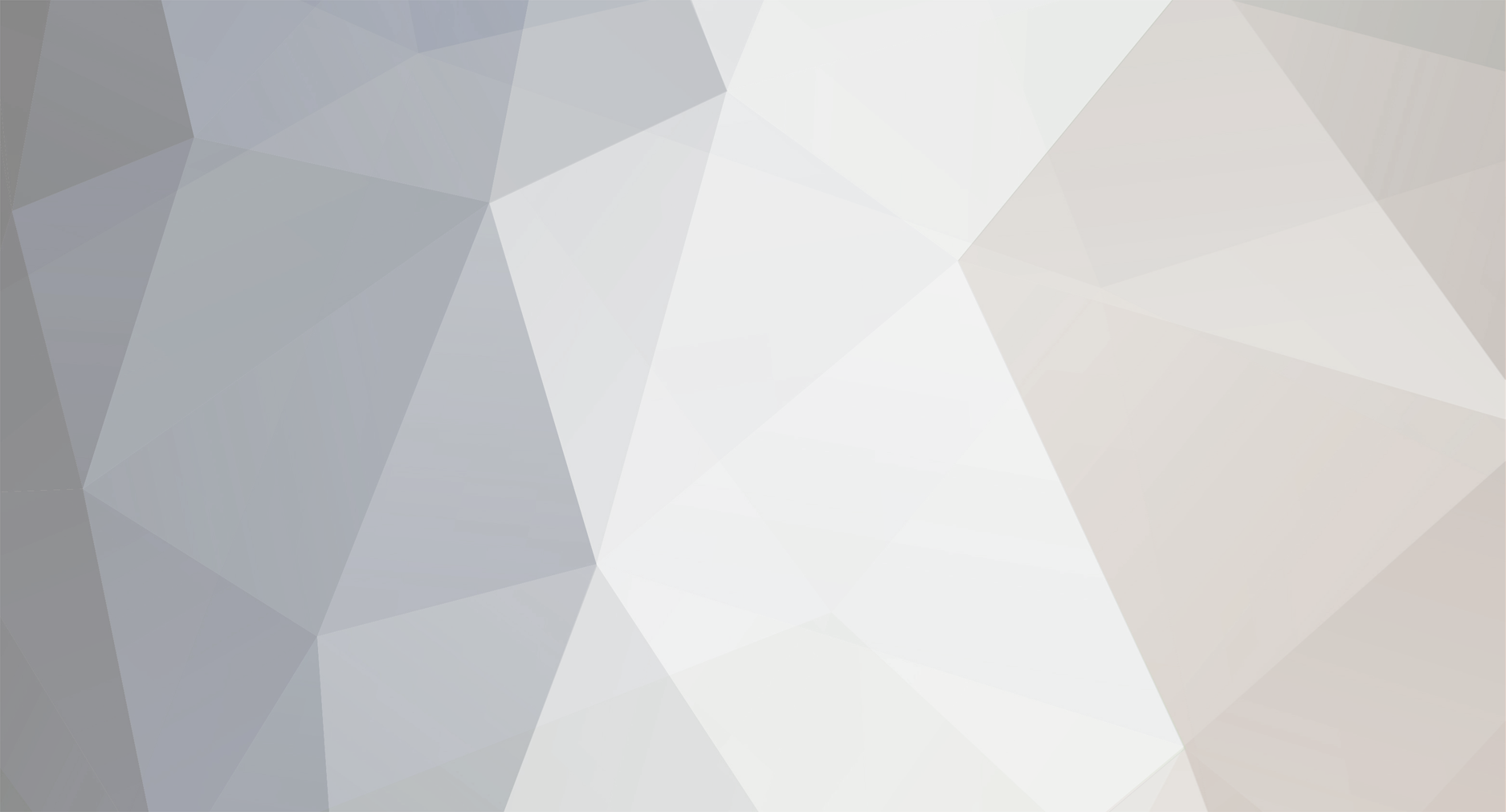
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I really don't understand your second sentence. But, with a mufti-dimensional array I can't think of any solution other than looping through each primary array. But, you can make it more efficient by exiting the loop as soon as the first non-positive number is encountered. <?php $all_positive = true; foreach($theArray as $subArray) { if($subArray['key_to_be_searched'] < 0) { $all_positive = false; break; } } if($all_positive) { echo "All the elements were positive"; } else { echo "All the elements were not positive"; } ?> If you need to do this for multiple columns, then I'd make it a function
-
I'm not really following your explanation. You state you want to exclude the output where "2 items ARE inside a table". I read that as if there are two records with the same value. but, I think you mean you want to exclude records where to fields have two different values. It also looks like you are trying to run two queries to accomplish that. That''s unnecessary. If there are records you are not going to use - then don't query them. You have one table for events and another table for the bets by users for those events and you want to exclude the events where the user has already placed a bet. So change the first query to exclude the records you don't want. I'm not sure what fields from the two table are used to associate them, but I am going to guess that the fight_id in the bets table is used to associate with the id in the fights table. Not sure, but you can make the correction as needed. I can think of two ways to accomplish this, but not sure which is more efficient: SELECT * FROM fights WHERE event='$viewevent' AND id NOT IN (SELECT fight_id FROM betting WHERE user_id='$user->id') ORDER by id ASC SELECT fights.* FROM fights LEFT JOIN betting ON betting.fight_id = events.event_id AND user_id='$user->id' WHERE fights.event='$viewevent' AND betting.fight_id IS NULL ORDER by fights.id ASC EDIT: Modified errors in 2nd query
-
You apparently did not understand my response. There is no problem with supporting three companies (or three hundred). The problem is how that has been implemented in the database. There should not be a separate column to specify the association with each company. That creates significant problems in being able to perform simple queries and will not scale. If you ever needed to add a new company you would have to go back and modify the code and database to support it. If it was implemented correctly you could easily add more companies by adding just one record to a companies table. There should be tables such as: companies: a table to describe just the companies products: a table to describe JUST the product details prod_comp: a table to describe each individual product-company association. So, the companies table would have three records such as comp_id | comp_name 1 Company 1 2 Company 2 3 Company 3 Then let's say you have a product "ABC" that should be associated with company 1 and company 3. The product table would ONLY contain the product specific information: product name, product id, description etc. Such as: prod_id | prod_name | description 13 ABC Description for product ABC Then in the product_company table you would have two records to associate the product to the two companies: prod_id | comp_id 13 1 13 3 But, if you want to stay with the DB structure you have, which I do not advise, I've provided an explanation of why your current code does not work as well as sample code to get the results you want. But, I do not have your database nor am I willing to invest the time necessary to test the code. but, if you run into problems and can't figure it out you can post back.
-
This is not the correct way to query records based upon a date range AND ($date - create_date <= 20) What does '20' represent? Minutes, days, seconds, microseconds? See the problem. So, that query to check for a duplicate is probably never finding duplicates - if that is even the problem. Look into the date_sub() and date_add() MySQL functions. Then you can do something such as this AND (date_add(create_date, INTERVAL 20 MINUTE) > NOW()) OR AND (date_sub(NOW(), INTERVAL 20 MINUTE) > create_date)
-
You don't need to ADD in $_GET coding for each of the pages. You write the page one time with the appropriate code to grab the code from the URL. Then that ONE PAGE page works for ALL users/codes. No need to create directories, copy files, etc.
-
On an unrelated note, why are you storing some of the same data in the separate tables? The only different information I see being put into the "tkts_replies" table is the text and a visibility value. based upon your initial explanation it seems there is always supposed to be 1 and only 1 record in the "tkts_replies" table for each record in the "tkts_topics". So, it makes no sense to duplicate data and I don't even see the logic in having two tables. Also, I see the value of the 'visibility' field is being set to the string 'on'. Assuming that there are only two possible values ('on' and 'off') you should not be using text for those fields. For logical true/false values you should be using a tinyint type field and using the values of 1 (true) and 0 (false). Then change the name to 'visible' so the true/false make more sense. Lastly, if the default value will always be true (i.e. 'on') then set that as the default value for that field in the database and you can remove that field from the insert query. Same goes for the data - you can set up a date field to auto-populate with the creation and/or modification date.
-
Although it may seem counter intuitive what you want is a single record for each friend association. So, if a user has five friends there will be five records in the friends table. If you put many fried records into a single field you cannot do appropriate searches. And, having multiple columns ina table wont scale.
-
You don't need (and can't have) two auto-increment ids in a single table. Each table can have one auto-increment ID and then you use those keys in other tables to relate the data. In your first post you stated you had a table with the following fields: bet_id match_id user_id fighter_id method round bonus Assuming that is the bet tables then those fields should be something as follows: bet_id (auto-increment field for this table) match_id (a foreign key of the auto-increment field from the matches tables) user_id (a foreign key of the auto-increment field from the users tables) fighter_id (a foreign key of the auto-increment field from the fighters tables) method (???) round (a numeric field) bonus (assume this is a float field for an amount)
-
There is nothing inherently wrong with how you are passing the array. Let's trace back through the code to figure out what may be wrong. The error you are getting is triggered here: if (submitNewTeam($_POST['user_id'], $_POST['user_team_name'], $_POST['team_id'])) { echo "<p class='normal'>Thank you for submitting a new team.</p>"; } else { echo "<p class='fail'>Team registration failed! Please try again.</p>"; show_team_selections(); } That tells us that submitNewTeam() is not returning a true value. So, now we need to look at that function. function submitNewTeam($user_id, $user_team_name, $valuesAry) { $query1 = "INSERT INTO user_teams (user_team_id, user_id, team_name, date)VALUES ('', '{$user_id}', '{$user_team_name}', NOW())"; $result = mysql_query($query1) or die("Query: {$query1}<br>Error: ".mysql_error()); $user_team_id = mysql_insert_id(); //Put group selections into arry in MySQL INSERT value format $valuesAry = array(); foreach($_POST['team_id'] as $team_id) { $team_id = mysql_real_escape_string(trim($team_id)); if($team_id != '') { //Only add if not empty $valuesAry[] = "('{$user_team_id}', '{$team_id}', NOW(), '2100-1-1 00:00:01')"; } } $query = "INSERT INTO team_selections (user_team_id, team_id, transfer_in, transfer_out)VALUES " . implode(', ', $valuesAry); $result = mysql_query($query) or die("Query: {$query}<br>Error: ".mysql_error()); } Well, that code looks pretty good. You have some error handling in there and it is logically constructed. But I see a couple problem and have some suggestions: 1. The first ptoblem is that you are passing the array of IDs to the function, but then inside the function you are using $_POST['team_id'] instead of the passed value! 2. Don't use mysql_real_escape_string() for ID values. I would assume those should be integer values so you should use intval() or something similar. Because if a non numeric value was passed it would cause an error. 3. Also, you can easily process all the fields in the array without a foreach() loop by using array_map() to apply intval() to all the values and array_filter() to remove empty values. $valuesAry = array_filter(array_map('intval', $valuesAry)); 4. Your queries are using NOW() for the dates. If those fields are supposed to be the creation date or a modification date you should set the fields up in the database so they will be auto-populated/updated. Then you can remove that from your code and let the database do that work. 5. It looks like 'user_team_id' is an auto-increment field. If so, it should be removed from the query. 6. You are running two queries and assigning the results of both to a variable called $result. In both instances you never use that variable. In the first instance that's not a problem. But, in the second you need to be returning that result at the end of the function so your previous if() condition will work! Give this a try <?php function submitNewTeam($user_id, $user_team_name, $teamIDs) { $user_id = intval($user_id); $user_team_name = mysql_real_escape_string($user_team_name); $query = "INSERT INTO user_teams (user_id, team_name, date) VALUES ('{$user_id}', '{$user_team_name}', NOW())"; mysql_query($query1) or die("Query: {$query}<br>Error: ".mysql_error()); $user_team_id = mysql_insert_id(); //Preprocess the array $teamIDs = array_filter(array_map('intval', $teamIDs)); //Put group selections into arry in MySQL INSERT value format $valuesAry = array(); foreach($teamIDs as $team_id) { $valuesAry[] = "('{$user_team_id}', '{$team_id}', NOW(), '2100-1-1 00:00:01')"; } $query = "INSERT INTO team_selections (user_team_id, team_id, transfer_in, transfer_out) VALUES " . implode(', ', $valuesAry); $result = mysql_query($query) or die("Query: {$query}<br>Error: ".mysql_error()); return $result; } ?>
-
OK, I don't see any solution but to use seven different variables because each iteration of the while() loop is a new event and you need to have a history of the events previously. But, it can be simplified. Here is some mock code <?php $file1 = false; $file2 = false; $file3 = false; // . . . //Loop through each fiel and set appropriate var to true if matching file found while($files_names=mysql_fetch_array($files)) { //Find files with SAA in the filename if (preg_match("/SAA/i", $files_names['name'])){ $file1 = true; } //Find files with CSP Seller in the filename if (preg_match("/CSP Seller/i", $files_names['name'])){ $file2 = true; } //Find files with STA POA in the filename if (preg_match("/STA POA/i", $files_names['name'])){ $file3 = true; } //Etc. . . . for each file you are looking for } //end the files_names while loop //One condition check for all files if($file1 && $file2 && $file3) { //All files are present } else { //All files are NOT present } ?>
-
OK, in retrospect I see that won't work. Each iteration of that loop is only processing one file. Give me a sec and I'll post a different solution. I think it will require multiple variables - but we can simplify it.
-
OK, before I get to your issue, if you have a condition that will only set a variable to true or false you do not need to create an if/else condition. Just do something like this $funding_book = (preg_match("/SAA/i", $files_names['name'])) I would assume you would want to provide some details to the case manager as to which files have not been uploaded. But to just check that all files are there or not just set a flag variable at the beginning to true, then as you check for each file you only need to check if it is NOT there - if so set the flag to false. <?php $allFiles = true; //This while loop will loop through all files for all that cases that were selected in while($files_names=mysql_fetch_array($files)) { //Find files with SAA in the filename if (!preg_match("/SAA/i", $files_names['name'])){ $allFiles = false; } //Find files with CSP Seller in the filename if (!preg_match("/CSP Seller/i", $files_names['name'])){ $allFiles = false; } // . . . etc. . . . ?> Then at the end you only need to check that the variable is true. but, I would do something to keep track of which files have and have not been uploaded so you can provide that info in the email.
-
OK, you have one problem with your DB structure. Ideally, you should not have three columns for company1, company2, and company3. You should have a separate to associate each record with each applicable company. So if a product is associated with two companies it would require two records in that 2nd table. If you don't do that it will make some processes more difficult - e.g. doing a search of products by company. But, that is not the reason you have the current problem. The reason you are only getting one company listed in your results is how you are processing the data. Your code STOPS processing company info at the first column where the value is 1 because you are using an if/elseif/else logic. So, if company1 is a 1, it doesn't check columns for the other two columns: <?php if($res['prod_company3'] == 1) { $theCompany = "100"; $theName = "Company3"; } elseif($res['prod_company2'] == 1) { $theCompany = "95"; $theName = "Company 2"; } else { $theCompany = "15"; $theName = "Company 1"; } ?> Does that make sense? using what you have now (which is not ideal) you can list out all the applicable companies for a single records pretty easily. I'm not sure how you want to output those results though. Here's one possibility: <?php function productViewBttn($prodID, $companyID, $companyName) { $output = "<small>({$companyName})</small></h5>\n"; $output .= "<span class='viewBtn'>"; $output .= "<a href='?page_id=169&company={$companyID}&singleProduct={$prodID}'>"; $output .= "</span><br>\n"; return $output; } $searchRequest = strtolower($_REQUEST['searchQuery']); $formQuery = "SELECT cat.cat_name, cat.cat_id, prod.prod_id, prod.prod_name,prod.prod_mainImage, prod.prod_model, prod_company1, prod_company2, prod_company3 FROM products AS prod LEFT JOIN category_assoc AS assoc ON assoc.prod_id = prod.prod_id LEFT JOIN categories AS cat ON cat.cat_id = assoc.cat_id WHERE LOWER(prod.prod_name) LIKE '%". $searchRequest ."%' OR LOWER(prod.prod_model) LIKE '%". $searchRequest ."%' OR LOWER(cat.cat_name) LIKE '%". $searchRequest ."%' OR LOWER(prod.prod_description) LIKE '%". $searchRequest. "%' ORDER BY prod.prod_name ASC"; $results = $wpdb->get_results($formQuery, ARRAY_A); get_header(); ?> <div id="mainContent"> <div id='interiorLeft'> <?php if (have_posts()) : while (have_posts()) : the_post(); ?> <h2><?php the_title();?> For <?php echo $searchRequest; ?></h2> <?php the_content(); ?> <?php endwhile; endif; ?> <hr/> <?php if(count($results) > 0) : foreach($results as $res) { $prImage = (!empty($res['prod_mainImage'])) ? $res['prod_mainImage'] : "noImage.png"; $prodLinks = ''; if($res['prod_company1'] == 1) { $prodLinks .= productViewBttn($res['prod_id'], '15', 'Company 1'); } if($res['prod_company2'] == 1) { $prodLinks .= productViewBttn($res['prod_id'], '95', 'Company 2'); } if($res['prod_company3'] == 1) { $prodLinks .= productViewBttn($res['prod_id'], '100', 'Company 3'); } ?> <div class='productHolder'> <div class='mainThumbnail'> <img src='<?php echo PRODUCT_IMAGE . "/thumbnails/". $prImage;?>' alt='MainThumbnail' /> </div> <h3><?php echo stripslashes($res['prod_name']);?></h3> <h5>Model : <?php echo $res['prod_model']; ?> <?php echo $prodLinks; ?>
-
Good catch PFMaBiSmAd, I forgot that. Although if really old dates are not allowed there might also be a requirement not to allow future dates - but that wasn't mentioned.
-
$day = isset($_POST['day']) ? (int) $_POST['day'] : 0; $month = isset($_POST['month']) ? (int) $_POST['month'] : 0; $year = isset($_POST['year']) ? (int) $_POST['year'] : 0; if(checkdate($month, $day, $year)) { echo "Valid date"; } else { echo "Invalid date"; }
-
Sorry, that isn't what I am looking for. So what ARE you looking for. I think your original question has been answered. Mahngiel provided a link to a post regarding a solution to fall back to a local resource if the hosted resource is not available. But, it really doesn't cover all the possibilities you mentioned. But, then again, why would you use an externally hosted CSS file? If you are going to rely upon externally hosted content and you are concerned about that content not being available then you can simply reference the content as you do now and deal with that problem and possibly use some client-side code to use a local source as a fallback. Another option, which would take some work would be to add some logic to your PHP code to look for new content fails and have a caching system. But there could be some licensing issues to do that. Basically, when the PHP file executes it will check the current modification date of the files you have cached on your machine then read the files at the external location to see if they are new. If they are newer then you replace the cached files. Then in the actual output you have point all the references to the local content. This would ensure the user is always getting the most current files from those external resources but it would load from your server. This would definitely add some overhead - so it's important to understand how important this is to you before implementing.
-
This forum is for people to get help with code they have written and the expectation is that they are knowledgeable enough to implement it. I have no clue about "spry tabs" and am not interested in reading the documentation to find out. Perhaps the freelance forum would be better suited for your needs if there is no one with that product knowledge willing to help you in the PHP Help forum. Good luck.
-
Not that I am aware of, I would assume that the browser has a setting to wait for x number of seconds before giving up. If it was configurable from the web server I could see some scenarios where that could be exploited. It might be configurable from the client side though. But, what is the real problem? If a simple resource such as an image or css file is taking so long it times out that is a connectivity and/or server problem. But, if the actual page is taking too long then that is usually due to some very inefficient code that is taking too long to process. I see this a lot when people put queries in loops that are processing a lot of data.
-
If you only have 1 category and 1 optional sub-category for each product then it is a simple as adding a sort. The problem is that you ARE using a subcategory when there isn't one. Don't use the string "None" to indicate that the record has no category. Either set the field to an empty string or set to NULL. Then you simply need to sort by Category, Subcategory. SELECT * FROM products ORDER BY category, subcategory But, if you are using a textual description to indicate when there is no subcategory you make it harder since you would have to create a derived column to use for sorting or add some other logic. This should work for you, but the better solution is to correct your data. SELECT * FROM products ORDER BY category, subcategory<>'none', subcategory So, records are all first sorted by category. Next they are sorted based upon the condition of the subcategory not being equal to the string 'none'. The records with none will be false(0) and the records that are not 'none' will be true(1) - so the none records come first. Lastly it sorts based upon the subcategory. EDIT: I also assume that you are putting the actual category and subcategory names in those fields - which is also wrong. You should have separate tables to define those and only include foreign key references in your product table.
-
This topic has been moved to MySQL Help. http://forums.phpfreaks.com/index.php?topic=361382.0
-
Retrieving posts and threads then sorting by date
Psycho replied to MySQL_Narb's topic in MySQL Help
It's hard to translate someone's tone over the internet, but I'm going to take it you have an attitude towards me. I've already tried UNION, but I'm not experienced in SQL. I'm not even sure it can help in this case. I've tried working with UNION, but I didn't receive any luck. No attitude - I was merely providing the solution in a succinct manner. I have no idea what your level of ability is with SQL (Are you really using SQL and not MySQL?). There are plenty of resources out there that do a much better job of explaining it than I could ever do in a forum post. So, I suggested you "look it up". FYI: If you had provided some information regarding the actual queries I probably would have provided a revised query for your problem. Plus, it was very late and I was on my way to bed. I though that a solution, no matter how brief, was better than none. If Barand's sample query does not help, then provide the two queries you are currently using. << Moving to the MySQL forum >> -
I think you are still completely missing the problem. What a mod rewrite does has absolutely NOTHING to do with what data you do or do not have in your database. A mod rewrite only does what it is specified to do. Just because you feel it should replace hyphens with spaces doesn't mean squat. Unless you put specifications into your rewrite rules to replace hyphens with spaces it will not replace them. It's like programming your DVR to record a show at 2pm but actually setting it at 2am and then asking aloud "Why would it record the wrong show?". As for my previous post I was not being malicious, but I think it is disingenuous of you to to try and profess that you are "new" to try and deflect criticism of the effort you put forth in helping us to help you. There was not enough accurate information provided in the numerous posts made and some simple tests such as echoing the query to the page were never done. Heck, just echoing $_GET['name'] to the page would have saved yourself and us a ton of time. It was also stated that the query was "blocked", which would infer it failed. But, that was not the case and it was never checked. Verifying input/output data is debugging 101. If some process doesn't work as you expect the first thing to do is to verify the inputs going into that process and the outputs from that process. The above statements are not meant to be malicious. I am merely holding you accountable for your actions and your statements. I can't force you to post "good" questions even though they may not adhere to the forum guidelines. We are here to help. We enjoy it. One suggestion I would make would be to re-read your posts from the perspective of a third person. If you were reading it as posted from someone else without any of the background information you know, would it make sense?
-
Retrieving posts and threads then sorting by date
Psycho replied to MySQL_Narb's topic in MySQL Help
UNION - Look it up -
I think he wants the output to be a string with each letter in single quotes and separated by commas. <?php $colour = "D-E-F-G-H-I-J-"; // string to be formatted. //Explode into array, array_filter() will remove the empty value at the end $colours = array_filter(explode('-', $colour)); //Put single quotes around each element foreach($colours as &$value) { $value = "'{$value}'"; } //Output the values, separated by commas echo implode(',', $colours); // OUTPUT: 'D','E','F','G','H','I','J' ?>
-
Your registration date for this site shows February 18, 2007 (6 years ago). But, I'm questioning that date since the earliest post I can find for you is February 02, 2011 - but that is still well over a year ago. That's MORE than enough time to know that there is a manual at php.net and you can easily look up the details of a function with examples and such.