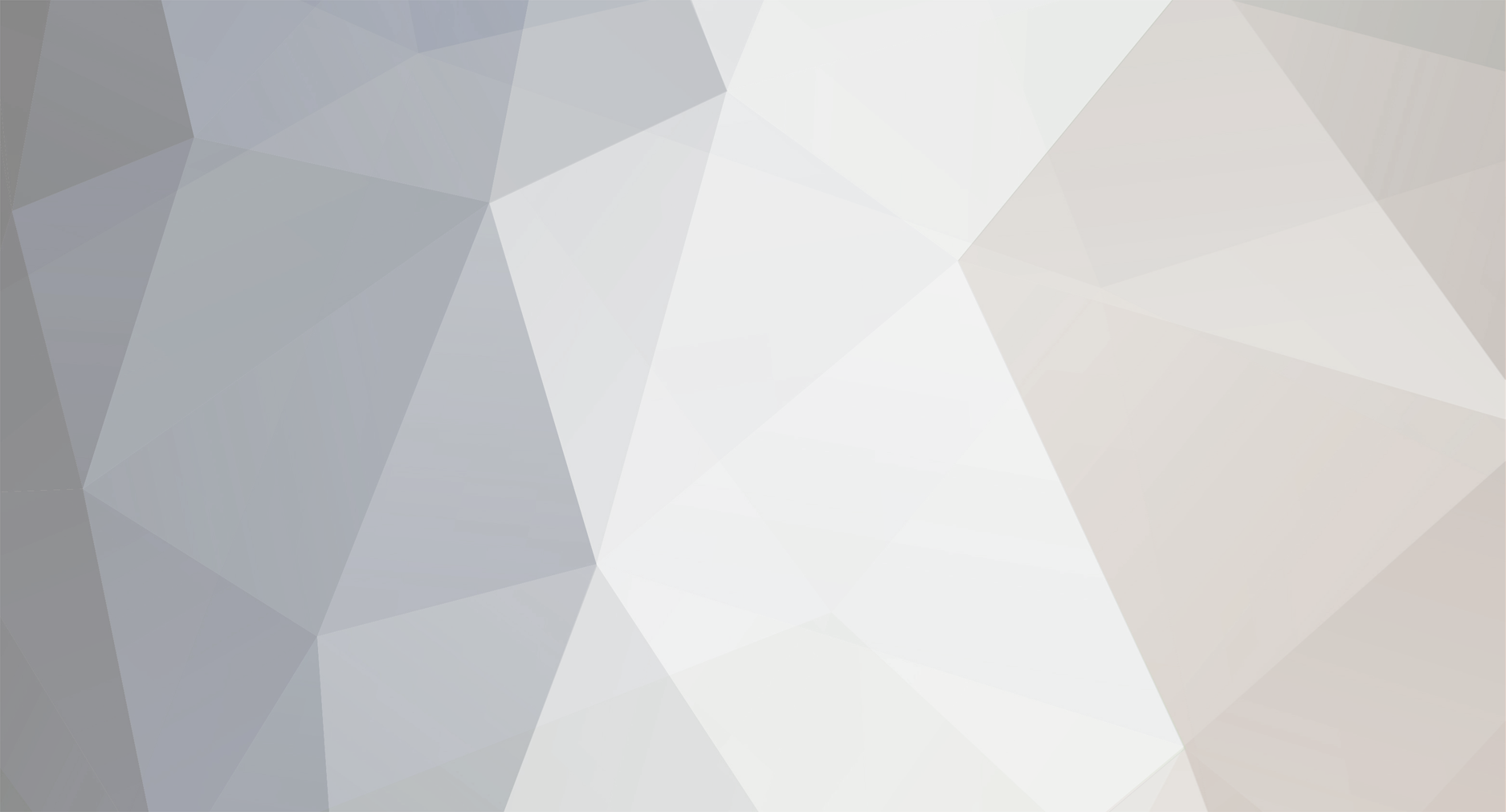
steve448
Members-
Posts
55 -
Joined
-
Last visited
Never
Everything posted by steve448
-
<?php if($_GET['id'] == 1) { //display this link } elseif($_GET['id'] == 2) { //display this link } ?>
-
Why not start by assuming that all the $uploadFile variables = '' Then if it passes all your validation checks set it to the filename. You can then finish with: <?php if($copied > 0) { //do your insert stuff if($copied != $uploadNeed) { $not_uploaded = $uploadNeed - $copied; echo $not_uploaded . ' files were not uploaded, please make sure they are valid file types etc.'; } } ?>
-
If $equipment is taken from another table then you will need to do that sql query first and use the result as $equipment before your insert
-
Does the update work if they are changing their details to something other than nothing? Also if you change: mysql_query($q, $this->connection) To: mysql_query($q, $this->connection) or die(mysql_error()); And see if it returns an error. Otherwise can you post the rest of the php script which calls the function.
-
To do this in php you will need to reload the page when they click link 1 because php is interpreted on the server and not the users browser. So: <?php echo '<a href="?id=1">link 1</a>'; if(isset($_GET['id'])) { echo '<a href="http://www.website.com/leadstoapageelsewhere">link 2</a>'; } ?> For something like this your probably better of using javascript though because then you can do it without reloading the page.
-
http://www.white-hat-web-design.co.uk/articles/php-captcha.php Is a good image verification script if we're thinking the same type of image verification
-
Your missing a semi colon ; at the end of your $message just before the mail function
-
You need to use 2 equals if your evaluating that both sides are the same as each other So this: if (($_REQUEST['areas'] = 'All') && ($_REQUEST['reps'] = 'All') && ($_REQUEST['outlets'] = 'All')) Becomes: if (($_REQUEST['areas'] == 'All') && ($_REQUEST['reps'] == 'All') && ($_REQUEST['outlets'] == 'All'))
-
if page = 'about' show the about.html page?
steve448 replied to deed02392's topic in PHP Coding Help
Try changing: elseif ($_GET['page'] == 'index' || 'home') To: elseif (($_GET['page'] == 'index') || ($_GET['page'] == 'home')) -
You need to submit the form one place first then see which radio button was selected and redirect them accordingly Example: <?php if($_POST['radio_button'] == 'button1') { header("Location: button1_page.php"); } elseif($_POST['radio_button'] == 'button2') { header("Location: button2_page.php"); } ?> In this case its probably best to put this in the top of your page with the form and set the form action to: <form action="?" method="post">
-
There are 10 different pagination scripts listed in phpfreaks code section http://www.phpfreaks.com/quickcode_cats/33/Pagination.php
-
Mysql_fetch _array help.. total number rows vs. output
steve448 replied to arpowers's topic in PHP Coding Help
You could also change your sql query to limit the number of rows returned and use SQL_CALC_FOUND_ROWS to get the total that would have been returned. -
I think I had a similar problem to this a while ago. Are you using relative includes in the cron files which include all the constants being defined? Because I found that because the script was running from the location of php and not the actual file called it wasn't finding the includes. My hosting company wouldn't allow me to use http includes so my workaround was to make it one long script with no includes, not ideal but luckily for me it wasn't that big a job.
-
Help my registration code stops processing for no reason!!
steve448 replied to daveh33's topic in PHP Coding Help
Try debugging it like the code below in a separate file away from the other code, so we can really try to isolate the error //this should force it to show the errors error_reporting("E_ALL"); $connection = mysql_connect("localhost", "dbusername", "dbpassword"); mysql_select_db("tablename", $connection); $username = '' //make this equal to a username you know exists so we are sure to get a result //Use if to test if it returns true if($result = mysql_query("SELECT * from registration WHERE username ='$username'")) { echo 'did the mysql_query part<br />'; } if($row = mysql_fetch_array($result)) { echo 'did the mysql_fetch_array part<br />'; } -
Help my registration code stops processing for no reason!!
steve448 replied to daveh33's topic in PHP Coding Help
The only thing I can suggest is that you test each line on its own. I would guess that the error is on this line: $result = mysql_query("SELECT * from registration WHERE username ='$username'") or die(mysql_error()); But it is not showing an error because you have error reporting switched off. Are you certain that in your include dbconnect.php file you have selected your database and not just connected to it using mysql_select_db($database_name, $connection_id); -
Help my registration code stops processing for no reason!!
steve448 replied to daveh33's topic in PHP Coding Help
To be honest if it's nothing to do with the first mysql_query then I have no idea. Although I would still bet that it is, try commenting that whole section out and running it again. On a slightly related note, I assume you don't have a column in your db called 'numrows' so $numrows = $row['numrows']; if ($numrows>0) { is not going to be any good, you will want to use mysql_num_rows, although this is not going to be your problem at the moment -
Help my registration code stops processing for no reason!!
steve448 replied to daveh33's topic in PHP Coding Help
Also try with mysql_error() on the first query, at the moment its just on the mysql_fetch_array I'm assuming you've selected your database in your connection file? -
Help my registration code stops processing for no reason!!
steve448 replied to daveh33's topic in PHP Coding Help
Try changing the last mysql_query to: mysql_query("INSERT INTO registration (username, password, age, msid, email) VALUES ('$username', '$password', '$dob', '$mobile', '$email')") or die(mysql_error()); I.e. Added or die(mysql_error()); So we can make sure the sql is valid and not killing the script -
Well that looks a bit easier, where were you an hour ago
-
Ok, This: fputs($fp, $content); Should be: fputs($fp, $contents); And this: foreach($sql as $sql) { $contents .= $sql . "\n"; $contents .= $sql . "\n"; $contents .= $sql . "\n"; } Should just be: foreach($sql as $sql) { $contents .= $sql . "\n"; } Sorry about this, think I've been in front of this computer too long today and my brain is frazzled, but this should work
-
Can you echo $contents to the screen, to make sure it contains the data
-
Sorry what I posted before isn't going to work at all, I've spent too much time working with my own class which gets rid of most of these steps The code you want is: <?php require_once('Connection link'); ?> <?php mysql_select_db($database_bsACKM, $bsACKM); $query = "SELECT ProductName, ProductDetails, ProductPrice FROM store"; $bsProduct = mysql_query($query, $bsACKM) or die(mysql_error()); $contents = ''; // leave this empty //use while so we can loop through the results while($sql = mysql_fetch_assoc($bsProduct)) { foreach($sql as $sql) { $contents .= $sql . "\n"; $contents .= $sql . "\n"; $contents .= $sql . "\n"; } } $file = 'test.txt'; //the filename you want to write to if($fp = fopen($file, w)) { // open the file ready for writing fputs($fp, $content); // write $contents to the file fclose($fp); // close the file } mysql_free_result($bsProduct); ?> Hopefully
-
Change this: foreach($sql as $sql) For: foreach($row_bsProduct as $sql) I only called it $sql because I didn't know the name of the array you've got. Also, leave: $contents = 'ProductName, ProductDetails, ProductPrice'; as just: $contents = ''; unless you want it displayed in the text file Let me know if this works
-
Change: mysql_query("INSERT INTO 'dino' ('idnum', 'found_by', $city', 'state', 'country') VALUES ('$idnum', '$found_by', '$city', '$state', '$country')") To: mysql_query("INSERT INTO dino (idnum, found_by, city, state, country) VALUES ('$idnum', '$found_by', '$city', '$state', '$country')"); Basically you don't want the quotes around the table columns only the inserted variables. To redirect the page you can use: header("Location: trakked.html");
-
As a workaround why not try looping through your array and building the $area_clause like: $area_clause = "AND ("; foreach($_POST['area'] as $value) { $area_clause .= 'Area = ' .$value . ' OR '; } $area_clause = substr($area_clause,0,-4); //remove the last OR $area_clause .= ")"; Then storing it in a session which you can retrieve on the next page Or you could pass the new $area_clause in the url Just an idea but should solve your problem.