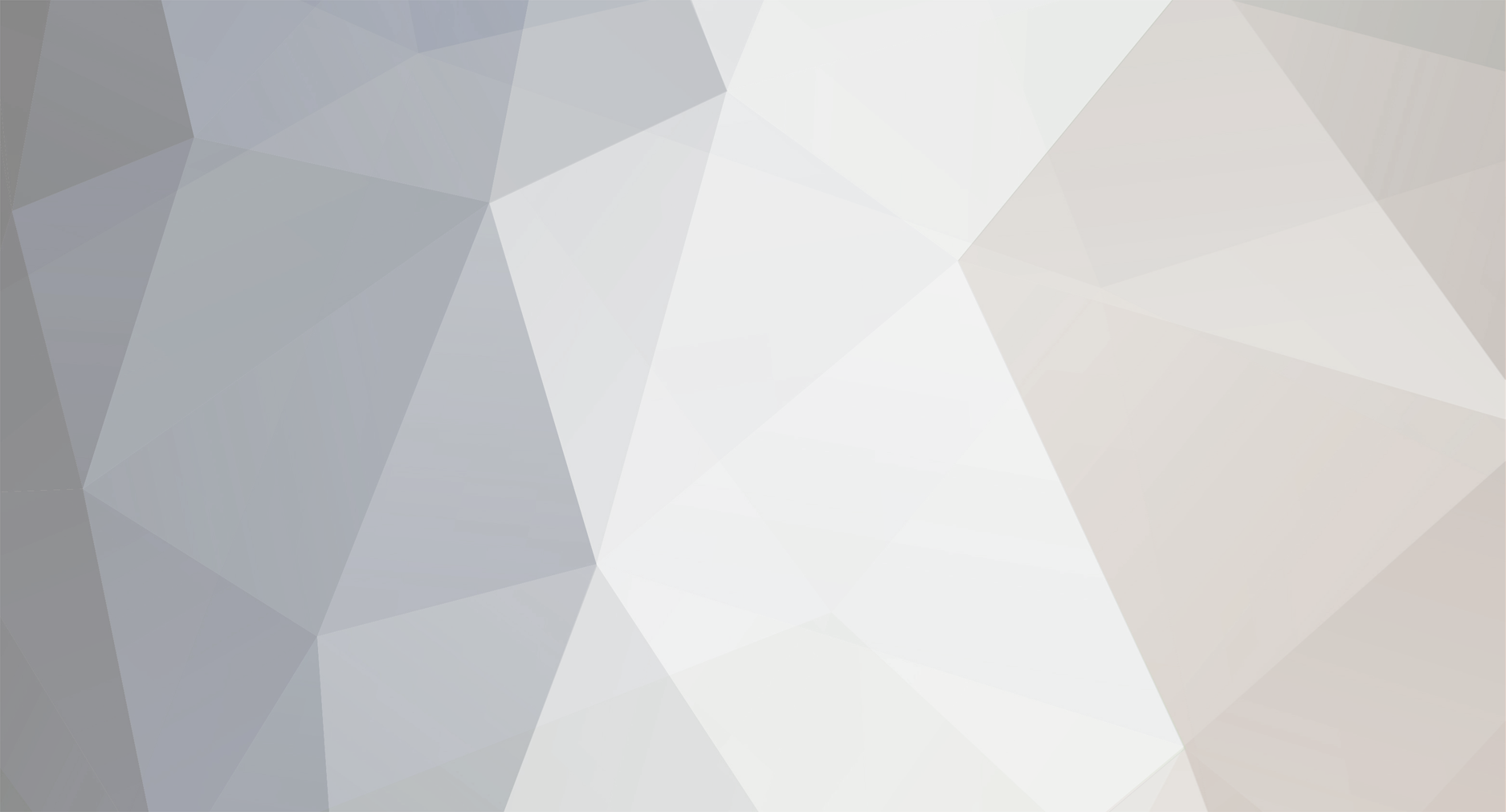
KrisNz
Members-
Posts
271 -
Joined
-
Last visited
Everything posted by KrisNz
-
What errors does it come up with? On What line(s)?
-
Yeah, if you want to write games, php is not the way to go. The easiest place to start would be to learn vb.net. Theres a few books on learning vb.net through developing games so you should try one of those.
-
If you want to keep your code separate from your template then you shouldn't have php in it. Use placeholders in your template for the pieces of the email that are going to change. e.g [code] #template.html Dear %NAME%, thanks for registering with %SITENAME% #code $personsName = "Bob"; $siteName = "foo.bar"; $body = file_get_contents("template.html"); $body = str_replace("%NAME%",$personsName,$body); $body = str_replace("%SITENAME%",$siteName,$body); [/code]
-
[code] #i took this function of php.net/array_combine #if you're using php5 you can just call array_combine function array_combine_emulated( $keys, $vals ) { $keys = array_values( (array) $keys ); $vals = array_values( (array) $vals ); $n = max( count( $keys ), count( $vals ) ); $r = array(); for( $i=0; $i<$n; $i++ ) { $r[ $keys[ $i ] ] = $vals[ $i ]; } return $r; } $testArray = array("10","12","13","34","15"); $keys = array_values(($testArray)); $newArray = array_combine_emulated($keys,$testArray); print_r($newArray); # prints Array ( [10] => 10 [12] => 12 [13] => 13 [34] => 34 [15] => 15 ) [/code]
-
Are you on a windows OS?
-
For starters, your form has some issues, your checkboxes dont have any values, when you use checkboxes to allow for multiple values you have to name them as an array like so [code]I am well respected:<input name="iam[]" value="respected" type="checkbox" /><br /> I am well renowned:<input name="iam[]" value="renowned" type="checkbox" /><br /> I am very short:<input name="iam[]" value="short" type="checkbox" /><br /> etc etc... [/code] Secondly, [!--quoteo--][div class=\'quotetop\']QUOTE[/div][div class=\'quotemain\'][!--quotec--]$recipient = $_POST['businessman332211@hotmail.com']; $subject = $_POST['This is from my site'];[/quote] you set these variables incorrectly, they aren't posted from a form, just set them yourself. Your code says "set the recipient variable to the value contained in the $_POST array at the index of 'businessman332211@hotmail.com' . [code] $recipient = 'businessman332211@hotmail.com'; [/code] have you looked at the method signature for php's mail function? Or for that matter the help page for php's mail function? You cant just pass it some random variables and expect it to send an email. In your code you dont seem to be passing in an email address, a subject, a message, any extra headers or any extra parameters. Perhaps you should take a few steps back, you're obviously very keen to learn but might be getting a bit ahead of yourself?
-
[!--quoteo(post=368207:date=Apr 25 2006, 09:33 AM:name=ssjskipp)--][div class=\'quotetop\']QUOTE(ssjskipp @ Apr 25 2006, 09:33 AM) [snapback]368207[/snapback][/div][div class=\'quotemain\'][!--quotec--] Thanks mate! I've got one more quick question -- how would I go about recalling the images? Like, after I query the database, get the data back, how do I convert it to an 'image'? [/quote] base64_decode the data and then write it out to a file. So when you write it to the db you'll also need to save the filename or at least the file extension so you know what to recreate it as later.
-
[!--quoteo(post=367834:date=Apr 24 2006, 11:55 AM:name=KDragon)--][div class=\'quotetop\']QUOTE(KDragon @ Apr 24 2006, 11:55 AM) [snapback]367834[/snapback][/div][div class=\'quotemain\'][!--quotec--] Well, I wrote this php page and when i try to load it just sits there saying that it's transferring data from localhost (the server is on my comp). I'm pretty sure the following code is trying to do what I want it to do, which is change the text color from white to black (incremently, which is why I put it in a loop). I would just like to know why it doesn't load and just keeps transfering data. [code] <html> <head> <title>Untitled Document</title> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"> </head> <body> <?php function colorDec($colorNum){ $colorNum--; return $colorNum; } ?> <?php $colorNum=255; ?> <p style="color: rgb(<?php while($colorNum > 0){echo colorDec($colorNum);} $colorNum=255; ?>,<?php while($colorNum > 0){echo colorDec($colorNum);} $colorNum=255; ?>,<?php while($colorNum > 0){echo colorDec($colorNum);} ?>)">This be some text.</p> </body> </html> [/code] [/quote] Your not setting $colourNum to its new value hence colurNum is always going to be 255 and your code will execute forever. The easiest fix is to make your method parameter a reference. [code] function colorDec(&$colorNum){ $colorNum--; return $colorNum; } [/code] With that said, your code doesn't appear to work and you should start again. take a look at this tutorial which does something similar to what I think you are trying to achieve. [a href=\"http://www.phpfreaks.com/tutorials/5/0.php\" target=\"_blank\"]Tutorial[/a] Good Luck!
-
This is pretty rough but should get you started. [code] $tempname ="/tmp/".uniqid("img"); imagepng($im,$tempname); $rawImageData = file_get_contents($tempname); $rawImageData = chunk_split(base64_encode($rawImageData)); $sql = "insert into TABLE('imagedata') VALUES('$rawImageData')"; mysql_query($sql); unlink($tempname); [/code] with your code try removing the header and passing imagepng an absolute path.
-
imagepng will save the file for you if you pass it a path to save to. if you want to put the raw image data into a mysql field you'll need to save the image at least temporarily, read the image data into a variable with file_get_contents, base64_encode & chunk_split the data and write it to a medium blob field.
-
change all your if's and elseifs into switch/case statements.
-
[!--quoteo(post=367584:date=Apr 23 2006, 12:54 PM:name=Twentyoneth)--][div class=\'quotetop\']QUOTE(Twentyoneth @ Apr 23 2006, 12:54 PM) [snapback]367584[/snapback][/div][div class=\'quotemain\'][!--quotec--] I have this code to pull a variable from the address: $view = $_GET['view']; But that variable will be a persons name, so how do I auto capitalize it, just the first letter? [/quote] [a href=\"http://nz.php.net/ucfirst\" target=\"_blank\"]ucfirst[/a]
-
[!--quoteo(post=367491:date=Apr 23 2006, 03:23 AM:name=superpimp)--][div class=\'quotetop\']QUOTE(superpimp @ Apr 23 2006, 03:23 AM) [snapback]367491[/snapback][/div][div class=\'quotemain\'][!--quotec--] Is there a page where I can get an explation for fosockopen's $errno? I searched the php.net site and google but didnt found a thing. When I echo $errstr, it's blank. [code] <?php $host = "www.efzefrzefgoogle.com"; //zu prüfender host $timeout = 10; //timeout in sekunden if(@fsockopen($host, "80",$errno,$errstr,$timeout)) //prüfen, ob der host erreichbar ist, das @ unterdrückt fehlerausgabe { echo "<p>Online!</p>"; } else { echo "<p>Offline!</p>".$errno; } ?> [/code] thx! [/quote] In your code you're supressing error messages, Im not sure if that effects the error string being modified though. Also you aren't going to be able to do anything with your connection without getting the resource returned by fsockopen. Try following the example code on the php.net page for fsockopen. php.net/fsockopen
-
[!--quoteo(post=367092:date=Apr 21 2006, 02:12 PM:name=ColinDoody)--][div class=\'quotetop\']QUOTE(ColinDoody @ Apr 21 2006, 02:12 PM) [snapback]367092[/snapback][/div][div class=\'quotemain\'][!--quotec--] My issue is that by calling a javascript function I then replace the POST method, correct? And if I am replacing the POST method, I dont believe my POST data will be available when I recall the page. So how could i do this? I dont want to set the variables through GET because there is a password involved. Is there any way that in javascript i can force the variables into the POST slots? Thank you. I hope that made sense. I can be quite a n00b sometimes. [/quote] No, validating with javascript wont stop you posting your form to the server. Validating with js can be good as it saves your user a round trip to the server only to find out they've missed a field but its not fullproof as javascript can be turned off, so you'll at least want to perform the same validations with your php code as you do with javascript.
-
[!--quoteo(post=367011:date=Apr 21 2006, 07:45 AM:name=koritsi)--][div class=\'quotetop\']QUOTE(koritsi @ Apr 21 2006, 07:45 AM) [snapback]367011[/snapback][/div][div class=\'quotemain\'][!--quotec--] Hello guys and girls! I need some help because I'm new and not so good on programming. The thing is that I have a php login page with a form in it. The form's action is on another page in case username and password are correct. This works fine but I must also have a fail page in case username and password are incorrect or an alert message on submit that will not allow user to leave the home page before entering the correct username and password. This is how the php code looks like: <?php $ena= $_POST[textfield]; //username from home page $dyo = $_POST[textfield2]; //password >> >> $conn = mysql_connect("localhost", "root", "12365875") or die(mysql_error()); mysql_select_db("e-kiosk",$conn) or die(mysql_error()); $sql = "SELECT username,password FROM customers"; $result = mysql_query($sql,$conn) or die(mysql_error()); $user = $row['username']; //username from database $pass = $row['password']; //password >> >> if ($ena!==$user and $dyo!==$pass) { } ?> Is there something I can do on submit of the form? I have tried to compine php and javascript there but it doesn't work. What else can i do? This is a small piece of my form: <form name="form1" method="post" action="/loganswer.php"> <p><font color="ff9900"><strong>Username:</strong></font></p> [/quote] You need a line of code to get the database information you are after. Put this line after you execute your query. [code] $row = mysql_fetch_assoc($result); [/code]
-
[!--quoteo(post=366231:date=Apr 19 2006, 08:56 AM:name=Vids)--][div class=\'quotetop\']QUOTE(Vids @ Apr 19 2006, 08:56 AM) [snapback]366231[/snapback][/div][div class=\'quotemain\'][!--quotec--] [code] <form method="get" action="http://www.rcsb.org/pdb/downloadFile.do?fileFormat=pdb&compression=NO&structureId=$_GET['id']"> <input type=text name=id value=""> <input type=submit value="Download"> </form> [/code] I have a problem. In the above form, When some one types the value in the text, and clicks on download, i want the browser to go to the following site [a href=\"http://www.rcsb.org/pdb/downloadFile.do?fileFormat=pdb&compression=NO&structureId=$id\" target=\"_blank\"]http://www.rcsb.org/pdb/downloadFile.do?fi...tureId=$id[/a] where $_GET['id'] is what they typed in. For Example, If they type in 1LYN, The browser must download [a href=\"http://www.rcsb.org/pdb/downloadFile.do?fileFormat=pdb&compression=NO&structureId=1LYN\" target=\"_blank\"]http://www.rcsb.org/pdb/downloadFile.do?fi...tructureId=1LYN[/a] Only the end of the URL changes. Whats wrong with my code? Any help will be appreciated! [/quote] change your form action to just [a href=\"http://www.rcsb.org/pdb/downloadFile.do\" target=\"_blank\"]http://www.rcsb.org/pdb/downloadFile.do[/a] then access id through the $_GET array in your downloadFile.do script. Obviously I dont know the purpose of this script but you should also probably do some checks on the file the user is requesting if you aren't already.
-
[!--quoteo(post=365798:date=Apr 18 2006, 11:14 AM:name=michaellunsford)--][div class=\'quotetop\']QUOTE(michaellunsford @ Apr 18 2006, 11:14 AM) [snapback]365798[/snapback][/div][div class=\'quotemain\'][!--quotec--] I saw something about this somewhere, but I can't find it. Maybe I'm imagining things? I have a set of variables I'd like to use in a while(next($array)) loop. I'd like to pass that array using the GET method. What I remember seeing was naming form fields like an array, for example: [code]<input type="text" name="field[1]">[/code] would result in PHP associating it as an array when received. But, how to access that array is escaping me. I guess I could strpos(current($_GET),"[") and recreate the arrays? This seems like the long way around though. thanks for any direction. [/quote] If your talking about a variable you want to recreate across server requests then you need to look into serialization. The code you've shown is for form controls such as multiple select enabled listboxes or a series of checkboxes.
-
[!--quoteo(post=364744:date=Apr 14 2006, 08:26 PM:name=prefix)--][div class=\'quotetop\']QUOTE(prefix @ Apr 14 2006, 08:26 PM) [snapback]364744[/snapback][/div][div class=\'quotemain\'][!--quotec--] What I'm trying to do is add an '*' to the end of a single word search string, if an asterixes isn't already there. I've been trying with if (strstr($searchstring,'*')) but can't seem to get it to work. Any help much appreciated. [/quote] I think you want if (!strstr($searchstring,'*')) $searchstring .= "*"; The '!' means not
-
If you take a look at the link I've given in firefox you'll see the problem I'm having. It doesn't occur in IE6. The links push the content to the right down the page creating a nasty gap between the rows. Can anyone tell me how to get around this issue? Thanks in advance. [a href=\"http://bmv.shinydev.co.nz/product.php?categoryid=7&comp=0\" target=\"_blank\"]Example of problem[/a] [a href=\"http://bmv.shinydev.co.nz/bmv.css\" target=\"_blank\"]css file[/a] **Never Mind, sorted it, thanks if you had a look :)**
-
[!--quoteo(post=364686:date=Apr 14 2006, 02:29 PM:name=Jarin)--][div class=\'quotetop\']QUOTE(Jarin @ Apr 14 2006, 02:29 PM) [snapback]364686[/snapback][/div][div class=\'quotemain\'][!--quotec--] Well, considering I'm not very math savvy, I need a bit of help. I'm trying to determine whether one integer is evenly divisible into another integer, IE is 1033343 a multiple of 3, is 105 a multiple of 5, etc. How would one go about determining this in a memory-conservative manner? Thanks in advance. [/quote] use the '%' operator which gives you the remainder of a division. e.g $i = 5 % 5; #$i=0;
-
[a href=\"http://dev.mysql.com/doc/refman/4.1/en/mysqldump.html\" target=\"_blank\"]Yes.[/a]