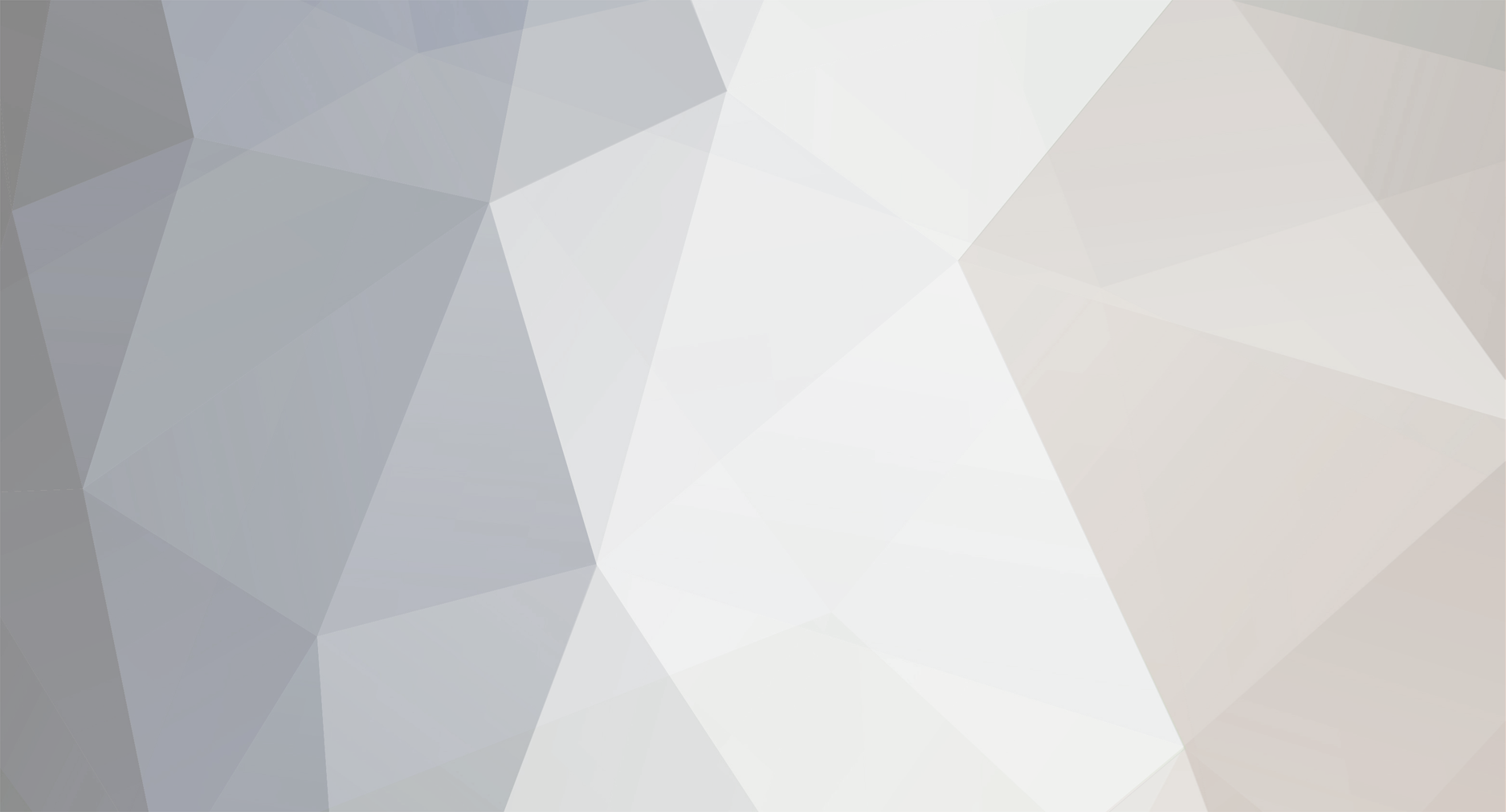
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
OK, gotcha. But, I would think the better solution is to fix $total_pages. Since we want the current page to default to 1, even when there are no records, then $total_pages should always be at least 1. I would have done this $total_pages = max(ceil($total_records / $rec_per_page), 1);
-
Maybe I'm just not seeing it, but I don't think the order of those two would matter. One would only do something if $current_page was less than 1 and the other would only do something if $current_page was greater than $total_pages. Assuming $total_pages is 1 or greater, only one of those conditions could ever be true - not both. So, the order of the two would not matter.
-
Hmm . . . "5px"? I think you mean "5pt"
-
Here is a rewrite of your script for scenario #1. I made a lot of other improvements int he code as well. <?php //Configurable variables $rec_per_page = 50; //Should be an even multiple of $rec_per_page $cols_per_page = 2; $page_link_range = 3; //Connect to database include"scripts/connect.php" ; mysql_connect('localhost',$username,$password); @mysql_select_db($database) or trigger_error("SQL", E_USER_ERROR); //Run query to get total records $query = "SELECT COUNT(*) FROM movie WHERE type = 'movie'"; $result = mysql_query($query) or trigger_error("SQL", E_USER_ERROR); $total_records = mysql_result($result, 0); $total_pages = ceil($total_records / $rec_per_page); //Set current page $current_page = (isset($_GET['currentpage'])) ? (int) $_GET['currentpage'] : 1; if ($current_page < 1) { $current_page = 1; } if ($current_page > $totalpages) { $current_page = $totalpages; } //Run query to get records for current page $offset = ($current_page - 1) * $rec_per_page; $query = "SELECT title FROM movie WHERE type = 'movie' ORDER BY title LIMIT $offset, $rec_per_page"; $result = mysql_query($query) or trigger_error("SQL", E_USER_ERROR); //Loop through results to create output $movie_list = ''; $rec_idx= 0; while ($row = mysql_fetch_assoc($result)) { //Open new row if needed if($rec_idx% $cols_per_page == 0) { $movie_list .= "<tr>\n"; } $movie_url = urlencode($row['title']); $movie_text = htmlspecialchars($row['title']); $movie_list .= "<td> <a class='nav' href=/rules.php?title={$movie_url}>{$movie_text}</a></td>\n"; //Close current row if needed if($rec_idx% $cols_per_page == $cols_per_page-1) { $movie_list .= "</tr>\n"; } $rec_idx++; } //Close out last row if needed if($rec_idx% $cols_per_page != $cols_per_page-1) { $movie_list .= "</tr>\n"; } //Create pagination links $page_links = ''; //First and Prev pages if ($current_page > 1) { $prevpage = $current_page - 1; $page_links .= " <a class='nav' href='movies.php?currentpage=1'><<</a> \n"; $page_links .= " <a class='nav' href='movies.php?currentpage={$prevpage}'><</a> \n"; } //Surrounding pages $beg_page = max($current_page - $page_link_range, 1); $end_page = min($current_page + $range, $totalpages); for ($page = $beg_page; $page <= $end_page; $page++) { if($page == $current_page) { $page_links .= " [<b>{$page}</b>] \n"; } else { $page_links .= " <a class='nav' href='movies.php?currentpage={$page}'>{$page}</a> \n"; } } //Next and last pages if ($current_page != $totalpages) { $nextpage = $current_page + 1; $page_links .= " <a class='nav' href='movies.php?currentpage={$nextpage}'>></a> \n"; $page_links .= " <a class='nav' href='movies.php?currentpage={$totalpages}'>>></a> \n"; } ?> <table align="center"> <th class="rulemain" colspan="<?php echo $cols_per_page; ?>">Movies</th> <?php echo $movie_list; ?> <tr><td colspan="<?php echo $cols_per_page; ?>"><?php echo $page_links; ?></td></tr> </table> Or, replace the relevant section with this should give you scenario #2 //Loop through results to create output $row_content = array(); $rec_idx = 0; $rows_per_page = $rec_per_page / $cols_per_page; while ($row = mysql_fetch_assoc($result)) { $row_idx = $rows_per_page % $rec_idx; $movie_url = urlencode($row['title']); $movie_text = htmlspecialchars($row['title']); $row_content[$row_idx][] = "<td><a class='nav' href=/rules.php?title={$movie_url}>{$movie_text}</a></td>\n"; } $movie_list = ''; foreach($row_content as $row) { $movie_list .= "<tr>\n"; $movie_list .= "<tr>" . implode("</tr>\n<tr>", $row) . "</tr>"; $movie_list .= "</tr>\n"; } I did not test any of this so there may be some minor bugs
-
I screwed up scenario #1, it should have looked like this Scenario #1 1 2 3 4 5 6 etc.
-
But, how do you want them listed in those two columns? Scenario #1 1 2 2 4 3 6 etc. Scenario #2 1 25 3 26 5 27 etc. Scenario #1 is the easiest, but #2 is not too difficult
-
How to customize mysql error messages? (get key with duplicate entry)
Psycho replied to DavidT's topic in PHP Coding Help
I don't think there is any way to determine which unique fields were possibly duplicated based on the error. I would just attempt the insert and trap the error if it occurs. Then if there is an error run a select query to get current records matching any of the unique values and loop through to determine which ones are duplicated. -
define directories for images, css and js.
Psycho replied to yahelarmster's topic in PHP Coding Help
A constant is pretty much just like variable - just without the dollar sign. Just echo it out. But in your example you didn't provide the file name. <link rel="stylesheet" href="<?php echo DIR_STYLES . 'name_of_css_file.css'; ?>" type="text/css" /> or <link rel="stylesheet" href="<?php echo DIR_STYLES; ?>name_of_css_file.css" type="text/css" /> -
I did say " . . . might be overkill". But, I put mine into a function which is good practice. And you added a function_exists() check which added even more overhead. When I reduced both methods down to the bare minimum my tests found the substr() solution to be faster. These were the lines I ran for the tests which are as apples to apples as I could get $value = preg_replace('~([0-9]{3})([0-9]{3})([0-9]{4})~', '($1)-$2-$3', $number); $value = substr($number, 0, 3) . '-' . substr($number, 3, 3) . '-' . substr($number, 6); Running each line 10,000 times with three runs each I got the following times: First method: Test 1: 0.025115966796875 Test 2: 0.025408029556274 Test 3: 0.025764942169189 Second method: Test 1: 0.022403955459595 Test 2: 0.022188186645508 Test 3: 0.022217035293579 Pwnd by .003 seconds!
-
@jcbones, since the value is only numbers then there is no need to the capture expressions to only be numbers. You could just as easily use $pattern = '~(.{3})(.{3})(.{4})~'; However, in this case RegEx might be overkill since you can just use substr(). It might look like it's more complicated, but it's probably more efficient. Here's my two cents function formatPhone($p) { $phone = substr($p, 0, 3) . ' ' . substr($p, 3, 3) . ' ' . substr($p, 6); return $phone }
-
You could use jcbones's solution of creating the two or, just create a ternary operator int he code to switch from red to green in the loop. I would do this: <?php function format_time($t) { $minutes = floor($t / 60000); $seconds = sprintf('%02d',floor(($t / 1000) % 60)); $ms = sprintf('%03d', $t % 1000); return $minutes . ":" . $seconds . "." . $ms; } $highscores = file_get_contents('http://highscores.adrenalinex.co.uk/tourney4.php?c=1&r=2'); $lines = explode("\n", $highscores); //Get index of where red records should start $red_idx = count($lines) - 8; $table_data = ''; foreach ($lines as $idx => $line) { list($name, $time) = sscanf($line, "%s %d"); $no = $idx + 1; $f_time = format_time($time); if($no == 1) { $first_time = $time; $int_to_first = '-'; $int_to_prev = '-'; } else { $int_to_first = format_time($time - $first_time); $int_to_prev = format_time($time - $last_time); } $class = ($idx<$red_idx) ? 'green' : 'red'; $table_data .= "<tr class='$class'><td>$no</td><td>$name</td><td>$f_time</td><td>$int_to_first</td><td>$int_to_prev</td></tr>\n"; $last_time = $time; } ?> <html> <head> <style> .results_table { text-align: center; font-weight: bold; border: 1px solid #CCC; } th { background: url('wp-content/themes/arras/images/feed-title-white.jpg'); font-weight: bold; color: red; } .green td { color: green; } .red td { color: red; } </style> </head> <body> <table class="results_table" border="1" cellpadding="4" align="center"> <tr> <th style="width: 40px;"></th> <th style="width: 200px;">Drivers Name</th> <th style="width: 100px;">Time</th> <th style="width: 100px;">Interval to 1st</th> <th style="width: 100px;">Interval to driver ahead</th> </tr> <?php echo $table_data; ?> </table> </body> </html>
-
What you have really doesn't make sense. The "replace" checkbox is only deleting the existing image of the same name. The move_uploaded_file() would do that anyway. So, if someone uploads an image with the same name, but does not check the replace checkbox, the image will still get replaced. But, even if the user does check that checkbox you are still going to end up with a duplicate entry in the database. So, the checkbox serves no purpose. Based upon what you have done it seems you are only wanting to update the image IF it has the same name. So, a better solution would be this: Don't use a "replace" checkbox. Instead, just check if the image already exists to determine whether you should insert a new record or not when you get tot he Query part. Also, there are other problems. For example, you are using mysql_real_escape_string() on the values to use in the file operations. That could cause errors. Use mysql_real_escape_string() for string data WHEN it is being used in the query. You should validate data for file operations differently.
-
Your code is a little difficult to follow. You can just strip off the last 8 lines the loop using array_slice(). There are also more efficient ways to handle the different handling of the first and subsequent records. <?php function format_time($t) { $minutes = floor($t / 60000); $seconds = sprintf('%02d',floor(($t / 1000) % 60)); $ms = sprintf('%03d', $t % 1000); return $minutes . ":" . $seconds . "." . $ms; } $highscores = file_get_contents('http://highscores.adrenalinex.co.uk/tourney4.php?c=1&r=2'); $lines = explode("\n", $highscores); //Strip off the last 8 records $lines = array_slice($lines, 0, count($lines)-9); $table_data = ''; foreach ($lines as $idx => $line) { list($name, $time) = sscanf($line, "%s %d"); $no = $idx + 1; $f_time = format_time($time); if($no == 1) { $first_time = $time; $int_to_first = '-'; $int_to_prev = '-'; } else { $int_to_first = format_time($time - $first_time); $int_to_prev = format_time($time - $last_time); } $table_data .= "<tr><td>$no</td><td>$name</td><td>$f_time</td><td>$int_to_first</td><td>$int_to_prev</td></tr>\n"; $last_time = $time; } ?> <html> <head> <style> .results_table { text-align: center; font-weight: bold; border: 1px solid #CCC; } th { background: url('wp-content/themes/arras/images/feed-title-white.jpg'); font-weight: bold; color: red; } </style> </head> <body> <table class="results_table" border="1" cellpadding="4" align="center"> <tr> <th style="width: 40px;"></th> <th style="width: 200px;">Drivers Name</th> <th style="width: 100px;">Time</th> <th style="width: 100px;">Interval to 1st</th> <th style="width: 100px;">Interval to driver ahead</th> </tr> <?php echo $table_data; ?> </table> </body> </html>
-
Well, it depends . . . Are you wanting the user to upload another file of the same name to overwrite the original? If so, move_uploaded_file() will do that automatically. But, the problem comes with the query whether or not the user has uploaded a new file with the same or different name. I can't discern any unique field from your query. Typically I would expect to see a user_id or something that would associate the record in some way to be unique. If you DO have a unique field in that query AND it is set as unique in that table then you can use the ON DUPLICATE KEY UPDATE clause in your query. INSERT INTO table (a,b,c) VALUES (1,2,3),(4,5,6) ON DUPLICATE KEY UPDATE c=VALUES(a)+VALUES(b); So, if the record being added would cause a conflict on a unique column the existing record would be updated. But, what about the original image (if the new one has a different name)? You would likely want to delete that image. That is why you usually see images renamed according to the unique key (e.g. user images may be named per the user ID). So, I can't really provide a meaningful solution because there are too many unanswered questions. You can either take the suggestions given and build your solution or provide more details.
-
This topic has been moved to JavaScript Help. http://forums.phpfreaks.com/index.php?topic=362083.0
-
What you are asking is really a JavaScript problem. You may need PHP to help populate the listbox(es), but the framework to make them work will require JavaScript. So, until you have a JavaScript solution there's nothing that PHP can do with this. Moving to the JavaScript forum. FYI: You might want to look into JQuery - it might already have a pre-built solution.
-
This isn't particularly constructive criticism. Telling him how he could do this would be helpful. I'd be amazed to find a programmer who didn't go through some confusion as to how to lay out code when starting out, and if we had been told to 'do better' at that time, it wouldn't have really helped at all. Well, your response wasn't particularly helpful to this problem at all. And, I believe it is constructive criticism. New programmers try to do too much without really thinking through what they are doing. Taking a few moments to jot down the logic of what needs to be built and doing one thing at a time would be much more productive. As to the OP, the errors he is getting are exceptionally informative compared to many PHP errors and I provided an explanation of why he was getting the errors he received. In fact, int he last post he completely changed the subject of the post to which I responded if the original question was resolved he should open a new thread. He then opens this thread with the exact same problem he opened the first one for. And, when a response is given it is completely ignored. I very clearly explained that the first two errors were due to the variable $result not being defined before it was attempted to be use. To which he replies Which was after the line causing the error. This was also the problem that was pointed out in his previous thread.
-
I'm not sure what to tell you. If all you left out was the HTML then you never define $result before the FIRST TIME that you use it. LATER int he code you define it and use it. But, on the 8th line of code you posted at the top you have the line $row = mysql_fetch_array($result); and $result had never been defined. I am going to offer the following advice/critiques: Your code is a mess. If it was structured in a more logical format the issues you face would be easier to find/fix You need to put more thought into your logic and the code you are creating. Remember YOU are the one who wrote the code and yet you can't seem to find/fix errors that PHP is providing you with line numbers.
-
That is not the entire code producing those errors. The code you posted above has only 134 lines, yet the very first error reported shows an error on line 138. So, I'm guessing you are including that fine in another or something. Anyway, look at the very top of your code <?php include ('php only scripts/db.php'); error_reporting(E_ALL); ?> <?php $row = mysql_fetch_array($result); See that last line? No where in the previous lines is $result ever defined. So, that explains the first couple of errors. Then the first place I see the variable $nr is here $lastPage = ceil($nr / $itemsPerPage); Again, nowhere before that line do I see it defined. As for the last request in your other post of about the page numbers. Yes you do force the $pn variable to be between 1 and $lastPage using this if($pn < 1){ $pn = 1; }else if($pn > $lastPage){ $pn = $lastPage; } But you were asking why you were seeing -1 and 0. And, I stated there that you were likely using completely different variables in your output. Well look here: $sub1 = $pn - 1; $sub2 = $pn - 2; $add1 = $pn + 1; $add2 = $pn + 2; So, if the page was "1", $sub1 and $sub2 would have values of -1 and 0, respectively
-
Are you sure there aren't already linebreaks in the data? I.e. does it look like this in the source of the HTML (if you don't add any formatting to it): 01/08/2007 this is the first line of text 01/04/2007 this is the second line of text 01/04/2007 this is the third line of text If so, then all you need to do is output the data using nl2br() such as while($row = mysql_fetch_array( $result )) { $output = nl2br($row['History']); echo "<tr><td>{$output}</td></tr>\n"; }
-
From what you've provided? No. Just because you have a block of code to force a variable to be no less than one and no more than $lastPage doesn't tell us anything about where you may be using those variables elsewhere on the page. Or, you might not even be using those variables for all we know - which was your first problem in this thread. In fact, this isn't even the issue you first started int his thread. You don't get your own dedicated thread to work through many different problems. Once a problem is resolved, mark the thread as solved and open a new thread for new problems.
-
I think we are getting to the same conclusion, but have different reasons for getting there. If the user wants a password called "password" or wants to enter the star spangled banner in 1337 code that's up to them. With a few exceptions (financial/medical information), an application should not be the arbiter of what is an acceptable password. And, the only reason I think financial/medical type sites should require "complex" passwords is not because they "should" but because they have to in order to prevent lawsuits from users that use simple passwords.
-
I disagree with that. If a malicious user is trying to hack a hashed password they will use common words/phrases before they start using something like "#$az8Q{mw". But, I would not suggest enforcing complexity to the user (unless it was a financial site). At some point you have to put some responsibility on the user. The same length as the username field. It's visually appealing for them to line up. That is the visual width of the element - which is not related to the length (i.e. how many character the field will accept)
-
If you do not use ENT_QUOTES and the content of $var contains a single quote mark it will break that output. Take the example of the value being "Tom O'Maley" (without the double quotes). The output would be <div data-title='Tom O'Maley'>Tom O'Maley</div> The value of the data-title parameter would resolve to "Tom O" because the single quote in "O'Maley" would close the opening single quote for that parameter.
-
Your question does not have a strait forward solution. There are some technical aspects and some legal ones. There's always the chance that the user may upload or provide the url of an image that is copyrighted. So, you need to be careful with how you use it. But, that aside, having the user provide a url is definitely riskier. Depending on how you implement this, you could be liking to an image from some site (or copying the image) which would very easily be violating the terms of user for the website that is hosting the image. So, make sure what you are doing will not put you into legal trouble. As for implementation. For uploads you will have to copy the image to your hosting server. Then you can simply send an HTML email with an image tag in the content pointing to the image that was uploaded. You *can* also embed the image in the email, but that is more difficult and a pain IMO. If the user provides a url you can just put the image tag in the email with that url. You would probably want to verify that the url is valid first. Alternatively, you could copy the image to your server, but that seems unnecessary.