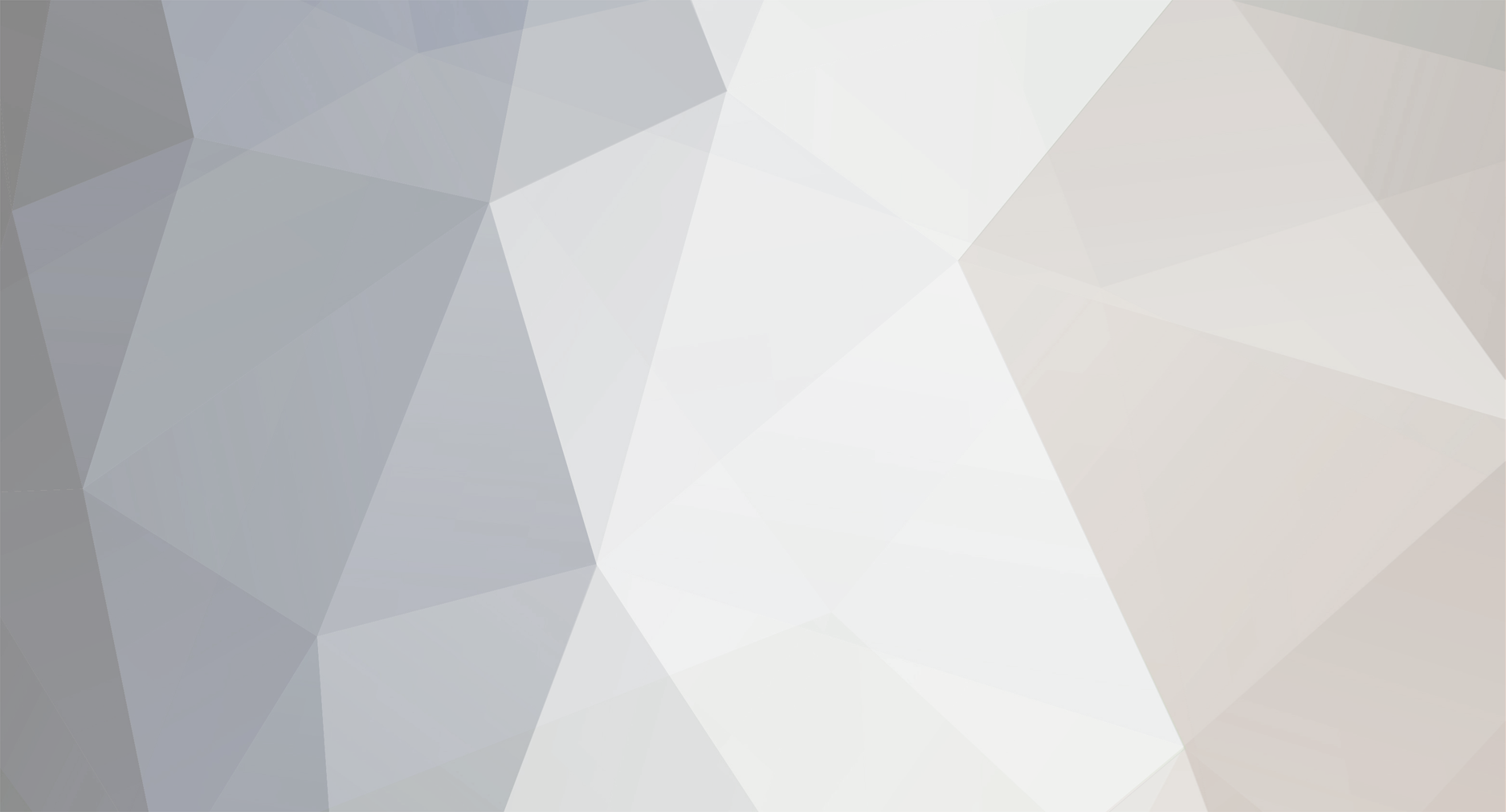
Prismatic
-
Posts
503 -
Joined
-
Last visited
Posts posted by Prismatic
-
-
I'm going to guess a properly normalized database table -
SELECT your_column, COUNT(*) FROM your_table GROUP BY your_column
You really only need to return the data you need,
$query = "SELECT disctinct(your_column), COUNT(*) FROM your_table GROUP BY your_column"
-
Do you want just a single row output to CSV or the entire database?
-
First, return will only run once, which means $username is the only var being returned.
If you need to return multiple variables stuff them in an array and return that.
Also, once you call return the function exits, so if($results != true) and everything below it doesn't executute.
also once you've returned your data you want to assign it to a varable,
$data = signin_ck($pg_priv);
now assuming you build an array with your data it will be accessable with $data["username"], $data["userpass"] etc.
-
Do you know how to write PHP well?
ha ha ha...
i'vent asked you for code, i asked for suggestion how to proceed.
FORUMS are for exchanging views and ideas not for exchanging "who knows waht? or who knows well? than others""
please take it as my advice and dun ever never post such silly replies..
for ur information i succeeded in writing the code.
i pointed code gurus and experts but NOT U personally,
if u know the solution then boost up the newbies with ur thoughts else simply delete your account and do ur home work.
coming to the point,
i used PHPEXCEL to read and write spreadsheets in a web browser, previously i used only php reader which parses the excel file and prints the cells which is not editable.
Many Thanks,
"Never argue with an idiot,they drag you down to their level and then beat you with experience" lol
Chillout. He was simply asking if you knew any PHP because otherwise what you're essentially doing is asking us to code this for you.
You won't find a pure PHP option to do this since php is server side. There's a Javascript spreadsheet engine called TrimSpreadsheet by Trimpath that can turn a table into a spreadsheet automatically.
You could use PHP to generate a table with all the information in the correct cells, then pass that table to TrimSpreadhseet to turn it into a functional spreadsheet.
Likewise you would use Javascript to turn the resulting spreadsheet data into an array to be passed to PHP when you wish to save the spreadsheet.
-
There's no reliable way to be sure your user's IP is real.
I can bounce my IP off 8 proxies before I hit your site and you'd have no idea what country I'm from.
-
<?php class myClass { public $var = "Hello World"; public function ePrint() { echo $this->var; } } $class = new myClass(); echo $class->var; echo "<br />"; $class->ePrint(); ?>
-
"" . $explode[$x] . ""
that basically adds nothing at all before and after $explode[$x]
-
Whats your reasoning for adding an empty string before and after each array entry?
-
Looks to me like someone tried to inject some PHP into your guestbook
-
I wanted to make the username case sensitive on my log-in page, so I made the script below.
But it redirects to http://mysite.com/login.php?cmd=nouser even if the username is typed correctly (in proper case)..?
Thanks in advance.
$check = mysql_query("SELECT username FROM users WHERE username = '".$_POST['username']."'")or die(mysql_error());if(strcmp($_POST['username'], $check) != 0) {
header("Location: http://mysite.com/login.php?cmd=nouser");
die('');
}
You need to fetch the row after running the query
$row = mysql_fetch_assoc($check);
if(strcmp($_POST['username'], $row['username']) != 0) {
-
-
-
my DB is similar to this
id | name | title
I need to be able to show 3 columns of data at a time, then drop to a new line
So it would go
Name | Name | Name
Name | Name | Name
....
In a table
Any ideas?
-
How do you physically delete files from a ftp server using a php script?
-
clean code!
<?php $countdown = "14"; if(date("dS F Y") < ($countdown) + ($row_rs1_events['myedate'])) { print ("<a href=\"regs/{$row_rs1_events['regs']}\" target=\"_blank\">Download Regs</a>"); } ?>
-
I can write you a script that converts images to pure html, though your pages tend to be several hundred megs
-
Prismatic, that requires calling the function twice...which is not efficient.
Doesn't require it.
$blah = myfunct();
if($blah !== true)
-
<?php function log_in($email, $password){ if(empty($email) and empty($password)){ $error = "Empty username or password"; } $email = clean($email); $password = md5(md5(clean($password))); db("discuss"); $res = sql("SELECT * FROM `users` WHERE upper(email)=upper('$email') and `password`='$password' "); if(mysql_num_rows($res) == 1){ $row = mysql_fetch_array($res); $_SESSION['logged_in'] = true; $_SESSION['user_id'] = $row['user_id']; $_SESSION['username'] = $row['username']; $_SESSION['key'] = $row['key']; //grab friends and put it in session if($row['user_id'] == 1){//if user id is 1 $_SESSION['admin'] = true; } $time = date("Y-m-d H:i:s"); $id = $row['user_id']; sql("DELETE FROM `online_now` WHERE `user_id`='$id' LIMIT 1"); sql("INSERT INTO `online_now` (`user_id`, `time`)VALUES('$id', '$time')"); }else{//end if one row is found $res = sql("SELECT `user_id` FROM `users` WHERE `email`='$email' "); if(mysql_num_rows($res) == 0){ $error = "Email does not exist"; }else{ $error = "Wrong Password"; } }//end else if(isset($error)){ return false; return $error; }else{ return true; } }//end log in ?>
Ok do this
<?php function log_in($email, $password){ if(empty($email) and empty($password)) { $error = "Empty username or password"; } $email = clean($email); $password = md5(md5(clean($password))); db("discuss"); $res = sql("SELECT * FROM `users` WHERE upper(email)=upper('$email') and `password`='$password' "); if(mysql_num_rows($res) == 1) { $row = mysql_fetch_array($res); $_SESSION['logged_in'] = true; $_SESSION['user_id'] = $row['user_id']; $_SESSION['username'] = $row['username']; $_SESSION['key'] = $row['key']; //grab friends and put it in session if($row['user_id'] == 1) {//if user id is 1 $_SESSION['admin'] = true; } $time = date("Y-m-d H:i:s"); $id = $row['user_id']; sql("DELETE FROM `online_now` WHERE `user_id`='$id' LIMIT 1"); sql("INSERT INTO `online_now` (`user_id`, `time`)VALUES('$id', '$time')"); } else {//end if one row is found $res = sql("SELECT `user_id` FROM `users` WHERE `email`='$email' "); if(mysql_num_rows($res) == 0){ $error = "Email does not exist"; } else { $error = "Wrong Password"; } }//end else if(isset($error)){ return $error; } else { return true; } }//end log in ?>
Then check your function
<?php if(log_in(blah, blah) !== true) { /** Function is not true, so it contains our error message */ echo log_in(blah, blah); } else { /** Function returned true */ echo "Success!"; } ?>
if it's
-
You can.
<?php function Boo($var) { if($var == "test") { return true; } else { return "This is false"; } } if(Boo() !== true) { echo Boo(); } else { echo "True"; } ?>
if(boo() !== true) returns "This is false"
if(boo("test") !== true) echos "True"
-
i have just done this to the function i am calling upon
<?php error_reporting(E_ALL); function account() { // ftp variables $settings['ftpServer'] = 'la'; $settings['ftpUsername'] = 'sadfs'; $settings['ftpPassword'] = 'Cdsfdsffsdfsdfsdf'; return $settings; } $setting = account(); print_r($setting); ?>
and when i run the script the array prints out. But when include i get undefined.
You're calling getSettings()
the function is account()
-
Don't tell me... Leading slash?
-
<?php include ("tax.php"); $amount = 95; echo "Price beforer tax: $amount"; echo " Price after tax: "; echo add_tax($amount); echo "To incorporate an external library file into another script: use INCLUDE "; print "The following includes tax.php so I can call add_tax "; ?>
WILL work assuming tax.php is in the same directory you're calling load20.php from
The system path is just where the file resides on the server. This is most always different on every machine. If you're unsure of your servers configuration simply running the following code in the same directory as load20.php will tell you the full system path to that file
<?php echo getcwd(); ?>
However absolute paths aren't required in most cases and a relative path will suffice, for example
if tax.php is in the same folder as load20.php you can just call include("tax.php");
if tax.php is in a folder ABOVE the folder load20.php is in, you get to it with include("../tax.php");
if it's BELOW the folder load20.php is in you include it with include("/folder/tax.php");
I can tell you right now the full path is "/usr/www/users/medbqq/jaco/sams2/" from your error you posted before, but like I said, you dont need it.
-
CSS isn't required for PHP since CSS is mainly for the design element of a site.
A lot will tell you a book is the best way, for me the best way was to download some scripts and jump in, look at what they do, scour the php.net manual figuring out what function does what, but thats just me, if you're a book learner then yes get a book, but if you lean via hands on, I recommend picking some scripts apart.
-
Gonna need to see some more code to help you out with this
I can count, but my code can't
in PHP Coding Help
Posted
Whatever!