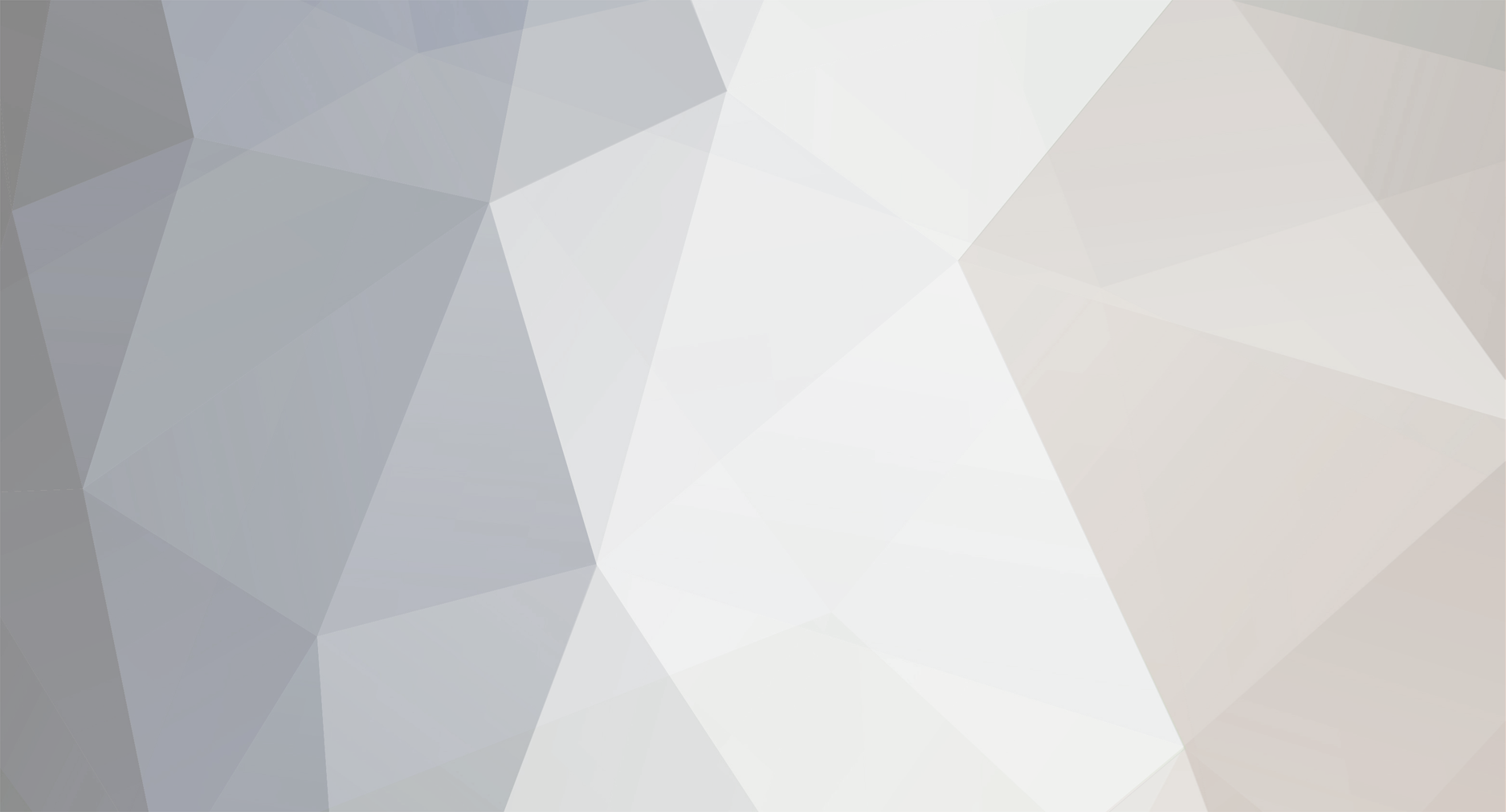
sasa
-
Posts
2,804 -
Joined
-
Last visited
-
Days Won
1
Posts posted by sasa
-
-
try to trim array elements before use it
<?php $words_text = 'badwords.txt'; $bad_words = file($words_text); foreach($bad_words as $k => $v) $bad_words[$k] = trim($v); $good_words = str_ireplace($bad_words,'[CENSORED]',$review); echo $good_words; ?>
-
in your script you didn't define names of input fields
-
<?php $name = trim($_POST['firstname']); //$name = 'h e l l o'; if(preg_match('/^([A-Z\'.-]\s?){2,}$/i', $name) and strlen($name) <= 20) echo 'ok';else echo 'no'; ?>
-
UPDATE `table` SET field2= '1' WHERE field1 IN ('1', '2', '3', '4', '5')
-
<?php $test = 'And today date 2011 03 28 and also some other text and this could be second line of7 2 6 0 and continue like this... some other number like 8 0 6 9 and some other as well. but it will not add dot to this single digit 01 or even to this word 2000 as those are single // there could be more this type of digits '; function my_to_dot($a){ return trim(preg_replace('/\s+/', '.', $a[0]),'.'). ' '; } echo preg_replace_callback('/(\d+\s+){2,}/', 'my_to_dot', $test); ?>
-
<?php $test = 'And today date 2011 03 28 and also some other text and this could be second line of7 2 6 0 and continue like this... some other number like 8 0 6 9 and some other as well. but it will not add dot to this single digit 01 or even to this word 2000 as those are single // there could be more this type of digits '; function my_to_dot($a){ return preg_replace('/\s+/', '.', $a[0]); } echo preg_replace_callback('/(\d+\s+){2,}/', 'my_to_dot', $test); ?>
-
<?php $test = '. http://www.example.com/testFolder/test.php'; preg_match('/http\:\/\/www\.([a-zA-Z0-9\-\.]+\/)+/', $test, $matches); echo $matches[0]; ?>
-
try
<?php $arr = array(); for ($i = 0; $i < 10; $i++) { $id = mt_rand() + $i; $arr[$id] = "some value $i"; } echo '<pre>', print_r($arr), "</pre>\n"; $id = array_rand($arr); echo "Srch element with key $id and value {$arr[$id]}<hr />\n"; function getValue($id, $arr) { end($arr); if ($id == key($arr)) $is_last = true; reset($arr); if ($id == key($arr)) $is_first = true; while (key($arr) != $id) next($arr); if ($is_last) echo "No next elemenrt.<br />\n"; else { next($arr); echo "Next element with key ", key($arr), " is '", current($arr), "'<br />\n"; prev($arr); } if($is_first) echo "No prev element"; prev($arr); echo "Prev element with key ", key($arr), " is '", current($arr), "'<br />\n"; } getValue($id, $arr); ?>
-
look next() function http://php.net/manual/en/function.next.php
-
look
<?php $sql = 'SELECT partyname, linkid FROM parties ORDER BY partyname'; $res = mysql_query($sql); $out = array(); while ($row = mysql_fetch_array($res)) { $out[$row['linkid']][] = $row['partyname']; } foreach ($out as $link => $parties){ if(count($parties) == 1 ){ echo "The party linked to $link is ", $parties[0], '.'; } else { $last = array_pop($parties); echo "The parties linked to $link are ", implode(', ', $parties), " and $last."; } } ?>
-
change
$num_rows=mysql_num_rows($result);
to
$num_rows=1;
and
<td><span class="style10"><?php echo $num_rows ?></span></td>
to
<td><span class="style10"><?php echo $num_rows++; ?></span></td>
-
<?php $color = 'white'; //before your while loop $x = 0; //not need $testing = 'the quick brown fox jumped over the lazy dog';//not need while ($x < 10) { echo "<span style='color:$color'>$testing</span><br>"; //inside your while loop //add style='background-color:$color;' // to <tr> tag $color = ($color == 'white')?'grey':'white';//inside while loop $x++;//not need } ?>
-
is it what you look for
<?php echo '<table border="3">'; for($row = 1; $row < 5; $row++){ $direction = 1 - ($row % 2) * 2; $staart = ($row % 2) * 49 + 1; echo "<tr>\n"; for($col = 0; $col < 3; $col++){ echo "\t<td>"; for($i = 0; $i < 10; $i++) { echo $staart, ' '; $staart+=$direction; } echo "</td>\n"; } echo "</tr>\n"; } echo '</table>'; ?>
-
are you try
<?php echo '<table border="3">'; for($row = 4; $row >= 0; $row--){ echo '<tr>'; for( $col = 10; $col >0; $col--) echo '<td>', $row * 10 + $col, '</td>'; echo "</tr>\n"; } echo '</table>'; ?>
-
i try this code
<?php $html = 'bla bl bla<div id="ST12345678_st" class="r_right_col"> <div class=""></div> <div id=""><span class=""></span></div> <div class=""></div> <div class=""><span class=""></span></div> <div class=""></div> <div class=""> <div style="" id="" fname=Abhishek lname=\'madhani\' division=8C house=\'Anything\'> </div> </div> </div>bla bla bla bl bla<div id="ST22345678_st" class="r_right_col"> <div class=""></div> <div id=""><span class=""></span></div> <div class=""></div> <div class=""><span class=""></span></div> <div class=""></div> <div class=""> <div style="" id="" fname=Abhishek lname=\'madhani\' division=8C house=\'Anything\'> </div> </div> </div>bla bla'; preg_match_all('/<div id="ST.*?house=\'Anything\'>\s*<\/div>\s*<\/div>\s*<\/div>/is', $html, $match); print_r($match); ?>
and it output this
X-Powered-By: PHP/5.2.0 Content-type: text/html Array ( [0] => Array ( [0] => <div id="ST12345678_st" class="r_right_col"> <div class=""></div> <div id=""><span class=""></span></div> <div class=""></div> <div class=""><span class=""></span></div> <div class=""></div> <div class=""> <div style="" id="" fname=Abhishek lname='madhani' division=8C house='Anything'> </div> </div> </div> [1] => <div id="ST22345678_st" class="r_right_col"> <div class=""></div> <div id=""><span class=""></span></div> <div class=""></div> <div class=""><span class=""></span></div> <div class=""></div> <div class=""> <div style="" id="" fname=Abhishek lname='madhani' division=8C house='Anything'> </div> </div> </div> ) )
-
preg_match_all('/<div id="ST.*?house=\'Anything\'>\s*<\/div>\s*<\/div>\s*<\/div>/is', $html, $match); print_r($match);
-
you don't close 1st else block
add } to the end of the script
-
SELECT * FROM $table WHERE (ump1 = '$umps' OR ump2 = '$umps' OR ump3 = '$umps' OR ump4 = '$umps' OR ump5 = '$umps') AND (visitor = '$town' OR home = '$town') AND sport = 'Baseball' AND `date` >= $start_date AND `date` <= $end_date
you must put strimg values in qutes and field name date in ``
-
try
<?php require '/home/srob/public_html/u-s-s-a.org/config.php'; $connect = mysql_connect($dbhost, $dbuser, $dbpass) or die('Cannot connect: ' . mysql_error()); mysql_select_db($dbname); $query = "SELECT * FROM directory ORDER BY state, flockname"; //add state in order by $result = mysql_query($query) or die('Error, query failed.'); echo "<tr><td width='33%' class='style9'><p align='left' class='style12'><a name='AR'></a>Arkansas</p></td><td width='34%'> </td><td width='33%' valign='top'><div align='right'><a href='#TOP'><img src='returnTOTOPGRAPHIC/RETURNTOTOP.gif' width='113' height='21' border='0' align='absmiddle'></a></div></td></tr>"; $cnt = 0; $state = ''; //declare state to empty while ($row = mysql_fetch_array($result, MYSQL_ASSOC)) { if ($row['state'] != $state) { //check if is new state $state = $row['state']; if ($cnt > 0) echo '</tr>'; //check if open table row $cnt = 0; // set $cnt to zero and echo table row for new state echo ' <tr> <td width="33%" class="style9"><p align="left" class="style12">' . $state . '</p> <p> </p></td> <td width="34%"> </td> <td width="33%" valign="top"><div align="right"><a href="#TOP"><img src="returnTOTOPGRAPHIC/RETURNTOTOP.gif" width="113" height="21" border="0" align="absmiddle"></a></div></td> </tr>'; } if ($cnt == 0) echo '<tr>'; echo "<td valign='top' class='style9'><p align='center'><span class='style10'><strong>"; if ($row['flockname']) echo $row['flockname']; echo "</strong></span><strong></br>"; if ($row['firstname']) echo $row['firstname'] . " " . $row['lastname'] . "</br>"; if ($row['address1']) echo $row['address1'] . "</br>"; if ($row['address2']) echo $row['address2'] . "</br>"; if ($row['city']) echo $row['city'] . ", " . $row['state'] . " " . $row['zip'] . "</br>"; if ($row['email1']) echo "<a href='mailto:" . $row['email1'] . "'>" . $row['email1'] . "</a></br>"; if ($row['email2']) echo "<a href='mailto:" . $row['email2'] . "'>" . $row['email2'] . "</a></br>"; if ($row['website1']) echo "<a target='_blank' href='http://" . $row['website1'] . "'>" . $row['website1'] . "</a></br>"; if ($row['website2']) echo "<a target='_blank' href='http://" . $row['website2'] . "'>" . $row['website2'] . "</a></br>"; echo "</br></p></td>"; $cnt++; if ($cnt == 3) { $cnt = 0; echo '</tr>'; } } mysql_close($connect); ?>
-
are you start session before use it?
-
preg_match is easiest solution
-
change
... $dynamicList = '<table width="100%" ...
to
... $dynamicList .= '<table width="100%" ...
-
ups my misteke
use
SELECT A.* FROM notifications A WHERE A.user_id='$session' AND A.from_id!='$session' ORDER BY A.state ASC, A.id DESC LIMIT 7
not '...ORDER BY B.state ASC ...'
-
SELECT A.* FROM notifications A WHERE A.user_id='$session' AND A.from_id!='$session' ORDER BY B.state ASC, A.id DESC LIMIT 7
Parsing HTML to strip required data
in PHP Coding Help
Posted
try