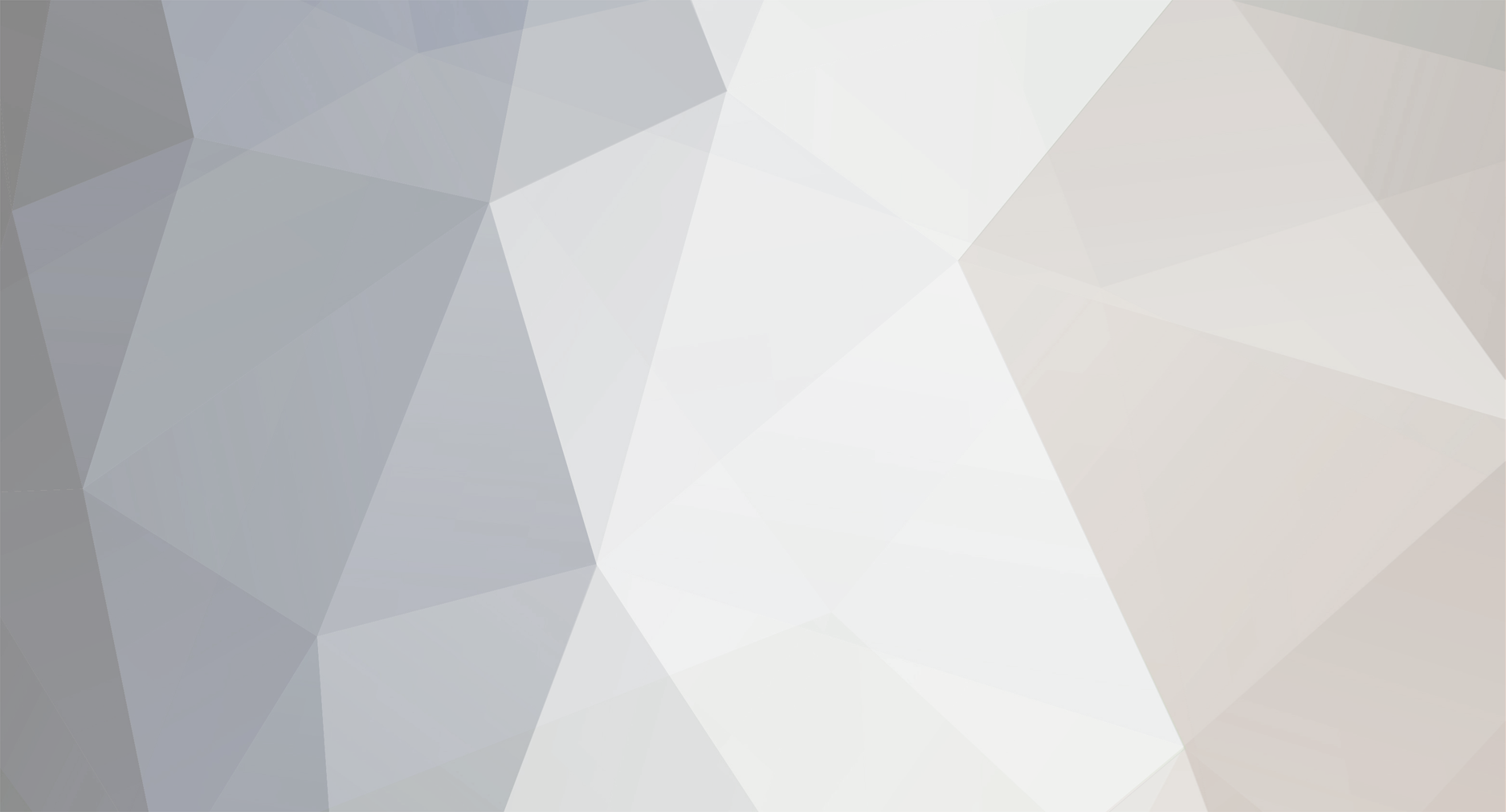
willfitch
Members-
Posts
109 -
Joined
-
Last visited
Never
Everything posted by willfitch
-
Accessing members and methods of classes using the Scope Resolution Operator ( :: ) is not a bad way, when you're referencing a parent or static member. The best way is through a form of polymorphism. For instance, if I were to want access to the MySQLi class (build into PHP http://us3.php.net/mysqli), I could do so like this: [code=php:0] <?php $mysqli = new mysqli('host','user','pass','db'); class myclass { private $db; public function __construct($db) { $this->db = $db; } public function __destruct() { $this->db->close(); } } $test = new myclass($mysqli); ?> [/code] This will give you access within any class. Another way would be this: [code=php:0] <?php class myclass { private $db; public function __destruct() { $this->db->close(); } public function __construct() { $this->db = new mysqli('host','user','pass','db'); } } ?> [/code] In the above example, the external class is actually instantiated within the class. The final way would be to acually extend the class.
-
You have to create the checkboxes with the same name and an HTML array identifier like so: <input type="checkbox" name="model[]" value="whatever_value" /> When you access this with your handler, access it as an array: $checks = $_POST['model']; $checks now contains an array with all checked boxes. Don't forget to filter your input!
-
Even though PHP will allow the string "delete" to be compared without quotes, you are completely wrong. Furthermore, if you don't put quotes within the GET, POST, COOKIE, and SESSION array keys, PHP will have to check for a constant named whatever your key is. This is not good practice, and it will issue E_STRICT errors if they are turned on. Also, this looks like its just a simple "controller" style script. Consider using a switch() statement rather if/elseif/else constructs. Here's the deal: you have two "actions" scoped: GET and POST. This leads me to believe you aren't sure which method the incoming data is executing. This is a serious security risk. Consider revising your script with quotes around your string, and quotes within your array keys. Also, validate the incoming GET or POST act by either echoing it to the screen, or using print_r or var_dump to show incoming variables. QUOTE YOUR STINGS!!
-
empty $_POST array so reload doesn't duplicate
willfitch replied to re5etsmyth's topic in PHP Coding Help
You can remove all array elements using unset() unset($_POST); -
Need help with a search script. *SOLVED*
willfitch replied to pocobueno1388's topic in PHP Coding Help
You could also consider doing a full-text index on your fields being searched and query by relevance like so: [code=php:0] <?php $query = "SELECT MATCH(name,breed) AGAINST ('$single_key_word') AS relevance, name, breed FROM dog HAVING relevance > 0 ORDER BY relevance DESC"; ?> [/code] This would be a true search engine and bring back results ordered by their relevance. Remember, the fields (name,breed) will have to have the FULLTEXT index assigned. -
Good catch Michael. He's write, Mark.
-
Well... It appears that Seagull is using the BSD license template. This is a very loose license that basically says we don't care how you use it, as long as you have given us credit. This seems to be the case with this "proprietary" system. Now, are you calling it proprietary, or are they just refusing to give you the source code? If it's the latter, then that doesn't necessarily mean it's proprietary. They may give you the source if you pay them, but not if you get the free version. The best situation is to tell your friend to find a hosting company that has PHP and MySQL installed on their servers, and get a free system like OS Commerce and slap a template on it.
-
Can you tell me what it is doing? Perhaps viewing the source and telling me what is says?
-
A quick fix to this could be the following scenario: Let's assume your script name is called contact.php. 1. Make your POSTed form action contact.php?somethere_here 2. Once validation is complete, and the user has passed, insert the data and header back to contact.php.
-
Remove the -f and just use php /var/www/tmi/output.php Also, keep in mind where this file is being executed.
-
Don't use your httpd.conf file to do this! Just set the permissions to 444 and it won't be web viewable. "chmod 444 hello.php"
-
how to enable mod_rewrite through .htaccess
willfitch replied to mojito's topic in Apache HTTP Server
Then create a .htaccess file in your directory and place this in it: RewriteEngine On After that line, place any rewrite conditions you want in it. If this doesn't work, tell your hosting company to set "AllowOveride All" in your virtual host to get it going. -
Gruzin, I thought we fixed this issue yesterday on a different post. You should not start a new post if there is one open; especially if they arrive to the same conclusion.
-
SQL needs to be "SELECT genre, user FROM users" Loop using while() [code=php:0] <?php // connect PHP to MySQL mysql_connect ($Server, $Username, $Pass) or die ("Connection Denied"); mysql_select_db("tbbc") or die ("Unavaliable DB"); $result = mysql_query("SELECT user FROM users"); $i=0; while ($row = mysql_fetch_object($result)) { echo $row->user.' Genre of choice: '.$row->genre.'<br />'; $i++; } echo "$i users signed up" ?> [/code]
-
SELECT * FROM table WHERE date_field > CURDATE()
-
Barand, I'm not sure what you mean by your post, but he does need to close his <select> tag with a > <select id="CB_V" name="CB_V" style="font-family: Arial,Helvetica,Geneva,Sans-serif; font-size: 10px; color: rgb(255,102,51);"> <OPTION VALUE="1">Any</option> <OPTION VALUE="2">Below 10,000</option> <OPTION VALUE="3">10,001 to 25,000</option> <OPTION VALUE="4">25,001 to 50,000</option> <OPTION VALUE="5">50,001 to 100,000</option> <OPTION VALUE="6">100,001 to 200,000</option> <OPTION VALUE="7">200,001 to 500,000</option> <OPTION VALUE="8">500,001 to 1,000,000</option> <OPTION VALUE="9">1,000,001 to 5,000,000</option> </select>
-
Would you post a code snippet besides that particular line so we can see what you are doing?
-
Very good analysis ChaosXero!
-
This sounds like something that should be done on the database side to prevent giving birth to spaghetti code. Have you tried a query like: SELECT * FROM table WHERE latitude BETWEEN 1 AND 5 AND longitude BETWEEN 1 AND 5?
-
It sounds like you are trying to insert a record into the database and manually requesting the primary key 127 everytime. This will not work. For future reference, please post code snippets when you have issues.
-
how can i make more then one table in one install file?
willfitch replied to scheols's topic in PHP Coding Help
Glad to see it works. Ok, take out the $result->close() portion, and you will be good to go. :)