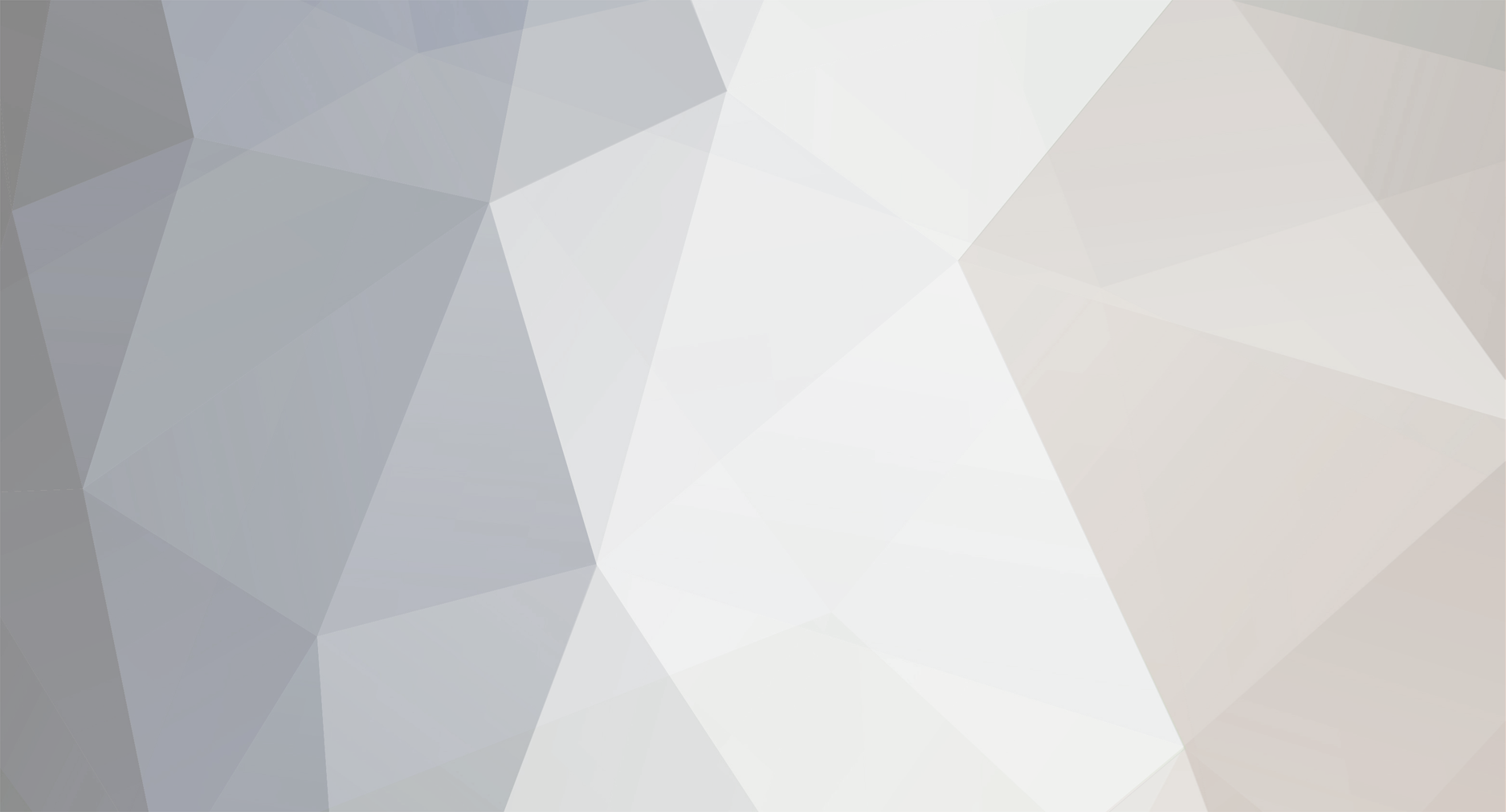
simcoweb
Members-
Posts
1,104 -
Joined
-
Last visited
Everything posted by simcoweb
-
quick question regarding the use of POST values and links
simcoweb replied to nikefido's topic in PHP Coding Help
Well, in a way you can. Not using the typical <a href> tag, though. You would use it in your 'action' tag in the form: action="nameofpage.php?memberid=$_POST['memberid']" which would pass along the data. You can string as many items as you wish on the end of your url. -
quick question regarding the use of POST values and links
simcoweb replied to nikefido's topic in PHP Coding Help
Actually, if i'm understanding your question, using 'POST' creates an array of the form data that can be manipulated in the form processing code. If you want to pass parameters via the url then you would use 'GET' to snag the info and use it. Like this: www.yoursite.com/page.php?memberid=333 Then use: $memberid = $_GET['memberid']; To do things like: echo $memberid; of insert into database, extract from database based upon that id, etc. etc. etc. -
[SOLVED] Making form feild required before submitting it
simcoweb replied to Dada78's topic in PHP Coding Help
In my PHP editor it didn't produce an error but it still looks goofy I'm thinking that the $num_rows isn't producing any value. Otherwise the code should work as it would either prove to be true/false and act accordingly. Try echoing the value of that variable to see if it carries anything. -
[SOLVED] Making form feild required before submitting it
simcoweb replied to Dada78's topic in PHP Coding Help
Be sure to take that extra ; out of Ken2k7's first query line as well. -
[SOLVED] Making form feild required before submitting it
simcoweb replied to Dada78's topic in PHP Coding Help
I did look at it carefully. There's two ; in it and you only need the last one. The " " around the entire statement from Select to the end ' should have one ; after it. -
This line: $sql_check_user = "select * from admin where username='$user'"); Take out the ) as it's not needed as is throwing an error in your code. Probably making it die.
-
Ken2k7's snippet will print them out on a single line if that's good enough. Otherwise you'd need in integrate some HTML into the 'echo' to have it return in table cells/rows.
-
You don't need brackets on the WHERE clause variable you're matching against: SELECT * FROM flightbids WHERE pid = '{$pid}' should be: SELECT * FROM flightbids WHERE pid = '$pid'
-
You have extra ; in this line: $match = "select id from $table where username = '".$_POST['username']."' and password = '".$_POST['password']."';";
-
Since you're running the 'while' loop you don't need to also assign the results to variables unless that's what you want. Are you wanting to print the results?
-
add: or die(mysql_error()); to the end of your query statements to see what the error message is.
-
[SOLVED] Making form feild required before submitting it
simcoweb replied to Dada78's topic in PHP Coding Help
Yeah, and another issue..his INSERT query is actually after the error so basically no matter what happens it's going to insert it. It needs to be moved up just before the 'header' section so that IF there's no match then it WILL insert the data. Right now, the way it's set up, the error message is going to display AND the INSERT is going to happen. -
[SOLVED] Making form feild required before submitting it
simcoweb replied to Dada78's topic in PHP Coding Help
Actually it's YOUR form code that redirects, not my validation code. In my forms I use <?php echo $_SERVER['PHP_SELF']; ?> in the 'action' tag so the page returns to itself. IF there's no errors then my final full code will redirect them to a success page. But, if there's errors they would display right above the form in a neat uniform list so it's easily viewable... and, quite professional looking And this line has an extra "; in it: $check = "select email from users where email = '".$_POST['email']."';"; -
Open affiliate.php, copy and paste the code into your next post.
-
[SOLVED] Making form feild required before submitting it
simcoweb replied to Dada78's topic in PHP Coding Help
Hmmm...what's not to like? Works like a charm! lol Ok, part of your problem is that your 'logic' in producing the error is wrong. I tried your form by inputting the email garbage@yourhouse.com and it told me that it was already in use. Now, i'm willing to bet that it's NOT in use. But, that's the only error you have. What it should say if there's NO match is that it doesn't recognize me and I need to register. -
Use this code for each of your form fields if you want whatever you've posted to remain in the boxes in the event there's an error that returns you back to the form so you don't have to fill it out again. This sample is for the 'Phone' field. It won't display anything unless the form has actually been submitted. <input type="text" name="Phone" size="20" value="<?php if (isset($_POST['Phone'])) echo $_POST['Phone']; ?>">
-
[SOLVED] Making form feild required before submitting it
simcoweb replied to Dada78's topic in PHP Coding Help
This code works for me in every form I do. Note the array for the error messages. That way they're much easier to handle. You can modify the form field variables to your needs as well as the field names in the 'if' statements. This checks that the fields are completed AND that the email is a valid format. Then loops through any errors as a <ul>. Form code: <?php session_start(); // Turn on magic quotes to prevent SQL injection attacks if(!get_magic_quotes_gpc()) set_magic_quotes_runtime(1); if (isset($_POST['submitted'])) { // get form data $name = strip_tags(trim($_POST['Name'])); $company = strip_tags(trim($_POST['Company'])); $email = strip_tags(trim($_POST['Email'])); $phone = strip_tags(trim($_POST['Phone'])); $contact = strip_tags(trim($_POST['Contact'])); $comments = strip_tags(trim($_POST['Comments'])); $today = date("F j, Y, g:i a"); // some validation $errors = array(); // input error checking if ($name=="") { $errors[] = "Please enter your full name.<br/>"; } if ($phone==""){ $errors[] = "Please enter your phone number.<br/>"; } if ($email=="") { $errors[] = "Please provide your email address<br>"; } if ($email) { if (!eregi("^[_a-z0-9-]+(\.[_a-z0-9-]+)*@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$", $email)) { $errors[] = $email. " is not a valid email address. Please enter a valid email address.<br/>"; } } if (!$errors) { // run your query code here for the email } } ?> Error display (insert this into your HTML where you want them to show if there's errors): <?php if(!empty($errors)) { echo "<font color='red'><strong id='errorTitle'>One or more input fields on the form has not been completed</strong>"; echo "<ul>"; foreach ($errors as $value) { echo "<font face='Verdana' size='2' color='red'><li>$value</li></font><br/>"; } echo "</ul><br/>\n"; } ?> -
I guess this statement threw me off. 'ALL' to me is more than one.
-
Heh... why couldn't he just put: $action = $_GET['action']; right after the opening <?php tag? That would be a simple way to populate the variable and allow his code to run.
-
If you want all users from that table your query should be: "SELECT * FROM table_name WHERE clauses go here"
-
Depends on how you want to pass that information. If you used a set variable then you'd just do this: $imagepath = "/path/to/image"; <img src="$imagepath/images/top_invoice.gif" width="560" height="33">
-
Final note on this. It was just the 'or die(mysql_error())' function assigned to the $numrows statement. Once that was removed everything worked perfectly. The explanation for anyone's edification: Again, thank all of you for your help. It turns out it was something that simple! Another lesson learned
-
Try changing this: $e = $_POST; to this: $e = $_POST['email'];
-
Ok, new update. I had someone look at this issue and what they had me do is remove the two 'or die(mysql_error()); ' statements associated with the $numrows and $results from the query. Once I did that the search worked perfectly. It displays the 'No results...' message when there are none (instead of just a blank page) and the results when there are matches. In explaining it, the 'or die' was causing the script to 'die' without producing an error message on the next page (since it was looking for a mysql_error). Therefore the blank page. In any event, i'd still like to know what was occurring for future edification and why it would 'die' at that spot. Just for reference, here's the final code used: if (isset($_POST['search'])) { // query for extracting lenders from amember database $category = strip_tags(trim($_POST['category'])); $loantype = strip_tags(trim($_POST['loan_type'])); //$loanamount = strip_tags(trim($_POST['loan_size'])); $sql = "SELECT * FROM amember_members WHERE is_lender='Yes' AND '$category' IN (category_1, category_2, category_3, category_4, category_5) AND '$loantype' IN (loan_type, loan_type2, loan_type3, loan_type4, loan_type5) ORDER BY top_3 LIMIT 10"; $results = mysql_query($sql); $numrows = mysql_num_rows($results); if ($numrows == 0){ include 'header.php'; echo "<h3>No Results Found</h3><br> <p class='bodytext'>No lenders matched your search criteria. Try modifying the search parameters for broader results.<br>\n"; echo "<br><input type=button value=\"Return to Search\" onClick=\"history.go(-1)\">"; include 'footer.php'; } else { include 'header.php'; echo "<h3>The following lenders meet your search criteria.</h3><br>\n"; // create display of results in abbreviated format while ($row = mysql_fetch_array($results)) { echo " <div class='wrapper_out' onmouseover=\"this.className='wrapper'\"; onmouseout=\"this.className='wrapper_out'\";> <table width='500' border='0' align='center'> <tr ><td colspan='2' class='lendertitle'>".$row['company_name']."</td></tr> <tr><td colspan='2' class='lenderdesc'>Company phone: ".$row['company_phone']."</td></tr> <tr><td width='300' class='lenderdesc'>Company contact: ".$row['company_contact']."</td> <td width='200'><a class='lenderlink' href='loanrequest.php?lenderid=".$row['member_id']."'>Submit Loan Request</a></td></tr> <tr><td colspan='2'>"; echo pullRating($row['member_id'],true,false,true); echo "</td></tr> </table></div><br />\n"; echo "<hr style='border-top: 1px dotted #666666' color='#666666' width='400' size='1'>"; } include 'footer.php'; } }
-
Ken2k7... it's a new day. I'll single quote 'em and give it a try again to get things started on the quest to solvation.. ! Kensk7, just wanted to report back, adding the single quotes actually broke the script. It returned...what else...a blank page! So I went back to the non-single quote setup. Still breaking, though, when searching for non-available data. Working on that. Ok, more info. I changed the second 'AND' condition (which is the loan_type) to an 'OR' and that works. Of course, it doesn't provide the accuracy of the 'AND' search that I need. But, at least it narrows possibly what the problem is. The second 'AND' condition is breaking when there's no matches.