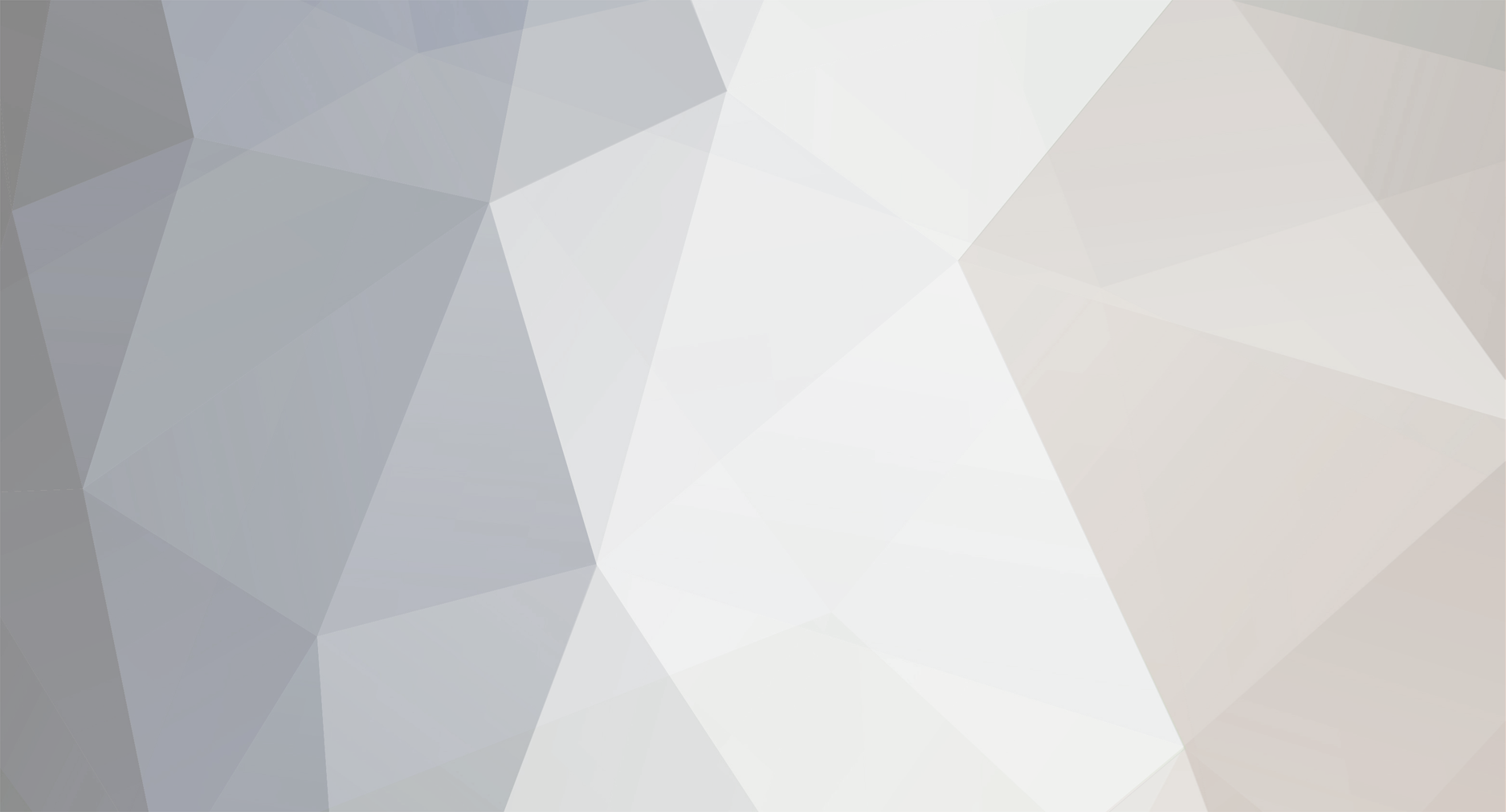
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
The OP is talking about guessing passwords, not decryption. You can guess md5() passwords, even if you cannot decrypt md5. maxcell, yes you can. What approach do you want to take to generating the dictionary? Generating and testing all 7 and 8 character strings is not feasible on today's hardware, so you will need to choose a strategy of some sort.
-
Try this. There may be a few typos as I am unable to test it, but you should be able to see the general idea. I am placing all the output into a variable $html, then returning that variable at the end. public function content_productshome($directories, $booksquery, $cardsquery, $categoriesquery) { //Create array from categories query $num_categoriesresults = $categoriesquery->num_rows; $categories = array(); for ($i=0; $i <$num_categoriesresults; $i++) { $currentcatrow = $categoriesquery->fetch_assoc(); $categories[] = $currentcatrow; } $html = ' <p> books: </p> <table width="80%" border="1" cellspacing="3" cellpadding="3"> <tr> <td>Title</td> <td>Category</td> <td>ISBN</td> <td>Price</td> </tr>'; $num_bookresults = $booksquery->num_rows; for ($i=0; $i<$num_bookresults; $i++) { $currentbookrow = $booksquery->fetch_assoc(); $html .= "<tr> <td>{$currentbookrow['Title']}</td> <td>{$categories[$currentbookrow['CategoryID']]}</td> <td>$currentbookrow['ISBN']}</td> <td>{$currentbookrow['Price']}</td> </tr>"; } $html .= '</table> <p> cards: </p> '; return $html; }
-
[SOLVED] php and postgres help, real easy for vets
btherl replied to Diceman's topic in PHP Coding Help
The question wasn't simple. But I think I understand what you are asking. Do you want something like this: $first_names = array(); $last_names = array(); $ages = array(); $sql = "SELECT blah blah blah"; $res = pg_query($pg_conn, $sql) or die("Query $sql failed"); while ($row = pg_fetch_assoc($res)) { $first_names[] = $row['first_name']; $last_names[] = $row['last_name']; $ages[] = $row['ages']; } print "Row 4 is {$first_name[4]} {$last_name[4]}, age {$ages[4]}\n"; Edit: Note that the first row will be numbered 0, not 1 -
[SOLVED] Need query to return results that start with a specified letter
btherl replied to mhoram's topic in PostgreSQL
This query may need to scan the whole table, depending on the locale your database was created in. Use "explain" to check the query plan to be sure. -
Ok.. on the assumption that each record is exactly 60 lines, you could do something like the following: $fp = fopen('file.txt', 'r') or die("Could not open file\n"); $line_count = 0; $record = ''; $records = array(); while (!feof($fp)) { $line = fgets($fp); $line_count++; if ($line_count < 60) { # Add to current record $record .= $line; } else { # Completed one record $records[] = $record; $record = ''; $line_count = 0; } }
-
The answer is here: http://dev.mysql.com/doc/refman/5.1/en/insert-on-duplicate.html It will update one row at random. Naturally, doing this is not recommended
-
I would solve this by storing the last processed line number somewhere. You can store it in a file, or in the database. Then when you re-read the file, you skip lines until you get past the last processed line number. Alternatively you could store a byte offset from the start of the file, which allows you to fseek() to the exact location that any new lines will start. http://sg2.php.net/manual/en/function.fseek.php
-
Can you tell us why you want to find a new line character? There is probably a more specialized solution for the task you want to do.
-
What happens when you select more than one specialization? And how does that differ from what you expected to happen?
-
Impossible to copy from to destination directory.
btherl replied to t_machine's topic in PHP Coding Help
Can you give more details about the upload form? Who wrote it? What does it ask you for? Does the error occur on every file you try to upload? -
There is a section on character sets and collation in the manual here: http://dev.mysql.com/doc/refman/5.1/en/charset.html I can't you more than that, due to lack of mysql experience.. but I suspect that setting the appropriate collation order will help. In particular look here: http://dev.mysql.com/doc/refman/5.1/en/charset-collate.html
-
Table users id name 1 Alice 2 Bob Table hitpoints id hp 1 50 3 20 SELECT name, hp FROM users JOIN hitpoints ON (users.id = hitpoints.id) This will match up the tables by id, allowing you to match names from one table with hit points from the other.
-
[SOLVED] Need help printing series numbers between $x and $y
btherl replied to rcorlew's topic in PHP Coding Help
The problem is that you are doing $x++ instead of $i++ -
The "END" of a heredoc must be in the first column, it can't be indented.
-
I put your script in a file and ran php -l with version 4.3.10 and 5.1.4, and it said your syntax is perfect..
-
For the purposes of security, you might want to use strip_tags(): http://sg.php.net/manual/en/function.strip-tags.php Regarding security, calling htmlentities() once should ensure that html is displayed, rather than interpreted by the browser. You should see the bold tags instead of seeing bold text. If you really want to see the htmlentities() output in the browser, call htmlentities() twice.
-
Did you view the source? The rendered HTML (which you see in the browser) will have the html entities converted back to normal. To see the source, look in the View menu for something like "View Source"
-
It would be easier if you used a date data type instead.. but since you've got integers (are they integers? or varchars?), you can do $cur_month = date('m'); $cur_year = date('Y'); $sql = "SELECT * FROM table WHERE user = 'chris' AND (year > $cur_year OR (month > $cur_month AND year = $cur_year))"; You can also fetch the date from within mysql, but I'm not familiar with how to do that. Edit: Oops.. fixed silly mistake
-
If you want to keep only the first record for each ip each day, do a select before your insert. If the select matches a row, don't do the insert. That's basically the way to do it If you're worried about efficiency, you could set a cookie or a session variable that lets you remember that you've already recorded that user that day. The variable could record the date and time of the last record inserted. Then you can check if the last record was inserted on the same day or not.
-
Add "echo $sql" just after you set $sql. If it's not clear what's wrong with it, then post it here for us to take a look at.
-
This sounds like a job for left join! SELECT name FROM roster_members LEFT JOIN users ON (roster_members.name = users.name) WHERE users.name IS NULL Why does it work? A left join will keep rows from the left table even when there is no match in the right table. It will fill the right table with NULL for every column for the rows that don't match. So to check for "no match", you just check if any column from the right table is null. This won't work if that column really can be null of course, but I assume users.name is never null.
-
Default values are only used when you leave the field unspecified. That is, if you don't mention it at all in your insert statement. For your case, it may be better to set the default value in php like this: if (empty($svideoifwalla)) { $query1 = "INSERT INTO `mainfilelist` ( `fileifwalla` ) values ( 'your_default_value' )"; } else { $query1 = "INSERT INTO `mainfilelist` ( `fileifwalla` ) values ( $svideoifwalla )"; } empty() will tell you if nothing was entered into the form field. An empty form field will always have a blank string value, rather than null. If you do it this way, it doesn't matter if your field is null or not null, and the default value (in sql) is not used.
-
mysql_fetch_array(): not a valid mysql result resource
btherl replied to scottreid1974's topic in PHP Coding Help
Scott, look at this carefully: $dbase = mysql_select_db($db) or die( "Unable to select database"); That is the call to mysql_select_db(), not mysql_query(). Modify your script with this. In your latest post your script still did not have this modification. You MUST add this. Even if it doesn't change the error message you get. All of mysql_connect(), mysql_select_db() AND mysql_query() MUST have an "or die()" after them. mysql_fetch_array() and mysql_num_rows() MUST NOT have an "or die()". Don't add it to those. Also, please post your EXACT error message each time. "No database found" is totally different from "No database selected". They are not even remotely the same. We need the exact words. Please use copy and paste to show us the error message. As for the details of which host, user, pass and db to use, you should ask your hosting company about that. -
Are you aware that there is a difference between an empty string and null? Edit: To insert null into a database, you must write the word "null", or leave the column unspecified altogether. If you insert a blank string value into the database, then you will get a blank string, not null.
-
Wow, 8 posts before you posted again Was your question answered?