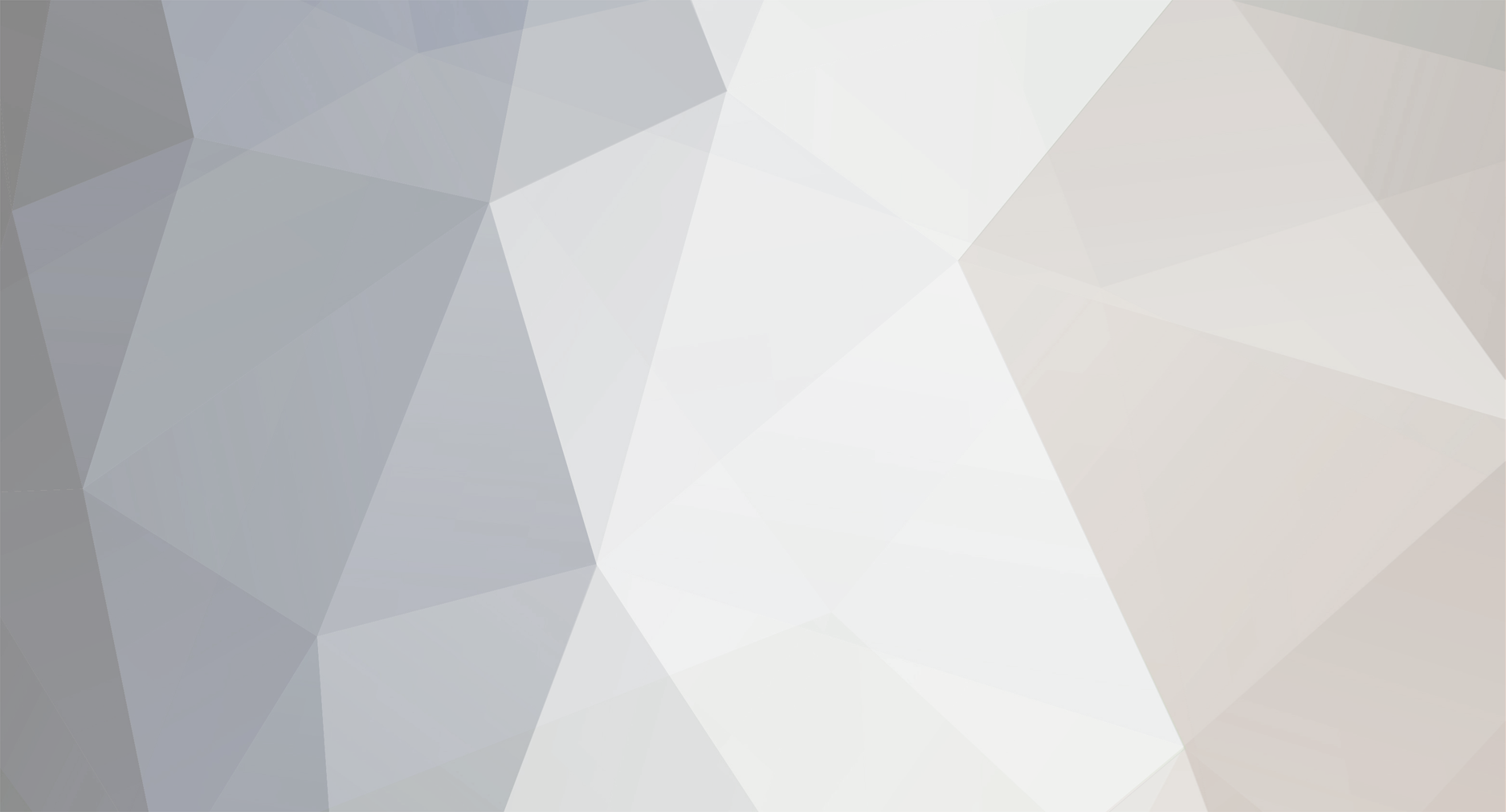
Cinner
-
Posts
17 -
Joined
-
Last visited
Never
Posts posted by Cinner
-
-
Hi guys,
I have a multidimensional array containing movie data. One of the elements is a movie title, and for each title that starts with "The", I want to cut that part and put it at the end of the element value (like "Terminator, The"). This would be easy if I would do this during a regular foreach loop echoing all the movie titles, but what I want to do is update the array itself, since I will be doing other things with this array later. Here's an example of what my array could look like:
$movies = Array ( [0] => Array ( [id] => 1 [year] => 1984 [title] => The Terminator), [1] => Array ( [id] => 2 [year] => 1994 [title] => True Lies) );
The real array is a bit more complex than that but you get the picture. How do I go through it and look for "The" in the title, and put it at the end of the movie title?
-
Hey guys, I'm building a shopping cart system for myself, and could use some help.
My MYSQL database has a table called PRODUCTS, with the following fields: ID | TITLE | TYPE
I have a shopping basket array (called $items) containing product ID's, for example: 1, 3, 2, 2, 3, 3.
I can query the products from my database, based on the ID's in that array. But I would like to count the corresponding TYPEs of those products, and I'm not sure how (the TYPE is not in the array)? I need to know (count) how many times an ID was placed in the $items array, and what the corresponding TYPES are by querying the database (so I can say TYPE x was placed x times in the shopping basket).
-
I downloaded this simple Javascript that changes the background of an element when you press a button. Like this:
<script type="text/javascript"> function ChangeBackground(background) { switch (background) { case 1: document.getElementById('myTableCell').style.backgroundImage = 'URL(/Images/Demos/ChangeBackground/Background1.gif)'; break; case 2: document.getElementById('myTableCell').style.backgroundImage = 'URL(/Images/Demos/ChangeBackground/Background2.gif)'; break; } } </script>
Then you give the button this simple property: onclick="ChangeBackground(1)" or "ChangeBackground(2)".
But the thing is, I have a list of items that come from a mysql datase with php, and each item has it's own two background images a user can choose from. Those images are stored as URL's in the database. Is there a way I can pass those URL's through the buttons along with the "1" and "2"? Something like ChangeBackground(1,url1). My javascript knowledge is very limited I'm afraid.
-
I have a multidimensional array that fills with data from a database. The array could look like this:
Array ( [0] => Array ( [guests] => Array ( [name] => Barbara Bouchet [id] => 32 [photo] => /bouchet.jpg [bio] => This is her biography. ) ) [1] => Array ( [guests] => Array ( [name] => Edwige Fenech [id] => 3 [photo] => /fenech.jpg [bio] => I'm a great actress. ) ) )
This is how the array is composed:
$posts[] = array( 'guests' => array( 'name' => $row['name'], 'id' => $row['guestID'], 'photo' => $row['photourl'], 'bio' => $row['biography'] ), );
Let's say I want to display all the information of a particular guest, but I only know his 'id' (like $guestID = 3). How do I do this? I think I would need to know which array key belongs to that 'id' and them I could just use something like echo $posts[1]['guests']['name'] to display the data, right?
-
Uh, the second one was supposed to be a slash followed by the letter that follows "q", not the word "are."
OMG that fixed it! Yes I used "are" instead of the "letter that follows q", and now I fixed that it actually works. Thanks you and everybody else for helping! Problem solved
-
Instead of returning the "straight" biography data, try using the following SQL query:
$guests_query = 'SELECT name, guestID, photour1, REPLACE(biography,"\n","<br>") AS biography FROM guests ORDER BY name';
If that doesn't work, try "\are\n" instead of "\n".
Well, we can rule that one out as well. Both versions don't work unfortunately.
-
Cinner, it looks like you're trying to define a JavaScript array by creating the code dynamically with PHP. Is that right? And you said the data comes from a database? Can I see the query line that extracts the data from the database? Is it MySQL?
Yes you are right: I'm defining a JS array with content coming from an array that's filled with data from a MYSQL database. Here's the code:
//select guests $guests_query = " SELECT * FROM guests ORDER BY name ASC"; $resultG = mysql_query($guests_query); //results for guests //search results & fill array while ($rowG = mysql_fetch_assoc($resultG)) { // fill array $postsG[] = array( 'guests' => array( 'name' => $rowG['name'], 'id' => $rowG['guestID'], 'photo' => $rowG['photourl'], 'bio' => $rowG['biography'] ), ); } mysql_free_result($resultG);
Because nl2br ADDS a HTML line break and doesn't replace it, try the following...That does not work either ???
-
-.-
linebreak = 13;
echo ord("\n"); //13
Shows how much I know. I looked "13" up and it refers to an "enter", right? Which makes sense. Thanks for clearing that up. But still, the value of $bio still breaks the code :-\
-
Just to be clear: the data isn't stored with \n, but it is stored in multiple lines (mysql longtext field). I can't replace \n because it's not there. I tried the above suggestion, but it doesn't work either. So keep those suggestions coming please :-)
-
Thanks, but I already tried that one. No result unfortunately. But maybe I didn't use it correctly:
<? foreach ($postsG as $p) { $bio = nl2br($p['guests']['bio']); ?> content[<? echo $p['guests']['id'];?>]= "<?echo '<big><b>' . $p['guests']['name'] . '</b></big>' , $bio , '';?>" <? } ?>
-
Correction, I'm looking for:
line 1 <br> line 2
-
It doesn't work. The code now looks like this:
<? foreach ($postsG as $p) { $bio = str_replace("\n", '\\n', $p['guests']['bio']); ?> content[<? echo $p['guests']['id'];?>]= "<?echo '<big><b>' . $p['guests']['name'] . '</b></big>' , $bio , '';?>" <? } ?>
Shouldn't I see that newline character when viewing the data in the database itself (phpmyadmin)? Because it's not there. The text is stored in several lines without <br> and such, like this:
line 1
line 2
Not:
line 1<br>
line 2
Also not:
line 1 line 2
I guess I'm looking for a way to make it:
line 1 <br> line 2
-
Sorry I made a typo, the content in the database is NOT stored with \n. So there's nothing to replace with str_replace, right?
because the content comes from a database where it's stored with \n.
-
What language to you want to use?
In both (Javascript and PHP) is \n a linebreak and can be used with "
So for example:
$content = "<p>My content</p>\n<p>so</p>";
Well I don't think I can use that because the content comes from a database where it's stored with \n. Could I add the \n when putting the data in the array for example (the array which 'content' - javascript - uses)?
-
I can't modify my first post it seems, but if I could, the title would be: "Help: multiple lines break my code".
Hopefully somebody can help with this problem.
-
First of, sorry for the poor Subject title, I'll change it once I know what the problem is.
I just ran into a problem that I think shouldn't be hard to fix (it is for me though). I'm trying to use a function that puts data from an array into a new array, like this:
var content=new Array() //change the array below to the text associated with your links Expand or contract the array, depending on how many links you have <? foreach ($postsG as $p) {?> content[<? echo $p['guests']['id'];?>]= '<?echo '<big><b>' . $p['guests']['name'] . '</b></big>' , $p['guests']['bio'] , ''; ?>' <? } ?>
You can probably tell by looking at that code I'm pretty new to php. I'm using a javascript function that I modified to work with my mysql database. It originally looks like this (and isn't php by default):
content[]='<br><big><b>Title</b></big><br>You content here.'
Everything works fine (there's more to the code than I've put up here), but it gets into trouble when I define "content" as text containing multiple <p> paragraphs. It's stored in the database like this:
<p>paragraph one</p>
<p>paragraph two</p>
Which unfortunately makes the above code look like this:
content[]='<p>paragraph one</p> <p>paragraph two</p>'
The problem is that those two paragraphs aren't one line but two, and that 'breaks' the code. In contrast, this works fine:
content[]='<p>paragraph one</p> <p>paragraph two</p>'
So I'm guessing I should find a way to put the database data into a single line somehow. Or not. Anybody a suggestion?
modify multidimensional array values that meet a criterion
in PHP Coding Help
Posted
Excellent, that was most helpful. Thanks!