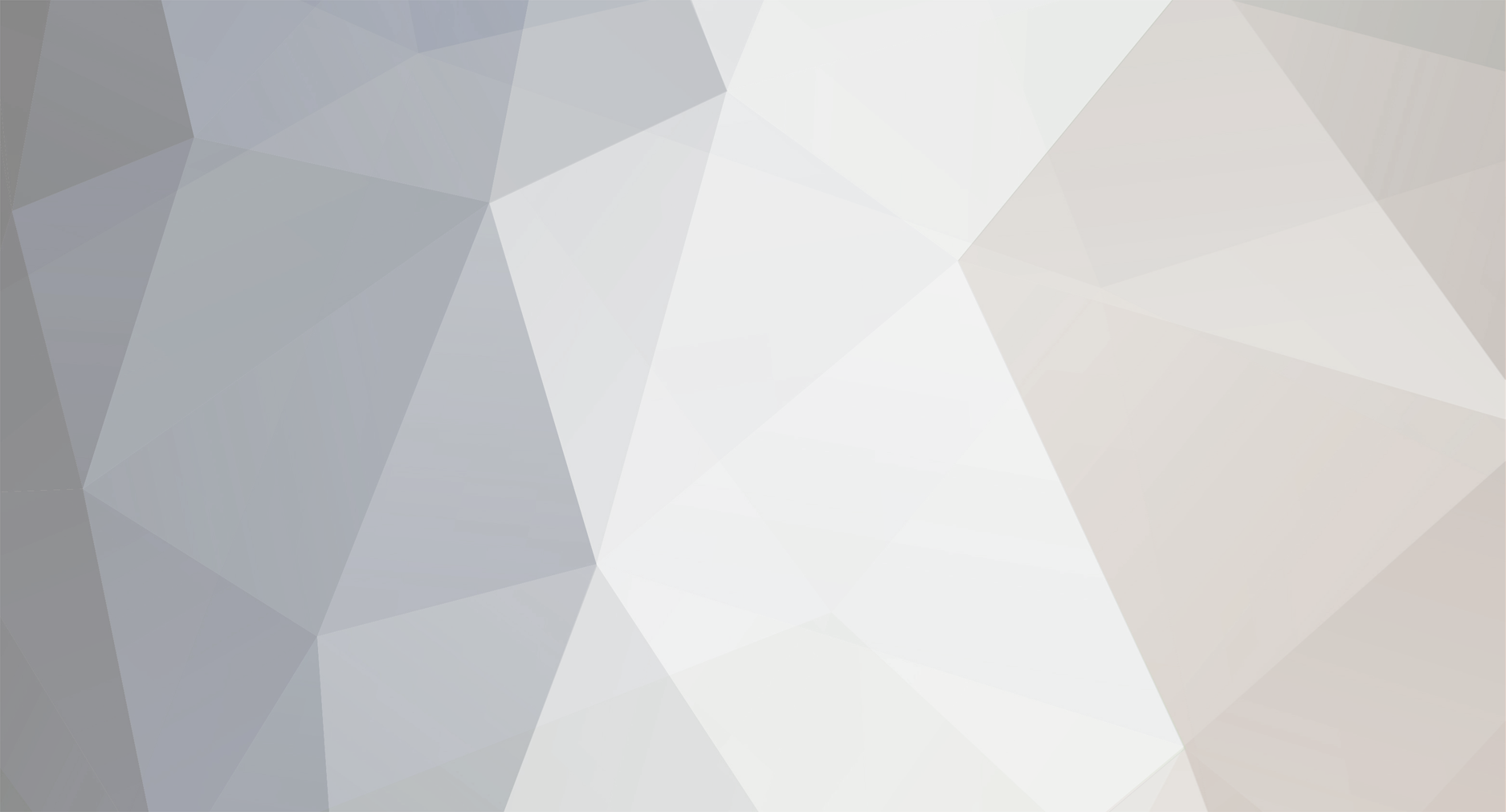
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Could you please provide some details about your experience that qualifies your response? Creating your own hashing method will not be more secure than one that is open source which hundreds, if not thousands, of people with advanced experience have reviewed for flaws. Suggesting someone with little to no experience to build their own hashing method is inadvisable IMO.
-
Pivot Table-like Output (indefinite rows and columns) From 'Flat Data'
Psycho replied to harcheng's topic in PHP Coding Help
So you have a table where each record has an explicit value for the row and column? Then it's very simple: $query = "SELECT * FROM table ORDER BY Row, Col"; $result = mysql_query($query); echo "<table>\n"; $column = false; while($row = mysql_fetch_assoc($result)) { //Detect change in column if($column != $row['Col']) { //If not first column, close the previous if($column != false) { echo "</tr>\n"; } //Open new column echo "</tr>\n"; } //Create data cell TD echo "<td>{$row['name']}({$row['id']})</td>\n"; } //Close last row and table echo "</tr>\n"; echo "</table>\n"; -
You are right, there are a lot of discussions on this. Which is why we encourage people to search for related posts before creating a new one. First off you "hash" passwords, not "encrypt" them. Encrypting implies you can unencrypt. A hash cannot be unencrypted. But, here is an existing post on the subject that should hopefully answer some of your questions as well as provide a solution that will be as secure as you need (PHPass). http://www.phpfreaks.com/forums/index.php?topic=358612.msg1695040#msg1695040
-
In this particular case you do not need to worry about SQL injection because you are not using any user defined values in the query. You are only using the user defined values to set the additional WHERE conditions which are hard-coded and safe (as they are currently written).
-
Yes. As for not being able to show the code for "security reasons", that a stupid excuse. You can easily change DB field names, remove DB credentials, etc. in order to show the code that produces the array. jesirose already pointed out that there are examples in the manual to do what you ask on the multi-dimensional array if you want to take that approach which, to me, is the wrong solution.
-
Good catch
-
As I stated previously, if you are dynamically building the array, then most likely the place to implement the fix will be where you build the array. Sow that code.
-
pbs is correct. I think a better question is why you have similar data types categorized differently. Why doesn't your original array look like this? $frnd1 = array( 0=> 'arfan', 1=> 'haider', 2=> 'azeem', 3=> 'jeme', 4=> 'haider', 5=> 'one' ) In fact, I have to assume that array is built dynamically, so perhaps the best place to solve this is where the array is created.
-
Your structure makes no sense. Don't create columns such as cid, cid1, & cid2 where you will set the same value. Instead create columns called bi, print & online then use a 0 or 1 to indicate if that record belongs to that type or not. Then do the same for your checkboxes: name them bi, print & online and just give them a value of 1. Then the URL will look something like this: index1.php?bi=1&online=1 Now, in your processing script you could do something such as this $whereClauses = array(); if(isset($_GET['bi'])) { $whereClauses = "bi=1"; } if(isset($_GET['print'])) { $whereClauses = "print=1"; } if(isset($_GET['online'])) { $whereClauses = "online=1"; } $query = "SELECT * FROM `$db_table`"; if(count($whereClauses)) { $query .= " WHERE " . implode(" AND ", $whereClauses); }
-
You don't need to do anything in PHP, simply set up the field in your database to automatically set the timestamp when the record is created. You can do this through the DB tools you have (e.g. PpMyAdmin), or you can run a query to modify the field such as ALTER TABLE `HelperFormData` CHANGE `timestamp` `timestamp` TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP
-
Why are you going to replace numbers? And, what are you going to replace them with?
-
Your search string is looking for the literal string " a-zA-Z0-9\:\/\-\?\&\.\=\_\~\#\'" - NOT those characters. You could put those characters in a character class to do what you intended [ a-zA-Z0-9\:\/\-\?\&\.\=\_\~\#\], but there is a much better way. Use a negative character class to look for any characters that are not a double quote. Also you should also "capture" the text before the replacement so you can put it back in. Otherwise you will lose the class parameter text (unless you were to hard code it in the replacement). $pattern = '#(headerTinymanPhoto uiProfilePhotoLarge img" src=")([^"]*)#'; $replacement = '${1}http://example.com/FC.jpg'; $page_html = preg_replace($pattern, $replacement, $page_html);
-
It's as simple as running a SELECT query using a WHERE clause such as WHERE prod_name='$new_name' then checking if the number of results [i.e. mysql_num_rows()] is greater than 0. However, there is another solution as well. Set the name field as unique. Then skip the process of checking for duplicates and just run the INSERT statement with a "ON DUPLICATE IGNORE" clause. If the insert query will cause a duplicate entry in a unique field the INSERT will not take place. Then, after you run the insert query you can check mysql_affected_rows() to see if the record was inserted or not. If yes, then it was a new record and you can show the success message. If not, then you can display a message that the record was a duplicate.
-
update a field in a record when value selected from dropdown list
Psycho replied to davids_media's topic in PHP Coding Help
Maybe I wasn't understanding your question then. Is your issue that you are unsure of how to create the options for the select list? Or, what specifically are you having problems with? -
update a field in a record when value selected from dropdown list
Psycho replied to davids_media's topic in PHP Coding Help
It looks like your form is only populating a single option in the category select list - so the user would never be able to change it anyway. But, assuming you have multiple categories available and the VALUE of the options is the ID, then you would just add that POST value to your UPDATE query: $id = intval($_POST['prodID']); $product = mysql_real_escape_string(trim($_POST['product'])); $descr = mysql_real_escape_string(trim($_POST['prod_descr'])); $price = floatval($_POST['price']); $stock = $_POST['stock']; $catID= intval($_POST['category']); // Update data $query = "UPDATE product SET product='$product', prod_descr='$descr', price='$price', stock='$stock', $category='$catID' WHERE prodID = '$id'"; $update = mysqli_query($dbc, $query); header( 'Location: update_save.php' ) ; That is just an example since I don't know the name of your category field in the DB. Also, you need to sanitize user input before uysing in a query so I added some code for that. -
that would be what the sort() was for.
-
With all due respect this seems like a perfect scenario for array_chunk() $numbers = range(1, 49); shuffle($numbers); $picks = array_chunk($numbers, 7); foreach($picks as $pick) { sort($pick); echo implode(', ', $pick) . "<br>\n"; } As for this: How many picks will exist in the list? As the list grows the chances of matches will increase. Over time the number of iterations needed to find a result with no matches will grow.
-
Add this after the while loop echo "Total: {$total}<br><br>\n";
-
Many times syntax errors occur on some line before the reported error. It is just the line that the parser can no longer continue that it shows the line number. In this case the actual error was on the immediately preceding line where I forgot to end with a semi-colon. None of the code I provided is difficult/advanced. It is pretty basic logic. If you want more information I would ask tat you put some time into trying to understand it then ask specific questions about what you don't understand.
-
You only want ONE query. You will then check for changes in the order number when processing the results and output the necessary content. You show a total in your example output but did not provide the field name that this would come from. So, I used a field called 'cost' in the query. Modify it as needed mysql_select_db($database_lol, $lol); $query = "SELECT ordre.ono, ordre.vare, order.cost FROM ordre WHERE ordre.bruker='{$_SESSION['MM_Username']}' ORDER BY ono"; $result = mysql_query($query_ono, $lol) or die(mysql_error()); $current_order_no = false while($row = mysql_fetch_assoc($result)) { //Check if this is a new order if($current_order_no !== $row['ono']) { if($current_order_no !== false) { //Display previous total echo "Total: {$total}<br><br>\n"; } $total = 0; $current_order_no = $row['ono']; echo "Ordernumber: {$row['ono']}<br>\n"; echo "Products:<br>\n"; } echo " {$row['ono']}<br>\n"; $total += $row['cost']; }
-
Yeah, that's right. The code I provided replaces EVERY fifth letter because that is EXACTLY what you requested. I guess I was supposed to assume you wanted something different than what you asked for? If this is not what you want I hope someone else is willing to help you because I won't $newstring = substr_replace($input, 'X', 4, 0);
-
I'm not sure I understand what you are asking. If you just want to order the results by a timestamp then do just that SELECT * FROM table ORDER BY timestamp_field If that's not what you want perhaps an example of some database records and the results you are trying to achieve
-
No, you could not solve that using just str_replace(). You *may* be able to use preg_replace(). But, you need to clarify your requirement. Are yu wanting to replace every fifth LETTER or every fifth CHARACTER? There is a bug difference. The former is definitely more difficult. You can accomplish the latter with $replaced = preg_replace("#(.{4}).#", '${1}X', $input);
-
Here's a simpler solution if (isset($_POST['calc'])) { $m = trim($_POST['mat']); $e = trim($_POST['da']); $s = trim($_POST['eng']); $f = trim($_POST['fys']); $h = trim($_POST['bio']); $p = trim($_POST['geo']); $count = ($m!=''?1:0) + ($e!=''?1:0) + ($s!=''?1:0) + ($f!=''?1:0) + ($h!=''?1:0) + ($p!=''?1:0); $ave = ($m+$e+$s+$f+$h+$p) / $count; } Although, for the purposes of scalability I would put the POST fields into an array. E.g. $_POST['vars']['mat'], $_POST['vars']['da'], etc. The code then could be simplified even further and would work with any number of fields without any modifications needed. if (isset($_POST['calc'])) { $total = 0; $count = 0; foreach($_POST['vars'] as $value) { $value = trim($value); $total += floatval($value); if($value!=0) { $count++; } } $ave = $total / $count; }
-
Here is a page that give a brief, less technical explanation. http://thomashunter.name/blog/mysql-replace-vs-insert-on-duplicate-key-update/ I don't think a forum is the appropriate venue to teach. Do some Googling to research then try it out on some sample tables. Once you've seen it work it will make more sense.