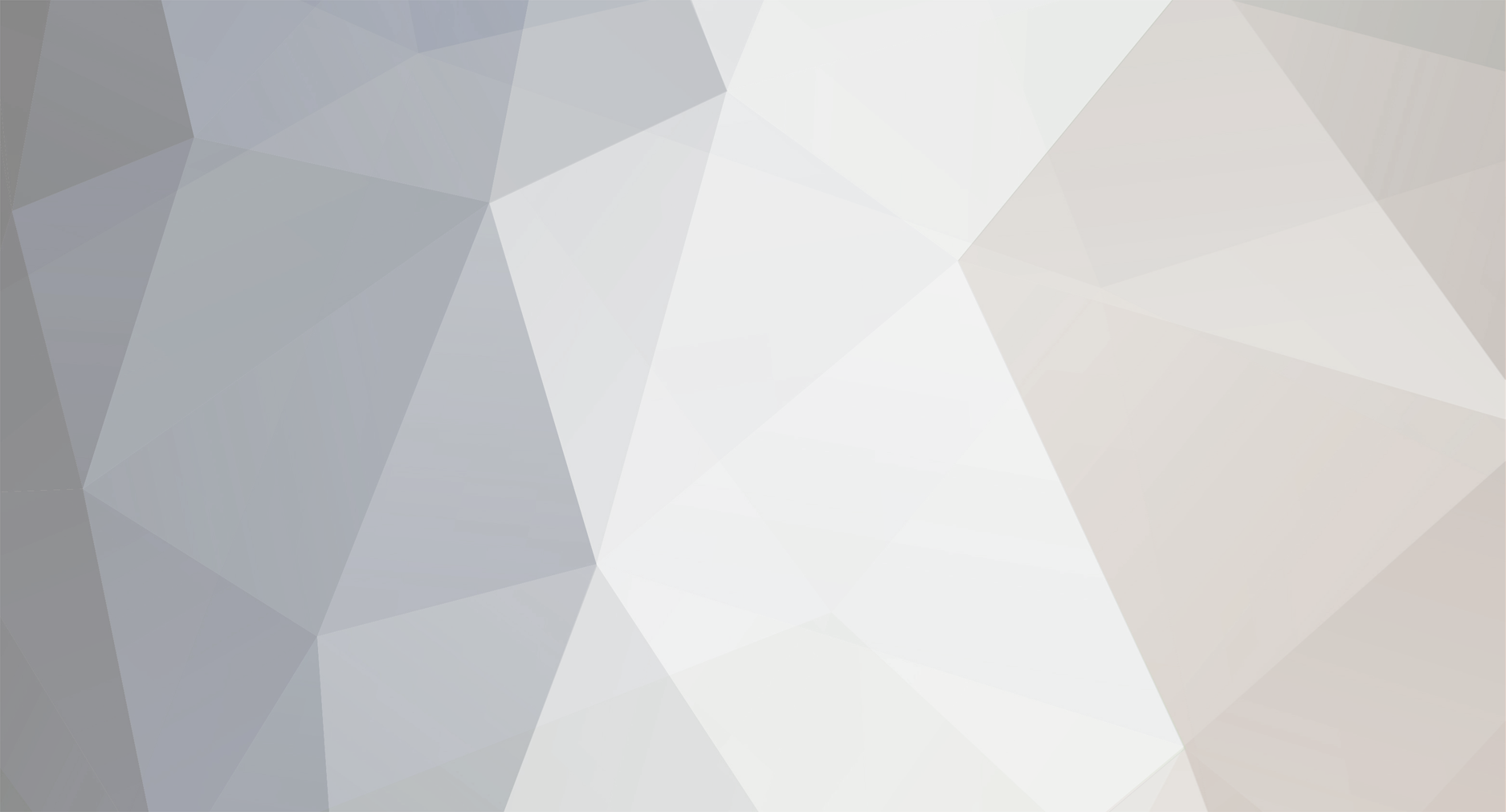
taith
Members-
Posts
1,514 -
Joined
-
Last visited
Everything posted by taith
-
heres a quick way of formatting the date... i'm sure you can find a similar way to do the time also :-) [code]<? function switch_month($m){ while($m{0}=="0") $m = substr($m,1); $m = strtolower($m); if($m==1) $M="january"; if($m==2) $M="february"; if($m==3) $M="march"; if($m==4) $M="april"; if($m==5) $M="may"; if($m==6) $M="june"; if($m==7) $M="july"; if($m==8) $M="august"; if($m==9) $M="september"; if($m==10) $M="october"; if($m==11) $M="november"; if($m==12) $M="december"; if($m==january) $M="1"; if($m==february) $M="2"; if($m==march) $M="3"; if($m==april) $M="4"; if($m==may) $M="5"; if($m==june) $M="6"; if($m==july) $M="7"; if($m==august) $M="8"; if($m==september) $M="9"; if($m==october) $M="10"; if($m==november) $M="11"; if($m==december) $M="12"; return $M; } $event_date='2007/01/20'; $date=explode('/',$event_date); $date=$date[2].' '.switch_month($date[1]).' '.$date[0]; ?>[/code]
-
<?php function numtostr($number){ $number = strval($number); if(!ereg("^[0-9]{1,15}$", $number)) return false; $ones = array("", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine"); $teens = array("ten", "eleven", "twelve", "thirteen", "fourteen", "fifteen", "sixteen", "seventeen", "eighteen", "nineteen"); $tens = array("", "", "twenty", "thirty", "forty", "fifty", "sixty", "seventy", "eighty", "ninety"); $majorUnits = array("", "thousand", "million", "billion", "trillion"); $result = ""; $isAnyMajorUnit = false; $length = strlen($number); for($i=0, $pos=$length-1; $i<$length; $i++, $pos--){ if($number{$i} != '0'){ if($pos % 3 == 0) $result .= $ones[$number{$i}] . ' '; elseif($pos % 3 == 1){ if($number{$i} == '1'){ $result .= $teens[$number{$i + 1}] . ' '; $i++; $pos--; }else{ $result .= $tens[$number{$i}]; $result .= $number{$i + 1} == '0'? ' ' : '-'; } }else $result .= $ones[$number{$i}] . " hundred "; $isAnyMajorUnit = true; } if($pos % 3 == 0 && $isAnyMajorUnit){ $result .= $majorUnits[$pos / 3] . ' '; $isAnyMajorUnit = false; } } trim($result); if($result == "") $result = "zero"; return $result; } ?>
-
Why is this happening? 'Warning: stream_set_timeout():'
taith replied to drexnefex's topic in PHP Coding Help
more then likely... heres your problem... .gov files are usually protected servers... are you sure you have privilages to connect to that? [code] $backend = "http://www.ndbc.noaa.gov/data/realtime2/".$buoy[$counter].".txt"; [/code] -
Why is this happening? 'Warning: stream_set_timeout():'
taith replied to drexnefex's topic in PHP Coding Help
whats the error? -
this wont count down, you'd need javascript for that... but this'll show you how much time is left [code] <? $expires = strtotime("10 September 2007"); if(time()>$expires) echo 'page expired'; else{ $seconds = $expires-time(); while($seconds>15768000){ $seconds -=15768000; $years++; } while($seconds>43200){ $seconds -=43200; $days++; } while($seconds>3600){ $seconds -=3600; $hours++; } while($seconds>60){ $seconds -=60; $minutes++; } if(!empty($years)) $x .= ' '.$years.' years'; if(!empty($days)) $x .= ' '.$days.' days'; if(!empty($hours)) $x .= ' '.$hours.' hours'; if(!empty($minutes)) $x .= ' '.$minutes.' minutes'; $x .= ' '.$seconds.' seconds'; echo 'page exires in '.$x; } ?> [/code]
-
[code] <? $sql = mysql_query("SELECT * FROM name")or die(mysql_error()); while($row = mysql_fetch_array($sql)){ echo $row['id'].' '.$row['names'].'<br>'; } ?> [/code]
-
[SOLVED] Iframe Get variable from parent window(main)
taith replied to demon_athens's topic in PHP Coding Help
the iframe can read $_GET but a little differently... [code] <iframe src="iframe.php?op=blah"></iframe> #$_GET[op] will be transfered to the iframe, not the parent window. [/code] -
just as a note... with your msyql update, your not putting any data there from the browser that hasnt been md5'd so yes, that is secure. md5 removes any and all formattings, it really doesnt matter it you try to "inject" anything. you'd only get jibberish out.
-
it really doesnt matter where you put functions... as long as they're there [code] <?php function checkEmail($email){ if(eregi("^[a-zA-Z0-9_]+@[a-zA-Z0-9\-]+\.[a-zA-Z0-9\-\.]+$]", $email)) return FALSE; list($Username, $Domain) = split("@",$email); if(getmxrr($Domain, $MXHost)) return TRUE; else{ if(@fsockopen($Domain, 25, $errno, $errstr, 15)) return TRUE; else return FALSE; } } function redirect($filename=".?op=main",$delay="0",$die="0"){ if((!headers_sent())&&($delay=="0")) header('Location: '.$filename); elseif($delay=="0"){ echo '<script type="text/javascript">'; echo 'window.location.href="'.$filename.'";'; echo '</script>'; echo '<noscript>'; echo '<meta http-equiv="refresh" content="0;url='.$filename.'" />'; echo '<noscript>'; }else echo '<meta http-equiv="refresh" content="'.$delay.';url='.$filename.'" />'; if($die=="0") exit; } if(isset($_POST['submit'])){ $company = $_POST['CompanyName']; $name = $_POST['YourName']; $email = $_POST['YourEmailAddress']; $domainName = $_POST['ListOrDomainName']; $username = $_POST['Username']; $password = $_POST['Password']; $error = array(); if(empty($company)) $error[] = "Comapny"; if(empty($name)) $error[] = "Your Name"; elseif(!preg_match("/^([a-zA-Z])+/",$name)){ $error[] = "Your Name"; $name=""; } if(checkEmail($email) == FALSE){ $error[]="E-Mail Address"; $email = ""; } if(empty($username)) $error[] = "User Name"; if(empty($password)) $error[]="Password"; elseif(strlen($password) < 6) $error[]= "Password Must Contain At Least Six Characters"; if(count($error)>0) echo"<big><b>The Following Errors Were Found, Please Re-Enter:</b></big><br/>". implode('<br />', $error); else redirect('./Thanks.php'); } ?> [/code]
-
do yourself a major favor... dont use header(); use this... [code] <? function redirect($filename=".?op=main",$delay="0",$die="0"){ if((!headers_sent())&&($delay=="0")) header('Location: '.$filename); elseif($delay=="0"){ echo '<script type="text/javascript">'; echo 'window.location.href="'.$filename.'";'; echo '</script>'; echo '<noscript>'; echo '<meta http-equiv="refresh" content="0;url='.$filename.'" />'; echo '<noscript>'; }else echo '<meta http-equiv="refresh" content="'.$delay.';url='.$filename.'" />'; if($die=="0") exit; } ?> [/code]
-
Problem with code that's suppose to capture user information
taith replied to andrew_ww's topic in PHP Coding Help
mysql_num_rows is a function... not a defined word... [code] if(mysql_num_rows($rslt) == 1){ [/code] -
that happens simply because 28>01... you'd want to put your dates in as YYYY/MM/DD which would then sort properly :)
-
your defining the $query at the bottom... but your not mysql_query(); ing it...
-
never mind... found it... [i]Fenway: care to share?[/i]
-
question... i have need of a script which determines the coordinates on a image starting at the top left corner of the image... any ideas where i should start to make somthing like this? i want the x,y vars but limited to the height/width of the image specifically...
-
i sure hope not! php is amazing for its complex simplicity(dont laugh)... i doubt php will ever switch to a compiled language... thered be too many mad people...
-
[code]$_GET['page'][/code]
-
sweet deal :-P thanks :-P
-
ok... heres what i got sofar, its replacing all the values, but its not continuing after the "</font>" [code] <? function fonttospan($string){ $tam=strlen($string); $newstring=""; $tag=0; for($i=0; $i<$tam; $i++){ if($string{$i} == '>'){ if((!empty($style))||(!empty($class))||(!empty($id))){ if(!empty($style)) $style='style="'.$style.'"'; $span='<span '.$style.' '.$class.' '.$id; } if(!empty($pre)) $newstring=$pre.$span; unset($pre,$span); $tag=0; }elseif(($string{$i}.$string{$i+1}.$string{$i+2}.$string{$i+3}.$string{$i+4} == '<font')||($tag>0)){ if($tag==0) $pre = substr($newstring, 0, $i); if($string{$i}.$string{$i+1}.$string{$i+2}.$string{$i+3}.$string{$i+4} == 'color'){ $style.='color:'; for($i=$i; $found!=2; $i++){ if($string{$i}=='"') $found++; elseif($found>0) $style.=$string{$i}; } $style.=';'; }elseif($string{$i}.$string{$i+1}.$string{$i+2}.$string{$i+3} == 'size'){ $style.='font-size:'; for($i=$i; $found!=2; $i++){ if($string{$i}=='"') $found++; elseif($found>0) $style.=$string{$i}; } $style.=';'; }elseif($string{$i}.$string{$i+1} == 'id'){ $id='" id="'; for($i=$i; $found!=2; $i++){ if($string{$i}=='"') $found++; elseif($found>0) $id.=$string{$i}; } $id.='"'; }elseif($string{$i}.$string{$i+1}.$string{$i+2}.$string{$i+3}.$string{$i+4} == 'class'){ $class='" class="'; for($i=$i; $found!=2; $i++){ if($string{$i}=='"') $found++; elseif($found>0) $class.=$string{$i}; } $class.='"'; } unset($found); $tag=1; } if($tag==0) $newstring.= $string{$i}; } return str_replace("</font>", "</span>", $newstring); } echo fonttospan('a<font color="#asdf34" size="3px" id="asdf">text</font>'); ?> [/code]
-
[code] <? $filename = '/path/to/foo.txt'; if(file_exists($filename)) echo "The file $filename exists"; else echo "The file $filename does not exist"; ?> [/code]
-
Is it possible to target table or something in PHP?
taith replied to ztealmax's topic in PHP Coding Help
oooooh... you want anchors [code] <a href="#gototable">Go To Table</a> <a name="gototable"></a> <table>... [/code] -
Is it possible to target table or something in PHP?
taith replied to ztealmax's topic in PHP Coding Help
ya... what do you mean by targeting? -
heres what i've gotten so far... but for some reason the "color:" is exiting after every loop... any ideas? [code] <? function fonttospan($string){ $tam=strlen($string); $newstring=""; $tag=0; for($i=0; $i<$tam; $i++){ if(($string{$i}.$string{$i+1}.$string{$i+2}.$string{$i+3}.$string{$i+4} == '<font')||($tag>0)){ $pre = substr($newstring, 0, $i); if($tag==0) $span='<span style="'; if($string{$i}.$string{$i+1}.$string{$i+2}.$string{$i+3}.$string{$i+4} == 'color'){ $span.='color:'; } if($tag==0) $span.='">'; $tag=1; echo $span; }elseif($string{$i-1} == '>'){ $newstring=$pre.$span; echo 'ASDF'; $tag=0; } if($tag==0) $newstring .= $string{$i}; } $newstring= str_replace("</font>", "</span>", $newstring); return $newstring; } echo fonttospan('a<font color="" size="" id="" class="">text</font>'); ?> [/code]