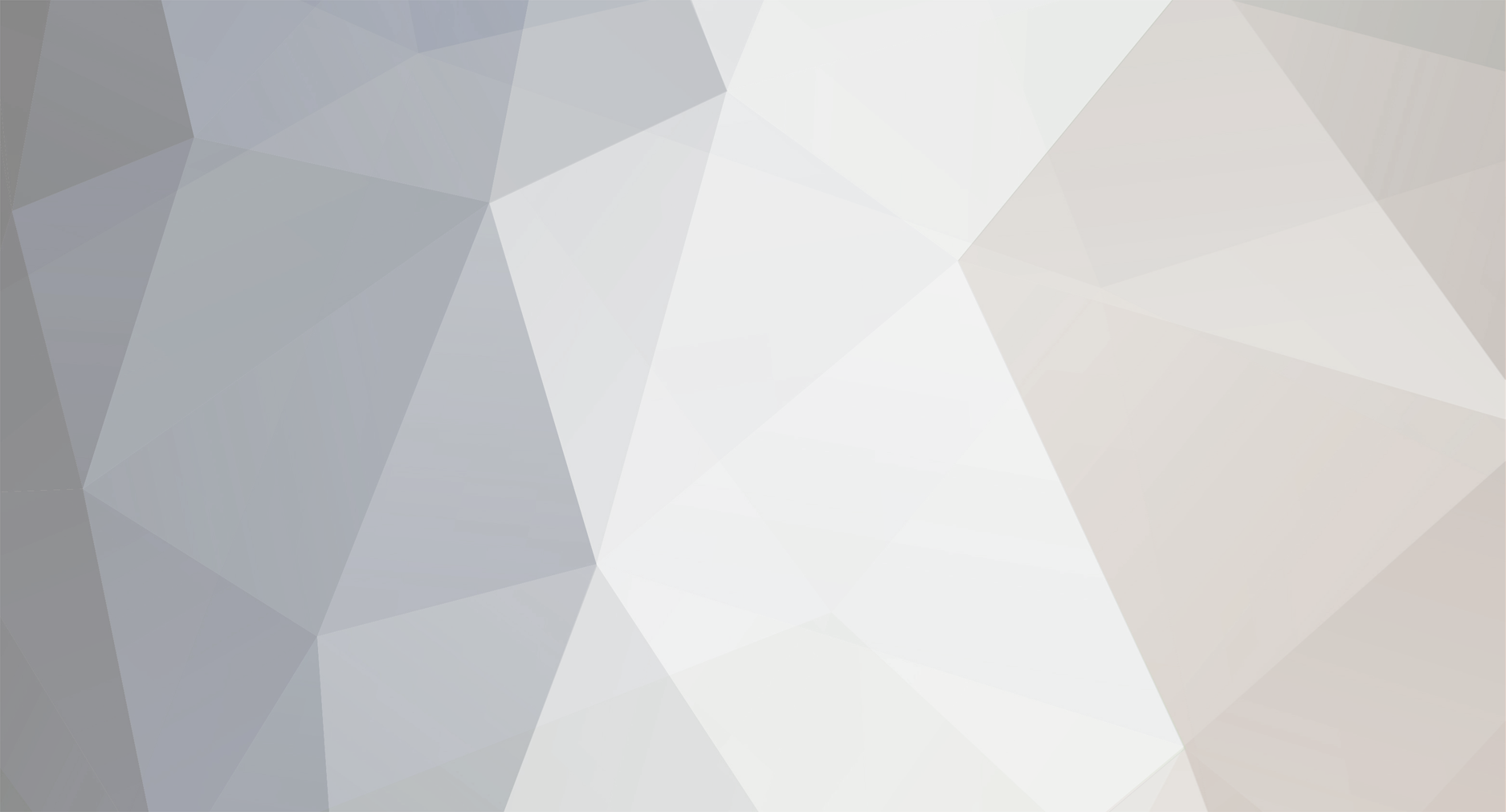
djfox
-
Posts
327 -
Joined
-
Last visited
Never
Posts posted by djfox
-
-
Just in case anyone else comes across this:
I find that putting plain
<td valign=center align=right>
does not work. I had to put
<td valign=center width=30% align=right>
to get it to work right.
-
Huh, before when I tried that it wouldn`t budge. Now it`s working. Perhaps I just misspelled it last time...
Thanks for the help.
-
I want a table column
<td valign=top>
to be aligned not only to the top but to the right as well. How do I do this?
-
How about
$checkhour = date("H");
That got it working. Thanks a bunch, buddy. ^^
-
The Topic Solved button got lost when they did the update.
Oh dear.
Have you tried echoing $checkhour to see what it displays when you pick 08?
It displays nothing.
-
I`ve tried this code:
<?php if ($checkhour == 00 || $checkhour == 12) { $dayname = "Evening"; $daynum = "2"; } elseif ($checkhour == 01 || $checkhour == 02 || $checkhour == 03 || $checkhour == 04 || $checkhour == 05 || $checkhour == 13 || $checkhour == 14 || $checkhour == 15 || $checkhour == 16 || $checkhour == 17) { $dayname = "Night"; $daynum = "3"; } elseif ($checkhour == 06 || $checkhour == 18) { $dayname = "Morning"; $daynum = "4"; } elseif ($checkhour == 07 || $checkhour == 08 || $checkhour == 09 || $checkhour == 10 || $checkhour == 11 || $checkhour == 19 || $checkhour == 20 || $checkhour == 21 || $checkhour == 22 || $checkhour == 23) { $dayname = "Day"; $daynum = "1"; } ?>
But it still will not work for hour 08.
-
Ok, first, here is the code:
<?php $checkhour = strftime("%H", time()); if ($checkhour == 00) { $dayname = "Evening"; $daynum = "2"; } elseif ($checkhour == 01) { $dayname = "Night"; $daynum = "3"; } elseif ($checkhour == 02) { $dayname = "Night"; $daynum = "3"; } elseif ($checkhour == 03) { $dayname = "Night"; $daynum = "3"; } elseif ($checkhour == 04) { $dayname = "Night"; $daynum = "3"; } elseif ($checkhour == 05) { $dayname = "Night"; $daynum = "3"; } elseif ($checkhour == 06) { $dayname = "Morning"; $daynum = "4"; } elseif ($checkhour == 07) { $dayname = "Day"; $daynum = "1"; } elseif ($checkhour == 08) { $dayname = "Day"; $daynum = "1"; } elseif ($checkhour == 09) { $dayname = "Day"; $daynum = "1"; } elseif ($checkhour == 10) { $dayname = "Day"; $daynum = "1"; } elseif ($checkhour == 11) { $dayname = "Day"; $daynum = "1"; } elseif ($checkhour == 12) { $dayname = "Evening"; $daynum = "2"; } elseif ($checkhour == 13) { $dayname = "Night"; $daynum = "3"; } elseif ($checkhour == 14) { $dayname = "Night"; $daynum = "3"; } elseif ($checkhour == 15) { $dayname = "Night"; $daynum = "3"; } elseif ($checkhour == 16) { $dayname = "Night"; $daynum = "3"; } elseif ($checkhour == 17) { $dayname = "Night"; $daynum = "3"; } elseif ($checkhour == 18) { $dayname = "Morning"; $daynum = "4"; } elseif ($checkhour == 19) { $dayname = "Day"; $daynum = "1"; } elseif ($checkhour == 20) { $dayname = "Day"; $daynum = "1"; } elseif ($checkhour == 21) { $dayname = "Day"; $daynum = "1"; } elseif ($checkhour == 22) { $dayname = "Day"; $daynum = "1"; } elseif ($checkhour == 23) { $dayname = "Day"; $daynum = "1"; } ?>
Here is what the code should do:
Figure out what hour the time is and declare if the time is day/night/morning/evening depending on what the hour is.
What the problem is:
It seems to have a problem with hour 08. All the other hour times work but that one. And by work I mean it will echo $dayname. With hour 08, it echos nothing.
EDIT:
Anyone know what happened to the "Topic Solved" tab? Past few posts of mine I cannot find that to mark my topics solved.
-
Thanks a bunch!
(For some reason the forum won`t let me mark this topic solved.)
-
I have a thumbnail creator that someone else had developed for me. It works for jpg images but my site also has png, gif bmps and probably more in the future. But the thumbnail creator can`t do thumbnails for these non-jpg images. Here`s what the thumbnail creator is:
<?php // File and new size //$imgfile = 'thumb.jpg'; //$percent = 0.2; header('Content-type: image/jpeg'); $imgfile = $_GET['img']; list($width, $height) = getimagesize($imgfile); //echo $width, $height; $newwidth = 100; $factor = $newwidth / $width; //echo $factor; $newheight = $height * $factor; $thumb = ImageCreateTrueColor($newwidth,$newheight); $source = imagecreatefromjpeg($imgfile); imagecopyresized($thumb, $source, 0, 0, 0, 0, $newwidth, $newheight, $width, $height); imagejpeg($thumb); ?>
Any advice?
-
why don't you use SESSION to store all the information like scores so people cant cheat at all
Scott.
Because the form url could be changed to cheat the code.
One thing that you can do is use the POST method instead of GET. Then the information isn't passed along via the URL.
Thanks, I`ll give that a shot.
-
I`m building a mini-game using php where there is a score involved. It will use forms to send the information along. Thus far, forms I`ve seen (and used) show the url as things like game.php?id=8&s=1000
Anything I can do to keep people from being able to just type in things in the url bar to cheat the code?
-
Oh, all I needed was that slash? Ok, thanks a bunch!
-
Their coding did not work.
You mean you didn't read it, tried to copy and paste, and nothing worked.
I did read it.
-
It isn`t picking it up it seems, did I do it correctly?
<?php if ($_SERVER['PHP_SELF'] == explore.php){ $whereloc = "Exploring"; } ?>
-
I tried using php.net to look this up myself but it keeps saying it has an internal error.
So!
What I would like to do is have the script check what the current url is, like if the page is currently explore.php or today.php
-
Well you can definitely google for such scripts but my cousin has been making really cool stuff with AJAX and he's online a lot of the time so if you want to ask him to point you in the right direction, just mail him: www.boedesign.com
All righty, I`ll see what he says.
-
Their coding did not work.
-
I hope this is the right forum for this.
I currently have some functioning javascript games on my site. What I would like for them to do is if the person playing them is logged in, they earn credits when they complete something in the game (basically updating the database for accomplishing a certain task in the game). Is this possible?
-
You can use the code The Little Guy posted, which is based on thorpe's idea.
I'm unsure how you want to do this. Do you want to get 1 person's data or all?
Something like this?
<?php $res3 = mysql_query("SELECT bought FROM userdata"); if ($res || (mysql_num_rows($res3) > 0)){ echo array_count_values(explode(",",$res['bought'])); } ?>
Yes, I wanted to count up all. I basically just wanted to see how many copies of an item was floating around.
I posted your code in but that seems to have made no effect.
-
What are the columns in your database? Does it contain the user's name or anything that you can use to get the particular user's inventory?
I`ll just post the code:
<?php if(!$offset) $offset=0; $recent = 0; $res = mysql_query("SELECT id,name FROM clothing ORDER BY name ASC")or die( mysql_error() ); while( $c = mysql_fetch_row($res) ){ if( $recent >= $offset && $recent < ($offset + 200000000 )){ $res2 = mysql_query("SELECT COUNT(*) FROM clot_sell WHERE item='$c[0]'"); $count1 = mysql_fetch_row($res2); mysql_free_result($res2); $res3 = mysql_query("SELECT COUNT(DISTINCT bought) FROM userdata WHERE bought='$c[0],'"); $count2 = mysql_fetch_row($res3); mysql_free_result($res3); $total = $count1[0] + $count2[0] ; echo "$c[1] -> <b>$total</b><br>"; } } $res4 = mysql_query("SELECT COUNT(*) FROM clot_sell"); $count3 = mysql_fetch_row($res4); mysql_free_result($res4); $res5 = mysql_query("SELECT COUNT(*) FROM clothing"); $count4 = mysql_fetch_row($res5); mysql_free_result($res5); echo "<p>Total for Sale: <b>$count3[0]</b>"; echo "<br>Total of types available: <b>$count4[0]</b><p>"; ?>
-
It`s gone bonkers saying it`s not a valid argument.
Ah never mind it. It was just something for a statistical curiosity anyhow. No big deal. Thanks for trying, guys.
-
You did see this, right?
SELECT LENGTH(`column`) - LENGTH(REPLACE(`column`, ',', '')) AS `count` FROM `the_table` WHERE ...
I must be doing something wrong since nothing shows up:
$resb = mysql_query("SELECT LENGTH(`bought`) - LENGTH(REPLACE(`bought`, ',', '')) AS `count` FROM `userdata` WHERE bought='$c[0]'"); $try = mysql_fetch_row($resb); mysql_free_result($resb);
-
Oh no, I totally know what you guys mean by the other way of setting this up. And I agree totally that it`s easier to work with in the long run. The reason I`m trying to stick with how the guy had it was because the entire doll system he made for me depends on the items listed in bought. I`m not skilled enough to redo it myself nor can I afford to have him (or even another person) redo it.
-
Like thorpe says, it's not efficient at all.
I`ve heard conflicting statements about that, back when I was trying to work out favourites system for my site. I wanted to have each favourite made by it`s own entry in a table and people in another forum (don`t ask me the name, I haven`t been there in a few months) said that was inefficient and that I should add them all into one field in the person`s account information table. But here you guys say the opposite. *headscratch*
Why isn`t this code working?
in PHP Coding Help
Posted
First the background story:
I`ve had this strip of coding working for several months, never touched it. The server host has changed something where all of my codes went into a seizure and no longer work so I`ve had to rebuild my site to get everything to work.
The code that needs looking over:
What the code is supposed to do:
In short, it is a virtual transaction.
The item is removed from the sales table, item is added to buyer`s inventory, the amount of echos (currency) the seller marks the item for should be removed from buyer`s echos amount and put the amount in sales for the buyer to pick up.
What the code is currently doing:
It will remove the item from the sales table, it is entering the item into the buyer`s inventory, the amount is entered into sales for seller to pick up, however the amount is not being correctly removed from the buyer`s echos.
What I have done:
I`ve echo`ed out all the information and the information being transferred through the form for the transaction is correct and all of the info is being picked up by the code. I`ve re-written the line of codes involving the removal of the ehcos amount from the buyer`s amount and have two different results: 1) the buyer`s echos remain the same 2) the buyer`s echos change to the negative amount of what $p is instead of just simple subtraction
References:
The following line of code
is used in another file where echos are being added to the user`s echos amount and this works perfectly fine. I post this because some people have issues with how I write the code for math calculations of the echos and claim it doesn`t work, however I have files where it does work, and this had worked for months.
Additional info:
$logged is defined in design.php which I am not posting because that is not the problem.
I think that`s all the info needed.