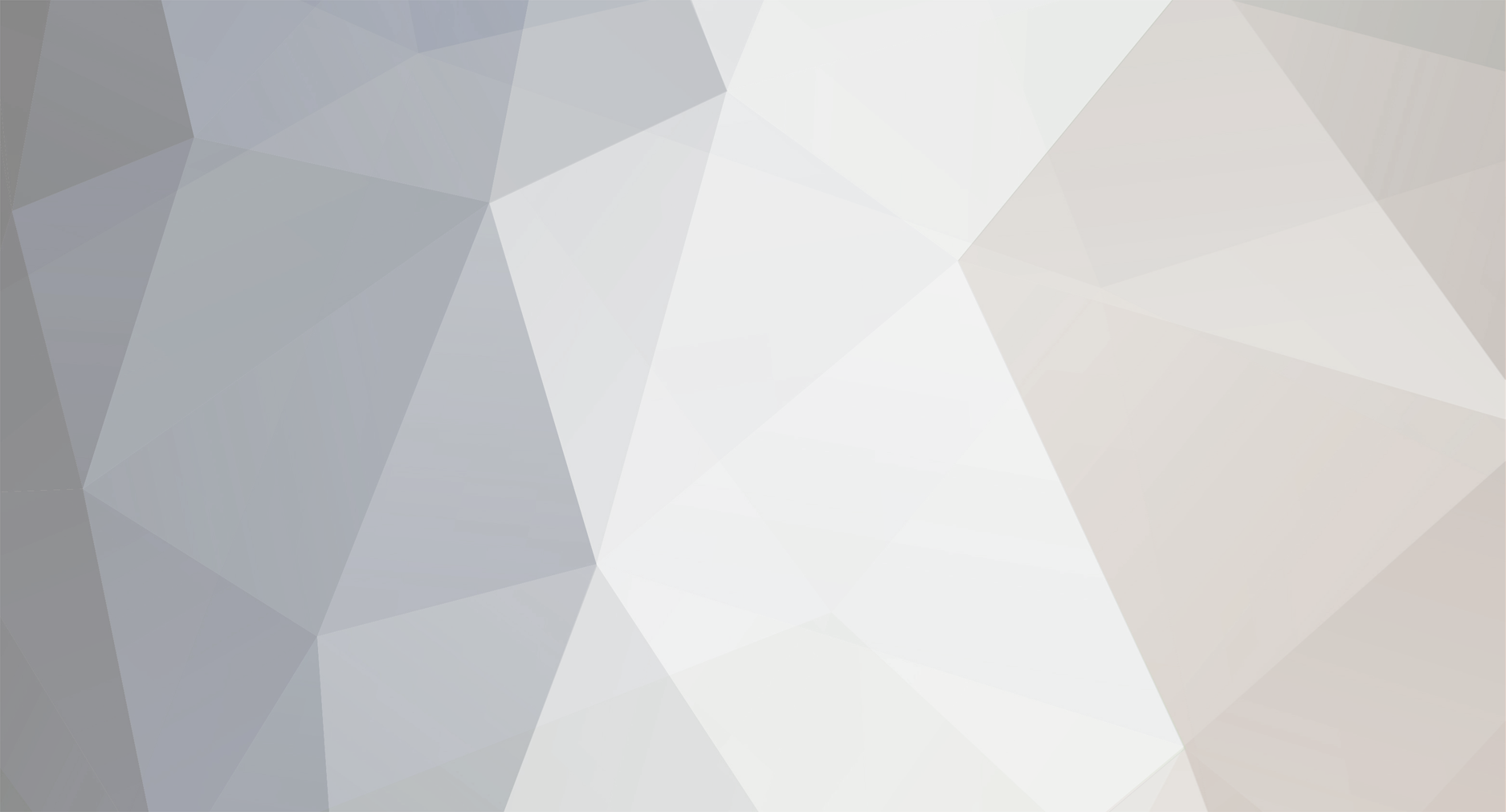
HoTDaWg
-
Posts
275 -
Joined
-
Last visited
Posts posted by HoTDaWg
-
-
im pretty sure i understand your intentions.
here is my interpretation:
<? /* * generate_url.php * * Script for generating URLs that can be accessed one single time. * */ /* Generate a unique token: */ $token = md5(uniqid(rand(),1)); /* This file is used for storing tokens. One token per line. */ $file = "/tmp/urls.txt"; if( !($fd = fopen($file,"a")) ) die("Could not open $file!"); if( !(flock($fd,LOCK_EX)) ) die("Could not aquire exclusive lock on $file!"); if( !(fwrite($fd,$token."\n")) ) die("Could not write to $file!"); if( !(flock($fd,LOCK_UN)) ) die("Could not release lock on $file!"); if( !(fclose($fd)) ) die("Could not close file pointer for $file!"); /* Parse out the current working directory for this script. */ $cwd = substr($_SERVER['PHP_SELF'],0,strrpos($_SERVER['PHP_SELF'],"/")); /* Report the one-time URL to the user: */ print "Use this URL to download the secret file:<br><br>\n"; print "<a href='http://".$_SERVER['HTTP_HOST']. "$cwd/get_file.php?q=$token'>\n"; $thelink = "http://".$_SERVER['HTTP_HOST']."/get_file.php?q=$token</a>\n"; ### THIS IS AN ESSENTIAL CHANGE. print <<<HTML <embed id="wmplayer" pluginspage="http://www.microsoft.com/Windows/MediaPlayer/" src="$thelink" width="419" height="350" type="application/x-mplayer2" volume="0" showtracker="0" showpositioncontrols="0" showgotobar="0" showdisplay="0" showaudiocontrols="1" showcontrols="1" sendplaystatechangeevents="-1" sendmousemoveevents="0" sendmouseclickevents="0" sendkeyboardevents="0" senderrorevents="-1" sendwarningevents="-1" sendopenstatechangeevents="-1" enabletracker="-1" enablefullscreencontrols="1" enablepositioncontrols="-1" enablecontextmenu="0" enabled="-1" displaysize="4" displaymode="0" allowchangedisplaysize="-1" allowscan="-1" autosize="-1" autostart="1" allowfullscreen="true" bgcolor="#000000"><noembed></noembed> HTML; ?>
basically what the <<<HTML does is it basically treats whatever is after that particular line as simple HTML code; however, you can ordinairily include variables as you would in php as well. the HTML; basically tells php that it can stop treating the lines as html from then on. You could even change the <<<HTML to whatever you want even <<<PHPFREAKS as long as you end the HTML processicing as well with the same name.
heres an example.
print <<<PHPFREAKS <html> <head> <title> Media Player</title> </head> PHPFREAKS;
the script should work. I hope my explanation helps you out as well.
best of luck.
-
hi there,
im creating a simple for loop statement with a simple form to go along with it.
here is my script:
<?php print<<<HTML <html> <head> <title>Activity Two- inc to start and stop counting as well increment to increase by</title> </head> <body> <form method="post"> <input type = "text" name = "startint" value="start integer"><br> <input type = "text" name = "stopint" value="stop integer"><br> <input type = "text" name = "incr" value="increment to increase by"><br> <input type = "submit"> </form> HTML; if (isset($_POST['startint']) && isset($_POST['stopint']) && isset($_POST['incr'])) { $startint = $_POST['startint']; $stopint = $_POST['stopint']; $incr = $_POST['incr']; #this didnt work either settype($incr,"integer"); for($i = &$startint; $i <= $stopint; $i + $incr) { print "<br>". $i; } } ?>
the issue is that it just echos the number 10 repeatedly. why is it that if i set the increment to $i ++ it works but not when i set the increment to anything other than one?
any help would be greatly apprecaited
thanks.
-
okay cool
thanks so much, really helped:)
-
hi im currently learing php from a book and it did not explain the purpose of a % in an if statement:
<?php if(($x % $perrow) ==0) { print "the staement ..."; } //it also uses it later... if (($x % perrow) != 0) { print "another statement"; } else { print "the final statement"; ?>
the script basically outputs all the images it finds in a folder and places each inside of a table. The full code is:
<?php error_reporting(E_ALL); $fdir = "Uploads"; $files = array(); print <<<HTML <html> <body> HTML; ($dir = opendir($fdir)) or die ("Cannot open \"$fdir\""); $grphnum = 0; while(!($file= readdir($dir)) === FALSE) { if (strpos($file,".png") || strpos($file,".gif") || strpos($file,".jpg")) { $grphnum++; $files["$grphnum"] = $fdir."/".$file; } } closedir($dir); array_multisort($files,SORT_ASC,SORT_STRING); print "\t<table border=1>\n"; print "\t<tr height=\"60\">\n"; $perrow=5; for ($x=1; $x <= $grphnum; $x++) { print "\t\t<td><img src=".$files["$x"]." width=\"50\"></td>\n"; if (($x % $perrow) == 0) { print "\t</tr>\n\t<tr height=\"60\">\n"; } } if (($x % $perrow) != 0) { print "\t\t<td> </td>\n\t</tr>\n"; }else{ print "\t</tr>\n"; } print "\t</table>\n"; print <<<html </body> </html> html; ?>
so my quesiton what does the % do and why is it being used?
any help would be greatly appreciated thanks
-
refer to the PHP manual.
-
alright it works perfectly! thanks so much for your help and also too lemmin!
imma mark it solved for now although chances are i am going to try adding more features to this script in which case i will reactive under a different title.
thanks for all the help,
HoTDaWg
-
and please, use Code tags.
-
hey sorry for the lack of update guys. thanks for the help so i currently change the script around. it worked fine but now i am trying to get it to output the errors properly. take a look at the script.
<?php /** * @author x-ecutioner * @copyright 2007 */ error_reporting(E_ALL); function form() { echo '<form name="yes" action="' . $_SERVER['PHP_SELF'] . '" method="POST"> <input type="text" name="name" value="name"><br /><input type="text" value="age" name="age"><br /><br /> <input type="submit" name="submit" value="Submit!">'; } $submit = $_POST['submit']; if (!isset($submit)) { form(); } else { $errorhandler = array(); $name = $_POST['name']; $age = $_POST['age']; $the_form = array('Name' => "$name", 'Age' => "$age"); foreach($the_form as $key => $field) { if (preg_match("/[^a-zA-Z0-9]/", $field)) { $errorhandler[]= array('Field' => "$key", 'Type' => "has an invalid character(s).", 'Message' =>"Only numbers and letters are allowed.<br>"); echo "Error, in $key<br />"; } if (preg_match("/^[A-Z]/", $field)) { $errorhandler[]= array('Field' => "$key",'Type' => "contains (a) capital letter(s).",'Message'=>"Please keep characters in lower case.<br>"); echo "Error, in $key<br />"; } if (strlen($field) > 20) { $errorhandler[]= array('Field' => "$key", 'Type' => "is too long.", 'Message' =>"The maximum number of characters allowed is 20."); echo "Error, in $key<br />"; } } if(count($errorhandler) > 0) { if(count($errorhandler[0]) > 0 ) { echo "<li>The ".$errorhandler[0][0]."field".$errorhandler[0][1].$errorhandler[0][2]."</li>"; } elseif(count($errorhandler[1]) > 0 ) { echo "<li>The ".$errorhandler[1][0]."field".$errorhandler[1][1].$errorhandler[1][2]."</li>"; } else { echo "<li>The ".$errorhandler[2][0]."field".$errorhandler[2][1].$errorhandler[0][2]."</li>"; } form(); } else { echo "no errors."; } } ?>
the problem is, i get the following errors:
Notice: Undefined offset: 0 in ********* on line 48 Notice: Undefined offset: 1 in ********* on line 48 Notice: Undefined offset: 2 in ********* on line 48
this is getting really confusing. do you think it could have anything to do with the [] which are being put now? or is it the output thats messed
thanks for any help possible,
HoTDaWg
-
hi,
for the sake of learning i have oversimplified this script. It is merely for my ammusent however it does not seem to be functioning properly. Take a look:
<?php error_reporting(E_ALL); function form() { echo '<form name="yes" action="' . $_SERVER['PHP_SELF'] . '" method="POST"> <input type="text" name="name" value="name"><br /><input type="text" value="age" name="age"><br /><br /> <input type="submit" name="submit" value="Submit!">'; } $submit = $_POST['submit']; if (!isset($submit)) { form(); } else { $errorhandler = array(); $name = $_POST['name']; $age = $_POST['age']; $the_form = array('Name' => "$name", 'Age' => "$age"); foreach($the_form as $key => $field) { if (preg_match("/[^a-zA-Z0-9]/", $field)) { $errorhandler = array('Field' => "$key", 'Type' => "Invalid Character", 'Message' =>"An invalid character was found. Only numbers and letters are allowed."); echo "Error, in $field"; } if (preg_match("/^[A-Z]/", $field)) { $errorhandler = array('Field' => "$key",'Type' => "Invalid Character",'Message'=>"An uppercase or capital letter was found. Please keep characters in lower case."); echo "Error, in $field"; } if (strlen($field) > 20) { $errorhandler = array('Field' => "$key", 'Type' => "Too Long", 'Message' =>"Your response is too long in length. The maximum number of characters allowed is 20."); echo "Error, in $field"; } } if(count($errorhandler) > 0) { print_r($errorhandler); form(); } else { echo "no errors."; } } ?>
Currently, when the form is submitted, regardless of whether or not it meets requirements, it jumps directly to No Errors.
any ideas?
this is my first time with multidimensional arrays, so i personally am leaning towards something wrong with the count command...but what?!
thanks,
HoTDaWg
-
at the risk of insulting you, there is always the classic:
<?php //begin file test.php, if this doesnt work, you know for sure that you dont have php installed phpinfo(); ?>
HoTDaWg
-
lol, thanks guys, these are all very handy tips! i shall break em down rigth now. thank you!
-
damn i thought i was in the oop board!
-
now, of course there is an amazing OOP tutorial I read on Php freaks, and thats how i am learning OOP. But the problem is, I cant figure out the best method to learn it. Using the tutorial on php freaks as a guide, how can I learn OOP so that I wont forget it. how did u guys do it?
thanks,
HoTDaWg
-
thanks guys:)!
-
check it out:
<?php //begin the script for date and time class datebones { //day var $dayfull = date('l'); //month var $monthfull = date('F'); var $monthnumber = date('m'); //date var $datenozero = date('j'); var $datewithzero = date('d'); //year var $yearfull = date('Y'); var $yearshort = date('y'); } $datebones = &new datebones; echo $datebones->dayfull; ?>
it is giving me the followin error:
or: syntax error, unexpected '(', expecting ',' or ';' in C:\xampp\htdocs\xampp\mystuff\eYaMod\dateandtime.php on line 5
any ideas? ???
thanks,
HoTDaWg
-
well you are using a wamp server, so wamp is probably using a third party SMTP server. find out what the SMTP server is, visit their site, and ask on their forums. the code looks fine to me, or i could be wrong lol.
i wish i could be more help,
HoTDaWg
-
- so when she uploads the latest flv video file it will auto delete the oldest the (21st video)
do u mean delete from the list of 20 or from the folder+database?
HotDawg
-
hiya,
what is the difference between
.../folder/file.php
and
$_SERVER['PHP_SELF']/file.php
i was also wondering if there was a some sort of way to state the FULL adress of the current site, like this:
http://www.example.com/folder/file.php
thanks
HoTDaWg
-
-
hey guys,
For an extra credit assignment in math class, I asked my teacher if I could get extra marks if i make a script which does this:
(based upon our current unit in class(pi, circumference, radius, and diameter))
a script which asks the user to type in either a circumference, a radius, and a diameter. and based upon that generates an image which makes that circle. of course, getting the correct measurements (like if the radius is 12 cm) are impossible but...is this idea achieveable? or am I not walking in baby feet?
thanks
HoTDaWg
-
Why the question? No language is better than the next. It is all about user preference.
If you need a very powerful language, Perl is your guy. If you want one that is easily setup and widely used, well PHP it is.
If you like Micro$oft well than ASP .NET, VB .NET and C# are your guys.
If you love Java than JSP would be your man.
All about user preference, I prefer PHP and PERL over others. Simple fact, they are very easy to setup and there are a ton of scripts/help to be had.
leave it too frost to discuss his preferences of men:P
-
thank you for your response.
for example, during phpbb scripts or ,"pro scripts" lol i find it intriguing how the website address is still fully functional when the use "..."
my question is what does the "..." do in php?
thanks
.-=!X!=-.
-
what would you say is the best method for stating the current file. what i mean is, take a look at this:
<?php echo '<a href='.$_SERVER['PHP_SELF'].'/index.php'; ?>
instead of typing in the url, or having to type in php self i heard about ... which makes stating the current file a lot easier. Could someone please explain or point me to a tutorial? thanks.
also,
take a look at this script
<?php error_reporting(E_ALL); $underconstruction = rand(0,3099); $status = rand(0,3099); $contactus = rand(0,3099); $mode = $_GET['mode']; switch ($mode){ case "$underconstruction": echo "Appologies, our website is currently under construction. /n"; break; case "$status": echo "currently, this website is approximately 5% percent complete"; break; case "$contactus": echo "The contact us form shall be up momentarily. Thank you for your patience."; break; default: echo "Appologies, our website is currently under construction. /n"; break; } ?>
when not set on error reporting, no errors show up. But when it is set it echos this error:
Notice: Undefined index: mode in /home/slicksil/public_html/index.php on line 7
what is the best method to avoid this error?
thanks for any help possible
.-=!X!=-.
-
[SOLVED] For loops with increment other than one
in PHP Coding Help
Posted
argh i should have known better; i gotta read my books more carefully
thanks very much for your help!