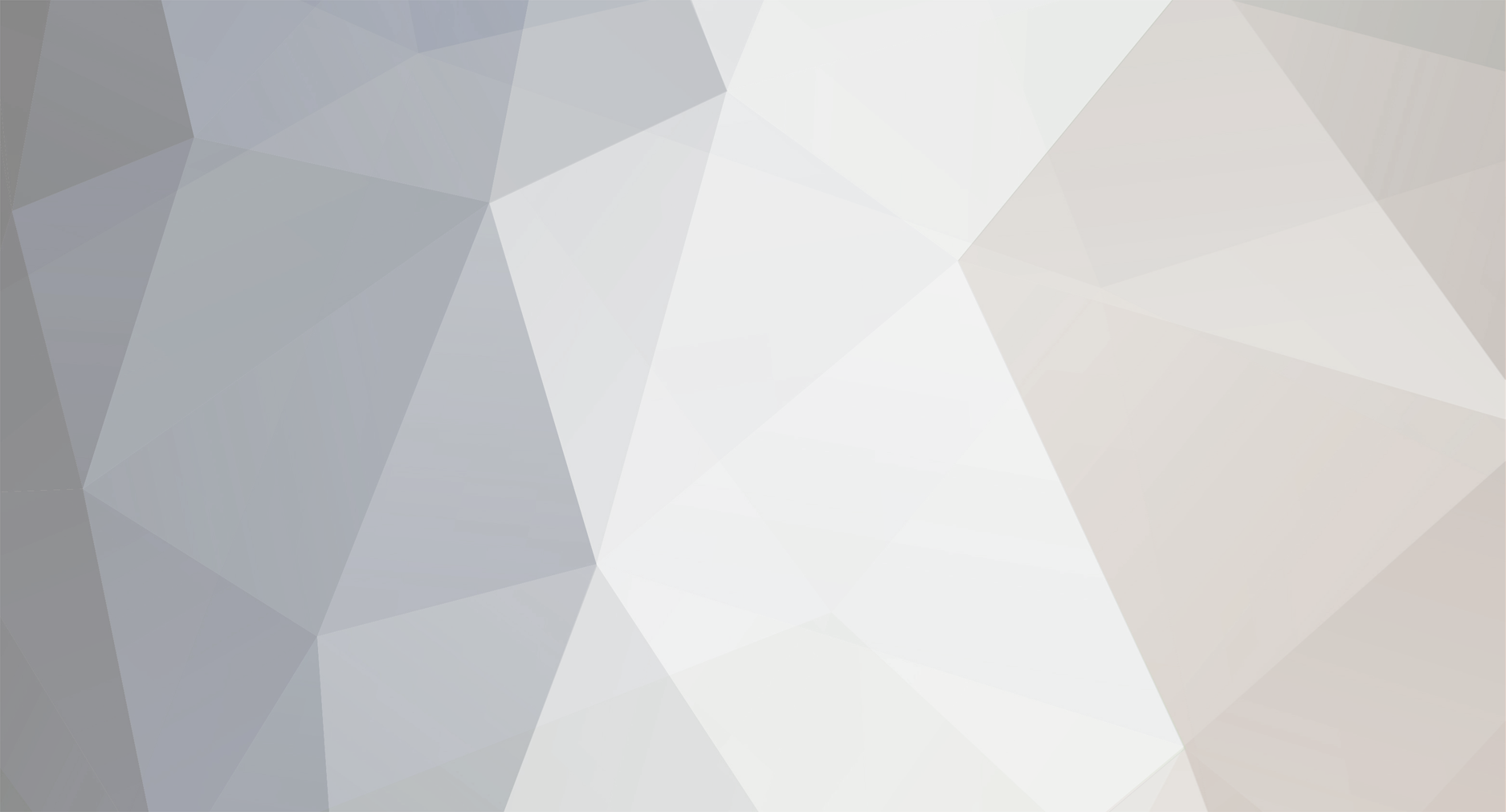
KrisNz
-
Posts
271 -
Joined
-
Last visited
Posts posted by KrisNz
-
-
Does this do the same thing as what you're trying?
$wordScramble = array("apple","superman","wireless","midlife","computer","string","massive","wrench","fiber"); $randWord = array_rand($wordScramble); $word_array = str_split($wordScramble[$randWord]); shuffle($word_array); $scrambled = implode("",$word_array); echo $scrambled;
-
Im going to hazard a guess and say try
foreach ($names as $a_person_object) { echo $a_person_object->id; echo $a_person_object->name; }
-
(to expand on whats already been pointed out)Do it as a csv (comma separated values) . A very basic php5 example would be
$my_csv_data = "$value1,$value2,$value3"; file_put_contents("example.csv",$my_csv_data);
You should find when you open that file in excel, the values in $value1 etc are in a1,a2,a3 respectively. You can then save that file as an xls if you want. Actually working with an excel object isn't a really realistic option in a web server environment, and in your case not necessary.
you could also check out
http://www.php.net/manual/en/function.fputcsv.php
http://www.php.net/manual/en/function.fgetcsv.php
-
Wildbug, that was exactly what I needed. I see now that thats even in the mysql manual for regexp, I just didn't read it thoroughly enough.
I should apologize, when I wrote
I realize now this was misleading, what I meant was that I wanted to count rows where the number appeared in that field. I've now been able to do that by working in Wildbug's regexp into my query.I'm trying to get a count of the number of times an id appears in a record for a particular useAnd yes, It would be nice to be able to move those ids into a look up table, but really thats the least of this projects problems!
Thanks heaps to both of you! Really appreciate it.
-
Yup, thats what Im after.
and so if the data looked like
id => 2
reaID => '1;3;2;22;'
I still need the count to be 1.
Thanks.
-
Hi, I have a column I'm trying to extract information from. The previous developer on a project has stored a number of ids in a text field separated by semicolons. an example of data in this field could be "1;2;3;4" or just "49" if theres only one id. Im trying to get a count of the number of times an id appears in a record for a particular user.
from php im running this query
select count(ID) as agent_tsr_count from TSR tsr where reaID REGEXP '149[^0-9][[.semicolon.]]{0,1}' and lawID = 31
This seems close but is definitely not right! Can someone please advise me on how to change the query so it only matches the whole number, optionally followed by a semi-colon?
Thanks for reading this,
Kris.
-
If you want to allow multiple files uploaded in one hit you need to add more file elements to your form like
<input type="file" name="form_data[]" size="40">
<input type="file" name="form_data[]" size="40">
<input type="file" name="form_data[]" size="40">
see http://au2.php.net/manual/en/features.file-upload.php for a more complete explanation.
You should also make a separate table for storing information about the files since theres often going to be more than one file associated with a lesson plan.
e.g
table : lesson_plan
id
title
description
age_group
date_added
email_address
----------------------------
table : lesson_plan_files
id
lesson_plan_id (foreign key - the id of the record associated with this file)
file_name
file_type
file_size
file_data
-
1. You've set $_SESSION['first'] after you set the variable $first, so you're trying to set $first to something that doesn't exist yet.
2. The session exists now, you set it on line 4 of page 2.
Note that on page three, you havent POSTed anything to it, you click a link to get to that page so the $_POST array will be empty.
3. Always create things before trying to use them.
-
You cant do it on a string like '2007/01/29', you need to convert it to a timestamp first.
$date_ts = strtotime('2007/01/29'); //then say you wanted to add 4 weeks to that date and print it to the user. $one_month_from_now = strtotime("+4 week",$date_ts); echo date("Y/m/d",$one_month_from_now);
strtotime is a really cool and powerful function, http://au3.php.net/strtotime
-
-
you could use strtotime() on their input but not reliably. Rather than have the user type a date in, Id recommend giving the user three select boxes for day, month and year respectively. That will give you a lot more control over the input. Then to make a timestamp you could use for example
//you should validate that the date they've posted is valid, e.g not 30 february, though mktime will work that out as 1 march i think $db_timestamp = mktime(12,0,0,$_POST['month'],$_POST['day'],$_POST['year']); //assuming of course those posted values are integers.
-
theres a number of MVC frameworks for php, heres links to a few of them.
-
nothing to do with php but you want
<input type="image" src="/path/to/image"/>
you can also ditch "style='margin=0px 0px 0px 0px'"
I'm also curious as to why you'd have a form with only hidden fields.
-
replace
if (mysql_result($sql_result,0,0) > 0)
with
if(mysql_num_rows($sql_result) > 0)
You're also missing an 'AND' in the sql statement before 'acccode '
-
My only tip at this stage would be not to include profanity in your question, however unintentional it may be
-
I had some success with the following...
$ch = curl_init(); $url ="http://www.php.net/curl_setopt"; curl_setopt($ch,CURLOPT_URL,$url); curl_setopt($ch,CURLOPT_RETURNTRANSFER,true); curl_setopt($ch,CURLOPT_FOLLOWLOCATION,true); $result = curl_exec($ch); $url_actual = curl_getinfo($ch,CURLINFO_EFFECTIVE_URL); curl_close($ch); echo ($url_actual); //for me it echo's http://au.php.net/curl_setopt but it will depend your location
I'm not 100% sure this will apply to your situation but give it a go.
-
Sounds like you're referring to the array's key.
$a = array(); $a['array_key_name'] = 'array_key_value'; $b = key($a); // echo $b; // echos array_key_name
You should read this http://au2.php.net/key
and this http://au2.php.net/manual/en/function.array-keys.php
..and probably this too http://au2.php.net/foreach
-
If you're doing this for text your going to output on an html page, then its much easier to do this...
<p style="text-transform:capitalize;">the fish was delish and it made quite a dish</p>
-
change "SELECT * FROM company WHERE cName = $cName",
to
"SELECT * FROM company WHERE cName = '$cName'",
since your searching for a string.
-
It says 'Array' because thats what it is - a variable that can hold a set of values, if you want to see its values you can use print_r() to give you a rough dump of whats in an array or use a loop to go through each value stored in the array. If its multi choice you might be better of with radio buttons so that only one answer can be provided. Heres some basic code you can play around with if you like, try changing type="radio" to type="checkbox" and you'll see that the code still works.
<html><body> <?php //print_r($_POST['answer']); - this gives you a basic dump of what is in the array //loop through each provided answer if(isset($_POST['answer']) and !empty($_POST['answer'])) { foreach($_POST['answer'] as $an_answer) { echo "The answer You chose was ".$an_answer."<br/>"; } } ?> <form method="post" action=""> 1<input type="radio" name="answer[]" value="1" /><br/> 2<input type="radio" name="answer[]" value="2" /><br/> 3<input type="radio" name="answer[]" value="3" /><br/> 4<input type="radio" name="answer[]" value="4" /><br/> <input type="submit"/> </form> </body></html>
Some reference
-
Yup, whats its doing is setting the 'protected $ID' - a property of the calendar to the value contained in $ID - the first argument of the function. That particular function is what's known as a constructor, and its called automatically when you create an instance of the object.
E.g
$cal = new Calendar(1,'2007-01-11'); //I dont know how it expects the date to be formatted so that may not work, its just an example echo $cal->ID; //echos '1_' because the constructor has been called and the objects property has been set to 1 and concatenated with an underscore by the constructor
Because that property is marked as protected, it means that its value can only be changed by that class or any class that extends it. So if you tried to go
$cal->ID = "some crazy value";
You would get an error.
-
I ran across a similar problem a while ago, I cant remember exactly what it was, but try
replacing <script>
with <script type="text/javascript">
alternatively try removing that script section altogether, failing that try only outputting a few lines of the file at a time, adding more till you get the blank page.
oh and it looks fine in ie6. -
try calling [code]session_write_close();[/code] before you do your redirect. Even if thats not the problem you should do it anyway. :)
-
[quote]My question is whether there is a chance that two users would trigger the script at the same time, and the queries that are supposed to run only once every 30 minutes could run twice.[/quote]
Its unlikely since your code is going to be executed in fractions of a second. If its absolutely imperative that the record is only accessed by one user at a time then you should look in to database transactions, but as redarrow suggested, the simplest solution is to set up a cron job (if you're on a web host there should be a way to do it through the hosts control panel), otherwise this: [url=http://www.faqts.com/knowledge_base/view.phtml/aid/1005]http://www.faqts.com/knowledge_base/view.phtml/aid/1005[/url] might be helpful.
Word Scramble
in PHP Coding Help
Posted
cool, upon looking up the manual, i see theres actually a function for shuffling a string! So you can replace lines 3 through 6 with
Moral of the story - read the manual