * FROM users')); } function findUserById($id) { return new User($this->db->fetchRow('SELECT * FROM users WHERE id = ..')); } function findUsersByLastName($lastName) { return new UserList(..); } }
-
Sexy Halloween Costumes and Lingerie Website
ignace replied to Colton.Wagner's topic in Website Critique
The website looks great only too bad it doesn't work with JS disabled. -
You used a global to pass an object while you should use the constructor or a setMethod() (just don't over-use the latter) class A { function sayHello($b) { echo 'Hello, ', get_class($b), '. How are you doing today?'; } } class B { function __construct(A $a) { $this->a = $a; } function greetings() { $this->a->sayHello($b); $this->sayHello($this->a); } function sayHello($a) { echo 'Fine, thanks for asking. And how are you doing on this sunny day ', get_class($a), '?'; } } $a = new A(); $b = new B($a); $b->greetings(); You could also pass $a on greetings() making it Lazy-Loaded
-
Are you suggesting, he needs more private methods? Ever heard of a thing called Unit-Testing and the problem with private methods? Now you may think that you need MySQL for everything but if whatever you are building becomes a success you'll find your design is too inflexible. Let the DB handle DB specific stuff (connect(), disconnect(), prepare(), execute(), ..) and create Gateway classes that will hold an instance of your DB and let your application communicate with the Gateway this way you decouple your application from your DB, as you can modify the Gateway classes without having to touch your application code. class UserGateway { private $db = null; function getAllUsers() { return new UserList($this->db->fetchAll('SELECT * FROM users')); } function findUserById($id) { return new User($this->db->fetchRow('SELECT * FROM users WHERE id = ..')); } function findUsersByLastName($lastName) { return new UserList(..); } } Also a good idea is to not return array() or int or stuff like that but instead return domain-specific classes the reason being that you can't modify the internal structure of array() or int but you can with domain-related classes.
-
Remember that the software you write will not always be maintained by you. So whenever a new developer joins your team or someone takes over you maintenance work they won't have any documentation to refer to.
-
The error message is self explanatory the log-in details are wrong! Show us a screen of you being logged into PHPMyAdmin this will give us more information to what the problem is.
-
100.000.000 I doubt you would want to insert all these records yourself, so a better approach would be: 1) define the boundaries of the game (can be any high number) 2) players are assigned a x- and y-coordinate (which needs to be unique and within the game boundaries for obvious reasons) 3) build the map: load any player-specific tiles for those present in the area and randomly generate tiles for all non-occupied tiles
-
query from a one database and insert in another database
ignace replied to fer0an's topic in PHP Coding Help
This script is incomplete and needs tweaking (hostname, user, pass, error descriptions, ..). It uses the SELECT .. INTO OUTFILE and LOAD DATA INFILE SQL commands. try { // database server #1 $db1 = db('db', 'pass', 'user', 'server1'); $outfile = dirname(__FILE__) . DIRECTORY_SEPARATOR . 'outfile.txt'; if(!mysql_query("SELECT * FROM table INTO OUTFILE '$outfile'")) throw new Exception(''); if(!file_exists($outfile) || filesize($outfile) == 0) throw new Exception(''); // database server #2 $db2 = db('db', 'pass', 'user', 'server2'); if(!mysql_query("LOAD DATA INFILE '$outfile' REPLACE INTO TABLE table")) throw new Exception(''); } catch(Exception $e) { echo $e->getMessage(); } function db($database, $pass, $user = 'root', $host = 'localhost') { $db = mysql_connect($host, $user, $pass); if($db == FALSE) throw new Exception(''); if(!mysql_select_db($database, $db)) throw new Exception(''); return $db; } -
Read MySQL database, write out each record to Txt file?
ignace replied to FredFogg's topic in PHP Coding Help
-
SELECT * FROM Manufacturer WHERE ManufacturerId = (SELECT ManufacturerId FROM Product WHERE someUniqueColumn = 'someUniqueValue') Euh... Why need the sub-query? SELECT * FROM manufacturer JOIN product ON manufacturer.id = product.manufacturer_id WHERE product.some_unique_value = 'some_unique_value';
-
Read MySQL database, write out each record to Txt file?
ignace replied to FredFogg's topic in PHP Coding Help
Too far fetched to me. If he wants to print it out then simply show him a [print] button that reads out your records and put's it in a table (repeat headers every x records) and implement a print-media stylesheet. <link href="print.css" type="text/css" media="print" rel="stylesheet"> -
Completely unnecessary: define('OBJECT','mysql_fetch_object'); define('ASSOC','mysql_fetch_assoc'); And can be re-written to: class Database { const FETCH_OBJECT = 'object'; const FETCH_ASSOC = 'assoc'; const FETCH_BOTH = 'both'; } Also completely useless is: define('DB_HOST','localhost'); define('DB_DB','mysite'); define('DB_USER','root'); define('DB_PASS',''); Not everyone uses constants, some like to use configuration files, environment variables, .. Your class although named db is mysql-specific, if I would want to shift from mysql to sqlite for example I would have to re-write most (if not all) of your methods. Plus in some cases (like my last project) I had to use mysql as my main database which was kept in sync with a program that ran the back-office and SQLite as a buffer to hold the most frequent executed queries to lower the load on the main mysql database.
-
reg.php also needs session_start() at the top.
-
Zend Framework removing default controller and action from url
ignace replied to lysitheas's topic in Frameworks
Add a custom route $router->addRoute( 'someroute', new Zend_Controller_Router_Route('lang/:lang/content/:content', array('controller' => 'index', 'action' => 'index')) ); $this->url(array('lang' => 'EN', 'content' => 5), 'someroute'); -
calling multiple functions in single call from class
ignace replied to ngreenwood6's topic in PHP Coding Help
We had actually could of have helped you better if you instead of Test had put up a real example of your code. I would create a Façade (or a Builder if you are actually building something in steps) if those methods needs to be called in sequence. Because you are familiar with those functions and how they are called, you can't assume someone else will if they join your project. -
if(logged_in() && $post['auhtor_id'] == $user['id'])
-
Prolly, I had a chat 2 or 3 weeks back where I suggested HTML5 as a solution to his problem and replied "HTML5?! No one uses that anymore." Yup some really believe XHTML is the future
-
Ouch, that was harsh!
-
class SomeClass { function __call($method, $args) { if(method_exists($this, $method)) return $this->$method(); return NULL; } function call($method, $args) { return $this->__call($method, $args); }} $class = new SomeClass();$output = $class->call($_GET['action']);