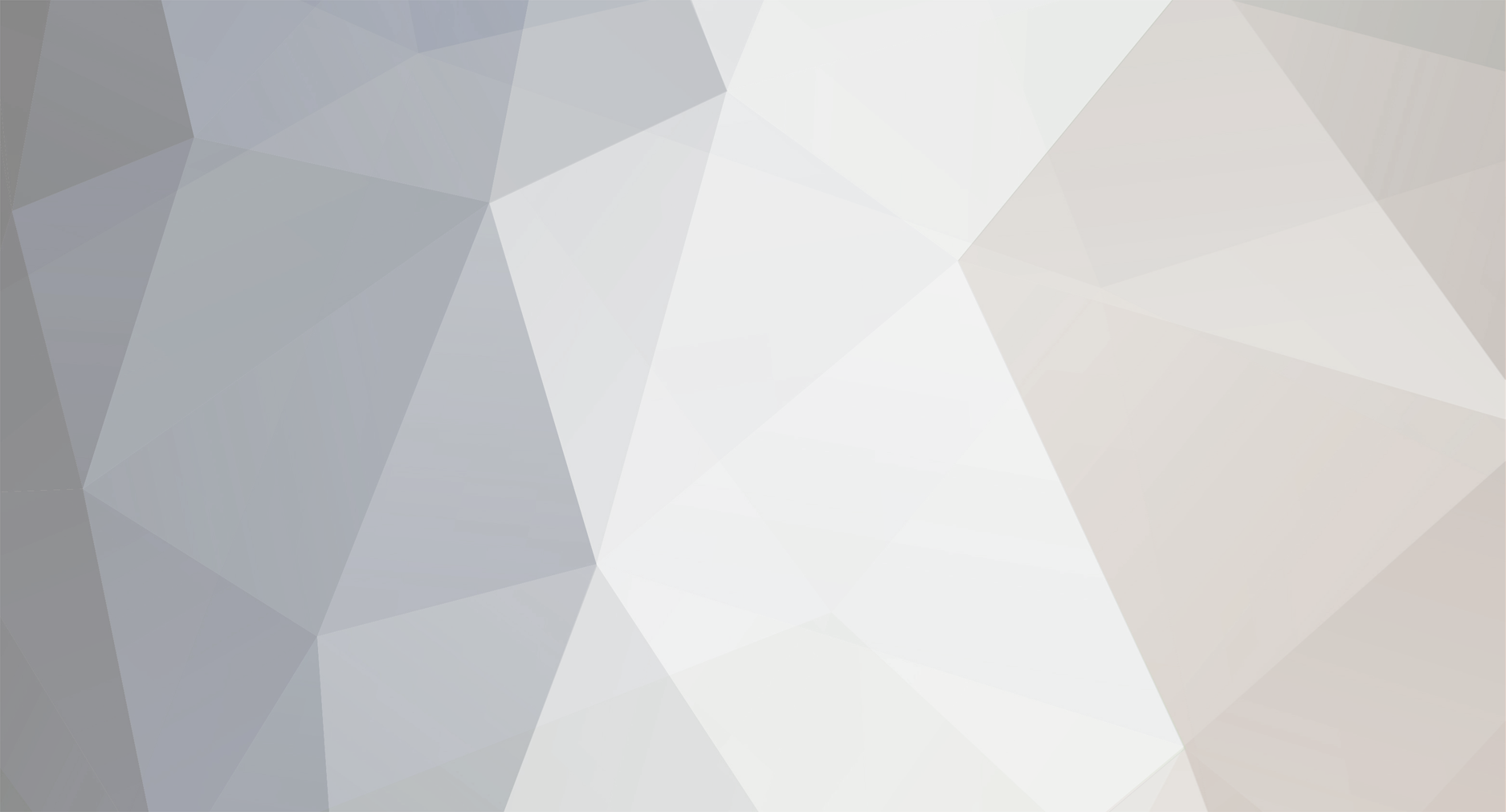
sKunKbad
-
Posts
1,832 -
Joined
-
Last visited
-
Days Won
3
Posts posted by sKunKbad
-
-
I've been successful blocking most spam with a CSRF type form token. Making javascript mandatory also gets rid of a lot of bot related spam, but the token is the real gem when it comes to blocking bots. You just set a random value in the form and in a cookie that only lasts for one request (the POST). If the value in the form doesn't match the value in the cookie, then you know the form didn't POST from a real browser.
-
Since you want to use Paypal, you should look at their IPN API. IPN stands for Instant Payment Notification. The notification can be sent to your website, where you intercept it and use the data in it to update your database. The data in the IPN should contain the email address of the site visitor, so you would use that as their user name to login, and you would send them an email with the password. Since I trust that site security is not extra critical, giving the site visitor the password through email is probably acceptable. Now that the user can log in, they would have access to the logged in area, until their access expires, which is something you would put in the database when you first get the IPN. So, you're going to get a little experience with the IPN API, whatever database you choose to use, and whatever Auth library you choose to use. If you use a PHP framework that already has an authentication library, then the rest is probably pretty easy.
-
I think it's important to consider what the modern PHP framework community is doing when it comes to routing and showing the right controller/method or anonymous function. Normally the front controller calls a bootstrap, which is a file or class that does the minimum to set up the application, route the request, and return output that is specific to the request. The request might even return a 404 page not found error.
This would be a good read for you:
http://phpmaster.com/autoloading-and-the-psr-0-standard/
Consider this is my front controller:
<?php // No cache headers header('Expires: Wed, 13 Dec 1972 18:37:00 GMT'); header('Cache-Control: no-store, no-cache, must-revalidate, post-check=0, pre-check=0'); header('Pragma: no-cache'); require_once 'vendor/autoload.php'; // PATH TO FRONT CONTROLLER (this file) define( 'FCPATH', __DIR__ . '/' ); // PATH TO HEART PACKAGE define( 'HPPATH', __DIR__ . '/vendor/heart/' ); // PATH TO APP define( 'APPPATH', __DIR__ . '/app/' ); require_once 'vendor/heart/config/core.php'; // Start an output buffer, because PHP may not be set up with one. ob_start(); $app = new \Heart\Lib\Container( $cfg ); // Show memory usage for request on local machine if( $app['config']->item('mode') == 'local' ) { \FB::log( round( memory_get_usage() / 1024 / 1024, 2 ) . 'MB memory allocated to PHP' ); } // Flush any/all output buffers while( @ob_end_flush() ); /* End of file /index.php */
So you can see that the routing is not actually done here. This front controller just loads my Container class (which is extending Pimple), and once all DI objects are set THEN routing is done. The router itself is a DI object. This is my own private framework, but if you look at the Silex framework you might see what I'm talking about, since it also uses Pimple and is slightly similar. If your routing needs are super basic, and you pretty much want to rely on your own code for everything else, you might try using Slim . For any of this your are going to need to get Composer up and running on your machine, but that's not that hard to do. The upside to using modern PHP frameworks that use Composer is that you can pull in a lot of existing packages and use them, and you don't have to spend time creating functionality that is complex and could take you many hours to achieve. I know all this might sound like a bit much, but going this route will give you the skills to do what you want to do fast, painless, and in a way that is maintainable by other devs. I'm not a big fan of Laravel, but I think it's something you should look at too, since it does use composer and has it's own built in routing, core classes, helpers, etc etc.
-
If you ever look at the source of an email that comes to you with an attachment, you will see that there is a standard way of including the file between some delimiters, and the file is (I believe) base64 encoded. You won't be able to use the script you have without changing it substantially, and like jazzman1 said, you should just use Swift Mailer. There is a lot to consider when sending mail, and it could take you many hours to come up with a script that is half as good as Swift Mailer.
-
Authentication is not for beginners. You will get hacked in 2 seconds. The code you have shown above demonstrates one of the easiest things to hack.
You need to read up on sql injection and proper password hashing before writing more code. You also need to read up on input validation and all of the common attack vectors.
The better alternative, unless you just want to learn more about PHP, is to use somebody else's authentication library.
-
I think to understand use of views, you should look at some of the basic frameworks, and how they use views:
CodeIgniter:
http://ellislab.com/codeigniter/user-guide/general/views.html
Laravel:
http://laravel.com/docs/responses#views
Kohana:
-
You'll need to store the failed login attempts in your database, then check the database to see if a site visitor has too many.
1) When the login page is presented, check the IP address to see if you should even show the form.
2) When the form is submitted, check if IP or username or email address is blocked for too many login attempts.
3) When login page is presented or form submitted, delete stale login attempts (this should actually be done first)
-
Never understood why people feel like arrays are easier than SimpleXML...
I work with a company that uses an XML API that has some pretty massive XML data requests/responses. I know all things SimpleXML, and many scores of hours of experience with it. I've parsed this XML in various ways, and experimented with generating the XML in various ways. It doesn't really matter when you're working with some small amount of XML data, but when you've got a few hundred nodes to parse (or generate), using SimpleXML is more time consuming than just converting the whole thing to an array (or automagically creating the XML from an array). It really just boils down to less typing. I'm not saying SimpleXML is hard, but less typing is easier, especially when dealing with big XML. So yes, in real life using an array may be easier. When your fingers hurt and have numbness from your countless hours of typing, you may agree with me.
-
There's only one difference between a template and a view, and that would be that a template is usually the parent of some nested views. A template is just another view though.
It sounds like you need a clear understanding of MVC, and devs have opinions on how MVC is to implemented, so if I describe MVC to you then keep in mind that this is how I understand it.
MVC =
----------
Request is handed to a controller.
Controller decides what to do with request.
Controller asks for data from Model.
Model may process data, including data validation.
Model may set view variables, or hand data back to controller which injects them into views.
Views use data to build HTML
HTML is output to the browser.
-
A view can be a template, but can also be a snippet of HTML where there is some or no PHP. The view is usually called with an include function, but after output buffering is started, and then output when desired by echoing the output buffer or just using ob_end_flush.
What all this does is allow you to set variables that are used in the view, but at the same time keep the view (which tends to be mostly HTML) out of the controller or model.
Views can be nested inside other views, and if you are doing this then the nested view is usually saved as a variable instead of being output. That variable is then inserted into the parent view, which is then output to the browser.
Some templating systems make use of special delimiters that designate a variable. For instance, instead of using php in a view to insert a variable named $something, some templating systems would allow you to write {{ something }}. I like my framework to be as simple as possible, so I don't use a templating system.
Hope this helps.
-
I've only used SimpleXML, and wonder why you are using xpath? If you just need a quick and easy way to get that value, why not consider this:
<?php $xml = simplexml_load_string( $xml ); $json = json_encode( $xml ); $arr = json_decode( $json, TRUE );
This will give you a simple array to work with, instead of XML which tends to take more effort to work with.
-
I think a problem with the CSV would be that if the file gets big then read/write times are going to be really long. You'd never have this problem with a MySQL insert. Personally I'd just use Google analytics or statcounter. You wouldn't be able to collect IP with Google analytics, because it's against their TOS, but statcounter shows it by default (unless they've changed it recently).
-
I was just wondering what people think, and what are your reasons why redirecting to a login page, or showing the login page instead of authenticated content is the right way.
To be clear, lets say that a site visitor requests a page that requires authentication, and that site visitor is not logged in.
1) Should they be redirected to a login page?
2) Should the login page magically appear without redirect, replacing the content that would have been showed if they were logged in?
Does it really matter which way login is handled? I have not been using redirects, and not experienced any problems with showing the login page instead of the authenticated content. It's actually very convenient to do login this way (at least for me). Are there any issues to be concerned with?
-
Look at the docs and read the part about views. You write all of your own views, which is the HTML output to the browser. Part of those views would include links to CSS, scripts, etc. So, you're not going to find anything pre-made. The whole point in using a PHP framework is to make custom apps, not just make a cookie-cutter website like Wordpress.
-
first check if a form has been submitted using $_SERVER['REQUEST_METHOD'] == "POST". then both the $_FILES and $_POST array will be empty for that condition or you can check $_SERVER['CONTENT_LENGTH'] to get the same information that is output in that warning message.
How about something like this:
if( isset( $_SERVER["CONTENT_LENGTH"] ) && $_SERVER['REQUEST_METHOD'] == "POST" ) { if( $_SERVER["CONTENT_LENGTH"] > ( (int) get_cfg_var('post_max_size') * 1024 * 1024 ) ) { $this->error_stack[] = 'File size too large to upload.'; } }
I'm using get_cfg_var because it works on all of the servers I tested, while ini_get didn't work on Litespeed.
-
I'm working on a file uploading script. It checks the file size, and won't let an upload complete if over a size specified in config. This all works, but if a file is uploaded that goes over PHP's limit, then I get the familiar warning:
Warning: POST Content-Length of 14622352 bytes exceeds the limit of 8388608 bytes in Unknown on line 0
I am not looking to allow this file to go through. I don't want to increase memory or the max post size. That's not the issue.
The upload script already has a way for the user to see if their file had problems uploading (showing errors), but how can I catch the error shown above? I know the error won't display if the error reporting and display of errors is turned off, but I don't want to leave the user in the dark. They should get some feedback. What can I do here?
-
Thanks requinix. Your proposed code is way better.
-
I'm combing through somebody else's php script (an upload class) and I'm trying to figure out exactly what a preg_replace function is trying to find and replace:
$this->upload_path = preg_replace("/(.+?)\/*$/", "\\1/", $this->upload_path);
I've tried going to some of the online regex testing sites and testing paths, but not able to get a match with anything I've tried. To me it looks like it "any path should have one final slash, but not more than one, and not less than one". Am I close?
-
Unless you are specifically querying for a country, city, or venue, then I assume you are pulling in the entire set from the DB. Because of that, you can specify you want the results as an array:
$arr = $query->result_array();
Now that all of the rows are in an array, you would simply use php's sorting functions to order the array. I'd recommend looking at uasort. Also, with this type of nested parent => child relationship, you can take a look at what I did with Community Auth, and the example that shows a category menu. Community Auth is at http://community-auth.com, and is a CodeIgniter project. Look at how the database is organized, and how the results are displayed. I think you will find that it will work well for your project.
-
Just looking at some examples online, it looks like you may have to set up all of your curl options for each request. I may be wrong, or what I was looking at might not be right, but you might try that.
-
You're going to need to do a second curl post to the page with the security question.
-
Codeigniter has less learning curve and has more support in online compared to other frameworks. Codeigniter has large community support and resources. It is one of the mostly used MVC frameworks.
Due to my longtime use of CodeIgniter I'd say the argument that is has large community support and resources is actually no longer something that should be said. Over time most of the serious devs have moved on, and "the community" aka the people who still use the forum seem to be mostly noobs that speak English through Google translate. The resouces that used to be in the wiki were at one point moved to github and most were not updated so most of the downloads disappeared. My biggest problem with CodeIgniter isn't so much that it's technically inferior, but that development is dead and if they ever do officially release v3 the license makes it unusable for me and the type of clients I want to do business with. Go with Laravel, Symphony, Yii, Cake, Slim, or anything else. Spending time with CodeIgniter is now just a waste of your time, and time is money....
-
I like it. It's simple, clean, and while to super fancy it looks like it would be nice for that type of business.
-
Oh... and speaking of which. Every developer should read at least the first chapter of this: https://leanpub.com/laravel-testing-decodedThe first chapter nails why we should all be "testing all the things". For that alone, it's worth the $15. Looking forward to reading the rest.
I could probably benefit from testing, but every time I read about it it seems like a total waste of time. I've been using PHP for 7 years, and never needed it before, so what I need is an explanation, or a real life situation where testing would be beneficial. I'll try reading this book and hope it clears things up for me.
Rewrite Code of PHP page not found
in PHP Coding Help
Posted
Where are your mod_rewrite rules? It's kind of hard to figure out what you're doing without more info.