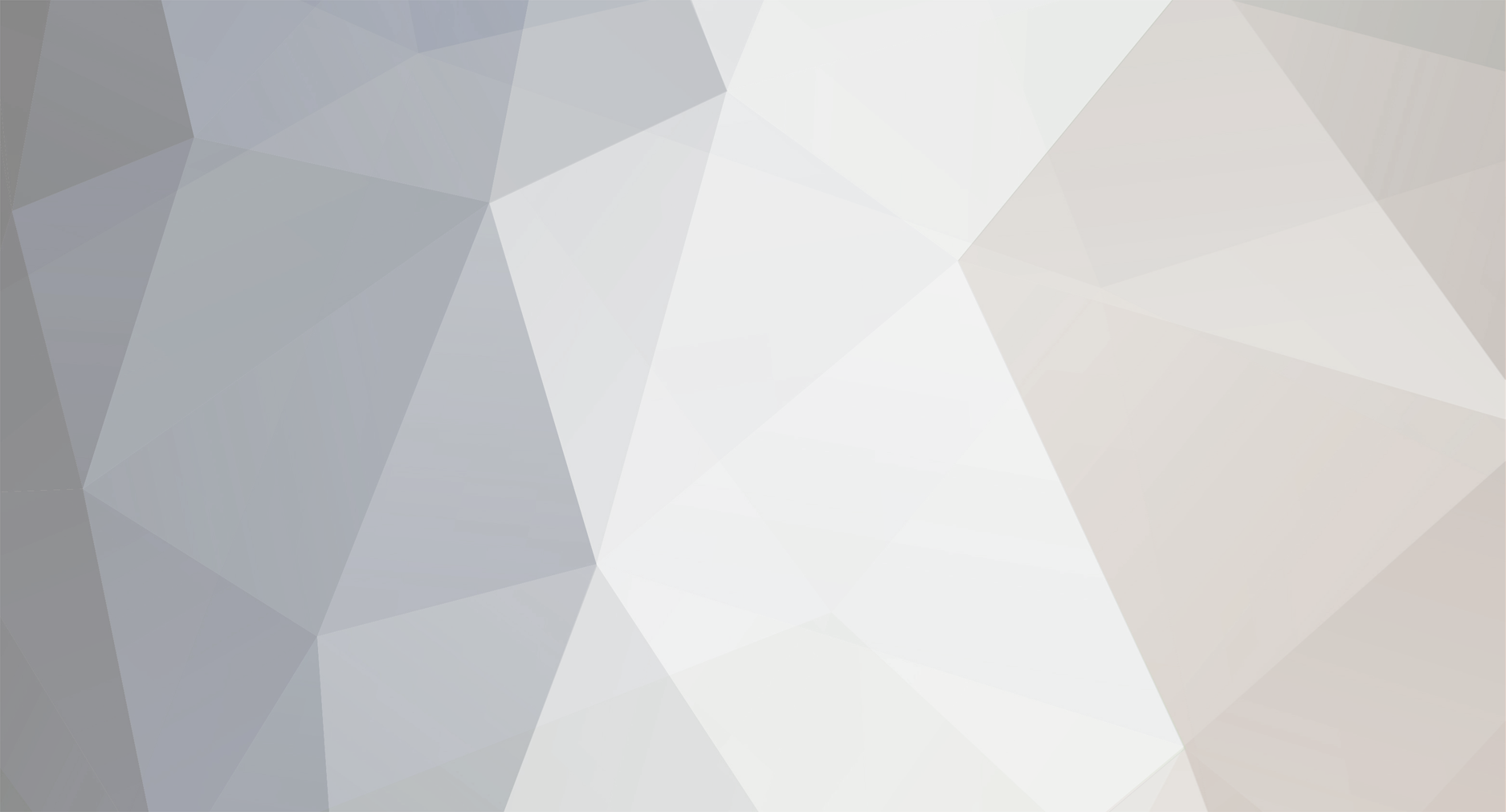
Orio
-
Posts
2,491 -
Joined
-
Last visited
Never
Posts posted by Orio
-
-
Found my problem, I think.
Change this:
unlink($file);
Into:
unlink($dir."/".$file);
Orio.
-
You didn't put that at your top of your script. You can't have output when downloading the file.
Orio.
-
<?php function empty_dir($dir); //$dir should NOT be passed with an ending "/". { $result = true; $h = opendir($dir); if($h === false) return false; while($file = readdir($h)) { if($file == "." || $file == "..") continue; if(is_dir($file)) { if(!empty_dir($dir."/".$file)) $result = false; continue; } unlink($file); //Regular file.. } closedir($dir); return $result; } $dir = "/home/gscl/public_html/stk/temp"; if(!empty_dir($dir)) echo "An error occured. Some of the files weren't deleted."; else echo "Dir empty."; ?>
Haven't tested it, use at your own risk.
Orio.
-
You script should start by checking if there should be a download. If so, update the database records and then force download the file. If a download was not requested, show the form.
<?php if((isset($_POST['do']) && $_POST['do'] == "Download") { //Do the query that adds 1 to mysql //Force download: $filename = "...."; //Your filename.... // required for IE, otherwise Content-disposition is ignored if(ini_get('zlib.output_compression')) ini_set('zlib.output_compression', 'Off'); // addition by Jorg Weske $file_extension = strtolower(substr(strrchr($filename,"."),1)); switch( $file_extension ) { case "pdf": $ctype="application/pdf"; break; case "exe": $ctype="application/octet-stream"; break; case "zip": $ctype="application/zip"; break; case "doc": $ctype="application/msword"; break; case "xls": $ctype="application/vnd.ms-excel"; break; case "ppt": $ctype="application/vnd.ms-powerpoint"; break; case "gif": $ctype="image/gif"; break; case "png": $ctype="image/png"; break; case "jpeg": case "jpg": $ctype="image/jpg"; break; default: $ctype="application/force-download"; } header("Pragma: public"); // required header("Expires: 0"); header("Cache-Control: must-revalidate, post-check=0, pre-check=0"); header("Cache-Control: private",false); // required for certain browsers header("Content-Type: $ctype"); // change, added quotes to allow spaces in filenames, by Rajkumar Singh header("Content-Disposition: attachment; filename=\"".basename($filename)."\";" ); header("Content-Transfer-Encoding: binary"); header("Content-Length: ".filesize($filename)); readfile("$filename"); exit(); } else { //Show the form, the file doesn't need to be downloaded } ?>
Credit for the force download code piece:
http://elouai.com/force-download.php
Orio.
-
Sure, go for it.
Orio.
-
I'd upload it to a folder with an obscure name, htaccess it so only you can access it (user/pass) and then test it there.
I think it's better doing that rather than testing it locally because this way you are testing it with all of your server's settings, so there will be no surprises when you move it for live use.
Orio.
-
You want to display the image, right? For that you simply need to use the <img> tag, with out includes:
echo "<img src=\"{$filename}\">";
Orio.
-
That's possible, but that would be a bit difficult. It's much easier having a form in your site letting your users submit articles. Why via email?
Orio.
-
You can, but that will just print out the image contents, as if you opened it with notepad or such (it won't display it).
What exactly are you trying to achieve?
Orio.
-
-
I think your problem is in your second if() (in registration.php):
if($row|| ...) //Should become if(!$row || ...)
Orio.
-
Color coding without having to add start/end php tags is nice
Certainly is. Good job.
Orio.
-
I also use:
<?php if (hours < 24 && minutes < 60 && seconds < 60) { return true; } else { return false; } ?>
But only when the block contains more than one line.
For an example, I do:
<?php for($i = 0; $i < 7; $i++) if($i % 2 == 0) echo $i."<br>"; //And while($i < 7) { if($i % 2 == 0) echo $i."<br>"; $i++; } ?>
Orio.
-
Try this:
<?php function random_file ($dirname) { if(!is_dir($dirname)) return false; if(!$h = opendir($dirname)) return false; $files = array(); while($file = readdir($h) !== FALSE) if(!is_dir($file)) $files[] = $file; return $files[array_rand($files)]; } $dir = "/frontpage/"; $img = random_file($dir); if($img !== false) echo "<img src=".$img." />" . filetype($dir.$img) . "\n"; ?>
Orio.
-
After the sorts you can just compare the arrays
I wasn't too sure how fast str_split() is, so I thought using str_split() only once and then looping over the characters would be faster.
But I guess that should be tested.
Orio.
-
You want to display a random image from that folder?
<?php $dir = "/frontpage/"; $files = scandir($dir); //Note, PHP5+ $rand = rand() % (count($files) - 2) + 2; echo "<img src=".$files[$rand]." />" . filetype($dir . $file[$rand]) . "\n"; ?>
Orio.
-
<?php function is_scrambled ($scrambled, $unscrambled) { if(strlen($scrambled) != strlen($unscrambled)) return false; $letters = str_split($scrambled); $num_letters = count($letters); sort($letters); for($i = 0; $i < $num_letters; $i++) if($letters[$i] != $unscrambled[$i]) return false; return true; } ?>
Orio.
-
I think this should be enough:
<?php $res = array('M'=>31, 'Y'=>1, 'D'=>365); asort($res); reset($res); echo key($res).": ".current($res)."<br>"; ?>
Orio.
-
I learned from tutorials.... Once I got the basic syntax, I just kept googling and checking php.net references....
Then again, some aspects of my knowledge I hate. For example, I hate that I suck at OOP (getting better though!), and I don't know many code designs.... Had I read a book or something, I probably would've been atleast introduced to that....
Pretty much the same..
I new basic C when I started learning PHP. But all my learning was (and is) from the web, a lot of trial and error.
But as I am not a professional PHP coder, there are a lot of things I still don't know. OOP as an example.
Always learning more though..
Orio.
-
Do you want to know if this variable contains that certain substring? Do you want to know where? Do you want the text around it?
I assume you only need to check whether $email_comments contains "processed successfully". This is done (in the most simple way) like that:
<?php $email_comments = "...text...processed successfully...text..."; if(strpos($email_cmments, "processed successfully") !== FALSE) echo "It was processed successfully"; else echo "It wasn't processed successfully"; ?>
Orio.
-
It''s a variable. From the given piece of code I can't tell you much more..
Orio.
-
Or simply...
<?php $browser = get_browser(null, true); echo "You are using {$browser['browser']} version {$browser['version']}"; ?>
Orio.
-
Your code doesn't work because this is what PHP understands from you:
$extra = 40000; $range = $extra-(250000+$extra); // = 40000-(250000+40000) = 250000
PHP thinks, as it should, that the expression you stated above is a mathematical expression. 250000 is passed as the third parameter. You need to clarify you are dealing with strings here.
This should solve your problem:
$extra = 40000; curl_setopt($conn[$i],CURLOPT_RANGE, $extra."-".(250000+$extra));
Orio.
-
Extract a long group of strings
in Regex Help
Posted
This should do.
Orio.