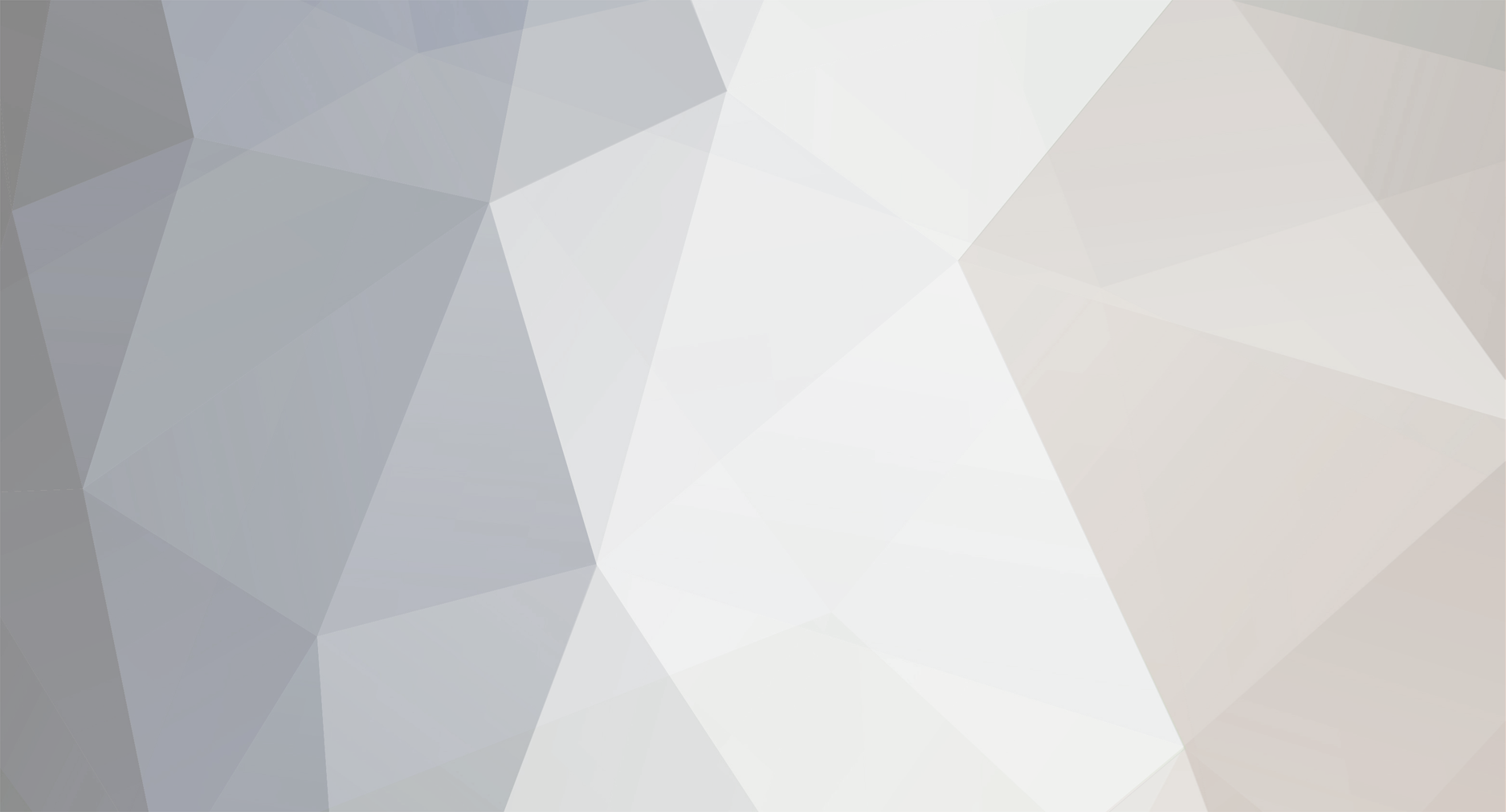
rshadarack
Members-
Posts
29 -
Joined
-
Last visited
Never
Everything posted by rshadarack
-
Implemented it, and it wasn't bad, though it still makes me feel a little... dirty.
-
From my understanding, hidden iframes are used to have persistent data in javascript, but you can't use one to transfer data from javascript to php. Is that correct? I didn't realize this before, but the size of the arrays I have to transfer can be determined when the php script is running. So I can create a hidden form by just using php to make html instead of modifying the dom, and then submit it by javascript code (I think?) which will send the data needed in POST.
-
I have a bunch of data in some client-side javascript code that I want to get to php code. I would typically just manually build the url string to send it over in GET, but because of the amount of data, this is not possible. So as far as I know, the only other method I have is to use POST. And the only way I know how to do this is to build a form by adding elements to the dom and submit it. However, this is rather ugly, is there any easier way?
-
Oy, don't know why I haven't heard of that. Thanks.
-
I'm attempting to build a site with a general php library, with different files such as database.php for database functions and string.php for string functions. Some of my database functions require string functions, so I include string.php in database.php. Now add on about 10 different library files, and things start to get complicated. I have no clue (without looking in my library) which files include other files. So I want to be able to include a file twice without getting a redeclaration error. Is there a php'y way to do this? I could mimic C++ and do: [code] if (!isset(DATABASE_PHP)) { DATABASE_PHP = 1; include("string.php"); ...rest of database.php code... } [/code] In all of the files. It's fairly ugly, but it works. Is there a better way?
-
I have a set column in a table in mysql. I wish to get a list (preferably some sort of array, but I could parse a list of text as well) of possible entries for that set. What is the query to do so?
-
Is there a way for me to take backslashes from a form input, and replace it with \ (the html '\' code)? By the time I retrieve data using post, the backslashes are gone already.
-
I'm using the following code to save a table to a matrix: [code]function tableToArray($tableName) { $table = mysql_query("SELECT * FROM $tableName"); $columns = mysql_query("SHOW COLUMNS FROM $tableName"); for($i = 0; $i < mysql_num_rows($columns); $i++) { $result[0][] = mysql_result($columns, $i); } $n = 1; while ($row = mysql_fetch_array($table)) { for ($x = 0; $x < sizeof($row)/2; $x++) { $result[$n][] = $row[$x]; } $n++; } return $result; }[/code] Which works fine. But notice in the last for loop (nested in the while loop), I have $x < sizeof($row)/2. I did this because I found that sizeof was always returning twice the amount of columns that I had. Before that, I was using a foreach statement, but it was saving data values to my matrix like: dataVal1 | dataVal1 | dataVal2 | dataVal2 | dataVal3 | dataVal3.... Doubling each value. I was wondering if anyone knew why this was happening.
-
Hah. Half the time I do things like: $num = $_SESSION['num']; To make it easier to code. Thanks, that fixed the problem.
-
I have the code: [code] echo "Location: $location<br>"; echo "Session: ".$_SESSION['location']."<br>"; $_SESSION['location'] = ""; echo "Location: $location<br>"; [/code] Which produces the output: [code] Location: main.php Session: main.php Location: [/code] Why is this happening? The only reason I can think of is that when I use the assignment operator, it's assigning a reference. But I didn't think php did that. How can I get it so that I take the value out of the session, but store it in a temporary variable?
-
What I didn't realize before was that my header.php script also includes another script. So if I use absolute addressing, it goes through http, and thus, all the php code is ignored (if it isn't echo'ed). So absolute addressing won't work. Another thing I could do is make it so that any file that uses header.php is always one directory below it, and thus, the addressing "../someFolder/someFile" from within header.php will always work. Edit: I never thought of doing such a thing, but keeping file address in php variables would make scripts much for flexible and easier to maintain/update. Thanks.
-
Most likely. If I change that directory name, then I have no option but to go through my code changing all the urls. What I wish to do is make it more flexible, so if I say move the entire site into a directory, everything will still work just fine using relative addressing. As I said before, changing the current working directory would solve all my troubles. Anyone know of a way to do this through php? I can't seem to find any functions of this sort, so I'm thinking the answer is no.
-
I know that, I addressed in the OP. I don't like this option because if I decide to move my files around, I will have to go through pages and pages of url's, changing them all. If I have no other option, it will probably be what I will do. But I would like to know if there is a better option.
-
What I have right now is: http://www.website.com/header.php - Writes the heading for every page on the site Now I like to group similar files into folders, so I also have: http://www.website.com/folder/file1.php And I would like to use the header on it, so I have the code: require("../header.php"); Problem is, header.php uses relative addressing for images, such as: <img src="/images/image1.jpg"> So when in file1.php, it includes header.php, header.php then looks for images in http://www.website.com/folder/images instead of http://www.website.com/images. Now changing the current working directory would solve this problem quite simply. But I can't seem to find such a function for php. Can php do this? If not, can something else? And if not, are there any other work arounds besides not using folders, using absolute address, and copying all the images to http://www.website.com/folder/images? While these would work, they aren't really good solutions.
-
I keep getting the warning: Warning: session_start(): Cannot send session cookie - headers already sent by... Everytime it comes to a page with session_start() in it. Why is this? Also, I'm not sure if it's the same problem, but it doesn't seem to be sending data. My code: [code] <?php session_start(); require("functions.php"); if (!validate($_POST['name'], $_POST['password'])) { echo "Login error, please try again. You will be redirected back to whence you came..."; $_SESSION['error'] = 1; echo "<script language=\"JavaScript\"> window.location=\"index.php\"</script>"; } else { echo "Login successful. Redirecting to the admin page..."; echo $_POST['name']." ".$_POST['password']; $_SESSION['name'] = $_POST['name']; $_SESSION['password'] = $_POST['password']; echo "<script language=\"JavaScript\"> window.location=\"main.php\"</script>"; } ?> [/code] Which validates it successfully. However, when I send the page to main.php: [code]<?php session_start(); require("functions.php"); if (!validate($_SESSION['name'], $_SESSION['password'])) { $_SESSION['error'] = 1; echo "Error<br>"; echo "\"".$_SESSION['name']."\" \"".$_SESSION['password']."\"<Br>"; echo "<script language=\"JavaScript\"> window.location=\"index.php\"</script>"; } ?> [/code] It fails and sends me back to index.php. As you can see, I echo'ed out the data, and I get: "" "" So why is this happening?
-
I'm not quite sure, but I think (s)he's asking how to represent a card in php. The way I did it when I made a game in C++ a while back is to have the integer part be the face value and the decimal part be the suit. For example: 2.1 Would be a 2 of (let's say) clubs. 13.3 Would be a king of hearts. 9.1 Would be a 9 of clubs. And so on. And if you aren't sure how to go about checking for wins, I don't think you're ready to make a game like poker. I would recommend trying blackjack first, if you're new to php and programming in general.
-
Did I word my question poorly? Can no one understand it? Or maybe there isn't a way to do this? Just in case: What I wish to do is have a php script in one frame on a page get the current URL of another frame.
-
Thanks! Exactly what I was looking for.
-
I wish to add data via $_POST without using a form, but I can't seem to find a function that will allow me to do this. Is there one? If not, how else can I go about passing hidden data between pages?
-
I wish to be able to detect what page one of my frames is on from a different frame. Is there any way to do this?
-
Thanks. After a bit more searching, I found a good page on local/global variables. My made the assumption that php works like C++, it first looks for local, then looks for global, and if both aren't found, it creates a new local variable. But this is wrong. It first looks for local, then creates a new local if it isn't found. That is, unless you use the global keyword. So doing: global $pages; As the first line in the function makes my code work. And for this, you do need to declare the array in the global scope. Or at least I think you do... Edit: Oh, and for that first question, I could have swore I was losing values when ending one php tag and starting another. But now it seems like I'm not. I think I'm going out of my mind.
-
I know a bunch of programming languages such as C++ and Java, and while php is quite similar, the way local/global variables and functions seems to be much different. First of all, is there anyway I can have: [code] <?php $someValue = 5; ?> ...html and junk... <?php $x = $someValue; ?> [/code] And have values carry over? I figure the answer is no. Second question: I have functions defined like this, which sets up a matrix (aray of arrays) for a menu with submenus: [code] <?php $pages = array(); function addMenuHeader($name) { $pages[] = array($name); } function addMenuItem($name) { $n = sizeof($pages); $pages[$n-1][] = $name; } addMenuHeader("Home"); addMenuHeader("About_Us"); addMenuItem("Mission"); addMenuItem("Management"); addMenuItem("Staff"); addMenuItem("History"); ?> [/code] But the value in $pages always gets lost as soon as a function exits. Why, and how do I fix it?
-
[!--quoteo(post=385762:date=Jun 19 2006, 03:25 PM:name=wildteen88)--][div class=\'quotetop\']QUOTE(wildteen88 @ Jun 19 2006, 03:25 PM) [snapback]385762[/snapback][/div][div class=\'quotemain\'][!--quotec--] If its hardcoded into the script itself then it should be secure, but make sure you change the password once or twice a week, just incase. I only gave you the database as an example. [/quote] Thanks.
-
I don't understand the need for a database. I am only creating access to 1, possibly 2 users. Is there any danger if I hardwire the password into the php script?
-
When creating a php login page, my first thought was to just have $username = "JoeSmith" and $password = "JoeSmith77" with a simple form which asks for input, then checks using these values. Then the page would reload itself, passing an "isValidUser" or something like that, and the same page would then show it's actual content. Since the php is always processed by the server and all php commands (besides output) are removed, I figured this would be secure. Then I go around looking an there are all these complex ways of password protecting a site. So is this not the case? Basically, why is this not a secure way of password protection?