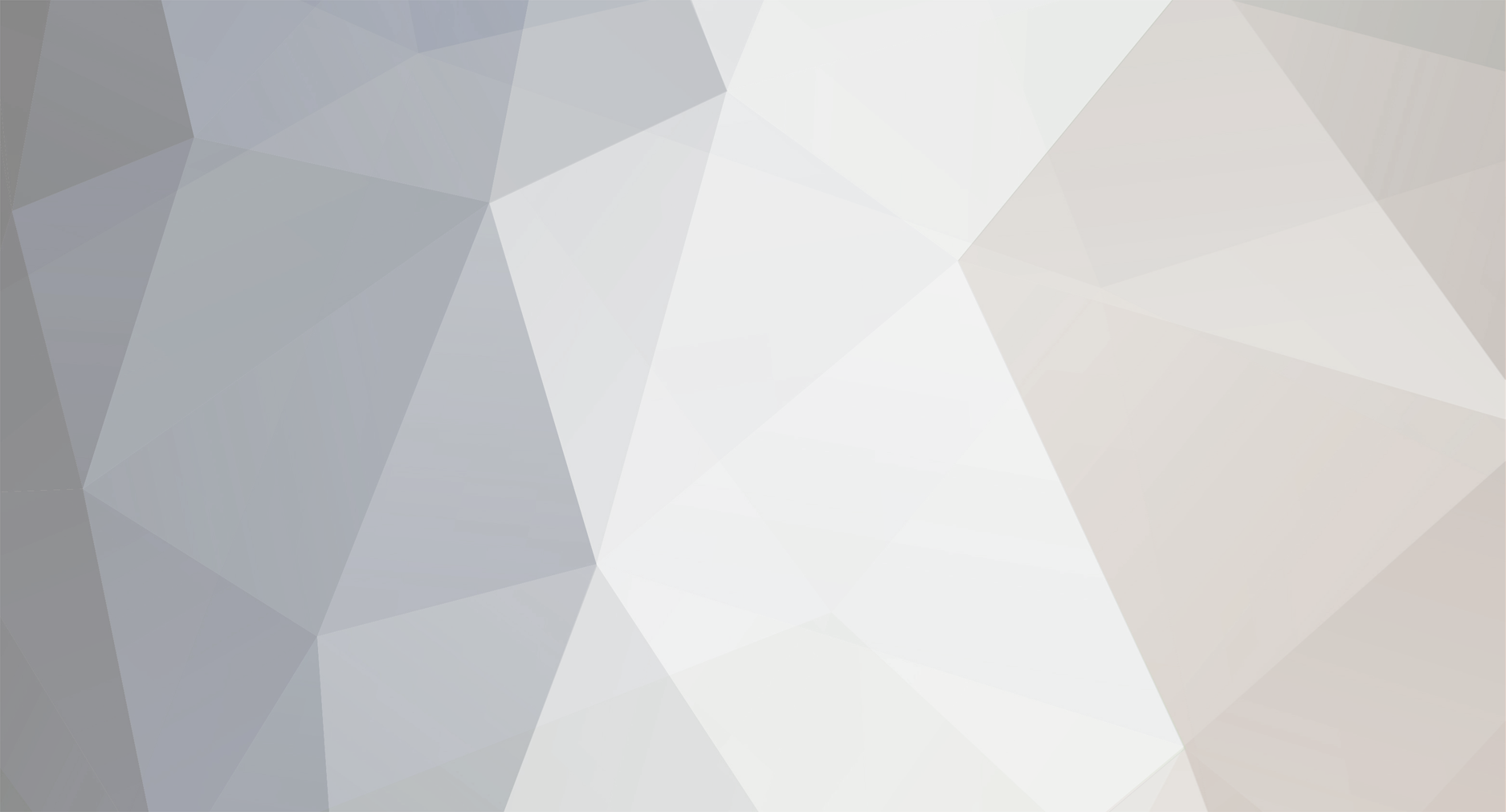
dcro2
-
Posts
489 -
Joined
-
Last visited
Never
Posts posted by dcro2
-
-
You could use the referer variable in php, $_SERVER['HTTP_REFERER'], but just remember that the referer can be spoofed very easily.
-
[!--quoteo(post=352665:date=Mar 7 2006, 06:04 PM:name=master82)--][div class=\'quotetop\']QUOTE(master82 @ Mar 7 2006, 06:04 PM) [snapback]352665[/snapback][/div][div class=\'quotemain\'][!--quotec--]$sql = mysql_query("SELECT * FROM Users");
$finalnumber = intval($_POST['sales']) * (0.75);
$finalnum = ($finalnumber + intval($row['salestodate']));
[/quote]
In this line:
$finalnum = ($finalnumber + intval($row['salestodate']));
you haven't fetched the results from:
$sql = mysql_query("SELECT * FROM Users");
You need to use:
[code]$sql = mysql_query("SELECT * FROM Users");
$row = mysql_fetch_assoc($sql);
$finalnumber = intval($_POST['sales']) * (0.75);
$finalnum = ($finalnumber + intval($row['salestodate']));[/code] -
[!--quoteo(post=352656:date=Mar 7 2006, 05:33 PM:name=sjones)--][div class=\'quotetop\']QUOTE(sjones @ Mar 7 2006, 05:33 PM) [snapback]352656[/snapback][/div][div class=\'quotemain\'][!--quotec--]
$record = mysql_fetch_row($result);
//...
<td><img src='http://uswebproducts.com/country_savings/uploads/$record'>
[/quote]
mysql_fetch_row returns an array, that's why the image displayed is:
"http://uswebproducts.com/country_savings/uploads/Array"
Use $record[0...1...2...etc] according to field order
It would be better to use mysql_fetch_array or mysql_fetch_assoc in your case. -
You could:
[code]$sql = mysql_query("SELECT * FROM Users WHERE user_id = '$userid'"); //I'm just guessing about the user_id.
$row = mysql_fetch_assoc($sql);
$finalnumber = intval($_POST['sales']) * (0.75);
$finalnumber = $finalnumber + intval($row['num_field']);
$sql = mysql_query("UPDATE Users SET num_field = '$finalnumber'");
if($sql) {
echo "Success!";
} else {
echo "Failed...";
}[/code]
Of course, replace 'num_field' with the field in your database. -
You could use substr() to limit the characters to 50, 100, etc.
[code]string substr ( string string, int start [, int length] )[/code]
So, I'm guessing in your case it would be:
[code]$message = substr($message, 0, 50);[/code]
For viewing only text, you could use the strip_tags() function
[code]string strip_tags ( string str [, string allowable_tags] )[/code] -
Should be that you are checking for 'submit' to be empty, while your submit button's name is 'vendor_id':
[code]if(empty($_POST['submit'])) { //If the submit button has not been set we will echo a welcome statement[/code]
[code]<input name='vendor_id' type='submit' value='Remove'>[/code]
So.. it is [b]always[/b] trying to remove the vendor_id 'Remove'...
It should be trying to remove $_POST['cat_id'] -
Your SQL syntax for DELETE is wrong, the correct syntax is:
[code]DELETE FROM table WHERE column = 'value';[/code] -
Well, first, are you sure your server has PHP installed? You might want to check that before anything.
And, second, are you using $_POST to get the values before this code?
Thirdly, you don't need to do $var = "$var", that's just wasting milliseconds :P -
If you are running community.php then why do you want to check if you're running community.php?
-
Hi there,
In order for PHP to parse (add the actual value of the var) you need double quotes around it:
$theData = ereg_replace('<b>', "<b> $person_name", $theData);
echo $theData;
OR:
$theData = ereg_replace('<b>', '<b> '.$person_name, $theData);
echo $theData; -
You copied the else two times, use this:
[code]include ('../connections/mysql_connect.php');
if(empty($_POST['submit'])) {
echo "<span class='content_blank'><strong>Welcome....</strong><BR></span>
<span class='content_blank'>You can enter a new Vendor by selecting the category that they belong<BR></span>
<span class='content_blank'>and entering the name of the new vendor</span><BR><BR>";
}else{
if(!empty($_POST['cat_id']) & !empty($_POST['name'])) {
$qry = mysql_query("INSERT INTO vendors (cat_id,vendor_name) VALUES ('".$_POST['cat_id']."','".$_POST['name']."');");
if($qry) { echo "<span class='content_blank'><strong>New vendor has been added</strong></span>"; }
else { echo "<span class='content_blank'><strong>Error inserting new vendor</strong></span>"; }
} else {
echo "<span class='content_blank'><strong>Error: something was left blank</strong></span>";
}
}
echo "<table width='100%' border='0' cellspacing='0' cellpadding='0'>
<tr>
<td>
<span class='content_blank'>Please choose a category and type in the name for the new vendor.</span><br><br>
<form method='post' action='csmag_admin.php?c=add_vendor'>
<select name='cat_id'>
<option>- Please select one -</option>\n";
$qry = mysql_query("SELECT * FROM categories;");
while($rows = mysql_fetch_assoc($qry)) {
echo "<option value='".$rows['cat_id']."'>".$rows['cat_name']."</option>\n";
}
echo "</select>
<input name='name' type='text' size=20>
<input name='submit' type='submit' value='Submit'>
</form>
</td>
</tr>
</table>";[/code] -
The <select>'s options have the cat_id as the value when you choose them, therefore you don't need to get it out of the database when you are inserting it. What you actually see in the select is the cat_name.
When you insert the new vendor, yes it will auto increment the vendor_id.
PS: the form needs to be submitted to [b]'cs_mag.php?c=add_vendor'[/b] not [b]'cs_mag?c=add_vendor.php'[/b] -
Um, well something like this?:
[code]if(empty($_POST['submit'])) {
echo "<span class='content_blank'><strong>Welcome....</strong></span>";
} else {
if(!empty($_POST['vendorid']) & !empty($_POST['name'])) {
$qry = mysql_query("INSERT INTO vendors (cat_id,vendor_name) VALUES ('".$_POST['vendorid']."','".$_POST['name']."');");
if($qry) { echo "<span class='content_blank'><strong>New vendor has been added</strong></span>"; }
else { echo "<span class='content_blank'><strong>Error inserting new vendor</strong></span>"; }
} else {
echo "<span class='content_blank'><strong>Error: something was left blank</strong></span>";
}
}
} else {
echo "<span class='content_blank'><strong>Error: something was left blank</strong></span>";
echo "<table width='100%' border='0' cellspacing='0' cellpadding='0'>
<tr>
<td>
<span class='content_blank'>Please choose a category and type in the name for the new vendor.</span><br><br>
<form method='post' action='csmag_admin.php?c=add_vendor'>
<select name='vendorid'>
<option>- Please select one -</option>\n";
$qry = mysql_query("SELECT * FROM categories;");
while($rows = mysql_fetch_assoc($qry)) {
echo "<option value='".$rows['cat_id']."'>".$rows['cat_name']."</option>\n";
}
echo "</select>
<input name='name' type='text' size=20>
<input name='submit' type='submit' value='Submit'>
</form>
</td>
</tr>
</table>";[/code]
Newsletter Signup Script Small Problem
in PHP Coding Help
Posted
INSERT INTO table_name VALUES (value1, value2,....)
not
INSERT INTO table_name SET column = value, column = value, ....
I don't know much about WordPress, so I'll leave it at that.