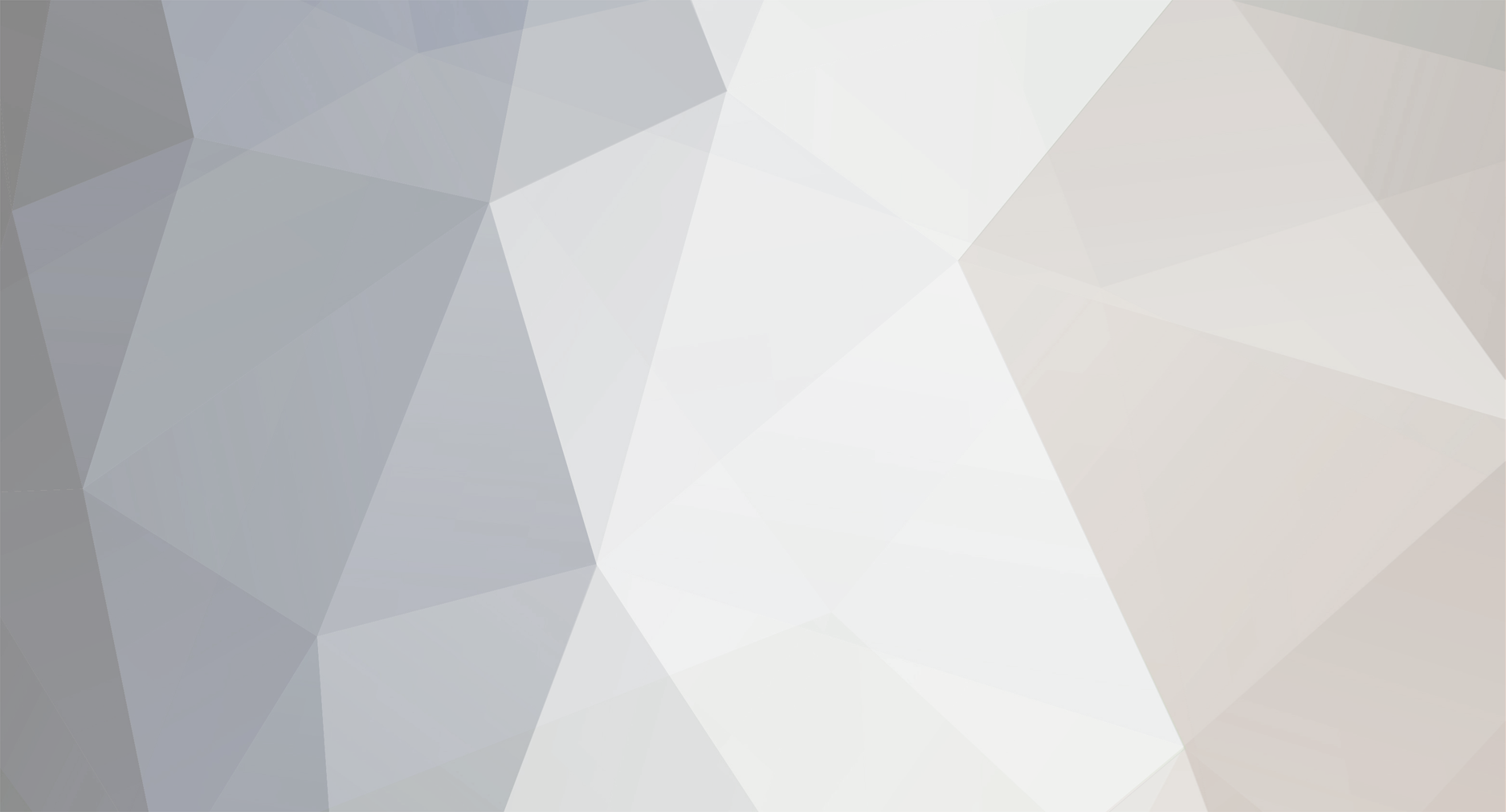
dbillings
Members-
Posts
190 -
Joined
-
Last visited
Never
Everything posted by dbillings
-
Are you using the text file as a type of database? i.e. do you have your heart set on the format you have. It's easier to break things up if you use some kind of repeatable pattern like below. sun glasses****20.00@@@@ Sun Glasses****11.00@@@@ Jeans****2.00@@@@
-
Say you have a form with 10 select lists. Is there a way to blur() all select boxes with one line of code? So replace... document.formname.selectName1.blur() document.formName.selectName2.blur() document.formName.selectName3.blur() etc... with... document.formName.something to refer to all select fields.blur() I'm not sure if that makes sense or if there is a short cut to blur all of one field type in a give form. Thanks for any help with this.
-
When I execute my create_form in my Form class it omits the first value of my $list array. It should print a select box with the options 1,2,3. Thanks for any help you can give. <?php #Form Class 6/20/08 #Last Edit class Form { var $data; var $input=array(); public function Form ($name, $id, $method, $action) { $this->data['name']= $name; $this->data['id']= $id; $this->data['method']= $method; $this->data['action']= $action; } public function add_Input($label, $name, $id, $type, $value) { $this->input[]= new Text($label, $name, $id, $type, $value); } public function add_Select($label, $name, $id, $option, $default) { $this->input[]= new Select($label, $name, $id, $option, $default); } public function create_Form() { foreach($this->input as $value){ if(get_class($value) == "Select"){ echo "<label>$value->label</label><select name='$value->name' id='$value->id'"; foreach($value->option as $option){ echo "<option value='$option'"; if($value->default == $option){ echo "selected='selected'"; } echo ">$option</option>"; } echo "</select>"; } if(get_class($value) == "Text"){ echo "<label>$value->label</label><input type='$value->type' id='$value->id name='$value->name' value='$value->value'>"; } } } } class Text extends Form { var $label; var $name; var $id; var $type; var $value; public function Text($label, $name, $id, $type, $value) { $this->label= $label; $this->name= $name; $this->id= $id; $this->type= $type; $this->value= $value; } } class Select extends Form { var $label; var $name; var $id; var $option= array(); var $default; public function Select($label, $name, $id, $option, $default) { $this->label= $label; $this->name= $name; $this->id= $id; $this->option= $option; $this->default= $default; } } $list= array(1,2,3); $registration_form= new Form("Name", "ID", "post", $_SERVER['PHP_SELF']); $registration_form->add_Input("Name", "name", "ID", "text","Name"); $registration_form->add_Input("Age", "age", "ID", "text","Age"); $registration_form->add_Select("Select", "test", "ID", $list, 1); $registration_form->create_Form(); ?>
-
Thankyou.
-
I'm setting up my class variables as an array ($text['label'], $text['type'] etc...). Then I'm creating the object in another class and saving the object to another array $input. When I loop through the $input array the object prints... <label>Array['label']</label><input type='Array['type']' id='Array['id]' name='Array['name']' value='Array['value']'><label>Array['label']</label><input type='Array['type']' id='Array['id]' name='Array['name']' value='Array['value']'> It doesn't seem to recognize my assciative indexs I created. Any ideas why? Here's the full code <?php #Form Class 6/20/08 #Last Edit class Form { var $data; var $input=array(); public function Form ($name, $id, $method, $action) { $this->data['name']= $name; $this->data['id']= $id; $this->data['method']= $method; $this->data['action']= $action; } public function add_Input($label, $name, $id, $type, $value) { $this->input[]= new Text($label, $name, $id, $type, $value); } public function create_Form() { foreach($this->input as $value){ echo "<label>$value->text['label']</label><input type='$value->text['type']' id='$value->text['id]' name='$value->text['name']' value='$value->text['value']'>"; } } } Class Text extends Form { var $text; public function Text($label, $name, $id, $type, $value) { $this->text['label']= $label; $this->text['name']= $name; $this->text['id']= $id; $this->text['type']= $type; $this->text['value']= $value; } } $registration_form= new Form("Name", "ID", "post", $_SERVER['PHP_SELF']); $registration_form->add_Input("Name", "name", "ID", "text","Name"); $registration_form->add_Input("Age", "age", "ID", "text","Age"); $registration_form->create_Form(); ?>
-
[SOLVED] Passing an array of objects as a parameter in method
dbillings replied to dbillings's topic in PHP Coding Help
Syntax Error! -
[SOLVED] Passing an array of objects as a parameter in method
dbillings replied to dbillings's topic in PHP Coding Help
Thank you for that Mastodont. I'm still having trouble though it's throwing this error, Fatal error: Cannot access empty property in C:\wamp\www\clan\Form.php on line 38 I added a comment showing line 38 <?php #Form Class 6/20/08 #Last Edit Class Text { var $text; public function Text($label, $name, $id, $type, $value='NULL') { $this->text['label']= $label; $this->text['name']= $name; $this->text['id']= $id; $this->text['type']= $type; if($value != 'NULL'){ $this->text['value']= $value; } } } class Form { var $data; var $input=array(); public function Form ($name, $id, $method, $action) { $this->data['name']= $name; $this->data['id']= $id; $this->data['method']= $method; $this->data['action']= $action; } public function add_Input($label, $name, $id, $type, $value='NULL') { $this->$input= new Text($label, $name, $id, $type, $value); // Line 38 } public function create_Form() { foreach($this->input as $value){ echo $value->text['name']; } } } $registration_form= new Form("Name", "ID", "post", $_SERVER['PHP_SELF']); $registration_form->add_Input("Name", "name", "ID", "text"); $registration_form->add_Input("Age", "age", "ID", "text"); $registration_form->create_Form(); ?> -
[SOLVED] Passing an array of objects as a parameter in method
dbillings replied to dbillings's topic in PHP Coding Help
I've modified the code a bit I added an addInput field to my form class and just created the new input object in the form class. I still can't get my loop to print any of my object data to the page. Help! <?php #Form Class 6/20/08 #Last Edit class Form { var $data; var $input=array(); public function Form ($name, $id, $method, $action) { $this->data['name']= $name; $this->data['id']= $id; $this->data['method']= $method; $this->data['action']= $action; } public function add_Input($label, $name, $id, $type, $value='NULL') { $input[]= new Text($label, $name, $id, $type, $value); } public function create_Form() { foreach($this->input as $value){ echo $value->text['name']; } } } Class Text extends Form { var $text; public function Text($label, $name, $id, $type, $value='NULL') { $this->text['label']= $label; $this->text['name']= $name; $this->text['id']= $id; $this->text['type']= $type; if($value != 'NULL'){ $this->text['value']= $value; } } } $registration_form= new Form("Name", "ID", "post", $_SERVER['PHP_SELF']); $registration_form->add_Input("Name", "name", "ID", "text"); $registration_form->add_Input("Age", "age", "ID", "text"); $registration_form->create_Form(); ?> -
Hi all, I'm trying to pass an array of objects as a parameter in a method. I keep receiving the error Fatal error: Cannot use object of type Text as array in C:\wamp\www\clan\Form.php on line 22 Any help would be appreciated. <?php #Form Class 6/20/08 #Last Edit class Form { var $data; public function Form ($name, $id, $method, $action) { $this->data['name']= $name; $this->data['id']= $id; $this->data['method']= $method; $this->data['action']= $action; } public function create_Form($inputs) { $i=0; while($inputs){ echo" <label>{$inputs[$i]->text['label']}</label><input type='{$inputs[$i]->text['type']}' name='{$inputs[$i]->text['name']}'><br /> "; $i++; } } } Class Text extends Form { var $text; public function Text($label, $name, $id, $type, $value='NULL') { $this->text['label']= $label; $this->text['name']= $name; $this->text['id']= $id; $this->text['type']= $type; if($value != 'NULL'){ $this->text['value']= $value; } } } $form_name= 'Name'; $form_id= 'ID'; $form_method= 'post'; $form_action= $_SERVER['PHP_SELF']; $input_label= 'Name'; $input_name= 'name'; $input_id= 'ID'; $input_type= 'text'; $name_input= array(); $input_label='Name2'; $input_name= 'name2'; $input_id='ID2'; $input_type='text'; $registration_form= new Form($form_name, $form_id, $form_method, $form_action); $name_input= new Text($input_label, $input_name, $input_id, $input_type); $name_input= new Text($input_label2, $input_name2, $input_id2, $input_type2); $registration_form->create_Form($name_input); ?>
-
Thanks Zanus I appreciate the help. I get the concept.
-
what do you mean f of n and g of n?
-
Does this make sense to anyone? Apparently the ... means that other statements are there they are just omitted, it's a head scratcher for me. Assuming that n is an integer argument, write a function to compute f(n) where f(n) = 1 + 2 + 3 + … + n Then, write a function to compute g(n) where g(n) = f(1) + f(2) + … f(n)
-
It's a setting that is adjustable in the php.ini configuration file.... ; Allow the <? tag. Otherwise, only <?php and <script> tags are recognized. ; NOTE: Using short tags should be avoided when developing applications or ; libraries that are meant for redistribution, or deployment on PHP ; servers which are not under your control, because short tags may not ; be supported on the target server. For portable, redistributable code, ; be sure not to use short tags. short_open_tag = Off
-
<?php $username = $_REQUEST['username']; $password = md5($_REQUEST['password']); $sql = "SELECT username, email FROM userdb WHERE username = '$username' AND password = '$password'"; $result = mysql_query('$sql') or die(mysql_error()); if($result) { while($row = mysql_fetch_array($result, MYSQL_ASSOC) { echo "$row['username'] <br />"; echo "$row['email'] <br />"; } }else{ echo "No data was retrieved."; } ?> Not tested.
-
I believe md5 is one way encryption.
-
<html> <head> <title></title> </head> <body> <?php $deck = array( "ac.gif", "2c.gif", "3c.gif", "4c.gif", "5c.gif", "6c.gif", "7c.gif", "8c.gif", "9c.gif", "tc.gif", "jc.gif", "qc.gif", "kc.gif", "ah.gif", "2h.gif", "3h.gif", "4h.gif", "5h.gif", "6h.gif", "7h.gif", "8h.gif", "9h.gif", "th.gif", "jh.gif", "qh.gif", "kh.gif", "as.gif", "2s.gif", "3s.gif", "4s.gif", "5s.gif", "6s.gif", "7s.gif", "8s.gif", "9s.gif", "ts.gif", "js.gif", "qs.gif", "ks.gif", "ad.gif", "2d.gif", "3d.gif", "4d.gif", "5d.gif", "6d.gif", "7d.gif", "8d.gif", "9d.gif", "td.gif", "jd.gif", "qd.gif", "kd.gif", ); echo "<table> <tr>"; for ( $a =0; $a <=7; $a++ ) { echo "</td><img src=\"../cards/" . $deck[$a] . "\"/><td>"; } echo "</tr> <tr>"; for ( $b =8; $b <=14; $b++ ) { echo "</td><img src=\"../cards/" . $deck[$b] . "\"/><td>"; } echo "</tr> <tr>"; for ( $c =15; $c <=21; $c++ ) { echo "</td><img src=\"../cards/" . $deck[$c] . "\"/><td>"; } echo "</tr> <tr>"; for ( $d =22; $d <=29; $d++ ) { echo "</td><img src=\"../cards/" . $deck[$d] . "\"/><td>"; } echo "</tr> <tr>"; for ( $e =30; $e <=34; $e++ ) { echo "</td><img src=\"../cards/" . $deck[$e] . "\"/><td>"; } echo "</tr> </table>"; ?> </body> </html>
-
The page now redirects via a javascript back to PHPFREAKS.
-
like the forum your in now? hit refresh and new posts appear.
-
maybe try exploding it? $pizza = "piece1 piece2 piece3 piece4 piece5 piece6"; $pieces = explode(" ", $pizza); sort($pieces);
-
<?php $color = "[n]".{$_REQUEST['color']}."[/n]"; $hex = "[c]".{$_REQUEST['hex']}."[/c]"; <form method='post' action='<?php $_SERVER['PHP_SELF']; ?>'> Color: <input type='text' name='color'><br /> Hex value: <input type='text' name='hex'><br /> <input type='submit' value='Submit'>
-
check out this link http://keithdevens.com/software/php_calendar
-
BTW that script is designed to be run on a server on my computer and uploaded to my hosted webserver. PHP will not resize an image before uploading because it is executed on the webserver itself. So you will still chew up bandwidth if you upload a monsterous digital image and it will take some time.
-
not perfect... <?php if(isset($_REQUEST['directory'])){ $thumbarray = array(); $imagearray = array(); $dir = $_REQUEST['directory']; ini_set('memory_limit', '-1'); $jpeg_check = array(); // Checks if at least one of the files processed successfuly. if(is_dir($dir)) { if ($dh = opendir($dir)){ while (($file = readdir($dh)) !== false) { if(filetype($dir . $file) == "file") { if(exif_imagetype($dir . $file) != IMAGETYPE_JPEG) { echo $dir . $file . " Resize failed not a JPEG image. <br />"; $jpeg_check[] = 'false'; }else{ $jpeg_check[] = 'true'; $tmpname = $dir.$file; $filesize = filesize($tmpname); echo $tmpname." Type: JPEG <br />"; $orig_image = imagecreatefromjpeg($tmpname); list($width, $height) = getimagesize($tmpname); if($filesize > 157286) { // Resize image if it's over 150 kb's. $percent = 157286 / $filesize; $newwidth = $width * $percent; $newheight = $height * $percent; $new_image = imagecreatetruecolor($newwidth, $newheight); imagecopyresampled($new_image, $orig_image, 0, 0, 0, 0,$newwidth, $newheight, $width, $height); } // Generate thumbnail images. $thumbw = 50; $thumbh = $thumbw / $width * $height; $thumb = imagecreatetruecolor($thumbw, $thumbh); imagecopyresampled($thumb, $orig_image, 0, 0, 0, 0, $thumbw, $thumbh, $width, $height); $imagename = microtime().".jpg"; imagejpeg($thumb, "C:/wamp/www/thumbs/".$imagename); imagejpeg($new_image, "C:/wamp/www/images/".$imagename); } }else{ echo "$dir.$file is not a file. <br />"; } } }else{ echo "could not open $dir. <br />"; } closedir($dh); }else{ echo "The submited directory is invalid. <br />"; } $dir = "C:/wamp/www/images/"; if(is_dir($dir)) { if ($dh = opendir($dir)){ while (($file = readdir($dh)) !== false) { if(filetype($dir . $file) == "file") { $ftp_server = "ftp.yourdomain.com"; $ftp_username = "yourusername"; $ftp_user_pass = "yourpassword"; $source_file = $dir . $file; $destination_file = "images/{$file}"; $conn_id = ftp_connect($ftp_server) or die("Couldn't connect to $ftp_server"); $login_result = ftp_login ($conn_id, $ftp_username, $ftp_user_pass); ftp_pasv($conn_id, true); if((!$conn_id || !$login_result)) { echo "FTP connection has failed."; echo "Attempted to connect to $ftp_server for $ftp_username."; exit(); }else{ echo "Connected to FTP server for $ftp_username."; } $upload = ftp_put ($conn_id, $destination_file, $source_file, FTP_BINARY); if(!$upload){ echo "FTP upload has failed."; }else{ echo "Uploaded $source_file to $ftp_server as $destination_file"; } ftp_close($conn_id); } } } }else{ echo "No JPEG images were detected for FTP upload."; } $dir = "C:/wamp/www/thumbs/"; if(is_dir($dir)) { if ($dh = opendir($dir)){ while (($file = readdir($dh)) !== false) { if(filetype($dir . $file) == "file") { $ftp_server = "ftp.yourdomain.com"; $ftp_username = "yourusername"; $ftp_user_pass = "yourpassword"; $source_file = $dir . $file; $destination_file = "thumbs/{$file}"; $conn_id = ftp_connect($ftp_server) or die("Couldn't connect to $ftp_server"); $login_result = ftp_login ($conn_id, $ftp_username, $ftp_user_pass); ftp_pasv($conn_id, true); if((!$conn_id || !$login_result)) { echo "FTP connection has failed."; echo "Attempted to connect to $ftp_server for $ftp_username."; exit(); }else{ echo "Connected to FTP server for $ftp_username."; } $upload = ftp_put ($conn_id, $destination_file, $source_file, FTP_BINARY); if(!$upload){ echo "FTP upload has failed."; }else{ echo "Uploaded $source_file to $ftp_server as $destination_file"; } ftp_close($conn_id); } } } }else{ echo "No JPEG thumbs were detected for FTP upload."; } } ?> <div>Format C:/upload/ </div> <form method="post" action="<?php $_SERVER['PHP_SELF']; ?>"> Directory<input type="text" name="directory"><br /> <input type="submit" name="submit" value="Submit"> </form>