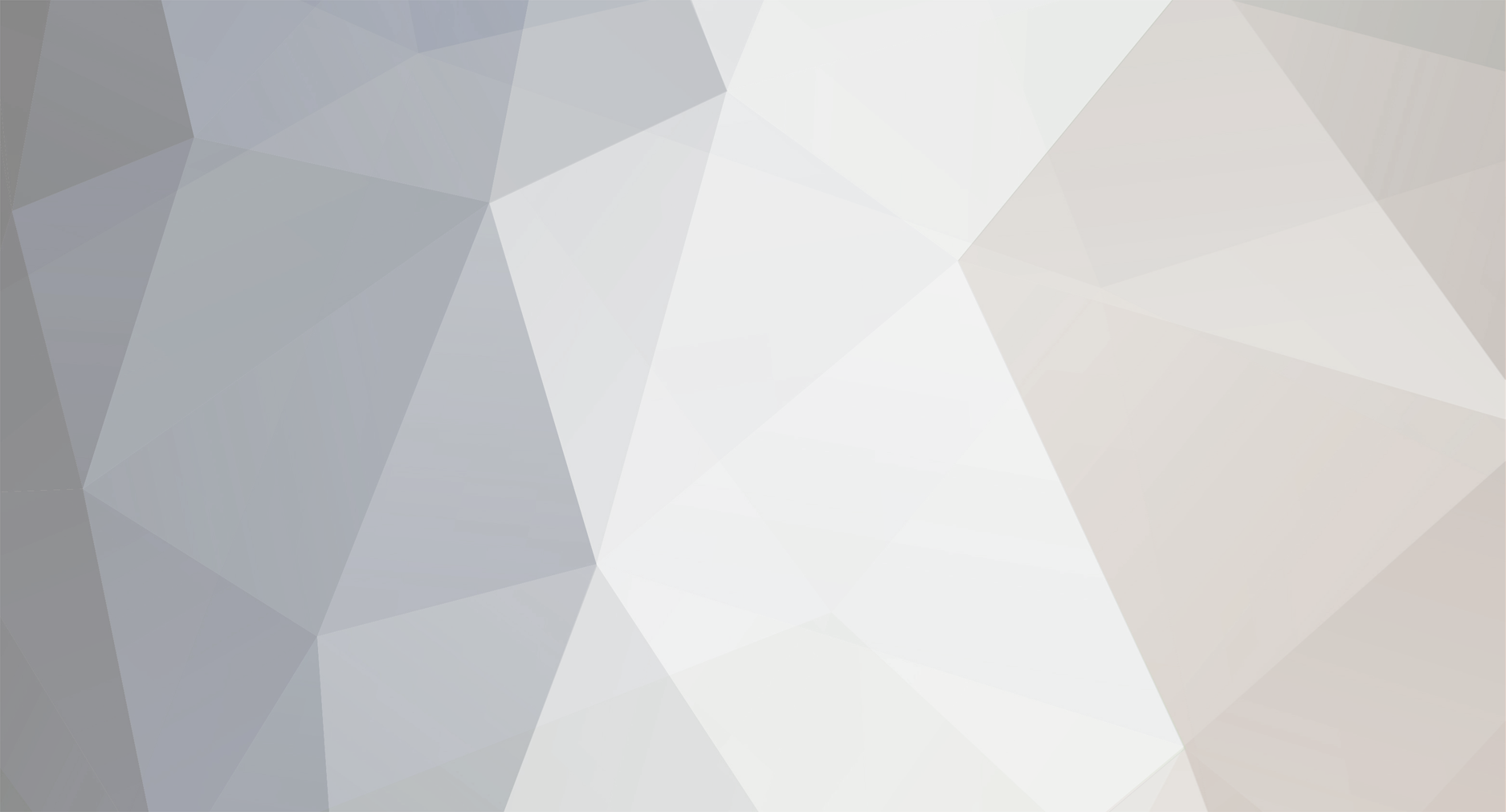
jarvis
-
Posts
543 -
Joined
-
Last visited
Posts posted by jarvis
-
-
Hi PFMaBiSmAd
What will those lines of code show? Also, that is an error on my part, the logout script shouldn't used first_name but name.
As for the new password on signout, this is something that's required. The idea is that when they logout, the password is reset & the member has to request a new log in. Something the client wanted!
Thanks
-
Ok, here's 4 scripts:
- login
- a secure page
- the header include
- logout
Login
*****
<?php // This is the login page for the site. // Include the configuration file for error management and such. require_once ('./includes/config.inc.php'); // Set the page title and include the HTML header. $page_title = 'Login'; include ('./includes/header.html'); if (isset($_POST['submitted'])) { // Check if the form has been submitted. require_once ('../mysql_connect.php'); // Connect to the database. // Validate the email address. if (!empty($_POST['email'])) { $e = escape_data($_POST['email']); } else { echo '<p class="error">You forgot to enter your email address!</p>'; $e = FALSE; } // Validate the password. if (!empty($_POST['pass'])) { $p = escape_data($_POST['pass']); } else { $p = FALSE; echo '<p class="error">You forgot to enter your password!</p>'; } if ($e && $p) { // If everything's OK. // Query the database. $query = "SELECT user_id, name, acc_type FROM users WHERE (email='$e' AND pass=SHA('$p')) AND active IS NULL"; $result = mysql_query ($query) or trigger_error("Query: $query\n<br />MySQL Error: " . mysql_error()); if (@mysql_num_rows($result) == 1) { // A match was made. // Register the values & redirect. $row = mysql_fetch_array ($result, MYSQL_NUM); mysql_free_result($result); mysql_close(); // Close the database connection. $_SESSION['user_id'] = $row[0]; $_SESSION['name'] = $row[1]; $_SESSION['acc_type'] = $row[2]; // Start defining the URL. $url = 'http://' . $_SERVER['HTTP_HOST'] . dirname($_SERVER['PHP_SELF']); // Check for a trailing slash. if ((substr($url, -1) == '/') OR (substr($url, -1) == '\\') ) { $url = substr ($url, 0, -1); // Chop off the slash. } // Add the page. $url .= '/category.php'; ob_end_clean(); // Delete the buffer. header("Location: $url"); exit(); // Quit the script. } else { // No match was made. echo '<p class="error">Either the email address and password entered do not match those on file or you have not yet activated your account.</p>'; } } else { // If everything wasn't OK. echo '<p class="error">Please try again.</p>'; } mysql_close(); // Close the database connection. } // End of SUBMIT conditional. ?>
Secure page
***********
<?php // This page allows users to add categories to the database. // Set the page title and include the HTML header. $page_title = 'Add a Category'; include ('./includes/header.html'); include('saveThumbCategories.php'); // If no first_name variable exists, redirect the user. if (!isset($_SESSION['name'])) { // Start defining the URL. $url = 'http://' . $_SERVER['HTTP_HOST'] . dirname($_SERVER['PHP_SELF']); // Check for a trailing slash. if ((substr($url, -1) == '/') OR (substr($url, -1) == '\\') ) { $url = substr ($url, 0, -1); // Chop off the slash. } // Add the page. $url .= '/index.php'; ob_end_clean(); // Delete the buffer. header("Location: $url"); exit(); // Quit the script. } else { ?>
The header include has
**********************
<?php // This page begins the HTML header for the site. // Start output buffering. ob_start(); // Initialize a session. session_start(); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head>
Logout
******
<?php // This is the logout page for the site. // Include the configuration file for error management and such. require_once ('./includes/config.inc.php'); // Set the page title and include the HTML header. $page_title = 'Logout'; include ('./includes/header.html'); // If no first_name variable exists, redirect the user. if (!isset($_SESSION['first_name'])) { // Start defining the URL. $url = 'http://' . $_SERVER['HTTP_HOST'] . dirname($_SERVER['PHP_SELF']); // Check for a trailing slash. if ((substr($url, -1) == '/') OR (substr($url, -1) == '\\') ) { $url = substr ($url, 0, -1); // Chop off the slash. } // Add the page. $url .= '/index.php'; ob_end_clean(); // Delete the buffer. header("Location: $url"); exit(); // Quit the script. } else { // Logout the user. $user_id = $_SESSION['user_id']; require_once('../mysql_connect.php');// Connect to the db // Create a new, random password. $p = substr ( md5(uniqid(rand(),1)), 3, 10); // Make the query. $query = "UPDATE users SET pass=SHA('$p') WHERE user_id=$user_id"; $result = mysql_query ($query) or trigger_error("Query: $query\n<br />MySQL Error: " . mysql_error()); if (mysql_affected_rows() == 1) { // If it ran OK. #echo 'pw reset'; } // Send an email. $body = "Your password to log into Lemon Ribbon has been temporarily changed to '$p'. Please log in using this password and your username. At that time you may change your password to something more familiar."; #mail ($_POST['email'], 'Your temporary password.', $body, 'From: admin@sitename.com'); mail ('test@test.co.uk', 'Your temporary password.', $body, 'From: admin@sitename.com'); echo '<h3>Your password has been changed. You will receive the new, temporary password at the email address with which you registered. Once you have logged in with this password, you may change it by clicking on the "Change Password" link.</h3>'; mysql_close(); // Close the database connection. $_SESSION = array(); // Destroy the variables. session_destroy(); // Destroy the session itself. setcookie (session_name(), '', time()-300, '/', '', 0); // Destroy the cookie. } // Print a customized message. echo "<h3>You are now logged out.</h3>"; include ('./includes/footer.html'); ?>
Does that help at all? Thank you for your time & assistance on this, it's very much appreciated!
-
Sorry, the code on the pages is like:
// If no first_name variable exists, redirect the user. if (!isset($_SESSION['name'])) { // Start defining the URL. $url = 'http://' . $_SERVER['HTTP_HOST'] . dirname($_SERVER['PHP_SELF']); // Check for a trailing slash. if ((substr($url, -1) == '/') OR (substr($url, -1) == '\\') ) { $url = substr ($url, 0, -1); // Chop off the slash. } // Add the page. $url .= '/index.php'; ob_end_clean(); // Delete the buffer. header("Location: $url"); exit(); // Quit the script. } else { //run the script...
Some pages use an id as well depending on what rights the user has.
THANKS
-
Hi MadTechie,
They do still have there rights. The code on my login page is
// Query the database. $query = "SELECT user_id, name, acc_type FROM users WHERE (email='$e' AND pass=SHA('$p')) AND active IS NULL"; $result = mysql_query ($query) or trigger_error("Query: $query\n<br />MySQL Error: " . mysql_error()); if (@mysql_num_rows($result) == 1) { // A match was made. // Register the values & redirect. $row = mysql_fetch_array ($result, MYSQL_NUM); mysql_free_result($result); mysql_close(); // Close the database connection. $_SESSION['user_id'] = $row[0]; $_SESSION['name'] = $row[1]; $_SESSION['acc_type'] = $row[2]; // Start defining the URL. $url = 'http://' . $_SERVER['HTTP_HOST'] . dirname($_SERVER['PHP_SELF']); // Check for a trailing slash. if ((substr($url, -1) == '/') OR (substr($url, -1) == '\\') ) { $url = substr ($url, 0, -1); // Chop off the slash. } // Add the page. $url .= '/category.php'; ob_end_clean(); // Delete the buffer. header("Location: $url"); exit(); // Quit the script.
Does that help?
-
Hi All,
I've got a cms that members can log into. When they logout, the session is destroyed, however, if you click the back button, you can get back into the CMS.
How can I get around this?
My logout code has
$_SESSION = array(); // Destroy the variables. session_destroy(); // Destroy the session itself. setcookie (session_name(), '', time()-300, '/', '', 0); // Destroy the cookie.
I've also tried adding this to my header file
// HTTP/1.1 header("cache-Control: no-store, no-cache, must-revalidate"); header("cache-Control: post-check=0, pre-check=0", false); // HTTP/1.0 header("Pragma: no-cache"); // Date in the past header("Expires: Mon, 26 Jul 1997 05:00:00 GMT"); // always modified header("Last-Modified: " . gmdate("D, d M Y H:i:s") . " GMT"); // This page begins the HTML header for the site. // Start output buffering. ob_start(); // Initialize a session. session_start();
Am i doing something wrong?
thanks
-
Hi guys,
I really hope someone can help. I've got the following 2 scripts. The first handles my resizing and the second adds a watermark:
<?php function saveThumb($gal,$fn) { define("MAX_XY_SMALL", 120); // maximum width or height of thumbnail image define("MAX_XY_LARGE", 800); // maximum width or height of thumbnail image $fpath = "categories/$gal/$fn"; //print $fpath."\n"; /* GetImageSize returns an array: * $info[0] - horizontal size of image in pixels * $info[1] - vertical size of image in pixels * $info[2] - type of image (1=GIF, 2=JPEG, 3=PNG) */ $info = GetImageSize("$fpath"); //print_r($info); // do we need to resize image? if ($info[0] > MAX_XY_SMALL || $info[1] > MAX_XY_SMALL) { // is image landscape? if ($info[0] >= $info[1]) { $width = MAX_XY_SMALL; $height = $info[1]*MAX_XY_SMALL/$info[0]; // or portrait? } else { $height = MAX_XY_SMALL; $width = $info[0]*MAX_XY_SMALL/$info[1]; } } else { // use original dimensions $width = $info[0]; $height = $info[1]; } if ($info[0] > MAX_XY_LARGE || $info[1] > MAX_XY_LARGE) { // is image landscape? if ($info[0] >= $info[1]) { $width_large = MAX_XY_LARGE; $height_large = $info[1]*MAX_XY_LARGE/$info[0]; // or portrait? } else { $height_large = MAX_XY_LARGE; $width_large = $info[0]*MAX_XY_LARGE/$info[1]; } } else { // use original dimensions $width_large = $info[0]; $height_large = $info[1]; } // create new thumbnail image //echo "<br>$width - $height<br>"; $im = ImageCreateTrueColor($width, $height); $im_large = ImageCreateTrueColor($width_large, $height_large); /* determine image type and load original image. Notice new tests for image * types. The GD extension has changed a lot over the years, dropping GIFs * and adding PNG support. By testing, we avoid trying to process an image * PHP can't deal with */ $im_big = ""; // default is no image switch ($info[2]) { case 1 : if (ImageTypes() & IMG_GIF) // test for GIF support $im_big = ImageCreateFromGIF("$fpath"); break; case 2 : if (ImageTypes() & IMG_JPG) // test for JPEG support $im_big = ImageCreateFromJPEG("$fpath"); break; case 3 : if (ImageTypes() & IMG_PNG) // test for PNG support $im_big = ImageCreateFromPNG("$fpath"); break; case 4 : if (ImageTypes() & IMG_BMP) // test for BMP support $im_big = ImageCreateFromBMP("$fpath"); break; } if ($im_big) { /* resize original image into thumbnail - see PHP Manual for details on * the arguements ImageCopyResized takes */ ImageCopyResized($im,$im_big,0,0,0,0,$width,$height,$info[0],$info[1]); ImageCopyResized($im_large,$im_big,0,0,0,0,$width_large,$height_large,$info[0],$info[1]); } else { // couldn't load original image, so generate 'no thumbnail' image instead $width = 150; $height = 150; $im = ImageCreate($width, $height); // create a blank image $bgc = ImageColorAllocate($im, 0, 0, 0); // background color = black $tc = ImageColorAllocate($im, 255, 255, 255); // text color = white ImageFilledRectangle($im, 0, 0, $width, $height, $bgc); // draw rectangle ImageString($im, 3, 9, 36, "No thumbnail", $tc); // add text ImageString($im, 3, 17, 48, "available", $tc); } /* save our image in thumb directory - if the second argument is left out, * the image gets sent to the browser, as in thumbnail.php */ ImageJPEG($im, "categories/".$gal."/t_".$fn); ImageJPEG($im_large, "categories/".$gal."/".$fn); // clean up our working images ImageDestroy($im_big); ImageDestroy($im); ImageDestroy($im_large); } ?>
The below is my watermark script
$imagesource = $_GET['path']; $filetype = substr($imagesource,strlen($imagesource)-4,4); $filetype = strtolower($filetype); if($filetype == ".gif") $image = @imagecreatefromgif($imagesource); if($filetype == ".jpg") $image = @imagecreatefromjpeg($imagesource); if($filetype == ".png") $image = @imagecreatefrompng($imagesource); if (!$image) die(); $watermark = @imagecreatefromgif('watermark.gif'); $imagewidth = imagesx($image); $imageheight = imagesy($image); $watermarkwidth = imagesx($watermark); $watermarkheight = imagesy($watermark); $startwidth = (($imagewidth - $watermarkwidth)/2); $startheight = (($imageheight - $watermarkheight)/2); imagecopy($image, $watermark, $startwidth, $startheight, 0, 0, $watermarkwidth, $watermarkheight); imagejpeg($image); imagedestroy($image); imagedestroy($watermark);
Is there a way to combine the 2 so that the watermark is added to the larger resized file. Also, I notice my watermark script isn't writing the watermark to the image, it simply adds it on the screen over the top, meaning you can download the image without problems. How can I sort this too?
Thanks sooo much!
-
Hi all,
Ok I've worked out my above query but I do have another - sorry!
At the mo the links are:
jan - feb
mar - apr
etc
What I need to do is go dec (08) - Jan (09)
feb - mar
apr - may
I need to start one month back in theory, so the year end link is oct-nov then starts dec 09 - jan 10
I dont need to show the year, it's just to illustrate this
Thanks again, all help is much appreciated!!
-
thanks again darkvengance, i'll go take a look!
Cheers
-
Thanks darkvengance that's very kind!
Although it doesn't check for image types and looks like it doesn't generate 2 images. I need the thumb & the original but need to resize both. Maybe even copy the original too (just incase!)
Thanks
-
Thanks Sasa, that's awesome! I've been going mad trying to suss that one out!
I've one question though, in my old script, I used the month to pass it into my query. with the following code
// Set the sorting order by months if (isset($_GET['month'])) { // $month will be appended to the links $month = $_GET['month']; } else { // Set a default sorting month to current month $month= date('F'); }
Then passed that into my query with
AND DATE_FORMAT(articles.date_entered,'%M') = '$month'
As the link now shows Nov-Dec, I noticed when you hover over it, month it set to Nov, or the first month out the pair, i.e. sept-oct the link is sept. How can I also grab the oct and pass it into the query?
The reason I ask, is if the articles were added in oct not sept, then no articles will show for the sept-oct edition. I hope that makes sense?
-
Hi all,
I've got the following script which nicely creates my thumbnail at 120px high or wide. Rather than copy the original size image as well, I'd like the original resized to 800x600
I cannot see the best way to do this, so any help is much appreciated!
Here's my code.
function saveThumb($gal,$fn) { define("MAX_XY", 120); // maximum width or height of thumbnail image $fpath = "categories/$gal/$fn"; $info = GetImageSize("$fpath"); // do we need to resize image? if ($info[0] > MAX_XY || $info[1] > MAX_XY) { // is image landscape? if ($info[0] >= $info[1]) { $width = MAX_XY; $height = $info[1]*MAX_XY/$info[0]; // or portrait? } else { $height = MAX_XY; $width = $info[0]*MAX_XY/$info[1]; } } else { // use original dimensions $width = $info[0]; $height = $info[1]; } // create new thumbnail image $im = ImageCreateTrueColor($width, $height); $im_big = ""; // default is no image switch ($info[2]) { case 1 : if (ImageTypes() & IMG_GIF) // test for GIF support $im_big = ImageCreateFromGIF("$fpath"); break; case 2 : if (ImageTypes() & IMG_JPG) // test for JPEG support $im_big = ImageCreateFromJPEG("$fpath"); break; case 3 : if (ImageTypes() & IMG_PNG) // test for PNG support $im_big = ImageCreateFromPNG("$fpath"); break; case 4 : if (ImageTypes() & IMG_BMP) // test for BMP support $im_big = ImageCreateFromBMP("$fpath"); break; } if ($im_big) { /* resize original image into thumbnail - see PHP Manual for details on * the arguements ImageCopyResized takes */ ImageCopyResized($im,$im_big,0,0,0,0,$width,$height,$info[0],$info[1]); } else { // couldn't load original image, so generate 'no thumbnail' image instead $width = 120; $height = 120; $im = ImageCreate($width, $height); // create a blank image $bgc = ImageColorAllocate($im, 0, 0, 0); // background color = black $tc = ImageColorAllocate($im, 255, 255, 255); // text color = white ImageFilledRectangle($im, 0, 0, $width, $height, $bgc); // draw rectangle ImageString($im, 3, 9, 36, "No thumbnail", $tc); // add text ImageString($im, 3, 17, 48, "available", $tc); } /* save our image in thumb directory - if the second argument is left out, * the image gets sent to the browser, as in thumbnail.php */ ImageJPEG($im, "categories/".$gal."/t_".$fn); // clean up our working images ImageDestroy($im_big); ImageDestroy($im); }
Thanks in advanced!
-
Hi all,
Am going mad trying to get something to work.
I'm creating a CMS for a magazine. The magazine is published every 2 months and therefore the edition is nov-dec or jan-feb etc.
I've an archive option on the site and rather than listing:
dec
nov
oct
sept etc
I'd like to follow the magazine editions:
nov-dec
sept-oct
The code I have so far is:
echo '<p>Sort By:</p>'; $year = date("Y"); //get the current year $startDate = "1 january".$year; // set the end date to current year function printMonths($var) { $start = strtotime($var); //timestamp of the entered date $now = strtotime("Now"); //timestamp of now so it does not write anything in the future while ($now > $start) //while the start is less than now { echo '<a href="news_archive.php?s=&id=' . get_id() . '&month=' . date("F", $now) .'">'.date("F", $now).'</a>'; echo " | "; $now = strtotime("-1 month", $now); // subtract a month from the start timestamp } } printMonths($startDate); //execute the function
this works well listing the months from the current month back. But this is not what I need. If I alter the line
$now = strtotime("-1 month", $now);
to
$now = strtotime("-2 month", $now);
It starts in Nov and goes back to sept which is not right. It also doesn't show nov-dec etc.
Any help on this is much appreciated!
Thanks
-
Thanx bricktop, of course!
I was working on the theory that as this was the edit page, you needed to retrieve the value and also display it!
I'll go give that a bash!
-
Hi All,
Probably very simple but; I've got fckeditor installed on a CMS, whenever you add an image or a link, it saves the info twice and seperate the entire duplicate content with
Quote:
" />
It's as if it's not closing a tag. Has anyone else had this or knows how to resolve it?
Just as a subnote:
My line of code is:
Code:
<td><textarea rows="20" name="content" cols="60" value="' . $row[4] . '" />' . $row[4] . '</textarea></td>
The duplicate " /> is coming in from the above as I've removed the space in the above line so it's just "/> and this is then what appears in the duplicate text.
Hmmm....
-
Thanks cags & apologies!
Will try & get that working
-
Thanks cags,
I can't seem to get that working though:
<?php // Create category options $query = "SELECT * FROM categories ORDER BY category ASC"; $column_count = 5; $row_count = ceil(mysql_num_rows($query) / $column_count); echo '<table'; for($r = 0; $r < $row_count; ++$r) { // start row echo '<tr>'; for($c = 0; $c < $column_count; ++$c) { if(mysql_fetch_array($result, MYSQL_NUM)) { echo '<td><input type="checkbox" name="types[]" value="' . $row[1] . '" />' .$row[1] . '</td>'; } else { echo '<td> </td>'; } } // end row echo '</tr>'; } echo '</table>'; ?>
Gives the error:
Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in C:\Program Files\Apache Group\Apache2\htdocs\lemon_ribbon\web\register.php on line 159Line 159:
$row_count = ceil(mysql_num_rows($query) / $column_count);
Sorry to sound thick!
-
Hi all,
I've the following code
<?php // Create category options $query = "SELECT * FROM categories ORDER BY category ASC"; $result = mysql_query ($query); while ($row = mysql_fetch_array ($result, MYSQL_NUM)) { echo "<input type=\"checkbox\" name=\"types[]\" value=\"$row[1]\">$row[1]\n"; } ?>
This lists out up to 15 checkboxes, but can increase or decrease if more are added/removed. At present this simply produces a list.
How can I alter the above to produce a nice table with say 5 checkboxes per row?
Thanks
-
Ah, good point! Could that be hidden and included as a php include? That way it's only see server side?
-
Could I use:
<META HTTP-EQUIV="Refresh" content="600;url=http://mydomain.com/logout.php">
If I put this in the header, surely after 600 seconds it will redirect to my log out script?
Or is this not deemed acceptable?
-
Sorry to bring this one back up but I'm still having issues.
In a nutshell, I need to kill my session, without the need of a page refresh after a set time period. It's not after inactivity, the site can be in use by someone but after say 2 hours will just end.
Upon killing the session, I need to run a php script.
is this possible?
do i need to add the code to each page or just to my header?
please see above for how I set up my session and the code used.
thanks in advanced!
-
Wicked! Thanks very much!! Simple when you know how
-
Hi all,
OK, I've found this code which works well to time out a session without inactivity
session_start(); //set timeout period in seconds $inactive = 30; // check to see if $_SESSION['timeout'] is set if(isset($_SESSION['timeout']) ) { $session_life = time() - $_SESSION['timeout']; if($session_life > $inactive) { session_destroy(); header("Location: logout.php"); } } $_SESSION['timeout'] = time();
How can I set it to auto expire after a set time? i've tried
ini_set('session.gc_maxlifetime', 15);
but that doesn't work. I placed that at the top of all pages. Any help is much apprciated!
-
Hi All,
Ok I've the following code to handle my image upload:
// Check for an image. if (is_uploaded_file ($_FILES['image']['tmp_name'])) { // Relocate the image if (move_uploaded_file($_FILES['image']['tmp_name'], 'categories/'.$_POST['id'].'/'.$_FILES['image']['name'])) { saveThumb($_POST['id'],$_FILES['image']['name']); #$insert_sql = 'INSERT INTO uploads values("",'.$_POST['id'].', "'.$_FILES['image']['name'].'", "'.$_FILES['image']['size'].'", "'.$_FILES['image']['type'].'", "'.$_POST['image_description'].'", NOW())'; $insert_sql = 'INSERT INTO uploads values(NULL,'.$_POST['category'].', "'.$_FILES['image']['name'].'", "'.$_FILES['image']['size'].'", "'.$_FILES['image']['type'].'", "'.$_POST['image_description'].'", NOW())'; echo $insert_sql; $insert_rs = mysql_query($insert_sql) or die(mysql_error()); echo '<p class="error">The image has been uploaded!</p>'; } else { // Inform user if problem relocating image echo '<p class="error">The file could not be Uploaded!</p>'; } } else { echo '<p class="error">You must also select an image to upload!</p>'; }
How can i stop the same file being uploaded twice? I figure the easiest way is to rename the file when uploading, how can I do this with the above code?
Thanks in advanced!
-
Thanks GiriJ, that's more what I'm after. Would that be a session of 10 mins overall or per page if they remain on one page?
Do I add that on each page?
If this is an overall 10 mins, this is perfect as I can increase it to 2 hours. How could I then add in my pw reset?
This is my code to create a random pw
// Create a new, random password. $p = substr ( md5(uniqid(rand(),1)), 3, 10); // Make the query. $query = "UPDATE users SET pass=SHA('$p') WHERE user_id=$uid"; $result = mysql_query ($query) or trigger_error("Query: $query\n<br />MySQL Error: " . mysql_error()); if (mysql_affected_rows() == 1) { // If it ran OK. // Send an email. $body = "Your password to log into domain.co.uk has been temporarily changed to '$p'. Please log in using this password and your username. At that time you may change your password to something more familiar."; mail ($_POST['email'], 'Your temporary password.', $body, 'From: admin@domain.co.uk'); echo '<h3>Your password has been changed. You will receive the new, temporary password at the email address with which you registered. Once you have logged in with this password, you may change it by clicking on the "Change Password" link.</h3>'; mysql_close(); // Close the database connection. include ('./includes/footer.html'); // Include the HTML footer. exit(); }
I can pull the username from the session I guess?
Thanks again!
php sessions,logouts & the bloomin back button!
in PHP Coding Help
Posted
Hi,
It was the first_name not name issue in the logout script! Thank you so much!