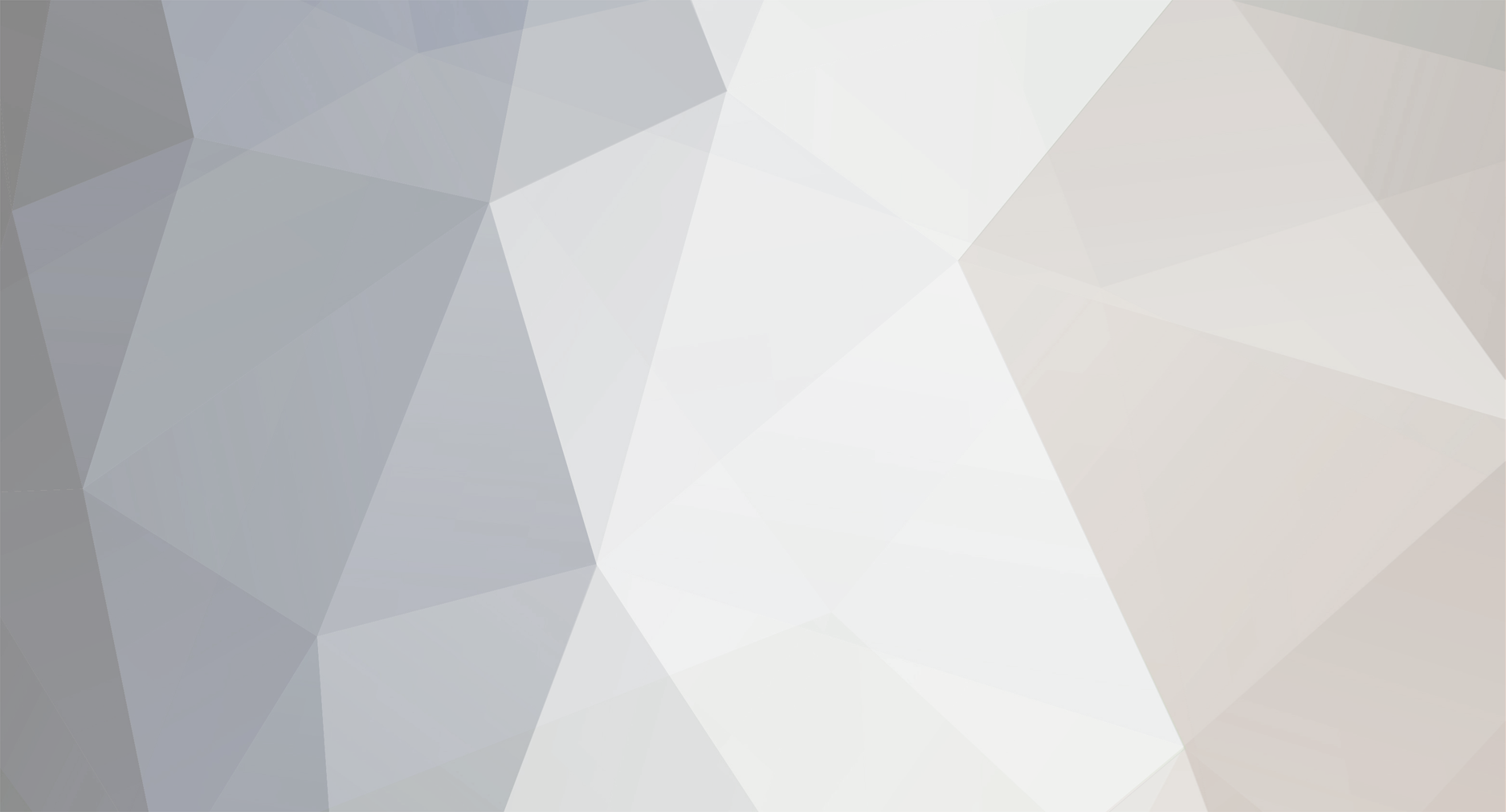
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
OK, playing around with "Lookahead and Lookbehind Zero-Width Assertions" I was able to generate a regex expression that will do all this in one go. Looked like a fun project that would help me learn some more complex regex. Using the same $string in Xyph's sample code I used this expression: #(?<=.\s)[^.]*?foo[\s]*bar[^.]*\b.#is To test I did a preg_match to verify what was being captured: preg_match_all("#(?<=.\s)[^.]*?foo[\s]*bar[^.]*\b.#is", $string, $matches); Here are the results: Array ( [0] => Array ( [0] => We're going to find instances of 'foo bar' and remove those sentences from the string. [1] => See if it can detect when 'foo bar' are on different lines, but the same sentence. [2] => Oh, yeah 'foo bar' ) ) So, going back to your requirement to remove all sentences that contain the target text you can do it in one line: $new_string = preg_replace("#(?<=.\s)[^.]*?foo[\s]*bar[^.]*\b.#is", "", $string); EDIT: Assuming this needs to be done with target text that is variable, you would want to have some process to format that specific string before using in the regex. For example, for "foo bar" I replaced the [space] with "\s" to handle scenarios where there is a line break, tab, etc. between the words. To accomplish that with variable target text you could do a replacement on the target text first. So here is a function that should work for most if not all your needs: function removeSentencesWithText($sentence, $text) { $text = preg_replace("#[\s]+#", "\s", $text); $regex = "#(?<=.\s)[^.]*?foo[\s]*bar[^.]*\b.#is"; return preg_replace($regex, "", $sentence); } $new_string = removeSentencesWithText($string, 'foo bar');
-
You were given a satisfactory response to your question in the very first and second responses. Mahngiel explained in the first response that using '*' would return all data - even the data you didn't need. However, I could see that that response may not have explained why that is a bad thing. So, I followed that up with explanations as to WHY that is ill-advised due to performance and security reasons. Yet, you continued to repeatedly ask for more explanation. And the final response that you say "you got" provided no more information than you had been provided before. Apparently because the text was bolded (?). You failed to do your part as the requester in this thread to read and analyze the information provided to understand it. By not taking the time to try and comprehend the information provided (unless it is bolded) you are going to alienate people from helping you. While there are a great many people that visit these forums, the vast majority of help is provided by a very small number of people that volunteer their time to do so.
-
I think Pikachu has hit the nail on the head. The OP was erroneously suggesting that the query was failing. But, if the code I provided did not produce an error then it must be that he is incorrectly assuming that a hyphen would match a space. All these replies could have been avoided with accurate details provided. @OP: If you want the hyphen in a value to be treated as spaces then do a simple str_replace() on the value before you use it in your query to replace the hyphens with spaced. Alternatively, you could also replace the hyphens with an underscore and change the query to do a LIKE comparison. Then the underscore will be used to match ANY single character.
-
Did you even LOOK at the code I provided? There is no such thing as a query being "blocked". Either the query runs or it doesn't. If it doesn't run an error will be generated. So, if the query doesn't run you should check 1) the actual content of the query and 2) the generated error. When a query has any dynamic content, don't assume you know what the query looks like. I provided revised code that added error handling so you can see #1 and #2 above.
-
I see a problem. The last sentence probably won't have a space after the period. Correct. I had thought about that but didn't include it in my first response as it was late. But, the real trick is not finding the search string within a sentence it is in determining what constitutes a sentence. The "best" solution I can think of is as follows: The beginning of a sentence is either: - The start of the entire text being processed - A character that follows a period + 1 or more white-space characters (space, tab, line-break, etc.) The end of a sentence is where the following comes after a beginning of a sentence: - The end of the entire text being processed - a period + space And, I'm sure if I gave it more thought I would find potential flaws in that. I know you can use ^ and & in the regex for the start and end of the string, but that won't work for this. I think there are separate start/end characters that can be used for this, but I forget how they are implemented.
-
OK, so did you check what the actual query was that was run and the associated error message? $query = " SELECT name, product_id, rrp, discount, image_link FROM productdbase p INNER JOIN furniture_groups f ON f.long_name = p.furniture_group WHERE name LIKE '%{$name}%' LIMIT 15"); $result = mysql_query($query) or die ("Query: <br>$query<br>Error:<br>".mysql_error()); while ($query_row = mysql_fetch_assoc($result)) {
-
Another alternative is to use an array and the in_array() function. If you will use the "list" of pages for several things this would probably be a better approach. You can define the array once and the reuse it for whatever you need. $pages = array('home', 'contact', 'about'); if(in_array($page, $pages)) { //Do something }
-
That's a pretty tall order due to the complexity of what you are asking. You could simply use a regular expression to look for a segment of the string that contains the text where the segment begins the string or follows a period and then ends with a period. But, that is not fool-proof. Because if a sentence contains something like "$12.35" the decimal would be considered an ending period. You could tweak that some more to be where the segment ends in a period + space or the period is the last character in the string, but it still doesn't consider all possible scenarios.
-
It is generally advised to only query the specific column names you need in your query. Using the * to get all columns if you don't actually need them adds additional overhead in running the query which could lead to performance problems. Also, if you use * you could potentially expose sensitive data. If someone were to find a hole in your security to, for example, use SQL Injection to potentially return records they shouldn't they would only get the fields returned by the query rather than all the data.
-
have you looked at any pagination function, tried to implement any code, or done anything? Pagination is pagination, the concept is the same no matter what you are pagination. There are only a few different parameters that you need to work with when implementing any pagination function. The two most important are the dataset and the number of records per page. For your purposes you already know the dataset: -6, -5, -4 . . . 0 . . . 4, 5, 6. And your records per page is 1. Below is a very basic script that creates single-page pagination based upon a set minimum and maximum integers (-6 and 6). I left out any functionality to convert those values into date displays to leave you somthing to actually do on your own. <?php $min_month = -6; $max_month = 6; $selected_month = 0; if( isset($_GET['month']) && $_GET['month']>=$min_month && $_GET['month']<=$max_month ) { $selected_month = intval($_GET['month']); } //Create month menu $month_menu = ''; //Create prev link $prev = $selected_month-1; $month_menu .= ($selected_month>$min_month) ? "<a href='?month={$prev}'><Prev</a> " : "<Prev "; //Create individual page links for($month=$min_month; $month<=$max_month; $month++) { $month_menu .= ($month!=$selected_month)? " <a href='?month={$month}'>{$month}</a> " : " <b>{$month}</b> "; } //Create next link $next = $selected_month+1; $month_menu .= ($selected_month<$max_month) ? "<a href='?month={$next}'>Next></a> " : " Next>"; ?> <html> <body> Select a month index:<br> <?php echo $month_menu; ?> <br><br> Selected Month Index <?php echo $selected_month; ?><br> </body> </html>
-
Take a look at the optional 2nd parameter for strtotime(). Use the "active" date as that parameter and you should get the +/- months based upon that value. Also, be sure to normalize the date to something like the first of the month. I've seen where descriptions like "+1 months" can give unwanted results. E.g. Jan 31st could give March 2nd because of the short number of days in February. I believe that different versions of PHP handle it differently, but I'd force it to give the values I want rather than assume PHP will do it correctly.
-
This is not the best practice of using an "updated_on" field updated_on=NOW() You can set the field up in the database to automatically set the value to the current timestamp when the record is updated. Then you remove that field from your INSERT/UPDATE queries entirely - as it will be automatically handled by the database. Then just run your update query for all the other fields. If none of them are different then no update occurs and affected rows will be 0.
-
Help with PHP MySQL percentage reports with loops?
Psycho replied to malakiahs's topic in MySQL Help
The best approach would be to restructure the database. But, that would mean having to go back and rewrite any functionality that currently writes/reads/deletes from the affected tables. but, that is the best solution since it will remove the complexity that you now face. If you stick with the current structure then I don't see any easy solutions that don't require a lot of loops and multiple queries. But, just to show you how easy it would be to get the data you need with the proper structure, let's assume you have a table for each result as I showed above. You could run just one query such as SELECT question_id, result, COUNT(result) as count FROM results ORDER BY question_id, result GROUP BY question_id, result That should produce a results set such as this question_id | result | count 1 1 10 1 2 13 1 3 8 1 4 3 1 5 22 2 1 3 2 2 10 2 3 6 2 4 13 2 5 4 . . . So, with that single query you get all the data you need. From that you can tell that Question 1 had 10 responses for result 1, 13 responses for result 2, etc. -
Oh, ok. The text-area display in my browser was just a tad too small to show that last line and I didn't notice to scroll down. So you have these two lines $db->importArray($data); _log('Import successful. All Finished!'); The importArray() method has logging events that are working, but the log message right after that method is not getting registered. I would again go back to the premise that something in the importArray() is causing the script to terminate completely. I assume that the actual importArray() method has a looping structure in it where the successful logging is taking place. Have you tried implementing a log message in that method right after the loop before control is returned to where the method was called? I don't know how many lines of code you may have after the loop completes, but you could add log messages between each line to see where the last one succeeds. That's really the only advice I can suggest - add as much debugging as you can to pinpoint the problem.
-
Well, if the 'Importing starting' message is displayed and the 'Importing finished' message is not displayed then something is happening within the call to $this->_import(); that is halting the script. You didn't show that code, so there's no way to tell what the problem is. But, I do have one question/suggestion: You say the process is taking a long time. Yes, 26K records is a lot. But, that also means that introducing even a small piece of inefficient code will have significant consequences. If you are using this data to insert records into a database you should definitely be concatenating all the INSERT records into ONE insert query. Running 26K queries is incredibly inefficient and changing ti to do one query will likely cut the processing time to a fraction of what it is now.
-
Please don't stoop to denigrating remarks to cover up your ineptitude for which you were called out on.
-
You know, if you were to tell us what you are trying to achieve you will save yourself and us a lot of time. You should NEVER run queries in loops. You can accomplish everything you want by running just a single query! $query = "INSERT INTO cart (price, product123, quantity, cart_id, product_id, academy, orderable, category) SELECT `price`, `product`, '1', '{$_SESSION['complete']}', `id`, '{$_SESSION['academy']}', `orderable`, `category` FROM products WHERE city='{$city}' AND package='Breaking'"; $result = mysql_query($query) or die(mysql_error());
-
Help with PHP MySQL percentage reports with loops?
Psycho replied to malakiahs's topic in MySQL Help
You are correct, that is inefficient. But he solution has nothing to with loops or array - you should never run queries in loops and you should not need to run that many queries. the problem is your data structure. You should have a table for the records - which holds just the basic data for the records. You example only shows a record ID, but I would assume you might have other data such as user_id, date, etc. But you should NOT store the results of the questions. You should do that in a separate table where each record is the result of ONE question results record_id | question_id | result record_id | question_id | result 1 1 5 1 2 5 1 25 4 2 1 4 2 2 5 2 25 6 You can then easily create one query to get the count and another to get the count for each number of each question. -
I think I understand what you are trying to and it makes even less sense. If I understand you correctly you want to loop through the database records and create an array, e.g. $price, of all the price values from the database result set. Ok, there are a few things wrong with that: 1. You are not defining/setting an array. You are simply setting a single var. You should define the variable as an array $price = array(); THEN you would add the values as a new index in the array $price[] = $row['price']; 2. If you want to define arrays for each field in the result set then you would NOT run the foreach() inside the while() loop. You would want to run the while() loop to put all the records from the result set into arrays. Once you have all the arrays THEN you would exit the while loop and run the foreach() loop. 3. In the foreach() loop you are only reassigning values to those variable and not doing anything with the values and they are getting overwritten on each iteration - so the foreach() loop is pointless. But, for the sake of argument, let's say you want to put the values from the DB result set into different array values and then do a foreach(0 to do something with those values. That's a waste of code. You are already iterating through the results in the while() loop. So, whatever you want to do with that data do it within the while loop. To use the while loop to dump the data into arrays only so you can do a foreach() loop is redundant.
-
Those variables $row['category'], $row['price'], etc. will be the values from those fields for the current record. How are you expecting them to be arrays?
-
You simply put a foreach() within a while() loop. I think the problem is not the foreach()/while() but in what you are doing with the. The first part of the code in the while() loop is defining some variables. But you do nothing with them. Then you have a foreach() loop which also defines some variables - one of which is overwriting the previous variable "$product". But, what really makes no sense is how you are trying to define the variables in the foreach() loop. I really have no idea what you are trying to accomplish. The code inside the foreach() loop is trying to define variables based upon an index of the variables defined at the beginning of the while loop while($row = mysql_fetch_array($sql)) { $id=$row['id']; //This will be a string $category = $row['category']; //This will be a string $price = $row['price']; //This will be a string $product = $row['product']; //This will be a string $order = $row['orderable']; //This will be a string foreach($product AS $key => $val) { $product = $val; $category1 = $category[$key]; //The $category var is a string, not an array $price1 = $price[$key]; //The $price var is a string, not an array $id1 = $id[$key]; //The $order var is a string, not an array $order1 = $order[$key]; //The $order var is a string, not an array } } But, those values at the beginning of the while() loop are apparently from a result set from a DB query. A record from a DB result is an array, but the values in that array will be strings. So, there is not index value for those items.
-
Problem with update process after adding session
Psycho replied to vianca12's topic in PHP Coding Help
Rather than post complete files expecting us to read through them and try to debug int he dark, it would be helpful for you to do some debugging first to identify where the error is occurring and provide the results of your findings along with the *relevant* code snippets. You didn't even explain what specifically *is* happening and what *should* be happening. Debugging is a skill you need to learn. Your code will have a process flow, typically with different branches (e.g. if/else type conditions). You simply need to follow the process flow in your code and include output to the browser to verify input/output values (especially those that affect conditional statements) as well as output within the condition blocks as well. -
Deleting a list and telling user Delete was successful!
Psycho replied to AibZilla's topic in PHP Coding Help
You are calling a function DeleteList() to perform the delete process. That function is returning a value $DeleteSuccessful which only tells you if the first delete 'lists' is successful. It doesn't tell you if the second delete 'items' is successful. You then use that return value to do one of two redirects if ($deleteResult) { // return to list page with success message header("Location: /index.php?message=Deleted List"); } else { // return to list page with failure message header("Location: /index.php?message=Sorry No Dice"); } In the success scenario, you are redirecting the user to index.php with the optional URL parameter 'message' set to 'Deleted List'. First of all I would change that value, remove the space, or urlencode() the value. For the sake of this argument I will assume the value is changed to 'DeletedList'. So, all you need to do in your index.php page is see if that url parameter is set and has that value. if(isset($_GET['message']) && $_GET['message']=='DeleteList') { echo "The list was successfully deleted."; } -
Ok, the problem is that those functions are echoing content to the page rather than returning a value. You should typically not build functions that output content and instead they should return the content. That a general programming standard - not a PHP thing. Using periods, as I did in an echo concatenates strings and would have worked if the functions were returning a value. The reasons the commas work is that the echo statement is executing on each value independently. But, it is not doing what you think it is doing - even if it is working For example, this line: echo "<span class='sub'>Objavljeno " ,the_time('j. F Y'), "</span>\n"; The echo is first outputting "<span class='sub'>Objavljeno " Then it executes the_time('j. F Y') and tries to echo the result. But, the function is actually echoing something and the result is a null string - so the echo is doing nothing on that. Lastly the echo is outputting "</span>\n" not to be too critical, but that is really sloppy, IMHO. But getting it working is the first battle.
-
I see some problems in that code: It is generally not advised to use "global". Since you are defining $post in the foreach loop, it really makes no sense why you are setting it as global. The only thing I can assume is that the other functions that are called [the_title(), the_time() and the_content()] are using the current value of $post. But, that's a pretty poor implementation. You should instead pass the appropriate value of the $post array within those functions. E.g. the_title($post['some_index']) Also, why do you have a three-dimensional array, $args, where the 2nd level only contains only element - another array. Seems you shoudl only need a two dimensional array. As to your problem, I would use array_chuck to split the $myposts array into the sections matching the number you want. Then do a nested foreach loop. The outer loop is for each group of four and the inner loop is for the individual records. $post_data = array_chunk($myposts, 4); foreach($post_data as $myposts) { echo "<div class='one-fourth-novicke'>\n"; foreach($myposts as $post) { setup_postdata($post); echo " <h6 class='line-divider-novicke'>" . the_title() . "</h6>\n"; echo " <span class='sub'>Objavljeno " . the_time('j. F Y') . "</span>\n"; echo " <p>" . the_content() . "</p>\n"; } echo "</div>\n"; }