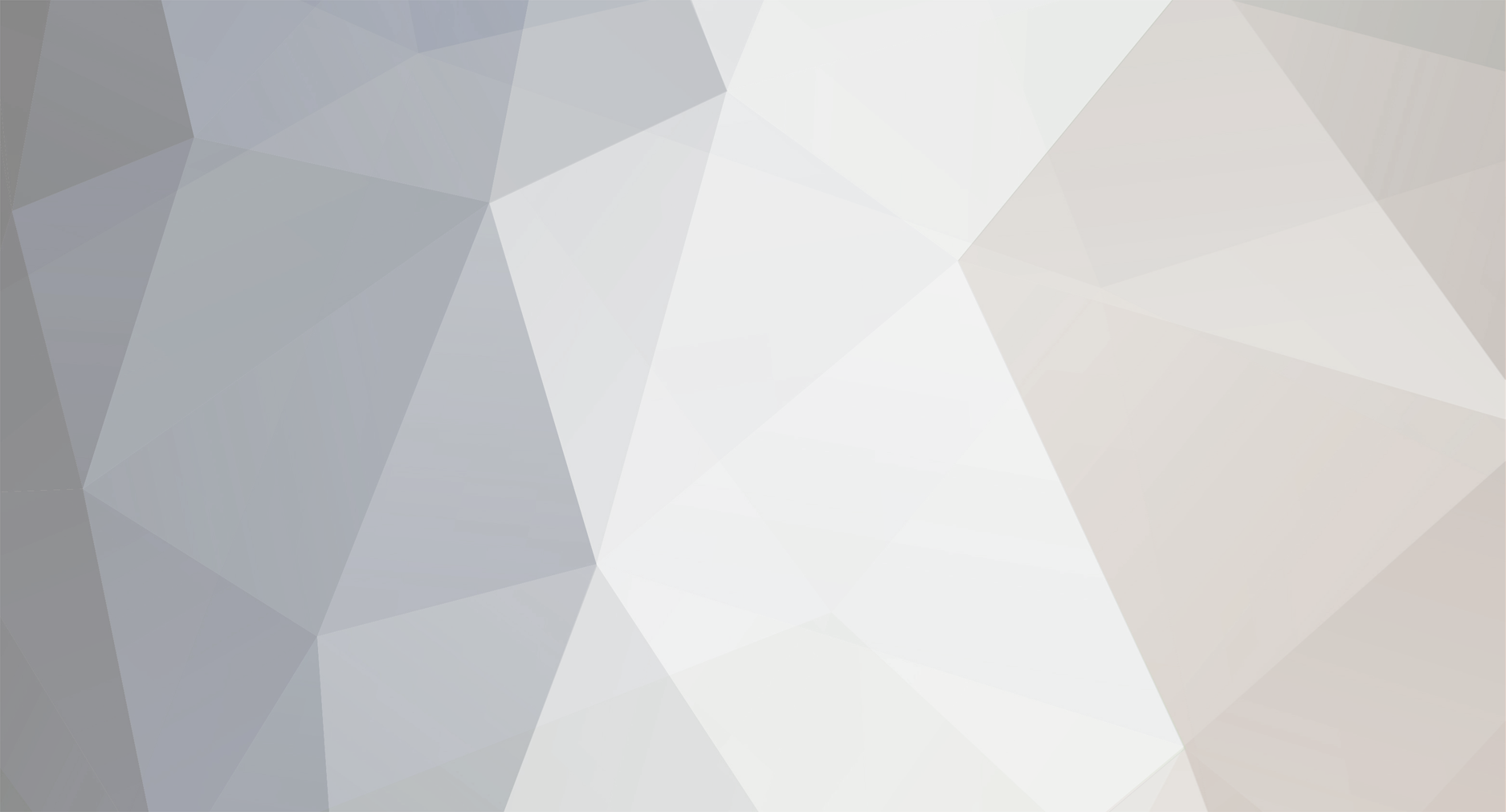
sasa
-
Posts
2,804 -
Joined
-
Last visited
-
Days Won
1
Posts posted by sasa
-
-
for($i= 'a'; $i <'zz'; $i++) echo $i;
not tested
-
or
<?php $ltrs = "ABCDEFGHJKLMPQRS"; $ltrs = str_split($ltrs); shuffle($ltrs); for($i=0; $i<3; $i+=2) $str .= $ltrs[$i]<$ltrs[$i+1] ? $ltrs[$i].$ltrs[$i+1] : $ltrs[$i+1].$ltrs[$i]; echo $str; ?>
-
1.
<?php $class_name = 'my_class'; $html = '<a id="23" href="sa\'sa.com" class="yyy" bla bla>xx</a>'; $pattern1 = '/(<a [^>]*) class=([\'"])[^\2]+\2([^>]*>)/is'; $replace1 = '\1\3'; $pattern2 = '/(<a [^>]*href=([\'"])[^\2]+\2)([^>]*)/is'; $replace2 = '\1 class="'. $class_name .'"\3'; $html = preg_replace($pattern1, $replace1, $html); echo $html = preg_replace($pattern2, $replace2, $html); ?>
-
try
$pattern = '@(body[^\{\}]*?\{[^\{\}]*?background:)#([0-9A-F]{6})(;[^\{\}]*?\})@s'; echo $new_str_1 = preg_replace($pattern,"$1#".$newcolor."$3",$str);
in your example replace string is "$11A2B3C$3"
start of it $11 means 11th subpatern that not exist
i remove # from 1st subpatern and add it literaly
-
try
<?php $test = Array ( 0 => Array ( 'row_color' => 'sectiontableentry1', 'product_name' => 'Standaard Roll-up banner - 85 x 205 cm Standaard: Roll-up banner (= €117,81)', 'product_attributes' => '', 'product_sku' => '00001', 'product_availability' => '', 'product_price' => '€117,81', 'quantity' => 1, 'subtotal' => '€117,81', 'update_form' => '', 'delete_form' => ''), 1 => Array ( 'row_color' => 'sectiontableentry2', 'product_name' => 'Test2 Losse: spandoek_UV-Print', 'product_attributes' =>'', 'product_sku' => '10001', 'product_availability' =>'', 'product_price' => '€258,83', 'quantity' => 1, 'subtotal' => '€258,83', 'update_form' =>'', 'delete_form' =>''), 2 => Array ( 'row_color' => 'sectiontableentry1', 'product_name' => 'Luxe Roll-up banner - 85 x 205 cm Luxe: Roll-up banner (= €213,01)', 'product_attributes' =>'', 'product_sku' => '00002', 'product_availability' =>'', 'product_price' => '€213,01', 'quantity' => 1, 'subtotal' => '€213,01', 'update_form' => '', 'delete_form' => '')); function my_find_in_sku($array, $srch){ foreach ($array as $v) if($v['product_sku'] == $srch) return true; return false; } if(my_find_in_sku($test, '10001')){ echo 'find it'; } else { echo 'not'; } ?>
-
<?php $page ="<input type=\"hidden\" autocomplete=\"off\" name=\"xhpc_composerid\" value=\"u269995_3\" \/>"; preg_match('|<input\s[^>]*name="xhpc_composerid"\s[^>]*value="([^"]*)"[^>]*>|', $page, $match); print_r($match); ?>
-
try
<?php // connect to the database $dbLink = mysql_connect("Blah", "Blah", "blah"); if (!$dbLink) { print "Could not connect: ".mysql_error(); exit(0); } if (!mysql_select_db("answerchoice", $dbLink)) { print "Could not select database: ".mysql_error(); exit(0); } $query= "SELECT AID FROM answer WHERE (QID = 18) AND UID IN (SELECT UID FROM answer WHERE Answer = 33) ORDER BY AID ASC"; $result = mysql_query($query, $dbLink); //this query returns data base answer choice numbers (commas added by me for readability) 52,52,52,52,52,52,52,52,54,54,55,55,55,56,56,56 $i = 0; $tmp = -1; $ans = array(); while($row = mysql_fetch_array($result)){ if($row['AID'] > $tmp){ $i++; $tmp = $row['AID']; $ans[$i]=0; } $ans[$i]++; //$i now holds the answer numbers converted into a 1-4 value (commas added by me for readability)1,1,1,1,1,1,1,1,2,2,3,3,3,4,4,4 I would like to average this string which would be 2.0265 } foreach ($ans as $v => $c){ $tot += $v * $c; $cnt += $c; } if($cnt ) $ave = $tot / $cnt; else $ave = 0; echo 'average = ',$ave; ?>
-
you have same fun qoutes in the begin of string
change “ to "
-
change $GET to$_GET in line 469
-
remove single qvotes around the filename in your query
$transfer = mysql_query("UPDATE members SET balance = '$newblanceto' WHERE bankaccount='$to_acct'") or die ("could not write to profile");
-
use implode() function
-
<?php $i = 1; foreach ($_POST['chk_b'] as $index => $option) { echo $option, '<br>'; $fields[$i] = "`EXP$i`"; $value[$i] = "'$option'"; $i++; } //echo $sql = "INSERT INTO table_name (". implode(', ', $fields). ") VALUES (". implode(', ', $value). ")"; ?>
-
change chkbox name and add value attribute
$tmp[] = '<li id="li' . $i . 'b"><label for="chk' . $i . 'b"><input name="chk_b[' . $i . ']" id="chk' . $i . 'b" type="checkbox" value ="'.$option.'"onchange="Enable(\'chk' . $i . 'b\',\'li' . $i . 'b\')">' . $option . '</label></li>';
and on submit page do
foreach $_POST['chk_b'] as $index => $option) echo $index, ' -> ', $option, '<br />\n';
-
number $s is overflow integers (try to echo it)
to fix it use modulo operator in calculating $s
<?php for ($i = 2; $i < 10; $i++) { // testing from (2^2 - 1) to (2^10 - 1) $s = 4; $m = pow(2, $i) - 1; // define M if ($i > 2) { // Start computing $s only if ($i - 2) > 0, otherwise $s remains equal to 4. for ($j = 1; $j <= $i - 2; $j++) { // Find S(p-2) $s = (($s * $s) - 2) % $m; } } if ($s == 0) { echo "<br />$m"; } // Print out detected prime number. } ?>
-
for e-mail
$pattern = '/From:.*?([A-Za-z0-9!#$%&\'*+-\/=?^_`{|}~\.]+@[A-Za-z0-9!#$%&\'*+-\/=?^_`{|}~\.]+)/';
-
add space after +1
change +1. to +1 .
-
$_GET['submit'] is nut array
try
print_r ($_GET); // test display form values foreach ($_GET as $key => $value) { echo '<br/>'.$key.'<br/> '; if($key != 'submit'){ foreach ($value as $iKey => $iValue) { // Line 72 foreach ($iValue as $xKey => $xValue) { echo " The $xKey for Player $iValue is $xValue <br/>"; } } } }
-
change
echo "<input type='checkbox' name='option".$i."' value='$objectname' checked>".$objectname;
to
echo "<input type='checkbox' name='option[".$i."]' value='$objectname' checked='checked'>".$objectname;
and in $_GET['option'] is array of checked object
-
i wrote just pseudo code in pascal notation
php code looks like
$trials = 2; // Number of trials $upgradeChance = 0.5; // Original percent rate of success 50% $rateSuccess = 0; // Total rate of success is 3 of 4 $rateSuccess = 1 - pow((1 - $upgradeChance), $trials) ; echo "Chance of Success: <b>".$rateSuccess * 100 ."%</b><br>";
-
try to use mysql function STR_TO_DATE()
http://dev.mysql.com/doc/refman/5.0/en/date-and-time-functions.html#function_str-to-date
-
chance_for_n_tries = 1 - (1 - chance)^n
for example chance is
1-(1-0.0096)^100
-
your query is wrong
try something like
$query = "SELECT * FROM users WHERE username = '$user_username' AND `password` = '$user_password'";
-
<? if ($_SESSION['memberid']!= 1000) include("themes/".$setts['default_theme']."/includes/side_nav/inc_side_nav_members_menu_tree.php"); ?> <? if ($_SESSION['memberid']== 1000) { ?> <? include("themes/".$setts['default_theme']."/includes/side_nav/inc_side_nav_members_menu_tree2.php"); ?>
-
you must escape . (dot) too
/^[1-9]?((\.|,|\'|\s)([0-9]{1,2}))$/
Adding class to links and finding links
in Regex Help
Posted
change $pattern2 to