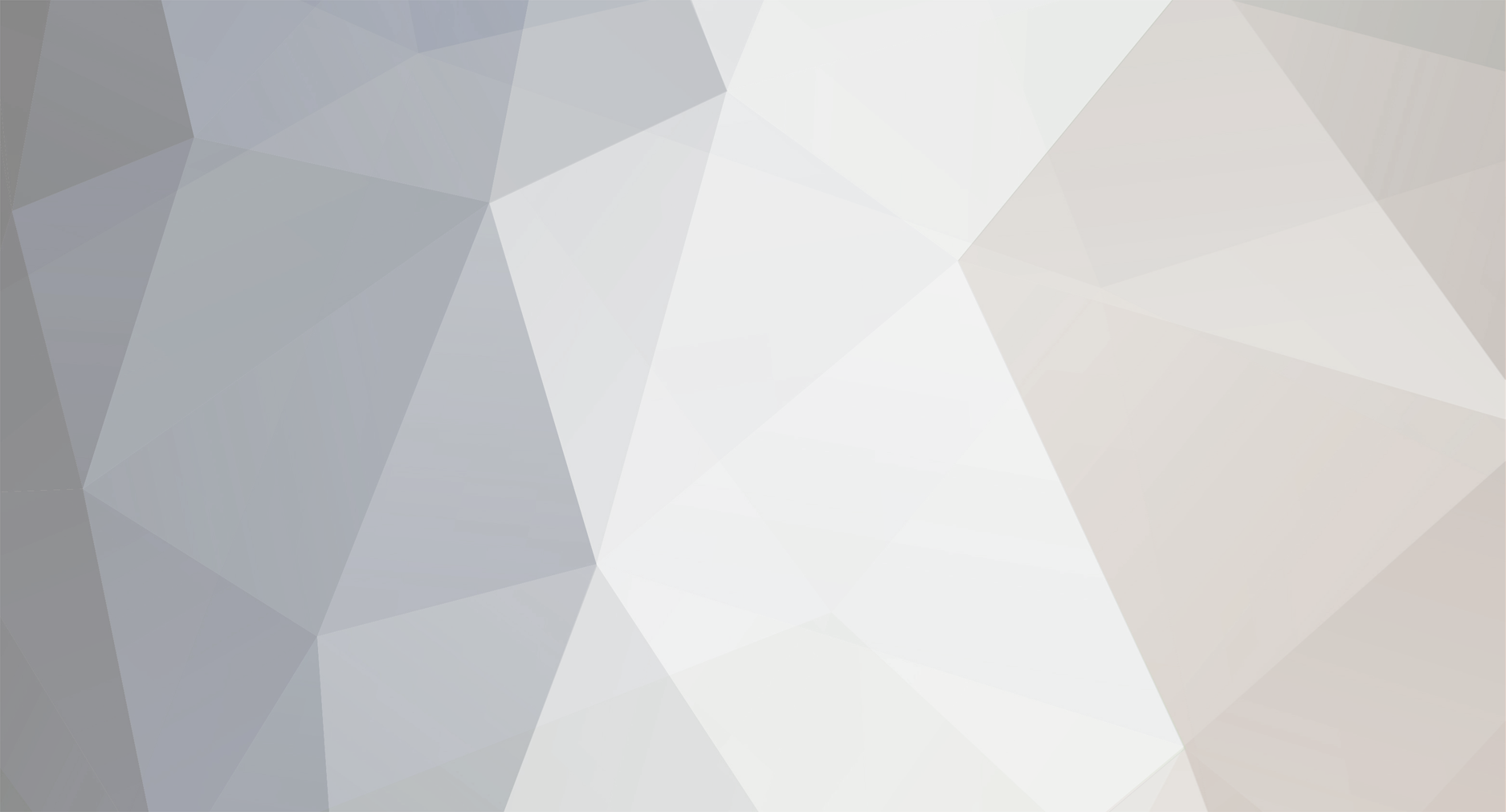
Nicklas
Members-
Posts
111 -
Joined
-
Last visited
Never
Everything posted by Nicklas
-
[quote author=rsammy link=topic=115083.msg468540#msg468540 date=1163620552]i wud like to show the first and last name initials for phy_fname and phy_lname in the code above! how do i do that. im not too comfortable with php yet! [/quote] ex [code=php:0]print($row->phy_fname[0] . $row->phy_lname[0]);[/code]
-
[quote author=businessman332211 link=topic=115083.msg468454#msg468454 date=1163613847]my middle name's are West Lee Joyel West Lee Puryear say I put west lee after joyel, then how is your program goign to cut that up, it won't. See what I mean, you have to accont for that as well. [/quote] ex: [code=php:0]$name = "Joyel West Lee Puryear"; echo preg_replace('/(\w)\w+ ?/', "\\1", $name);[/code]
-
Or [code]<?php $name = "John Smith"; list($first, $last) = sscanf($name, "%s%s"); echo $first[0] . $last[0]; // Output: JS ?>[/code]
-
ORDER BY and LIMIT should always be in the end of your query ex [code=php:0]SELECT title, category, salary, location, number FROM joborder WHERE category='Development' ORDER BY number ASC LIMIT 3[/code] Your query can also be written like this: ex [code=php:0]SELECT * FROM joborder WHERE category='Development' ORDER BY number LIMIT 3[/code]
-
How about something like this? [hr] [code=php:0]preg_match_all('/(?<=<a href=").*?(?=">)|[^<>]+(?=<\/a>)|(?<=<td >).*?(?=<)/', $string, $matches); $matches = array_chunk($matches[0], 14); print_r($matches);[/code] [hr] Gives me an array() like this [code]Array ( [0] => Array ( [0] => /nba/players/playerpage/6552 [1] => Tim Duncan [2] => 6 [3] => 6 [4] => 21.5 [5] => 49 [6] => 91 [7] => 53.8 [8] => 31 [9] => 57 [10] => 54.4 [11] => 0 [12] => 1 [13] => 0 ) [1] => Array ( [0] => /nba/players/playerpage/139066 [1] => Manu Ginobili [2] => 6 [3] => 6 [4] => 13.0 [5] => 25 [6] => 64 [7] => 39.1 [8] => 20 [9] => 25 [10] => 80.0 [11] => 8 [12] => 24 [13] => 33.3 ) )[/code]
-
To optimize your pattern, you can do something like this: [code=php:0]preg_match_all('~(?<=data">).*?(?=<)|\d{2}/\d{2}/\d{4}~is', $result, $matches); list($phno, $first, $last, $oneyr, $twoyr) = $matches[0];[/code]
-
to convert it back to a string, all you have to do, is something like this: [code=php:0]$decimal = "8571428571"; $rdays = '' . $decimal / "14.285714285"; echo $rdays[0]; // print first char: 6[/code]
-
This should do the trick! ;) [code=php:0]preg_match_all('/\w+/', $string, $matches); print_r($array);[/code] Gives me this: [code] Array ( [0] => BCABBOTSFORD [1] => KLINGON [2] => LUCY [3] => JORDAN [4] => 39994 [5] => EPSON [6] => PL [7] => ABBOTSFORD [8] => BC [9] => V3E [10] => 1L4 [11] => 6360287906496 [12] => 100819880801 [13] => 0AV3G1J4 [14] => M168 [15] => 54BRNHAZ [16] => U8 [17] => R [18] => 690 )[/code]
-
280 days into the future from now [code]<?php echo date("Y-m-d", strtotime("+280 days")); ?>[/code] add 280 days to a given date, ex starting from 2006-11-01 (good for countdowns etc) [code]<?php echo date("Y-m-d", strtotime("2006-11-01 +280 days")); ?>[/code] To get the time in pure unixtime, just remove the surrounding date() function. ex: [code]<?php echo strtotime("+280 days"); ?>[/code] Or [code]<?php echo strtotime("2006-11-01 +280 days"); ?>[/code]
-
Here´s yet another way [code]<?php $mystring = "[name][age][pet][mike][22][dog][john][25][cat][kim][26][bird][pam][18][fish]"; function toArray($name, $age, $pet) { global $info; $info[] = array('name' => $name, 'age' => $age, 'pet' => $pet); } preg_replace('/\[(.*?)]\[(.*?)]\[(.*?)]/e', 'toArray("\\1", "\\2", "\\3")', substr($mystring, 16)); print_r($info); ?>[/code]
-
Select a list of words in a DB and replace them if present in a text
Nicklas replied to anarchoi's topic in PHP Coding Help
Show us some code -
Hi again printf, nice code, I appreciate it! :) By curiosity, I compared your code with my code to see wich one is more effective, and I found that my code actually executes a bit faster than yours, not that much but still.
-
[quote]glob returns the full path so you need to change your test logic...[/quote] Did you even try the code ::) [quote]Also it would be better to remove all those if(?) and switch() out of the loop, so you sort by, not loop and then question, question![/quote] "so you sort by" ? To be able to sort by filesize, date or filename via variable, the array needs to built up in the right order and [u]then[/u] be sorted correctly with array_multisort(). If you happen to have a better suggestion, please share it with us and help [b]JustinMs66[/b]
-
Oops ;) then do something like this, add a switch-case to determine how the array should be sorted ex [hr] [code=php:0]<?php foreach(glob("*.*") as $file) { if ($file != "index.php" && $file != "upload.php" && $file != "index.html") $size = filesize($file); $date = date("Y-m-d H:i:s", filectime($file)); if (isset($_GET['sort'])) { switch ($_GET['sort']) { case 'size' : // Sort by size $array[] = array('size' => $size, 'date' => $date, 'name' => $file); break; case 'date' : // Sort by date $array[] = array('date' => $date, 'size' => $size, 'name' => $file); break; default : // Sort by name as default $array[] = array('name' => $file, 'size' => $size, 'date' => $date); } } else { // If $_GET['sort'] isn´t set, sort by filename as default $array[] = array('name' => $file, 'size' => $size, 'date' => $date); } } array_multisort($array); print_r($array); ?>[/code] [hr] If you´d like to sort by size - [color=blue]?sort=size[/color] If you´d like to sort by date - [color=blue]?sort=date[/color] If nothing or anything else is specified, the code will sort by filename as default
-
[code=php:0]$string = str_replace("\r\n", '', $string);[/code]
-
There´s no need for a callback-function to sort in this case. Just make sure the filesize goes into the first index of the multidimensionall array, like this: [code]$array[] = array( 'size' => filesize($file), 'name' => $file );[/code] and then run the array thru [url=http://www.php.net/array_multisort]array_multisort()[/url] Example: loads all files from the current directory and sort by filesize (ascending), if you´d like to sort it in reversed order, use [url=http://www.php.net/array_reverse]array_reverse()[/url] after the [url=http://www.php.net/array_multisort]array_multisort()[/url] function. [hr] [code=php:0]<?php foreach(glob("*.*") as $file) { if ($file != "index.php" && $file != "upload.php" && $file != "index.html") $array[] = array('size' => filesize($file), 'name' => $file); } array_multisort($array); print_r($array); ?>[/code] [hr] If you only want to fetch a specific filetype from your dir, all jpg´s for example, then remove the if-case and change the for-case to this: [code=php:0]foreach(glob("*.jpg") as $file)[/code] if you´d like to get more than 1 filetype, then you can add more extensions by doing like this: [code=php:0]foreach(glob("*.{jpg,png,gif}", GLOB_BRACE) as $file)[/code]
-
Select a list of words in a DB and replace them if present in a text
Nicklas replied to anarchoi's topic in PHP Coding Help
you could do something like this: ex [code=php:0]<?php $text = "Here´s your string with a lot of text and a few names like Peter Kropotkine and Joseph Proudhon"; include("db_connection.php"); $sql = "SELECT * FROM your_table"; $result = mysql_query($sql); while($row = mysql_fetch_assoc($result)) { $name = $row['name']; // name from your database $text = preg_replace("/($name)/ise", '"<a href=\"mypage.php?name=" . urlencode("\\1") . "\">\\1</a>"', $text); } echo $text; ?>[/code] -
If the word "me" is always followed by a < tag, then you could do like this: [code=php:0]echo preg_replace('/\bme\b(?=<)/s', 'Jon', $html);[/code]
-
***SOLVED Counting duplicates in a MySql query
Nicklas replied to switchdoc's topic in PHP Coding Help
[quote]Nicklas, what output does it produce?[/quote] Im sorry, I forgot to add [i]clickdate[/i] in my query when I posted it, my query looks like this [code=php:0]SELECT *, COUNT(LinkID) AS total FROM your_table GROUP BY LinkID, clickdate[/code] The data in my database looks like this: [code]LinkID clickdate 604 2006-11-08 111 2006-11-08 111 2006-11-08 111 2006-11-08 229 2006-11-09 662 2006-11-09 183 2006-11-25 229 2006-11-09 183 2006-11-09[/code] After the query, it looks like this [code]LinkID clickdate total 111 2006-11-08 3 183 2006-11-09 1 183 2006-11-25 1 229 2006-11-09 2 604 2006-11-08 1 662 2006-11-09 1[/code] -
***SOLVED Counting duplicates in a MySql query
Nicklas replied to switchdoc's topic in PHP Coding Help
[quote]"Select *" will not work because it selects clickdate without aggregating it (in theory anyway.. I have not tried this in mysql)[/quote] works for me... -
***SOLVED Counting duplicates in a MySql query
Nicklas replied to switchdoc's topic in PHP Coding Help
Try something like this [code=php:0]SELECT *, COUNT(LinkID) AS total FROM your_table GROUP BY LinkID[/code] -
[url=http://www.php.net/nl2br]nl2br()[/url]
-
Take a look at [url=http://www.php.net/array_unique]array_unique()[/url]
-
[code=php:0]<?php $string = '01234567890abcd[ignorethis]blahblahblah'; echo preg_replace('/\[.*]/', '', $string); ?>[/code]