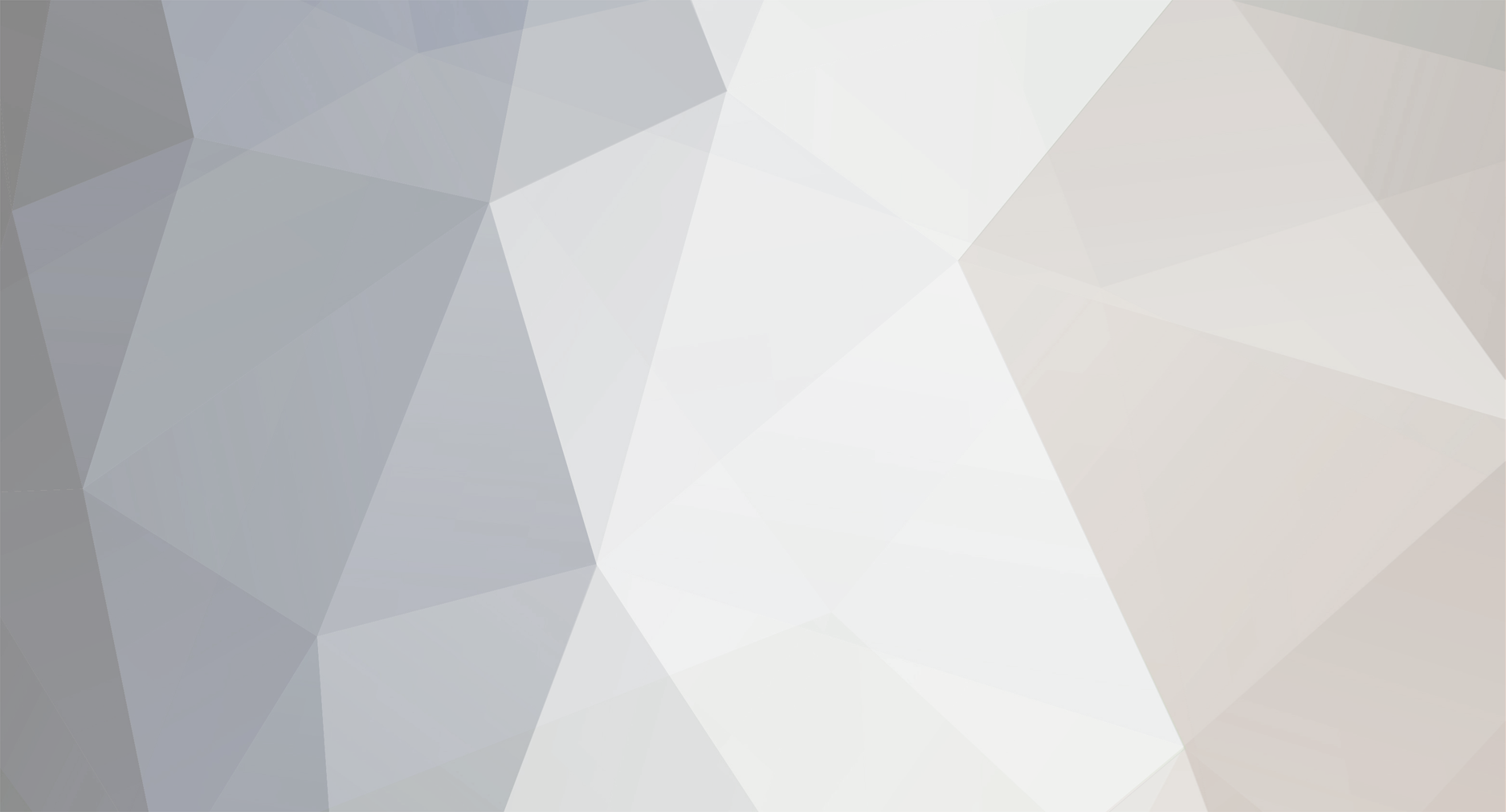
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
If statements inside message body - Form Email Output
Psycho replied to vschiano2008's topic in PHP Coding Help
OK, I enjoy these types of problems and wanted to provide some best practices to you. But trying to explain everything through a forum would take ages. So I went ahead and rewrote the form and the processing logic. Everything is pretty much driven by a set of arrays. So, you can add/remove options in any of the select list or add/remove additional rows in the form just by editing an array. Also, when processing the data, records with no appropriate values are excluded: - Employee records must have a first or last name - Equipment must have a number - Materials must have a QTY - Sub Contractors must have a Name or Hours I expect that you may want to tweak some of that logic. For example, you may want to reject Sub Contractors records unless they have a name. I.e. If only hours are entered exclude them. Or you may want to make those validation errors that prevent the form from submitting. So, if there is a Sub Contractor record with hours entered but no name, create an error and send back to the user. I'll leave that for you to implement. There are three files attached: FDC.php This is the main file. It will determine if the form was posted. If so it will perform any validations. If validations pass it will send the email. If there are errors or the form was not posted, the form script will be included. And, if there were errors an error message will be displayed at the top of the form AND the form fields will be sticky - i.e. any data the user entered will be populated so they don't have to re-input all the data. fdc_form.php This is the script to display the form. There are arrays right before each select field to determine how many sets of input to display and the defaults for the select fields. Just modify these arrays to add/remove as needed. lookup_data.php This script contains arrays of the values to use for each of the select fields. If you need to modify the available values, just edit these arrays. 18786_.php 18787_.php 18788_.php -
If statements inside message body - Form Email Output
Psycho replied to vschiano2008's topic in PHP Coding Help
I believe you meant to say "repetition" not "reputation". -
If statements inside message body - Form Email Output
Psycho replied to vschiano2008's topic in PHP Coding Help
OK, you need to start with modifying your form to make this work efficiently. Here was a quick rewrite of your form which was written as a flat HTML page. It was over 1,000 lines. The rewrite below creates the form exactly as you had it before except that it does it programatically and takes less than 200 lines of code. Note, I did make one change. For the start/end times I used single select lists for the time (including the AM/PM). having the user click two fields to enter a time is a waste and it makes processing that much more difficult. So, try this code out and look at what it is doing. I would also put the arrays to define the select lists into a separate file which is included. That way you can use the same arrays on the processing page. When I get some more time I go through your processing logic. <?php //Function to create options lists from an array function createOptions($values, $selectedValue) { $optionsHTML = ''; foreach($values as $value) { $selected = ($value==$selectedValue) ? ' selected="selected"': ''; $optionsHTML .= "<option value='{$value}'{$selected}>{$value}</option>\n"; } return $optionsHTML; } $startTimeOptions = ''; $endTimeOptions = ''; for($time=0; $time<24; $time+=.5) { $hour = ($time%12==0) ? '12' : $time%12; $min = ($time==ceil($time)) ? '00' : '30'; $ampm = ($time<12) ? 'AM' : 'PM'; $timeStr = "{$hour}:{$min} {$ampm}"; $startSelected = ($timeStr=='7:00 AM') ? ' selected="selected"': ''; $startTimeOptions .= "<option value='{$timeStr}'{$startSelected}>{$timeStr}</option>\n"; $endSelected = ($timeStr=='3:30 PM') ? ' selected="selected"': ''; $endTimeOptions .= "<option value='{$timeStr}'{$endSelected}>{$timeStr}</option>\n"; } $equipList = array('Backhoe', '6 Wheel Dump', 'Van/Truck', 'Compressor', 'HDD', 'Trailer', 'Road Saw'); $materialList = array('Asphalt Base', 'Asphalt Top', 'Sand', 'Fill', 'Stone', 'Debris Removed', 'Cold Patch', 'Poured Concrete', 'Cement Bags'); $subTypeList = array('Dump Truck', 'Vac Truck', 'Boom Truck', 'MPT', 'Other'); ?> <!DOCTYPE HTML> <html><!-- InstanceBegin template="/Templates/DWcourseMultiscreen.dwt" codeOutsideHTMLIsLocked="false" --> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width"> <!-- InstanceBeginEditable name="doctitle" --> <title>Field Data Capture</title> <!-- InstanceEndEditable --> <link href="file:///F|/Users/Victor/Downloads/assets/thrColHFmulti.css" rel="stylesheet" type="text/css"> <!-- Phone --> <link href="file:///F|/Users/Victor/Downloads/assets/phone.css" rel="stylesheet" type="text/css" media="only screen and (max-width:480px)"> <!-- Tablet --> <link href="file:///F|/Users/Victor/Downloads/assets/tablet.css" rel="stylesheet" type="text/css" media="only screen and (min-width:481px) and (max-width:800px)"> <!-- InstanceBeginEditable name="head" --> <!-- InstanceEndEditable --> </head> <body> <div class="content"><!-- InstanceBeginEditable name="content" --> <body bgcolor="#FFFFFF"> <h1>Field Data Capture</h1> <p> </p> <form action="EmailForm.php" method="post" name="EmailForm"> <table width="100%" border="1"> <tr> <td>Date</td> <td><input type="text" name="Date"></td> </tr> <tr> <td>Job Number</td> <td><input type="text" name="JobNum"></td> </tr> <tr> <td>Job Type</td> <td> <input type="text" name="JobType"></td> </tr> <tr> <td>Inspector</td> <td><input type="text" name="Inspector"></td> </tr> <tr> <td>On Street</td> <td><input type="text" name="OnStreet"></td> </tr> <tr> <td>Cross Street 1</td> <td><input type="text" name="CrsSreet1"></td> </tr> <tr> <td>Cross Street 2</td> <td><input type="text" name="CrsStreet2"></td> </tr> <tr> <td>Work Description</td> <td><textarea name="Desc" cols="50" rows="5"></textarea></td> </tr> </table> <table width="100%" border="1"> <tr> <th scope="col">First</th> <th scope="col">Last</th> <th scope="col">Start</th> <th scope="col">End</th> </tr> <?php //Create input fields for employee time entry $employeeCount = 12; for($empIdx=0; $empIdx<$employeeCount ; $empIdx++) { echo "<tr>\n"; echo "<td><input name='emp[{$empIdx}][first]' type='text'></td>\n"; echo "<td><input name='emp[{$empIdx}][last]' type='text'></td>\n"; echo "<td><select name='emp[{$empIdx}][start]'>{$startTimeOptions}</select></td>\n"; echo "<td><select name='emp[{$empIdx}][end]'>{$endTimeOptions}</select></td>\n"; echo "</tr>\n"; } ?> </table> <table width="100%" border="1"> <tr> <th scope="Equip#">Equip #</th> <th scope="EquipType">EquipType</th> </tr> <?php //Create input fields for equipment usage entry $selectedEquipment = array('Backhoe', '6 Wheel Dump', 'Van/Truck', 'Van/Truck', 'Compressor', 'Trailer', 'Road Saw'); foreach($selectedEquipment as $equipIdx => $equipment) { echo "<tr>\n"; echo "<td><input name='equip[{$equipIdx}][num]' type='text'></td>\n"; echo "<td><select name='equip[{$equipIdx}][type]'>\n"; echo createOptions($equipList, $equipment); echo "</select></td>\n"; echo "</tr>\n"; } ?> </table> <table width="100%" border="1"> <tr> <th scope="col">Material</th> <th scope="col">Qty</th> </tr> <?php //Create input fields for material usage entry $selectedMaterial = array('Debris Removed', 'Sand', 'Asphalt Base', 'Cold Patch', 'Cement Bags'); foreach($selectedMaterial as $materialIdx => $material) { echo "<tr>\n"; echo "<td><select name='material[{$materialIdx}][qty]'>\n"; echo createOptions($materialList, $material); echo "</select></td>\n"; echo "<td><input name='material[{$materialIdx}][type]' type='text'></td>\n"; echo "</tr>\n"; } ?> </table> <table width="100%" border="1"> <tr> <th scope="col">Subcontractor Type</th> <th scope="col">Vendor Name</th> <th scope="col">Hours</th> </tr> <?php //Create input fields for subcaontractor entry $selectedSubType = array('Dump Truck', 'Vac Truck', 'Other'); foreach($selectedSubType as $subTypeIdx => $subType) { echo "<tr>\n"; echo "<td><select name='sub[{$subTypeIdx}][type]'>\n"; echo createOptions($subTypeList, $subType); echo "</select></td>\n"; echo "<td><input name='sub[{$subTypeIdx}][name]' type='text'></td>\n"; echo "<td><input name='sub[{$subTypeIdx}][hours]' type='text'></td>\n"; echo "</tr>\n"; } ?> </table> <input type="Submit" name="Submit" value="Submit"> </form> <!-- end .content --> <p><br> </p> <!-- InstanceEndEditable --><!-- end .content --></div> <div class="footer"> <p>Network Infrastructure Inc., All Rights Reserved</p> <!-- end .footer --></div> <!-- end .container --></div> </body> <!-- InstanceEnd --></html> -
If statements inside message body - Form Email Output
Psycho replied to vschiano2008's topic in PHP Coding Help
If you implement your fields in that manner, then you will use a foreach() loop to process the individual field sets for the employees. By hardcoding everything you are making the code much more difficult to maintain and are drastically increasing the likelihood of introducing bugs that are going to be harder to find. If you use a loop to process different sets of records you are assured that if the loop is working correctly for one set it is working the same for all sets. Your form is a flat HTML file that is over 1,000 lines long! By creating loops to generate the field sets and the select options you could reduce the code to well under 25% of it's current size and it will be more flexible and scalable. Let me review your form and see what I can come up with. -
If statements inside message body - Form Email Output
Psycho replied to vschiano2008's topic in PHP Coding Help
OK, you can solve your problem AND greatly reduce the complexity and size of your code at the same time. Right now you have a lot of hard-coded field names. Instead, make your field names more programmatic. You are making this way harder than it should be. So, instead of having name fields such as <input type="text" name="first1"> <input type="text" name="last1"> <input type="text" name="first2"> <input type="text" name="last2"> <input type="text" name="first3"> <input type="text" name="last3"> Create your fields as arrays such as <input type="text" name="emp[1][first]"> <input type="text" name="emp[1][last]"> <input type="text" name="emp[2][first]"> <input type="text" name="emp[2][last]"> [code]<input type="text" name="emp[3][first]"> <input type="text" name="emp[3][last]"> Now you can do two things: 1. You can create your form programatically and you can process it programatically. In the processing code you could do somethign such as this foreach($_POST['emp'] as $empIndex => $employeeData) { //Process each employee separately } By writing all the same logic to process each employee the same you make it very possible of having errors that are difficult to find. by creating a loop to process each record you can reuse the same code. It will also make it easy to skip any records that have an empty value. -
Returning multiple instances of occurences in an array
Psycho replied to yoursurrogategod's topic in Javascript Help
I'm not too familiar with JQuery. There might be a function to do that, but I'm guessing not. Just wrap the code above in a function and use that. function (haystackArr, needle) { var indexMatches = newArray(); var index = 0; while(jQuery.inArray(haystackArr, needle, index) != -1) { index = jQuery.inArray(haystackArr, needle, index); indexMatches[indexMatches.length] = jQuery.inArray(haystackArr, needle, index); } return indexMatches; } -
Then, it seems, you're going to need to build a very robust post-processing engine Well, you apparently don't know how to do what you are trying to do now either. So, if you need to learn how to do something, why not learn a better way? I'm not an expert in MySQL, but I know that repopulating the data into appropriate tables can be achieved without modifying the code or functionality of the app you are currently using. A couple things you should look into are MySQL triggers and MySQL stored procedures. The first is a process to instruct MySQL to perform some action when a particular event occurs (in this case when a record is inserted or updated in the table). The former is a set of code that MySQL will perform. You may even be able to accomplish this only using a trigger. The only work to do would be to parse the metadata value and then perform operations based upon that. But, MySQL has plenty of functions that should be able to do that. http://net.tutsplus.com/tutorials/databases/introduction-to-mysql-triggers/ http://net.tutsplus.com/tutorials/an-introduction-to-stored-procedures/
-
Returning multiple instances of occurences in an array
Psycho replied to yoursurrogategod's topic in Javascript Help
Hmm . . . look at the page that you linked to Look at that last, optional parameter. That tells the function what index you want the searching to start from. The function returns the index of the first found match, right? So, just create a loop to continue looking for a match and increasing the index after each match. var indexMatches = newArray(); var index = 0; while(jQuery.inArray(haystackArr, needle, index) != -1) { index = jQuery.inArray(haystackArr, needle, index); indexMatches[indexMatches.length] = jQuery.inArray(haystackArr, needle, index); } Not tested -
$regEx = '#<a href="user\.php\?i=[^>]+>([^<]*)#ism'; preg_match_all($regEx, $text, $title); Although, I will proffer another suggestion. Sometimes I have had a similar problem and found that trying to build the perfect regex is either not possible, too much work, or not efficient. In those cases look to other means to post-process the data. In this instance if there was not a good solution for what you needed you could have simply used striptags() on the result to remove the image tag.
-
harder on the server to cycle through a directory or parse xml
Psycho replied to koolaid's topic in PHP Coding Help
Why not test it? Because depending on HOW you process the directory or XML file could have an impact. But, I would have to assume that an XML file would be more efficient. How many files are we talking about. If it's a small number then performance won't be an issue. And there are other considerations. For example, with an XML solution the data is only as good as the last time the XML file was created. By using the file system your results will be updated in real-time. -
You've had this discussion before because the way the data is being stored is incorrect for how you are trying to use it. As gizmola explained (and I believed I did in a previous thread) you are creating way more work than you have to be (or should be) doing. Even if you have no control over how that application puts data into the database, that doesn't mean you have to work with it that way! A better approach, IMHO, would be to create a process to repopulate that data into normalized tables so you can use the database how it should be used.
-
Need some help with a simple form validation
Psycho replied to p3nguattack's topic in PHP Coding Help
Here's my take. PHP is a loosely types language. This means that different values can resolve to the same thing. You have this in your comparison $_POST[$fieldname] != 0 That is checking if the value IS NOT equal to the INTEGER of 0. In PHP a 0 is also considered false. false is also equivalent to an unset value when using empty()! So, two things: 1 - you should have used $_POST[$fieldname] !== '0' To check that that value was not EXACTLY a string with the value of '0'. 2 - Even that would have flaws and I would suggest a different approach. For example, if the user only entered spaces the validation would pass because it would not be an empty value. I have to assume you don't want someone to enter just spaces. So, I would suggest trimming the value first (if set), then only checking that the value is not an empty STRING [i.e. don't use empty()]. foreach($required_fields as $fieldname) { $fieldValue = isset($_POST[$fieldname]) ? trim($_POST[$fieldname]) : ''; if ($fieldValue=='') { $errors[] = $fieldname; } } -
You are responsible for marking your post solved (although the staff can do that too). You should go ahead and do that now. You can always come back later and make it unsolved if you have issues or open a separate thread if the issue is somewhat different.
-
If your directory doesn't contain any wav files that you do NOT want to use for this, then there is an easier solution. The following will build the buttons (and play the selected file) automatically by reading the directory where the files reside. No need to define the start and end of the file numbers and the files don't even need to be named numerically. $nombre = 'DIRECTORY'; $ext = 'wav'; //Get list of wav files from directory //Requires file ext to be same case, i.e. 'wav' $files = glob("{$nombre}/*.{$ext}"); foreach ($files as $file) { $fileName = basename(substr($file, 0, strrpos($file, '.'))); echo "<form method='post' action=''> <input type='submit' name='escuchar2' value='{$fileName}'> </form>\n"; } if(isset($_POST['escuchar2'])) { $file = $_POST["escuchar2"]; echo "<embed src ='{$nombre}/{$file}.{$ext}' hidden='true' autostart='true'></embed>"; }
-
How To: Show Table TITLE Values; and Hide/Show DETAIL Value
Psycho replied to EmperorJazzy's topic in Javascript Help
<< This is a JavaScript issue, moving to the appropriate forum >> JQuery is simply a JavaScript framework of sorts that has a lot of built-in functionality that you can use without having to write from scratch. It does not need any support from your host as it is all run client-side. But, on the client-side you have to include the JQuery framework to be loaded into the browser. E.g. <script type="text/javascript" src="jquery.src"></script> -
If you are going to display the contents of the arrays the same way then there is a MUCH easier way then rewriting all that same code. Call the main function passing a parameter to determine which array to use, then use the same process to output the array. Here's some sample code where you would only need to modify the switch statement to select the right array object. function setCategory(category) { var outputObj = false; //Select the array to use switch(category) { case 'muscleTestingGrades': outputObj = abbreviationsMuscleTestingGrades; break; case 'doNotUse': outputObj = abbreviationsDoNotUse; break; } //Check that a valid value was passed if(outputObj===false) { return false; } var outputLength = outputObj.length; var HTMLToOutput = '<table>'; for(i = 0; i < outputLength; i++) { HTMLToOutput += '<tr>'; HTMLToOutput += '<td>' + outputObj [i].ACRONYM + '</td>'; HTMLToOutput += '<td>' + outputObj [i].VALUE + '</td>'; HTMLToOutput += '</tr>'; } HTMLToOutput += '</table>'; var outputDiv = document.getElementById('SearchResultsArea'); outputDiv.innerHTML = HTMLToOutput; return; }
-
No. You obviously didn't really read my response or even try it. What I provided was exactly what you just wrote, but mine was more efficient. I don't know why you changed the sample inputs in your later example - it only confuses the situation. //My code $num1 = 50; $num2 = 100; $result = ($num1/100) * $num2; //$result will be 50, i.e. 50% of 100 //Your code $number3 = 50; $number4 = 100; $number3 /= 100; $number1 = $number3 * $number4; //$number1 will be 50 (i.e. 50% of 100);
-
Because you apparently didn't change the calls to the function to pass the field 'object' as I suggested: onclick="setCategory(this);. Hard coding all those passed values is a waste since you can simply reference the field name/id. Hard coding values like that will lead to problems in maintaining the code. Just one typo could create a bug that could be difficult to find. If you want the results on the page - without wiping out the current content, then don't use document.write(). Instead, create a div where you want the contents displayed. Then create content you want to display into a string variable. Lastly, put the content into the div using something like document.getElementById('ouputDivID').innerHTML = contentVar;
-
Well, it worked for me. You state that the array does have the values you expect. But, apparently, the extracted array value using array_shift() is producing a '1'. Are you sure you implemented the code correctly? There's no logical reason I can think of that that would happen. Where's the exact code you implemented?
-
While I will agree that you are technically correct that a table name would not be escaped. However, if the data is user submitted then escaping will at least prevent SQL Injection. But, I completely agree that comparing the value against valid names is definitely the way to go. However, regarding the comment about a table name being an identifier and not needing to be quoted, I have to disagree. A column name is also an identifier. The reason for quoting them is to prevent issues when the name is a reserved word or has certain characters (e.g. a space). While using reserved words or certain characters is probably bad practice - it IS valid. So, adding quotes to cover those situations is valid. From the MySQL manual: http://dev.mysql.com/doc/refman/5.0/en/identifiers.html In this case the table name is a variable. I don't know what table names he is using now (or in the future). So, quoting the table name is appropriate. EDIT: OK, I have to go back on what I said about escaping the table name would at least prevent SQL Injection. If the table name did have certain special characters then escaping the name would actually prevent the table name to be used in the query. But, again, it's just bad practice to use it like that.
-
Hmm, your question, as stated, doesn't make sense. You state If you put a "0.10" behind $num1 it would result in 100.10. I *think* what you are wanting to do is treat $num1 as a percentage. So, $num1 * $num2 = (0.10) * 10 = 1. Is that correct? Then this is simple math and not a coding issue: $num1 = 10; $num2 = 10; $result = ($num1/100) * $num2; echo $result;
-
EDIT:scootstah beat me to it, but I'll post this anyway as I included some additional info You need to implement good coding standards. 1. Use 'correct' indexes in arrays. $_POST[arc] should be $_POST['arc']. What you have will work but is inefficient and poor practice 2. Create your queries as a string variable so you can echo to the page 3. Check for errors if your queries fail. 4. ALWAYS, ALWAYS escape user submitted data used in tables. If you had echo'd out the query the problem would be obvious. By concatenating strings on separate lines it *looks* proper. But, there is no spaces between the table name and "ORDER BY'. $dbt = mysql_real_escape_string($_POST['arc']); $query = "SELECT id, fname, mi, lname FROM `{$dbt}` ORDER BY `date` DESC LIMIT {$offset}, {$rec_limit}"; $result = mysql_query($query); if(!$result) { echo "There was an error running the query<br>\n"; echo "Query: {$query}<br>\n"; echo "Error: " . mysql_error(); } else { //Continue with processing results }
-
Then why didn't you say so. That's easy enough: $dir = 'home/test/'; $date = '2012-07-26'; //Get the contents of directory & remove folders $files = array_filter(glob($dir.'*'), 'is_file'); //Run query to get records $query = "SELECT CONCAT('{$dir}', number, '.jpg') as newName FROM test WHERE date = '{$date}' GROUP BY number ORDER BY date, time"; $result = mysql_query($query); if(mysql_num_rows($result) != count($files)) { die('The number of files and DB records do not match'); } while($oldName=array_shift($files) && $row=mysql_fetch_assoc($result)) { //Rename the file rename($oldName, $row['newname']); } Also added a change to format the new file name within the query!
-
There is no such thing as "javascript work[ing] within php". I guess that is where the confusion is coming from. You are using PHP to dynamically create the href value. That is all done on the server. Then, the completed page (including JavaScript) is sent to the user. There is no more PHP in the page the user receives. The JavaScript is then executed on the client-side. That is why I stated you should hard-code the link to work as you want it to and then figure out how to dynamically create it using PHP. This really isn't a PHP issue, but a JavaScript issue. What I provided should work depending on what you are really doing in that JavaScript code. My guess is that the JS code is doing something that is preventing the link from being processed.