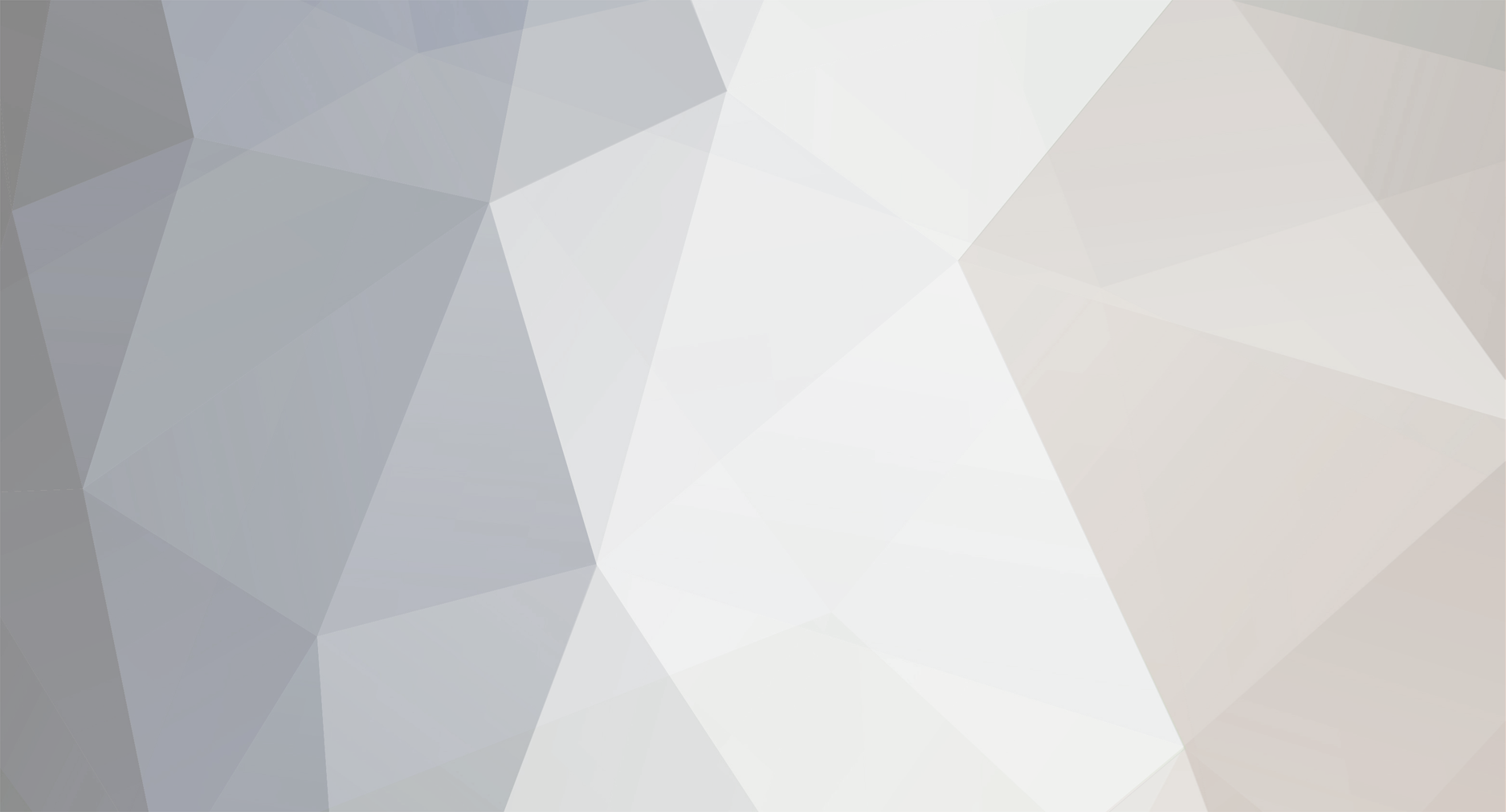
bqallover
Members-
Posts
111 -
Joined
-
Last visited
Never
Everything posted by bqallover
-
What you're doing looks good. Just access the value with $_GET['Client_Name'] as you would normally.
-
[resolved] using a URL (string) as a primary key
bqallover replied to countrybumpkin's topic in PHP Coding Help
Perhaps you need to wrap your url in quotes? Apart from that, it looks fine. [code] ("SELECT clientID FROM domains WHERE domain = '$SERVER_[HTTP_HOST]'"); [/code] -
In your echo statement, you're already 'in' PHP, so you don't need the <?= ?> bit. Instead try: [code] <? if (!$excel) { echo '<form action="emp_dtr.php" method="get" target="_parent"> <input type="hidden" name="eid" value="' . $_GET['eid'] . '">'; } ?> [/code] edit: apostrophes in the $_GET :)
-
It's just a matter of preference I think. It'll still work if echo'd, but just being pedantic you'd have to be careful to either escape the double-quotes OR wrap the echo-text in single quotes.
-
i want to add a table with every date since 1900 to current
bqallover replied to emehrkay's topic in PHP Coding Help
You could do something like this. It's not the most elegant but it will work. Also be aware that SQL DATETIMES before 1901 don't seem to work, so I've implemented this as a string, though with tweaking you can have it in any format. [code] <?php $leap = false; for( $i=1900; $i<2007; $i++ ) // Year number { if( ((year % 4 == 0) && (year % 100 != 0)) || (year % 400 == 0) ) { $leap = true; } for( $j=1; $j<13; $j++ ) // Month number { switch( $j ) { case 1: case 3: case 5: case 7: case 8: case 10: case 12: // 31-day months $days = 31; break; case 4: case 6: case 9: case 11: // 30-day months $days = 30; break; case 2: // Februray if( $leap ) { $days = 29; } else { $days = 28; } break; } for( $k=1; $k<=$days; $k++ ) { $date = $i . '-' . $j . '-' . $k; // Y-M-D format. $sql = "INSERT INTO date_table (date) VALUES ('$date')"; // // Use whatever DB technique you're using to send the query. // } } } ?> [/code] -
[quote author=brendandonhue link=topic=113297.msg460344#msg460344 date=1162263316] You could just do $array = glob('*.txt'); to get an array with the names of all the text files. You can use a loop like for() to display only the first 5. [/quote] Yeah, probably a better technique. Head like a sieve me... :D
-
NOT asking would be stupid. ;) What you need is a 'for loop' (read about them at [url=http://uk.php.net/manual/en/control-structures.for.php]http://uk.php.net/manual/en/control-structures.for.php[/url]) [code] for( $i=1; $i<=5; $i++ ) { echo $file_list[$i] . "\n" // print filename and a new-line } [/code] Be aware though that arrays are generally 0-indexed, meaning the first filename is at $file_list[0]. In that case change the for loop to [code] for ($i=0, $i<=4; $i++ ) [/code] There are simpler (better?) control structures like 'while' and 'foreach', but for something like this I'd use 'for'
-
Hmmmmm. It STILL bombs out with a div by zero error? It shouldn't as far as I can tell. Are you sure that's where the error is coming from?
-
Not sure what to say really :) It would seem the expression ($max-$old) is at some point zero, and thus creating the div. by zero error. Without knowing more about the system (what do those variables represent?) I'd suggest doing a check like [code] if( $max != $old ) { $percent = round((($rankp-$old)/($max-$old))*100); } else { ... handle the error ... } [/code]
-
This should do the trick! $dir is the path to the required directory. At the end, $file_list is an array of all filenames in the directory. [code] if($handle = opendir($dir)) { while(($file = readdir($handle)) !== false) { if( $file != "." && $file != ".." ) { $file_list[] = $file; } } closedir($handle); } [/code]
-
Do you mean list the filenames in an array or list the contents of the files in an array?
-
You could do something like: [code] $err_num = mysql_errno(); if( $err_num == 1217 ) // the number of the error you specified { echo "you can't delete this record, because there are some related records etc.."; } [/code] You can find a list of error codes at [url=http://dev.mysql.com/doc/refman/5.0/en/error-handling.html]http://dev.mysql.com/doc/refman/5.0/en/error-handling.html[/url] Also, if the query automatically echos errors (can't remember off the top of my head) you could prefix it with an @. Another technique might be to create an array of strings of the form $friendlySqlErr[1217] = "you can't delete this record, because there are some related records etc.."; possibly as a reusable library? Then have something like [code] $err_num = mysql_errno(); if( isset( $friendlySqlErr[$err_num] ) { echo $friendlySqlErr[$err_num]; } else { echo mysql_error(); } [/code]
-
Representing distant past/future datetimes...
bqallover replied to bqallover's topic in PHP Coding Help
*bump*. Anyone? -
It might be easier to store only the path to the file in the database, then store the images on your web server, then display them by retrieving filenames for your <img src>. If you [b]really[/b] want to store the images in the database, I think you need a BLOB field. Also, I expect you shouldn't use addslashes on a binary object like an image. Then to retrieve the image you would write a script called something like getimage.php and have variables like 'name' (e.g. getimage.php?name=spaceship.jpg. You could then have something like <img src="getimage.php?name=spaceship.jpg"> In that script you'd do a "SELECT bin_data FROM binary_data WHERE filename='$name'" ($name being a validated $_GET['name']. Then depending on the image type (jpg, gif) you'd output the correct headers followed by the 'bin_data'. (e.g. header('Content-Type: image/jpeg') ) Sorry it's a bit of a pseduo-answer, but hope it helps!
-
There are a few problems with that script. :) 1) The braces on the first while loop close a bit early again. 2) I [i]think[/i] you've got the syntax of the HEREDOC assignment a bit wrong, again if I read you right. To use HEREDOC when assigning to a variable you do something like... $variable = <<<END .... etc. 3) The SQL needs a bit of tidying up ;) Try this (or something very like it): [code] <? mysql_connect("localhost","username","password"); $query = "SELECT * from locations where converted= 'no'"; $result = mysql_db_query('blucat',$query); while($row = mysql_fetch_object($result)) { $display_company_name = "$row->company_name"; $display_postcode_district = "$row->postcode_district"; $display_job_number = "$row->job_number"; $display_job_date = "$row->job_date"; $query2 = "SELECT * from geolocations where postcode= '$display_postcode_district'"; $result2 = mysql_db_query('blucat',$query2); while($row = mysql_fetch_object($result2)) { $name = <<<END <font face="Tahoma" style="font-size: 8pt">$row->company_name<br /> $row->contact_name<br /> $row->town<br /> $row->postcode_district $row->postcode_sector<br /> T: $row->contact_number</font> END; /* Generates Insert Statement */ $query3 = "update locations set name='$name', lat='$row->latitude', lng='$row->longitude' where company_name='$display_company_name' and job_number='$display_job_number'"; mysql_db_query('blucat',$query3); } } /* Generates update converted statement */ $query4 = "UPDATE locations set converted='yes'"; $result4 = mysql_db_query('blucat',$query4); ?> [/code] Not sure how much help that will be, I'm not exactly sure what it's meant to do.
-
I think your problem lies in the position of the closing brace of the while loop. It should be lower - if I understand you correctly - like this... [code] <? mysql_connect("localhost","databse","password"); $query = "SELECT * from pda_jobs where converted= 'no'"; $result = mysql_db_query('blucat',$query); while($row = mysql_fetch_object($result)) { $display_company_name = "$row->company_name"; $display_postcode_district = "$row->postcode_district"; $display_postcode_sector = "$row->postcode_sector"; $display_job_number = "$row->job_number"; $display_job_date = "$row->job_date"; /* Generates Insert Statement */ $query1 = "insert into locations values( '', '', '', '', '$display_company_name', '$display_postcode_district', '$display_postcode_sector', '$display_job_number', '$display_job_date', 'no')"; $result1 = mysql_db_query('blucat',$query1); } $query3 = "UPDATE pda_jobs set converted='yes'"; $result3 = mysql_db_query('blucat',$query3); ?> [/code] Hope that's of some use. :)
-
You might want to take a look at this thread: [url=http://www.phpfreaks.com/forums/index.php/topic,112882.0.html]http://www.phpfreaks.com/forums/index.php/topic,112882.0.html[/url]
-
Getting values into variables in MySQL result field
bqallover replied to SoapLady's topic in PHP Coding Help
Maybe try something using eval? I'm not sure how this will work in your situation, but something like... [code] function __construct($firstname, $username, $password) { $php_code = '$this->htmlContent = "' . $this->mailText . '";'; eval( $php_code ); } [/code] $this->htmlContent should now be correct? Well, it MIGHT be. ;) -
Hi there. I think the following should also do the trick. [code] for( $i=1; $i<=100; $i++ ) { if( !isset($chk[$i]) ) { $chk[$i] = 0; } } [/code]
-
We've all made that miistake - no worries! It is strange that PHP didn't cough out more errors. I just quickly included your file in a command-line script and it just output the file contents. Oh well, who knows? :)
-
If that's a cut-and-paste of your code, can you spot the fatal typo on the very first line? ;)
-
You need to use regular expressions for this kind of thing. The following is untested but *should* work... [code] <?php $lines = file('data.txt'); // or whatever it's called foreach( $lines as $line ) { if( eregi( "([a-z0-9]+)\.level=([0-9]+)", $line, $regs ) ) { if( $regs[2] >= 3 ) { echo "$regs[1] = $regs[2]\n"; } } } ?> [/code]
-
Getting values into variables in MySQL result field
bqallover replied to SoapLady's topic in PHP Coding Help
Hmmm. It seems variable interpolation isn't being carried out on your returned database text. The following should work as a workaround, though it's untested. Obviously you can extend the arrays to include other variables you need. [code] function __construct($firstname, $username, $password) { $find = array('$firstname', '$username', '$password' ); $replace = array( $firstname, $username, $password ); $this->htmlContent = str_replace( $find, $replace, $this->mailText ); } [/code] Hope that helps! :) -
Hello! I'm currently working on a PHP/MySQL app that needs to be able to handle arbitrary dates, possibly WAY in the past or future - beyond the 1901-2038 of 32-bit unix time and the 1901-2155 of MySQL. I know that I can store the dates as strings and solve my problem that way, but then I miss out on the existing datetime functions. Does anyone know of a workaround to this, e.g. forcing PHP to use 64-bit timestamps?, etc. Or am I stuck with storing as strings? Thanks.
-
I'm working on a (messy!) gallery script at the moment and I've found that generating the thumbnails dynamically each time someone views the page is far too time-consuming. I'm using imagecopyresample, no imagecopyresize, so that explains the time-taking. My solution was to create a 'thumbs' directory in the gallery directory and when a file is uploaded, create the thumbnail then and put it in the thumbs directory. Then just 'img src' images in that directory with links to the high-res images. This is working well for me.