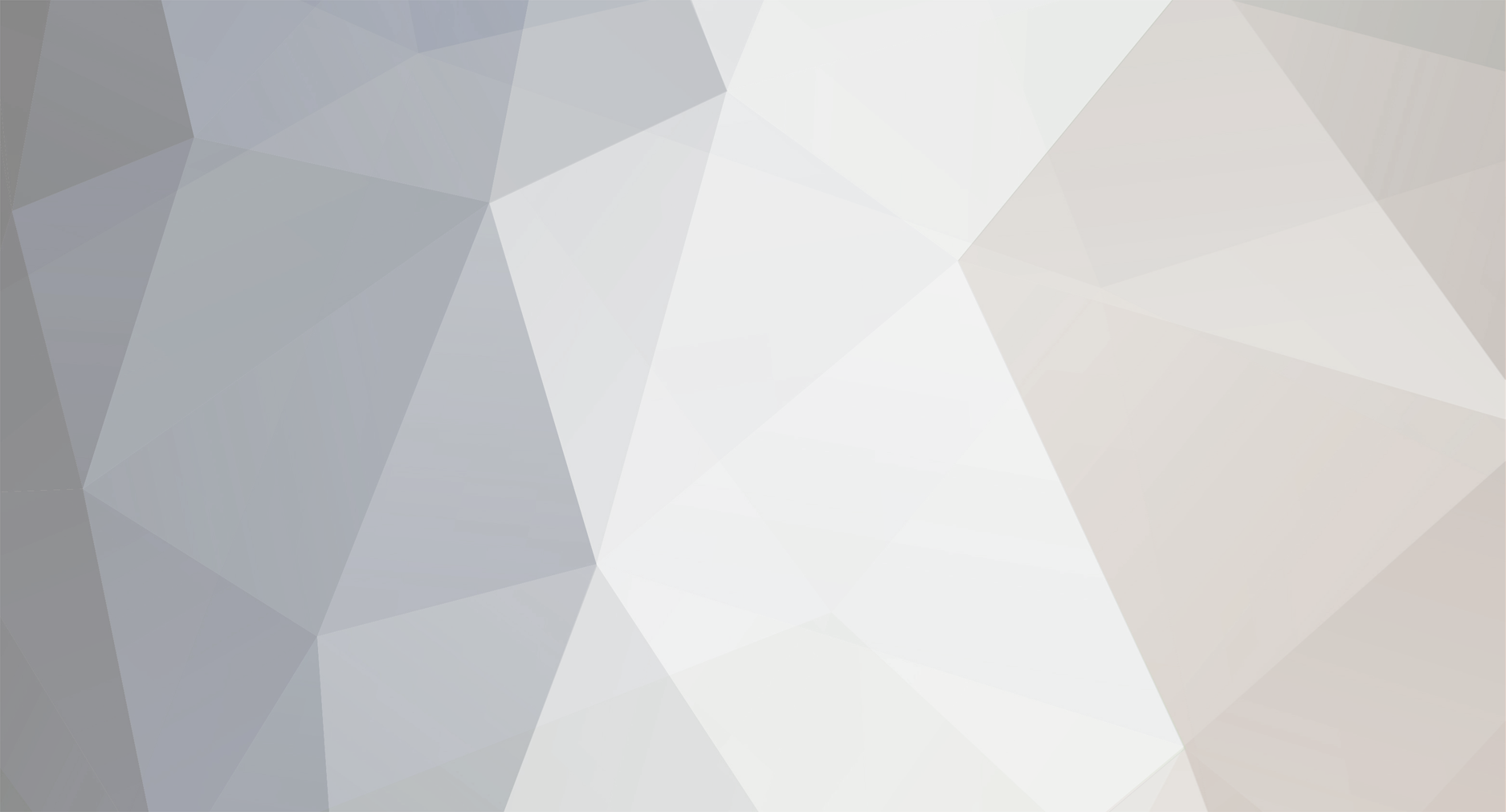
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Does your hosting provider support postgres?
-
How did you trace it to that function, and exactly which line of the function does it hang on?
-
Using cookies to pull data from multiple databases on two servers
btherl replied to bruckerrlb's topic in PHP Coding Help
Sessions are very much like cookies, since they use cookies to function. But the data is stored on the server, meaning the user can't modify it. Hmm.. to answer your question, any time you want to store data with a cookie, you could put it in $_SESSION instead. It will work just as well. The only thing you lose is being able to specify things like path, domain and expiry time. Instead you'll have to handle those things yourself. -
Yes, it's valid use of mysql_real_escape_string(). Like dbo says, it doesn't hurt and often helps to validate the input first.
-
Problem deleting from sql database - but same script works on other site?
btherl replied to Edward's topic in PHP Coding Help
Glad to hear it helped I would recommend you do it like this, as you don't want to expose such information to a user who may be a potential hacker: if ($result_2 = mysql_query($sql_2)) { echo '<p>Your file has been deleted.</p>'; } else { echo '<p>Unfortunately your file was only partially deleted. The webmaster has been notified of the problem.</p>'; $msg = "Query $sql_2 failed! The error was " . mysql_error(); mail ('admin@host.com', "Query failure!", $msg); } For debugging purposes though, you can just put this inside the else: print "Error in $sql\n" . mysql_error(); -
Using cookies to pull data from multiple databases on two servers
btherl replied to bruckerrlb's topic in PHP Coding Help
It's session_start(), not start_session() The session is remembered by storing a cookie in the client's browser (I see from your first post that you are familiar with cookies). That cookie is then interpreted by session_start() every time a new request is made. The cookie has a code in it which tells php to look for a file on the server storing the user's session data (the contents of $_SESSION) All those details don't matter though, as long as they work properly. As long as you call session_start(), you will have access to $_SESSION data that stays the same over multiple requests from the same user. -
[SOLVED] How do I determine the data type of any variable?
btherl replied to obay's topic in PHP Coding Help
The most likely reason is that you accidentally changed the value of $var and didn't realize it. Then you didn't realize when you fixed the code that changed it either The other most likely reason is a typo in a variable name, but that's unlikely for such a short name. -
[SOLVED] Multidimensional Arrays in HTML POST Forms
btherl replied to kael.shipman's topic in PHP Coding Help
Deep multi-dimensional arrays may be pushing the limits of something. An option may be to serialize the array before submitting it, or otherwise encode it yourself. Google shows that a few people have written simple javascript implementations of a php serializer. -
Try adding var_dump($_FILES) to the top of your script. That will show you the entire contents of the array. Then you can use that to determine what validation to add.
-
The two most likely problem I can think of are 1. The arguments you gave to mail() are not valid. This can be tough to track down. You can try simplifying the arguments until it starts working. Or 2. Your email provider is blocking the email for some reason. Edit: Your email provider may simply have delayed the email, due to suspicion of spam. If this is the case, you'll get the emails but only much later.
-
[SOLVED] File delete from check-boxes with dynamic not working
btherl replied to anolan13's topic in PHP Coding Help
Try adding var_dump($_POST) at the top of your script, to see what is in the $_POST array. -
[SOLVED] How do I determine the data type of any variable?
btherl replied to obay's topic in PHP Coding Help
Can you post a short script reproducing the problem? If that's not possible, please post your entire script. -
[SOLVED] How do I determine the data type of any variable?
btherl replied to obay's topic in PHP Coding Help
The function is http://sg.php.net/manual/en/function.gettype.php I'm interested to see what it returns for your mystery variable.. -
[SOLVED] session array showing empty in some files?
btherl replied to sayedsohail's topic in PHP Coding Help
If the session data isn't set, it's probably because the browser did not send the cookie. Are you using firefox or IE? We can try debugging it from the browser side. -
Using cookies to pull data from multiple databases on two servers
btherl replied to bruckerrlb's topic in PHP Coding Help
I think you've got the general idea. I'll explain in a bit more detail: The session is used primarily to store the user's data. It may have other information where it's convenient to store it there. So lets say a user wants a list of all the websites they have registered under the "foo" system. They make the request, sending "mode=list_websites&system=foo" from a form. session_start(); if (!empty($_SESSION['username'])) { # Ok, user is logged in as $_SESSION['username'] } else { # Not logged in, redirect to login page header("Location: http://www.foo.com/login.php"); exit(0); } if ($_REQUEST['system'] === 'foo') { # Ok, it's a foo request. require_once('foo.php'); do_foo(); } foo.php will contain: function do_foo() { if ($_REQUEST['mode'] === 'list_websites') { # We need data from foodb to complete this request ... fetch data from foo db, using $_SESSION['username'] to determine which data to fetch ... } if ($_REQUEST['mode'] === 'add_website') { # Add a website to foo db ... } } In the $_SESSION array you can also store other data, such as the user's access priveliges, and display settings such as "rows to display per page". -
[SOLVED] session array showing empty in some files?
btherl replied to sayedsohail's topic in PHP Coding Help
Can you copy and paste your script which is not able to access the session data? -
Using cookies to pull data from multiple databases on two servers
btherl replied to bruckerrlb's topic in PHP Coding Help
I'll tell you about the system I'm managing now. It has three front-end servers, all exact copies of each other, to handle load and for redundancy. These make requests to up to around 10 databases in total (usually only 2 or 3 databases for each individual request). The login data is stored in database 1, and after a user logs in, data is stored in their session to record that. Then data is fetched from the other databases, depending on what they request. Authentication after logging in is handled by checking the session. Some database requests go directly (eg with mysql_query()) and others go via web interfaces to the database, using CURL to make the requests. Then all the data is formatted and displayed nicely. -
Using cookies to pull data from multiple databases on two servers
btherl replied to bruckerrlb's topic in PHP Coding Help
I think you will find everything much easier with sessions: http://sg2.php.net/manual/en/ref.session.php They allow you to store a user's data between scripts without needing to worry about the details of cookies. A typical use is to store the logged in user's username in a session variable, so you know they have logged in. BTW, the structure you describe (separate server for display and for data storage) is actually quite normal. I don't see any problems with it. -
Can you give an example of what you want to do? I am guessing you either want to reorder your columns, or you want to rotate your data to the next column on the right.
-
[SOLVED] session array showing empty in some files?
btherl replied to sayedsohail's topic in PHP Coding Help
Are you a perl user? In PHP, you can't leave out the brackets from a function call. It just won't work. The only exceptions are builtins like print, echo and exit. -
Problem deleting from sql database - but same script works on other site?
btherl replied to Edward's topic in PHP Coding Help
Change all your mysql_query's to look like this: $result = mysql_query($sql) or die("Error in $sql\n" . mysql_error()); That will give you useful information about query failures. -
I don't think you can. You will need to store the expiry time on the server (which you can, since you created the cookie in the first place)
-
Call-time pass-by-reference? Deprecated Ampersand Question.
btherl replied to fubowl's topic in PHP Coding Help
referencing isn't built for php4, it just has slightly different rules between php4 and 5. There was a typo in my example. After fixing that, it works the same in both 4 and 5. $a = 1; foo(&$a); print "a = $a\n"; bar($a); print "a = $a\n"; function foo(&$a) { $a = $a + 1; } function bar($a) { $a = $a + 1; } -
file() returns an array. You can't subtract an array from a number. You can verify with the following script: print 1 - array(1);
-
Yep, sounds right. I would do it this way. Add this to the form: <input name=song_id type=hidden value="<?php echo $song_id;?>"> Same as you've done with the other variables. Then you can access it as $_POST['song_id'] for your SELECT query.